1、表结构ddl
MySQL建表如下: 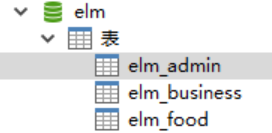
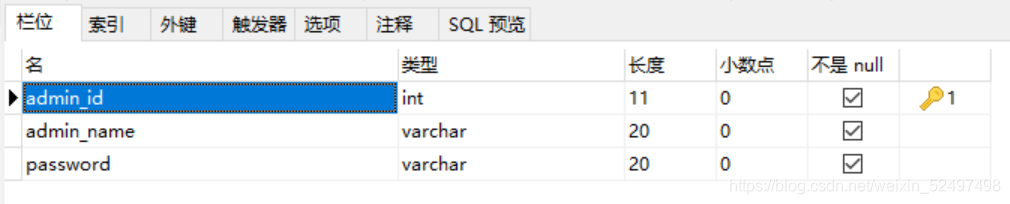 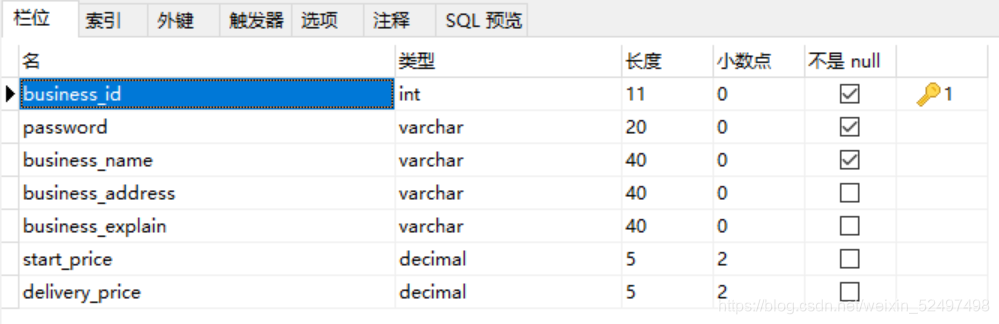 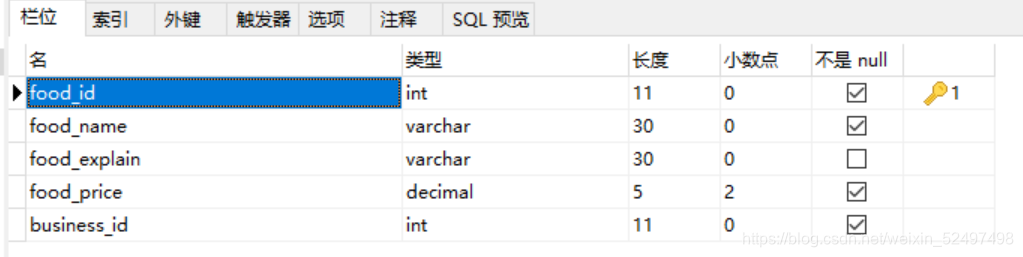
2、项目搭建
2.1 开发工具:STS 2.2 检查开发工具的jdk配置:jdk8 2.3 检查开发工具的文件编码配置:utf-8 2.4 STS内部结构如下: 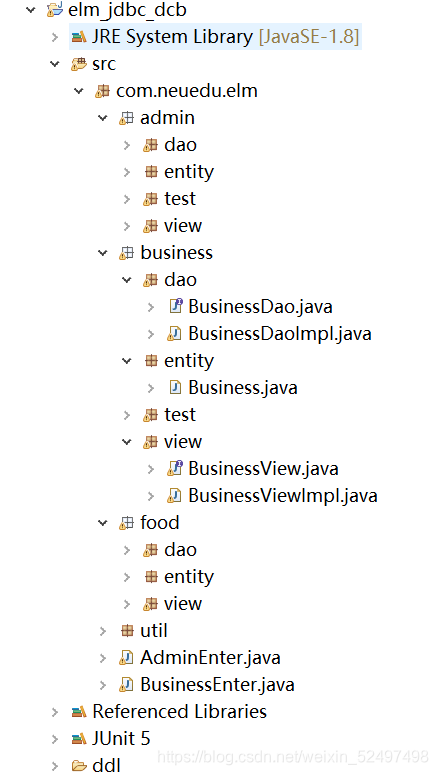
3、Util包
package com.neuedu.elm.util;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class DBUtil {
static {
try {
Class.forName("com.mysql.jdbc.Driver");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public static Connection getConnection() {
String url="jdbc:mysql://localhost:3306/elm?useSSL=false&useUnicode=true&characterEncoding=utf-8";
String username="root";
String password="123456";
Connection conn = null;
try {
conn = DriverManager.getConnection(url,username,password);
} catch (SQLException e) {
e.printStackTrace();
}
return conn;
}
public static void close(Connection conn,Statement st,ResultSet rs) {
try {
if(rs!=null) {
rs.close();
}
if(st!=null) {
st.close();
}
if(conn!=null) {
conn.close();
}
}catch(Exception e) {
e.printStackTrace();
}
}
}
4、项目代码
4.1、Entity层
package com.neuedu.elm.admin.entity;
public class Admin {
private Integer adminId;
private String adminName;
private String password;
public Admin() {
super();
}
public Admin(Integer adminId, String adminName, String password) {
super();
this.adminId = adminId;
this.adminName = adminName;
this.password = password;
}
public Integer getAdminId() {
return adminId;
}
public void setAdminId(Integer adminId) {
this.adminId = adminId;
}
public String getAdminName() {
return adminName;
}
public void setAdminName(String adminName) {
this.adminName = adminName;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Override
public String toString() {
return "Admin [adminId=" + adminId + ", adminName=" + adminName + ", password=" + password + "]";
}
}
package com.neuedu.elm.business.entity;
public class Business {
private Integer businessId;
private String password;
private String businessName;
private String businessAddress;
private String businessExplain;
private Double startPrice;
private Double deliveryPrice;
public Business() {
}
public Business(Integer businessId, String password, String businessName, String businessAddress,
String businessExplain, Double startPrice, Double deliveryPrice) {
super();
this.businessId = businessId;
this.password = password;
this.businessName = businessName;
this.businessAddress = businessAddress;
this.businessExplain = businessExplain;
this.startPrice = startPrice;
this.deliveryPrice = deliveryPrice;
}
public Integer getBusinessId() {
return businessId;
}
public void setBusinessId(Integer businessId) {
this.businessId = businessId;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getBusinessName() {
return businessName;
}
public void setBusinessName(String businessName) {
this.businessName = businessName;
}
public String getBusinessAddress() {
