前言
Teamcenter(下面简称TC)的RAC开发,指的是TC的胖客户端开发。 本文将介绍如何通过plugin in project项目实现对TC胖客户端的修改。
工具:
TC12(安装在虚拟机里),
eclipse
一、环境搭建(Target Platform)
1、合并TC和Eclipse的plugins
将【TC下的plugins文件夹】和【eclipse下的plugins文件夹】都复制并合并到一个新的文件夹里,文件位置参考如下
TC: D:\Siemens\Teamcenter12\portal\plugins
eclipse: C:\eclipse\plugins
合并后: 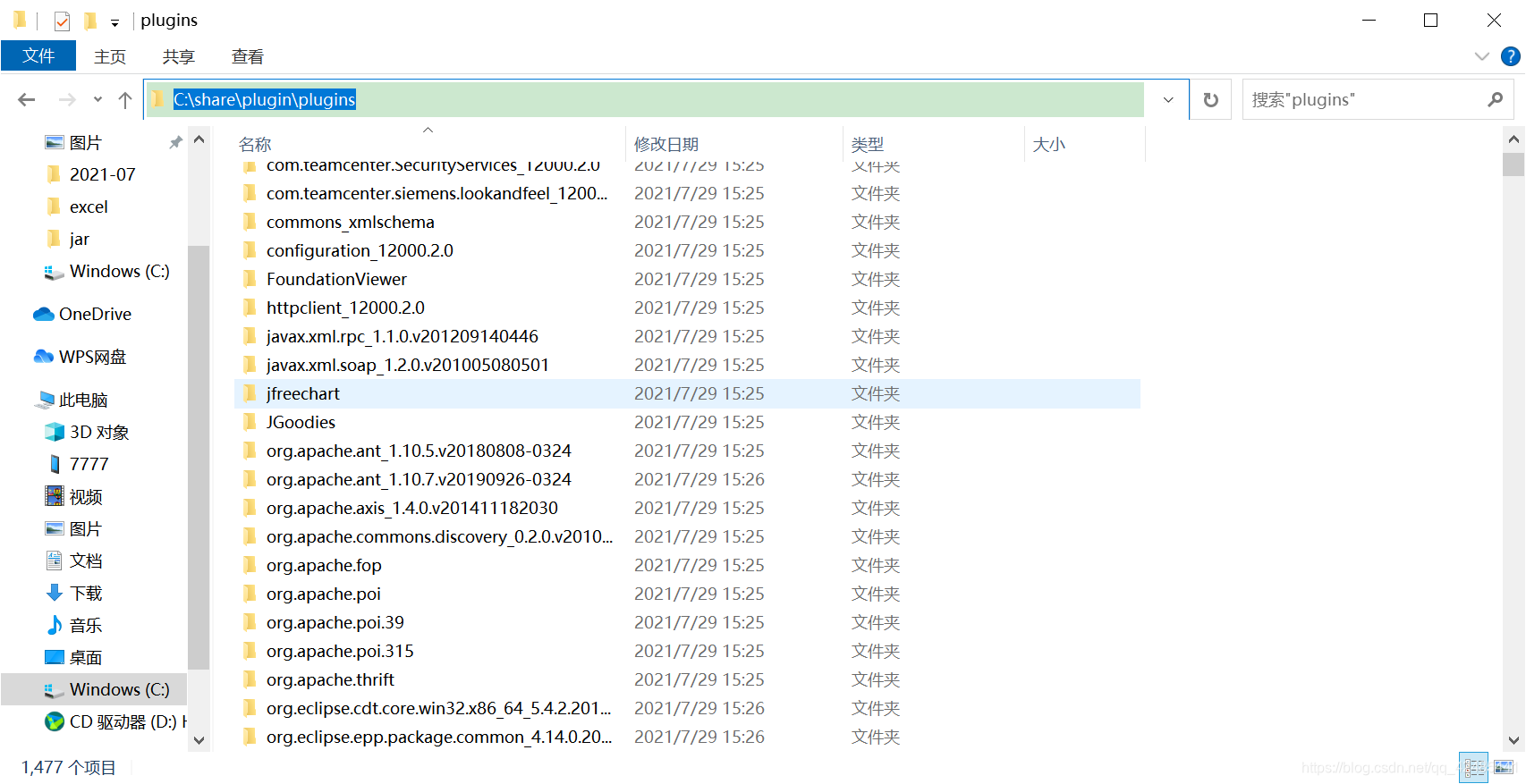
2、eclipse导入plugins并创建Target Platform
将上面合并得到的plugins文件夹导入eclipse并创建Target Platform ,文字步骤如下
打开eclipse -> Window -> Preferce -> Plugin-in Development -> Target Platform -> add
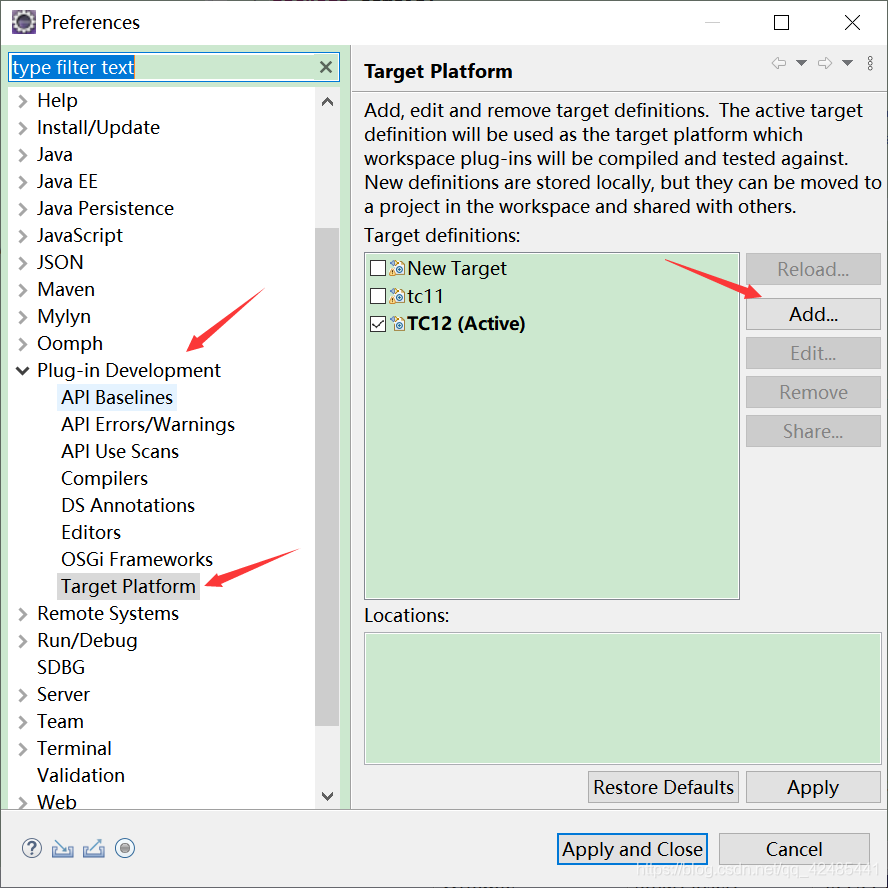
-> 默认选择Nothing:…选项 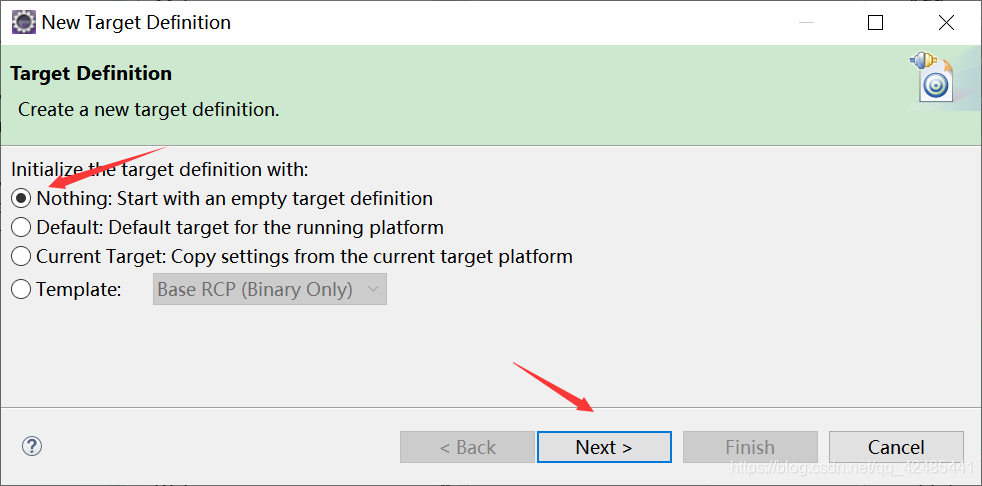
-> 起名 -> add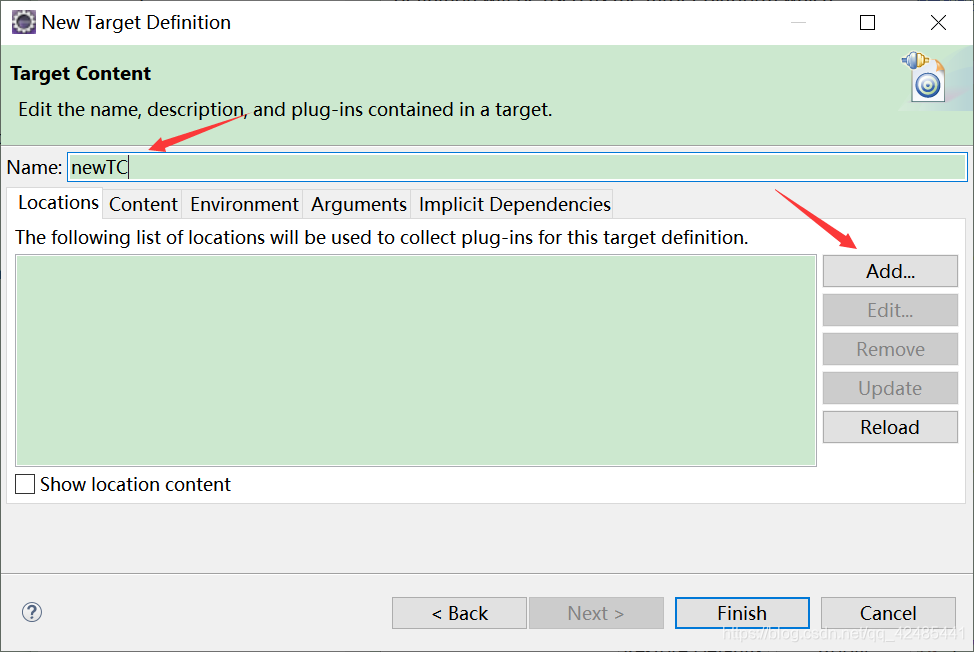
-> 选择Directory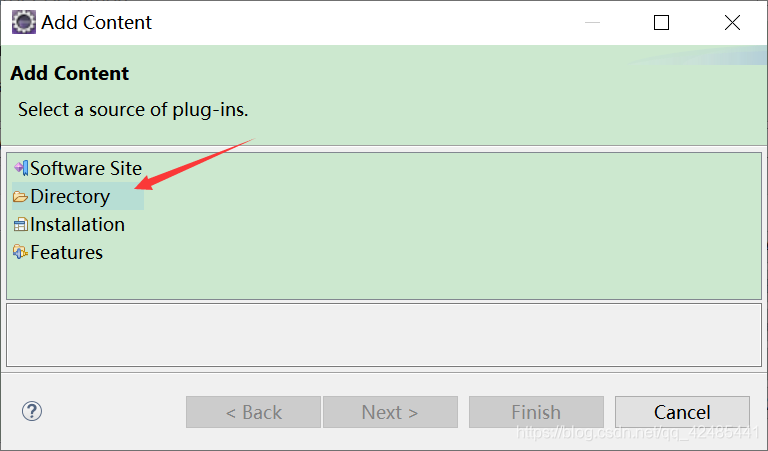 -> 找到tc与eclipse合并好的plugins文件夹 -> next 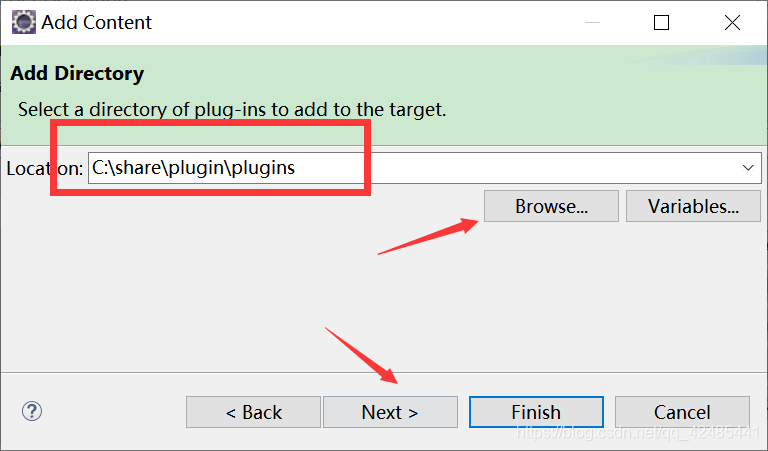
-> finish 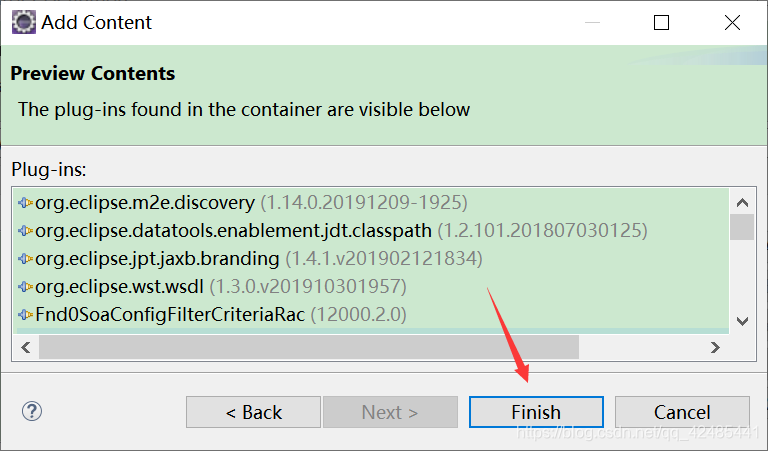
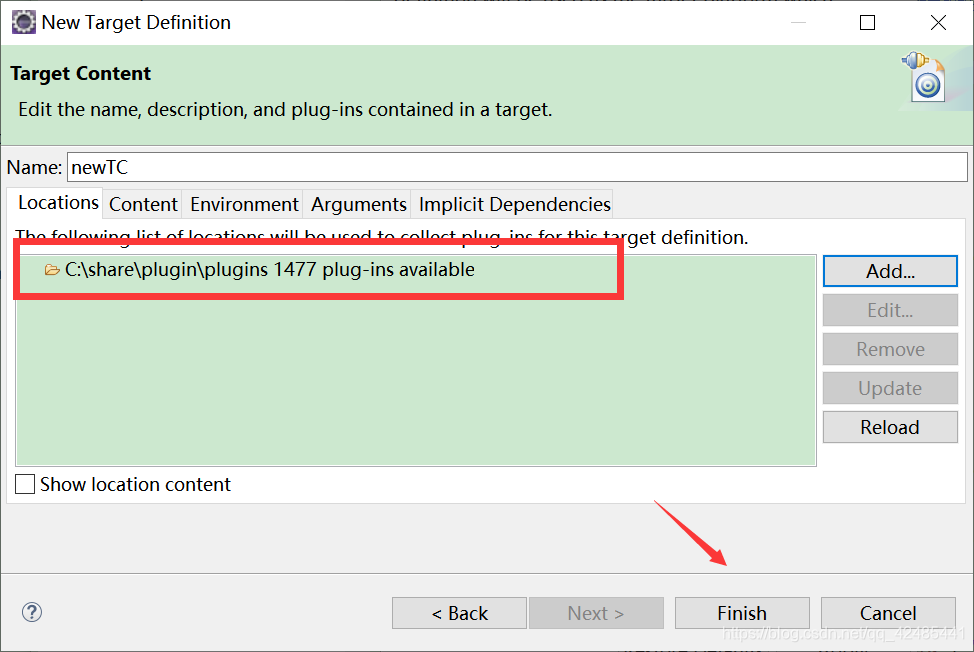
-> 选中新建好的Target Platform -> Apply and close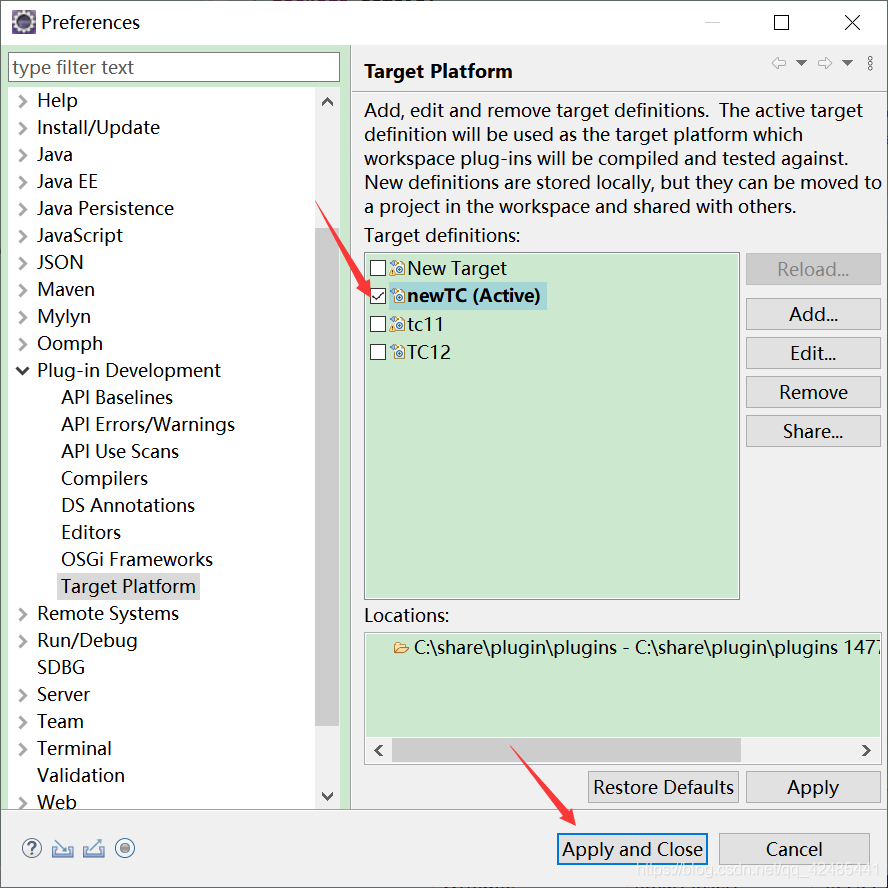
二、搭建Plugin-in Project项目
1、new一个Plugin-in Project项目
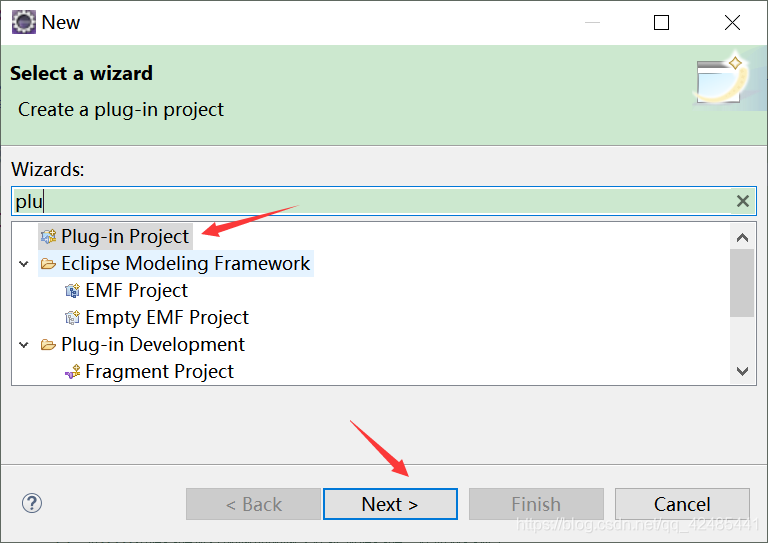 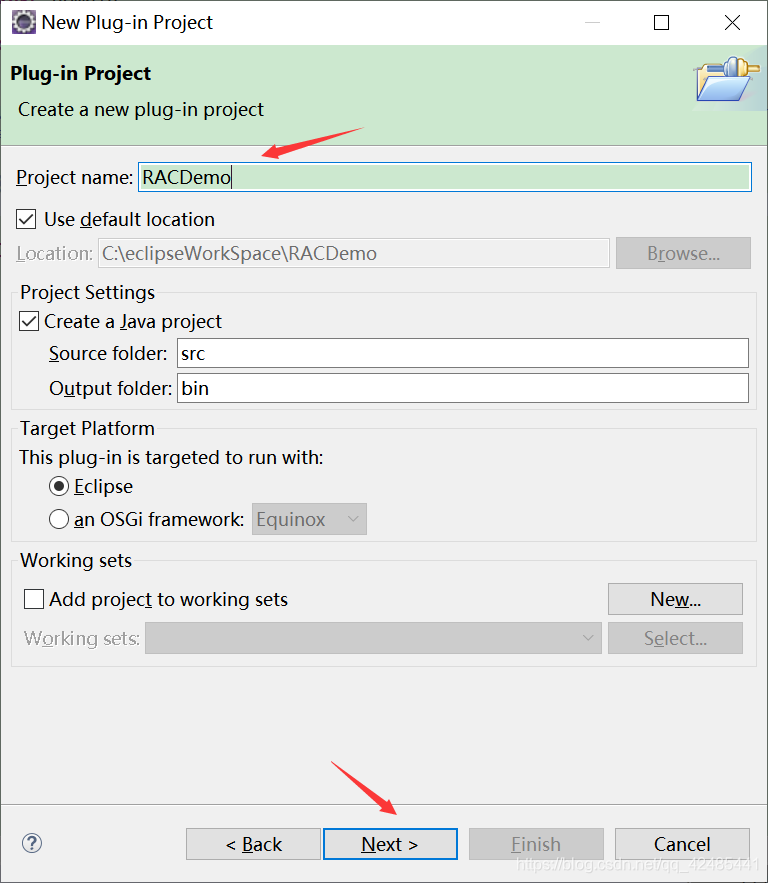 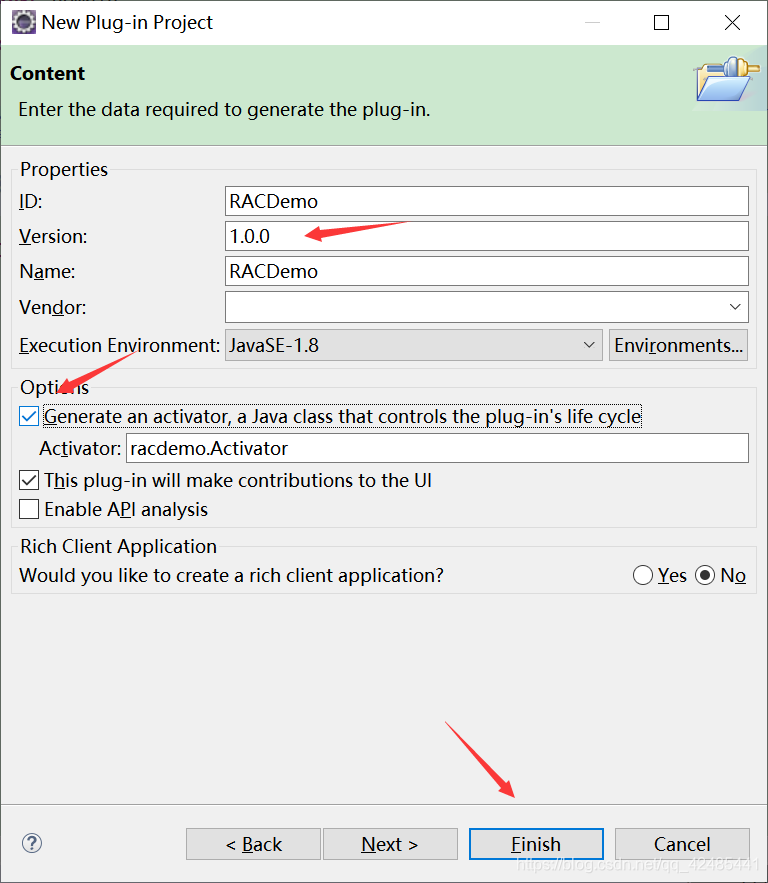
2、生成plugin.xml文件
打开项目,双击MANIFEST.MF文件 -> Extensions -> Add,生成plugin.xml文件 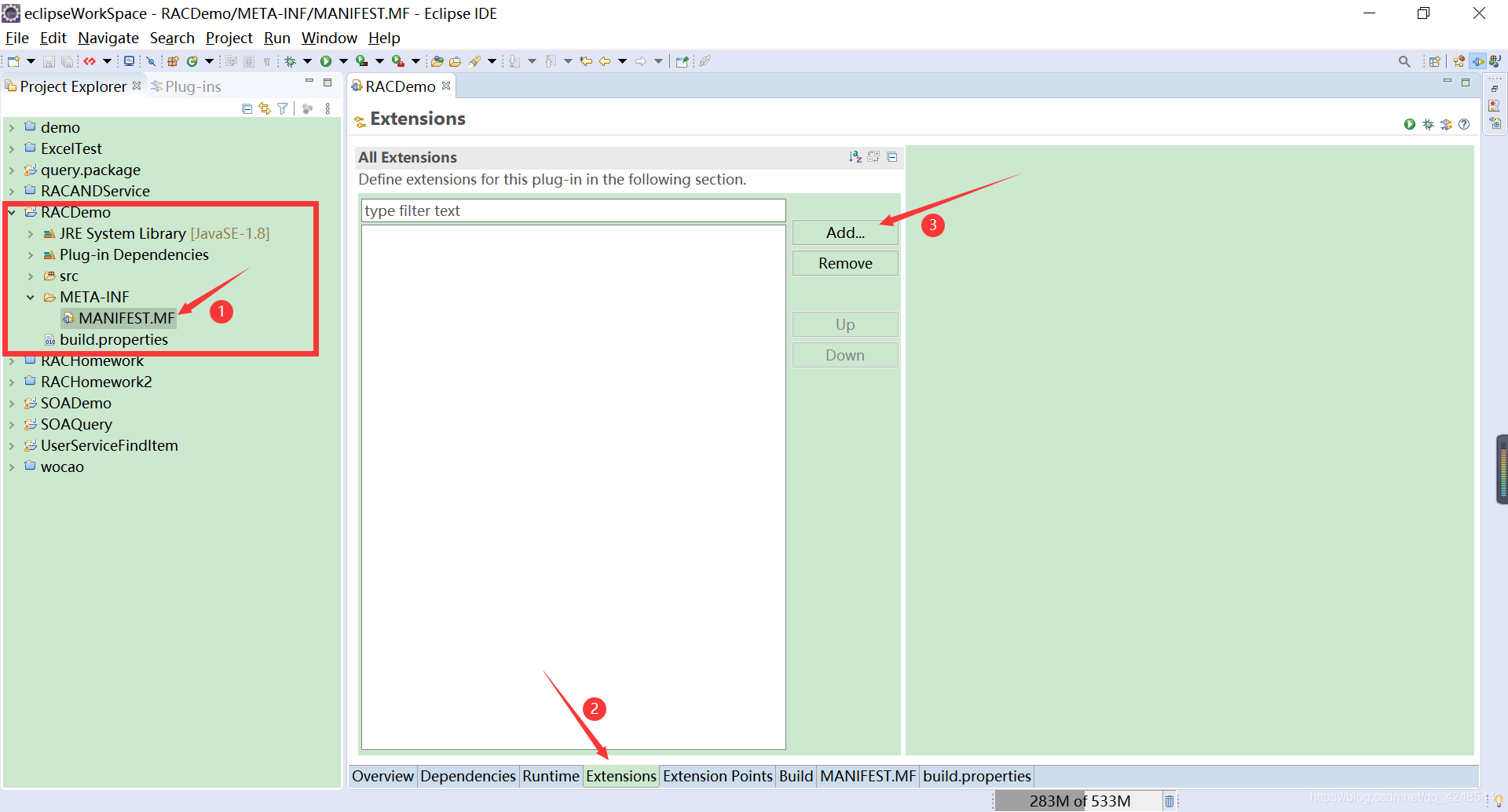 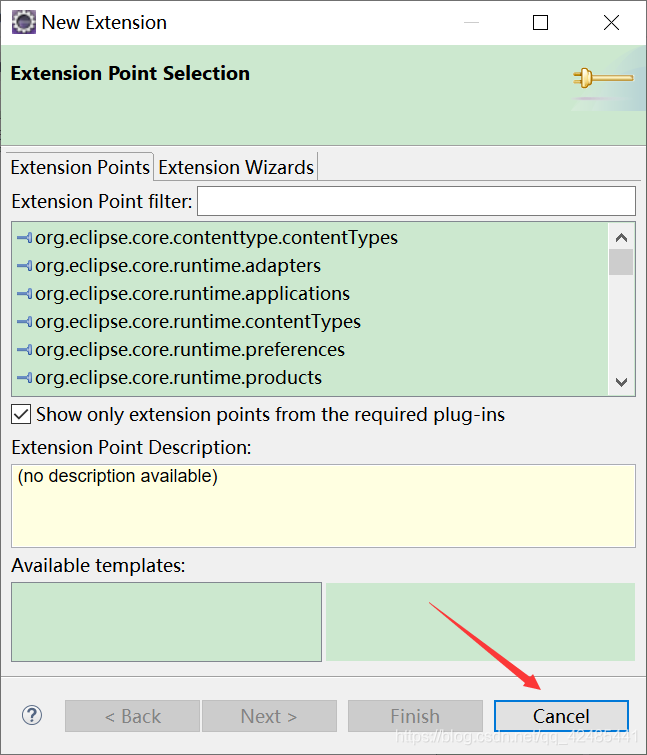 然后会发现项目内新增了一个plugin.xml文件 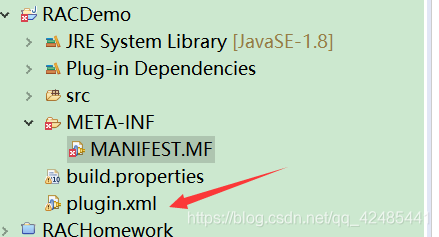 plugin.xml文件主要用来配置关于客户端菜单栏上面的东西,如 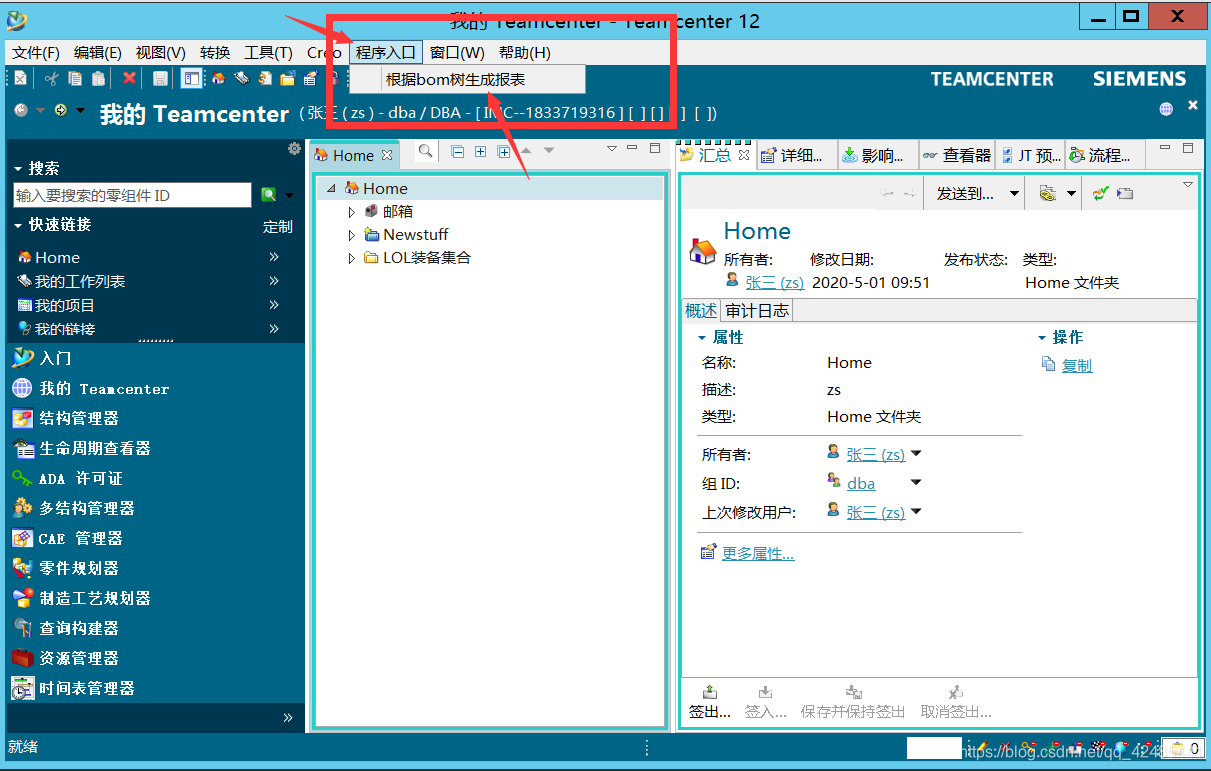
3、编写plugin.xml基础配置
<?xml version="1.0" encoding="UTF-8"?>
<?eclipse version="3.4"?>
<plugin>
<extension point="org.eclipse.ui.menus">
<menuContribution allPopups="false"
locationURI="menu:org.eclipse.ui.main.menu">
<menu id="be" label="程序入口">
<command commandId="lov" id="lov"
label="根据bom树生成报表">
</command>
</menu>
</menuContribution>
</extension>
<extension point="org.eclipse.ui.commands">
