ajax
知识点
express框架(ajax需要框架,需要先安装node) 右击vscode的文件夹空白部分,输入指令npm init --yes(初始化) 编写express.js代码,右键单击该文件夹,选择“集成终端中打开”,输入指令node js文件夹的名字
ajax请求基本操作
- 创建对象
- 初始化,设置请求方法和
url - 发送
- 事件绑定,处理服务端返回的结果
发送get请求
编写相关express框架
const { response } = require('express');
const express = require('express');
const app = express();
app.get('/server', (request, response) => {
response.setHeader('Access-Control-Allow-Origin', '*');
response.send('HELLO EXPRESS');
});
app.listen(8000, () => {
console.log("服务已经启动,8000 端口监听中....")
})
GET.html文件
实现点击“发送”按钮把响应体打印在div内
<button>发送</button>
<div id="result"></div>
#result {
width: 200px;
height: 100px;
border: 1px solid #ccccff;
}
window.addEventListener('load', function() {
const btn = document.querySelector('button');
const div = document.getElementById('result');
btn.addEventListener('click', function() {
const xhr = new XMLHttpRequest();
xhr.open('GET', 'http://127.0.0.1:8000/server?a=100&b=200&c=300');
xhr.send();
xhr.addEventListener('readystatechange', function() {
if (xhr.readyState === 4) {
if (xhr.status >= 200 && xhr.status < 300) {
console.log(xhr.status);
console.log(xhr.statusText);
console.log(xhr.getAllResponseHeaders());
console.log(xhr.response);
div.innerHTML = xhr.response;
}
}
})
})
})
效果
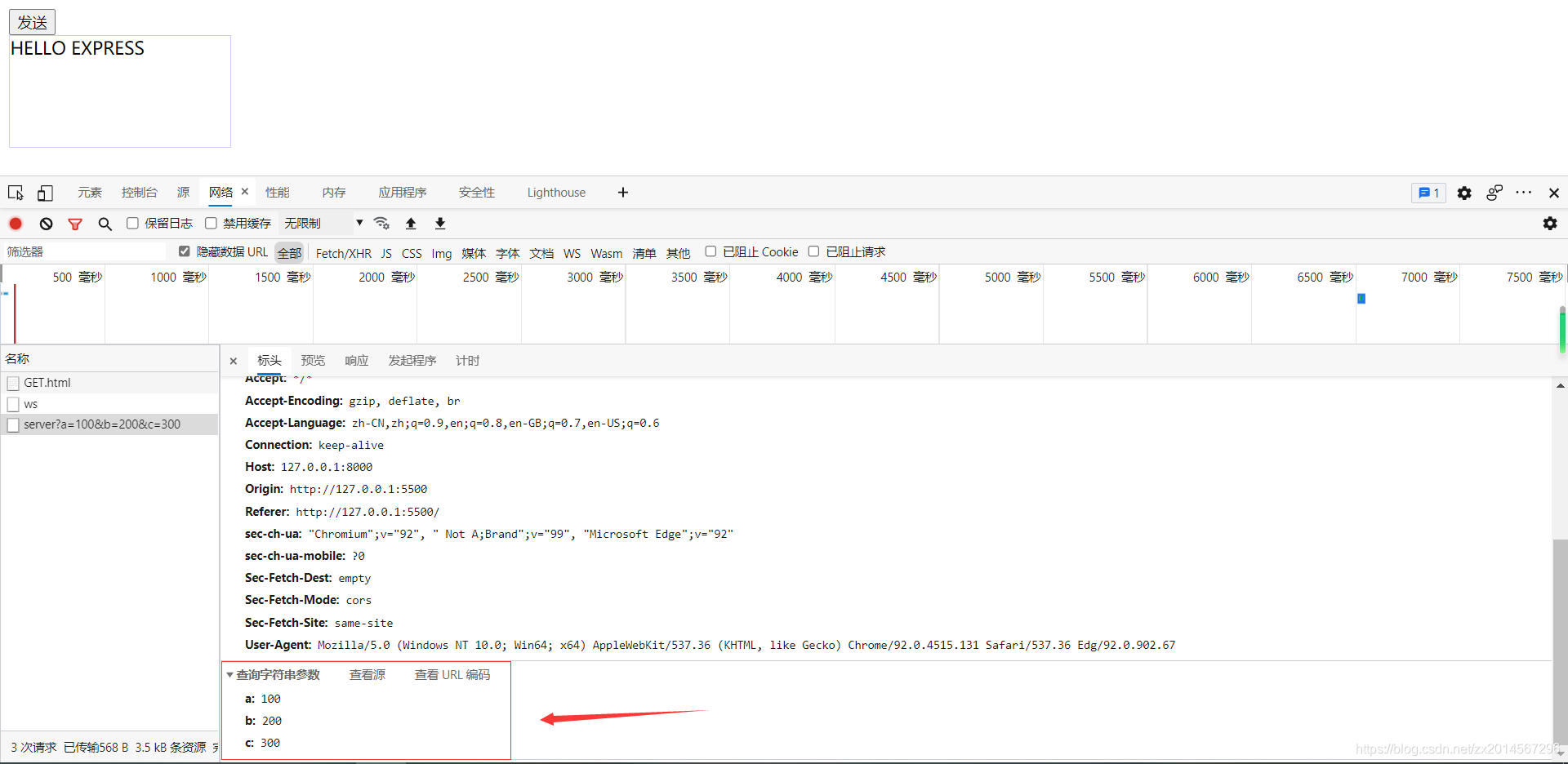
|