return businessAddress;
}
public void setBusinessAddress(String businessAddress) {
this.businessAddress = businessAddress;
}
public String getBusinessExplain() {
return businessExplain;
}
public void setBusinessExplain(String businessExplain) {
this.businessExplain = businessExplain;
}
public Double getStartPrice() {
return startPrice;
}
public void setStartPrice(Double startPrice) {
this.startPrice = startPrice;
}
public Double getDeliveryPrice() {
return deliveryPrice;
}
public void setDeliveryPrice(Double deliveryPrice) {
this.deliveryPrice = deliveryPrice;
}
@Override
public String toString() {
return businessId
+ "\t|\t" + password
+ "\t|\t" + businessName
+ "\t|\t" + businessAddress
+ "\t|\t" + businessExplain
+ "\t|\t" + startPrice
+ "\t|\t" + deliveryPrice;
}
}
package com.neuedu.elm.food.entity;
public class Food {
private Integer foodId;
private String foodName;
private String foodExplain;
private Double foodPrice;
private Integer businessId;
public Food() {
super();
}
public Food(Integer foodId, String foodName, String foodExplain, Double foodPrice, Integer businessId) {
super();
this.foodId = foodId;
this.foodName = foodName;
this.foodExplain = foodExplain;
this.foodPrice = foodPrice;
this.businessId = businessId;
}
public Integer getFoodId() {
return foodId;
}
public void setFoodId(Integer foodId) {
this.foodId = foodId;
}
public String getFoodName() {
return foodName;
}
public void setFoodName(String foodName) {
this.foodName = foodName;
}
public String getFoodExplain() {
return foodExplain;
}
public void setFoodExplain(String foodExplain) {
this.foodExplain = foodExplain;
}
public Double getFoodPrice() {
return foodPrice;
}
public void setFoodPrice(Double foodPrice) {
this.foodPrice = foodPrice;
}
public Integer getBusinessId() {
return businessId;
}
public void setBusinessId(Integer businessId) {
this.businessId = businessId;
}
@Override
public String toString() {
return "\t|\t" + foodId
+ "\t|\t" + foodName
+ "\t|\t" + foodExplain
+ "\t|\t" + foodPrice
+ "\t|\t" + businessId;
}
}
4.2、Dao层
AdminDao
package com.neuedu.elm.admin.dao;
import com.neuedu.elm.admin.entity.Admin;
import com.neuedu.elm.business.entity.Business;
public interface AdminDao {
public Admin login(Admin a);
}
AdminDaoImpl
package com.neuedu.elm.admin.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import com.neuedu.elm.admin.entity.Admin;
import com.neuedu.elm.business.entity.Business;
import com.neuedu.elm.util.DBUtil;
public class AdminDaoImpl implements AdminDao{
public Admin login(Admin a) {
Connection conn = null;
PreparedStatement st = null;
ResultSet rs = null;
Admin a2 = null;
try {
conn = DBUtil.getConnection();
String sql = "select * from elm_admin where admin_id =? and password=?";
st = conn.prepareStatement(sql);
st.setInt(1, a.getAdminId());
st.setString(2, a.getPassword());
rs = st.executeQuery();
while(rs.next()) {
a2 = new Admin();