<command
id="lov"
name="根据bom树生成报表">
</command>
</extension>
<extension point="org.eclipse.ui.handlers">
<handler
class="domain.MyHandler"
commandId="lov">
</handler>
</extension>
</plugin>
4、导入TC进行RAC开发的核心依赖包
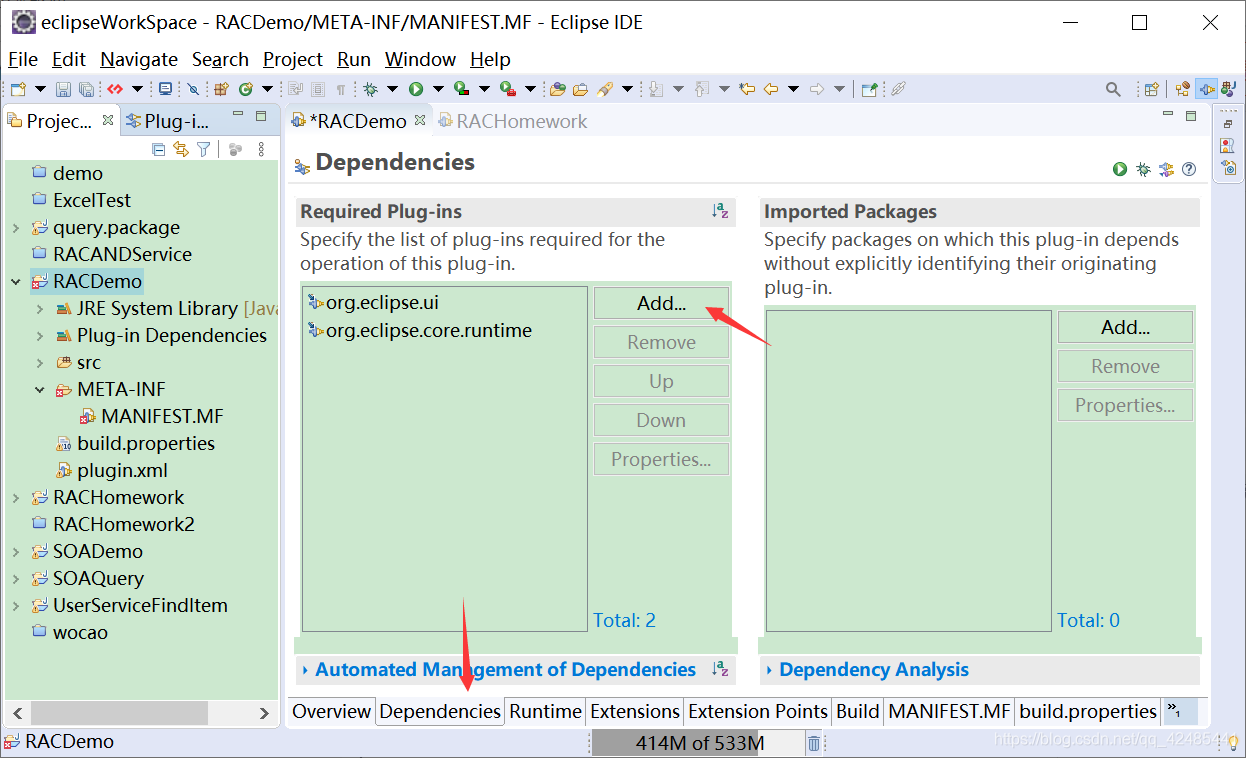
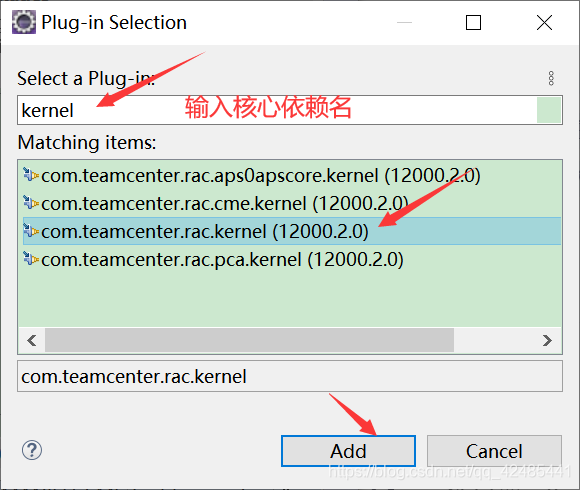 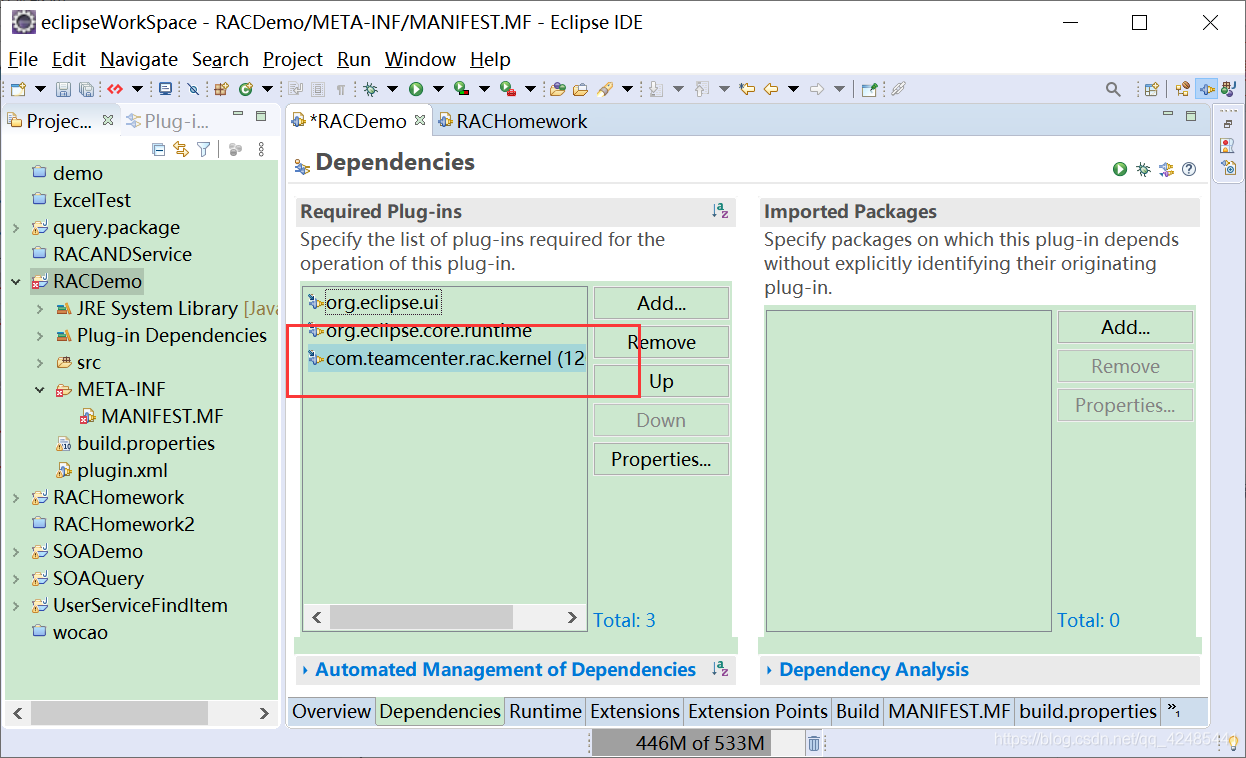
TC进行RAC开发必需的7个核心依赖包:
com.teamcenter.rac.kernel;
com.teamcenter.rac.common;
com.teamcenter.rac.tcapps;
com.teamcenter.rac.util;
com.teamcenter.rac.external;
com.teamcenter.rac.aifrcp;
com.teamcenter.rac.neva;
三、编写代码
1、创建一个类,继承AbstractHandler抽象类
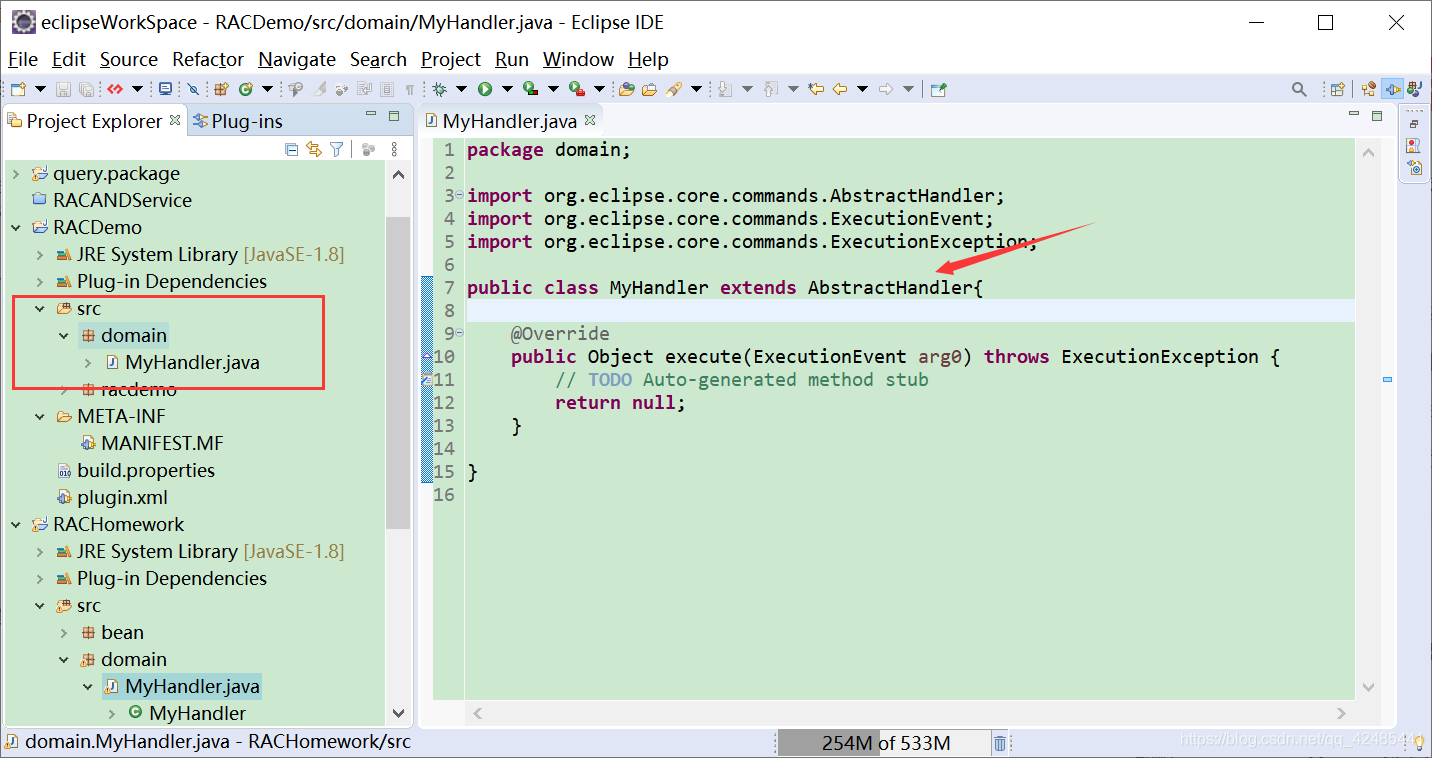
import org.eclipse.core.commands.AbstractHandler;
import org.eclipse.core.commands.ExecutionEvent;
import org.eclipse.core.commands.ExecutionException;
public class MyHandler extends AbstractHandler{
@Override
public Object execute(ExecutionEvent arg0) throws ExecutionException {
return null;
}
}
2、编码
RAC开发的程序入口就是execute方法,可以根据需求在execute方法里进行编码。
四、RAC开发例子
1、需求
遍历TC的一个BOM树,将BOM树里包含的零组件以及零组件版本的一些信息打印到一个excle文件里,并将该excle文件以规范关系挂在顶层BomLine所在的零组件版本下。
2、程序包结构
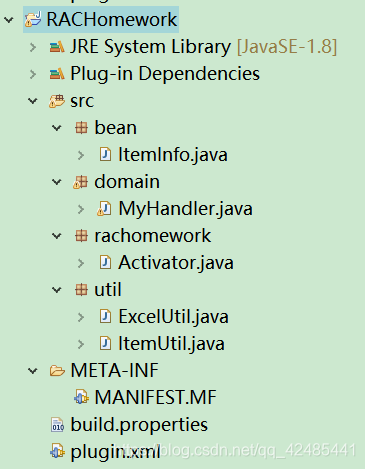
3、代码实现
plugin.xml
<?xml version="1.0" encoding="UTF-8"?>
<?eclipse version="3.4"?>
<plugin>
<extension point="org.eclipse.ui.menus">
<menuContribution allPopups="false"
locationURI="menu:org.eclipse.ui.main.menu">
<menu id="be" label="程序入口">
<command commandId="lov" id="lov"
label="根据bom树生成报表">
</command>
</menu>
</menuContribution>
</extension>
<extension point="org.eclipse.ui.commands">
<command
id="lov"
name="根据bom树生成报表">
</command>
</extension>
<extension point="org.eclipse.ui.handlers">
<handler
class="domain.MyHandler"
commandId="lov">
</handler>
</extension>
</plugin>
ItemUtil.java
package util;
import com.teamcenter.rac.kernel.TCComponentBOMLine;
import com.teamcenter.rac.kernel.TCComponentBOMWindow;