a2.setAdminId(rs.getInt("admin_id"));
a2.setAdminName(rs.getString("admin_name"));
a2.setPassword(rs.getString("password"));
}
}catch(Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn, st, rs);
}
return a2;
}
}
BusinessDao
package com.neuedu.elm.business.dao;
import java.util.List;
import com.neuedu.elm.business.entity.Business;
public interface BusinessDao {
public List<Business> selectAll();
public int add(Business b);
public int add(String businessName);
public int delete(Business b);
public int update(Business b);
public Business selectBusinessById(Integer businessId);
public int updateBusinessPassword(Integer businessId,String Password);
public Business login(Business b);
public Business getBusinessById(Integer businessId);
public List<Business> listBusiness(String businessName);
}
BusinessDaoImpl
package com.neuedu.elm.business.dao;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.PreparedStatement;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
import com.neuedu.elm.admin.entity.Admin;
import com.neuedu.elm.business.entity.Business;
import com.neuedu.elm.util.DBUtil;
public class BusinessDaoImpl implements BusinessDao{
Connection conn = null;
PreparedStatement st = null;
ResultSet rs = null;
public int add(String businessName) {
int i = 0;
try {
conn = DBUtil.getConnection();
String sql = "insert into elm_business(password,business_name) values('123', ?)";
st = conn.prepareStatement(sql,PreparedStatement.RETURN_GENERATED_KEYS);
st.setString(1, businessName);
st.executeUpdate();
rs = st.getGeneratedKeys();
if(rs.next()) {
i = rs.getInt(1);
}
}catch(Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn, st, rs);
}
return i;
}
@Override
public int add(Business b) {
int i = 0;
try {
conn = DBUtil.getConnection();
String sql = "insert into elm_business(password,business_name,"
+ "business_address,business_explain,start_price,delivery_price)"
+" values(?, ?, ?, ?, ?, ?)";
st = conn.prepareStatement(sql);
st.setString(1, b.getPassword());
st.setString(2, b.getBusinessName());
st.setString(3, b.getBusinessAddress());
st.setString(4, b.getBusinessExplain());
st.setDouble(5, b.getStartPrice());
st.setDouble(6, b.getDeliveryPrice());
i = st.executeUpdate();
}catch(Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn, st, rs);
}
return i;
}
@Override
public List<Business> selectAll() {
List<Business> list = new ArrayList<>();
try {
conn = DBUtil.getConnection();
String sql = "select * from elm_business";
st = conn.prepareStatement(sql);
rs = st.executeQuery();
while(rs.next()) {
Business b2 = new Business();
b2.setBusinessId( rs.getInt("business_id") );
b2.setPassword( rs.getString("password") );
b2.setBusinessName( rs.getString("business_name") );
b2.setBusinessAddress( rs.getString("business_address") );
b2.setBusinessExplain( rs.getString("business_explain") );
b2.setStartPrice( rs.getDouble("start_price") );
b2.setDeliveryPrice( rs.getDouble("delivery_price") );