import com.teamcenter.rac.kernel.TCComponentBOMWindowType;
import com.teamcenter.rac.kernel.TCComponentDataset;
import com.teamcenter.rac.kernel.TCComponentDatasetType;
import com.teamcenter.rac.kernel.TCComponentItem;
import com.teamcenter.rac.kernel.TCComponentItemRevision;
import com.teamcenter.rac.kernel.TCComponentItemType;
import com.teamcenter.rac.kernel.TCComponentType;
import com.teamcenter.rac.kernel.TCException;
import com.teamcenter.rac.kernel.TCSession;
public class ItemUtil {
public static TCComponentBOMLine getBOMLine(TCComponentItemRevision revision, TCSession session) {
TCComponentBOMLine bomLine = null;
try {
TCComponentType tccomponentType = session.getTypeComponent("BOMWindow");
if (tccomponentType != null && tccomponentType instanceof TCComponentBOMWindowType) {
TCComponentBOMWindowType bomwindowType = (TCComponentBOMWindowType) tccomponentType;
TCComponentBOMWindow bomwindow = bomwindowType.create(null);
if (bomwindow != null) {
bomLine = bomwindow.setWindowTopLine(null, revision, null, null);
} else {
System.out.println("bomwindow == null");
}
} else {
System.out.println(
"tccomponentType == null || (tccomponentType instanceof TCComponentBOMWindowType == false)");
}
} catch (TCException e) {
e.printStackTrace();
}
return bomLine;
}
public static TCComponentItem querySingleItem(String itemId, String itemType, TCSession session) {
try {
TCComponentType compType = session.getTypeComponent(itemType);
if (compType != null && compType instanceof TCComponentItemType) {
TCComponentItemType TCType = (TCComponentItemType) compType;
TCComponentItem[] items = TCType.findItems(itemId);
if (items != null && items.length > 0) {
for (int i = 0; i < items.length; i++) {
String type = items[i].getType();
if (type.equals(itemType)) {
return items[i];
}
}
} else {
System.out.println("items == null || items.length <= 0");
}
} else {
System.out.println("compType == null || (compType instanceof TCComponentItemType == false)");
}
} catch (TCException e) {
e.printStackTrace();
}
return null;
}
public static TCComponentDataset createDataset(String dtType, String dtName, String dtDesc, TCSession session) {
TCComponentDataset dataset = null;
try {
TCComponentType tctype = session.getTypeComponent(dtType);
if (tctype != null && tctype instanceof TCComponentDatasetType) {
TCComponentDatasetType tcDtType = (TCComponentDatasetType) tctype;
dataset = tcDtType.create(dtName, dtDesc, dtType);
}
} catch (TCException e) {
e.printStackTrace();
}
return dataset;
}
}
ExcelUtil.java
package util;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Date;
import org.apache.poi.xssf.usermodel.XSSFCell;
import org.apache.poi.xssf.usermodel.XSSFRow;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelUtil {
public static String createTempDir() {
String dir = "";
String tempDir = System.getProperty("java.io.tmpdir");
Date date = new Date();
long time = date.getTime();
if (tempDir.endsWith("\\")) {
dir = tempDir + time + "\\";
} else {
dir = tempDir + "\\" + time + "\\";
}
System.out.println("最终的dir == " + dir);
File file = new File(dir);
if (!file.exists()) {
boolean isOk = file.mkdirs();
if (isOk) {
System.out.println("文件目录创建成功!");
} else {
System.out.println("文件目录创建失败!");
}
} else {
System.out.println(dir + "目录已存在!");
}
return dir;
}
public static void writeWBToFile(XSSFWorkbook xwb, String filePath) {
try {
FileOutputStream fos = new FileOutputStream(filePath);
xwb.write(fos);
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void setCellValue(XSSFSheet sheet, int row, int cell, String value) {
XSSFRow myRow = sheet.getRow(row);
if (myRow == null) {
myRow = sheet.createRow(row);
}
XSSFCell myCell = myRow.getCell(cell);
if (myCell == null) {
myCell = myRow.createCell(cell);
}
myCell.setCellType(XSSFCell.CELL_TYPE_STRING);
myCell.setCellValue(value);
}
public static XSSFSheet getExcelSheetFromFilePath(String excelFilePath, int sheetIndex) {
try {
File file = new File(excelFilePath);
FileInputStream fis = new FileInputStream(file);
XSSFWorkbook xwb = new XSSFWorkbook(fis);
XSSFSheet sheet = xwb.getSheetAt(sheetIndex);
fis.close();
return sheet;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
}
ItemInfo.java
package bean;
public class ItemInfo {
private String item_id = "";
private String object_name = "";
private String object_desc = "";
private String bl_sequance_no = "";
private String bl_uom = "";
public ItemInfo() {
super();
}
public ItemInfo(String item_id, String object_name, String object_desc, String bl_sequance_no, String bl_uom) {
super();
this.item_id = item_id;
this.object_name = object_name;
this.object_desc = object_desc;
this.bl_sequance_no = bl_sequance_no;
this.bl_uom = bl_uom;
}
public String getItem_id() {
return item_id;
}
public void setItem_id(String item_id) {
this.item_id = item_id;
}
public String getObject_name() {
return object_name;
}
public void setObject_name(String object_name) {
this.object_name = object_name;
}
public String getObject_desc() {
return object_desc;
}
public void setObject_desc(String object_desc) {
this.object_desc = object_desc;
}
public String getBl_sequance_no() {
return bl_sequance_no;
}
public void setBl_sequance_no(String bl_sequance_no) {
this.bl_sequance_no = bl_sequance_no;
}
public String getBl_uom() {
return bl_uom;
}
public void setBl_uom(String bl_uom) {
this.bl_uom = bl_uom;
}
@Override
public String toString() {
return "ItemInfo [item_id=" + item_id + ", object_name=" + object_name + ", object_desc=" + object_desc
+ ", bl_sequance_no=" + bl_sequance_no + ", bl_uom=" + bl_uom + "]";
}
}
MyHandler.java
package domain;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.FileHandler;
import java.util.logging.Level;
import java.util.logging.Logger;
import java.util.logging.SimpleFormatter;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.eclipse.core.commands.AbstractHandler;
import org.eclipse.core.commands.ExecutionEvent;
import org.eclipse.core.commands.ExecutionException;
import com.sun.mail.handlers.message_rfc822;
import com.teamcenter.rac.aif.AbstractAIFUIApplication;
import com.teamcenter.rac.aif.kernel.AIFComponentContext;
import com.teamcenter.rac.aif.kernel.InterfaceAIFComponent;
import com.teamcenter.rac.aifrcp.AIFUtility;
import com.teamcenter.rac.kernel.TCComponentBOMLine;
import com.teamcenter.rac.kernel.TCComponentDataset;
import com.teamcenter.rac.kernel.TCComponentItem;
import com.teamcenter.rac.kernel.TCComponentItemRevision;
import com.teamcenter.rac.kernel.TCException;
import com.teamcenter.rac.kernel.TCPreferenceService;
import com.teamcenter.rac.kernel.TCSession;
import com.teamcenter.rac.util.MessageBox;
import bean.ItemInfo;
import util.ExcelUtil;
import util.ItemUtil;
public class MyHandler extends AbstractHandler {
private static AbstractAIFUIApplication app = null;
private static TCSession session = null;
private static Logger logger = null;
List<ItemInfo> list = new ArrayList<ItemInfo>();
public Object execute(ExecutionEvent arg0) throws ExecutionException {
logger = getLogger();
app = AIFUtility.getCurrentApplication();
session = (TCSession) app.getSession();
TCPreferenceService preferenceService = session.getPreferenceService();
if (preferenceService != null) {
String[] preValue = preferenceService.getStringValues("my_topBom_id");
if (preValue != null && preValue.length > 0) {
String itemId = preValue[0];
TCComponentItem item = ItemUtil.querySingleItem(itemId, "Item", session);
if (item != null) {
try {
TCComponentItemRevision itemRevision = item.getLatestItemRevision();
TCComponentBOMLine topBomLine = ItemUtil.getBOMLine(itemRevision, session);
list.add(new ItemInfo(itemRevision.getProperty("item_id"),
itemRevision.getProperty("object_name"), itemRevision.getProperty("object_desc"),
topBomLine.getProperty("bl_sequance_no"), topBomLine.getProperty("bl_uom")));
AIFComponentContext[] bomLines = topBomLine.getChildren();
for (int i = 0; i < bomLines.length; i++) {
InterfaceAIFComponent bomline = bomLines[i].getComponent();
if (bomline instanceof TCComponentBOMLine) {
TCComponentBOMLine bl = (TCComponentBOMLine) bomline;
TCComponentItemRevision itemRevision2 = ((TCComponentBOMLine) bl).getItemRevision();
list.add(new ItemInfo(itemRevision2.getProperty("item_id"),
itemRevision2.getProperty("object_name"),
itemRevision2.getProperty("object_desc"),
itemRevision2.getProperty("bl_sequance_no"),
itemRevision2.getProperty("bl_uom")));
recursion(bl);
}
}
String exclePath = "C:\\excel\\bomMessage.xlsx";
XSSFSheet sheet = ExcelUtil.getExcelSheetFromFilePath(exclePath, 0);
if (sheet != null) {
for (int i = 1; i < list.size() + 1; i++) {
ItemInfo itemInfo = list.get(i - 1);
for (int j = 0; j < 6; j++) {
if (j == 0) {
ExcelUtil.setCellValue(sheet, i, j, i + "");
} else if (j == 1) {
ExcelUtil.setCellValue(sheet, i, j, itemInfo.getItem_id());
} else if (j == 2) {
ExcelUtil.setCellValue(sheet, i, j, itemInfo.getObject_name());
} else if (j == 3) {
ExcelUtil.setCellValue(sheet, i, j, itemInfo.getObject_desc());
} else if (j == 4) {
ExcelUtil.setCellValue(sheet, i, j, itemInfo.getBl_sequance_no());
} else if (j == 5) {
ExcelUtil.setCellValue(sheet, i, j, itemInfo.getBl_uom());
}
}
}
XSSFWorkbook workbook = sheet.getWorkbook();
String tempDir = ExcelUtil.createTempDir();
String exortPath = tempDir + itemRevision.getProperty("object_name") + "信息表.xlsx";
ExcelUtil.writeWBToFile(workbook, exortPath);
TCComponentDataset dataset = ItemUtil.createDataset("MSExcelX",
itemRevision.getProperty("object_name") + "信息表", "", session);
dataset.setFiles(new String[] { exortPath }, new String[] { "excel" });
itemRevision.add("IMAN_specification", dataset);
MessageBox.post("恭喜,报表生成成功!", "提示", MessageBox.INFORMATION);
} else {
loginfo("sheet页为空!");
}
} catch (TCException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
} else {
loginfo("获取零组件对象失败!");
}
} else {
loginfo("获取首选项失败!");
}
} else {
loginfo("获取首选项服务失败!");
}
return null;
}
public void recursion(TCComponentBOMLine bomLine) throws Exception {
AIFComponentContext[] bomLines = bomLine.getChildren();
for (int i = 0; i < bomLines.length; i++) {
InterfaceAIFComponent bomline = bomLines[i].getComponent();
if (bomline instanceof TCComponentBOMLine) {
TCComponentBOMLine bl = (TCComponentBOMLine) bomline;
TCComponentItemRevision itemRevision2 = ((TCComponentBOMLine) bl).getItemRevision();
ItemInfo itemInfo = new ItemInfo(itemRevision2.getProperty("item_id"),
itemRevision2.getProperty("object_name"), itemRevision2.getProperty("object_desc"),
itemRevision2.getProperty("bl_sequance_no"), itemRevision2.getProperty("bl_uom"));
list.add(itemInfo);
loginfo(itemInfo.toString());
recursion(bl);
}
}
}
private void loginfo(String info) {
logger.info(info + "\r\n");
}
private Logger getLogger() {
if (logger != null) {
return logger;
}
Logger logger = Logger.getLogger("tang");
logger.setLevel(Level.ALL);
try {
FileHandler fileHandler = new FileHandler("C:\\lidesen.txt");
fileHandler.setFormatter(new SimpleFormatter());
logger.addHandler(fileHandler);
} catch (SecurityException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return logger;
}
}
|