list.add(b2);
}
}catch(Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn, st, rs);
}
return list;
}
@Override
public int delete(Business b) {
int i = 0;
try {
conn = DBUtil.getConnection();
String sql = "delete from elm_business where business_id= ?";
st = conn.prepareStatement(sql);
st.setInt(1,b.getBusinessId());
i = st.executeUpdate();
}catch(Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn, st, rs);
}
return i;
}
@Override
public int update(Business b) {
int i = 0;
String sql = "update elm_business set business_name = ?, business_address = ?, business_explain = ?,"
+ " start_price= ?, delivery_price = ? where business_id = ?";
try {
conn = DBUtil.getConnection();
st = conn.prepareStatement(sql);
st.setString(1,b.getBusinessName());
st.setString(2,b.getBusinessAddress());
st.setString(3,b.getBusinessExplain());
st.setDouble(4,b.getStartPrice());
st.setDouble(5,b.getDeliveryPrice());
st.setInt(6,b.getBusinessId());
i = st.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
return i;
}
@Override
public Business selectBusinessById(Integer businessId) {
Business business = null;
String sql = "select * from elm_business where business_id = ?";
try {
conn = DBUtil.getConnection();
st = conn.prepareStatement(sql);
st.setInt(1, businessId);
rs = st.executeQuery();
while (rs.next()){
Business b2 = new Business();
b2.setBusinessId( rs.getInt("business_id") );
b2.setPassword( rs.getString("password") );
b2.setBusinessName( rs.getString("business_name") );
b2.setBusinessAddress( rs.getString("business_address") );
b2.setBusinessExplain( rs.getString("business_explain") );
b2.setStartPrice( rs.getDouble("start_price") );
b2.setDeliveryPrice( rs.getDouble("delivery_price") );
}
} catch (Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn,st,rs);
}
return business;
}
@Override
public int updateBusinessPassword(Integer businessId, String Password) {
int i = 0;
String sql = "update elm_business set password = ? where business_id = ?";
try {
conn = DBUtil.getConnection();
st = conn.prepareStatement(sql);
st.setString(1, Password);
st.setInt(2, businessId);
i = st.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn,st,rs);
}
return i;
}
@Override
public Business login(Business b) {
Business b2 = null;
try {
conn = DBUtil.getConnection();
String sql = "select * from elm_business where business_id = ? and password= ?";
st = conn.prepareStatement(sql);
st.setInt(1, b.getBusinessId());
st.setString(2, b.getPassword());
rs = st.executeQuery();
while(rs.next()) {
b2 = new Business();
b2.setBusinessId(rs.getInt("business_id"));
b2.setPassword(rs.getString("password"));
b2.setBusinessName(rs.getString("business_name"));
b2.setBusinessAddress(rs.getString("business_address"));
b2.setBusinessExplain(rs.getString("business_explain"));
b2.setStartPrice(rs.getDouble("start_price"));
b2.setDeliveryPrice(rs.getDouble("delivery_price"));
}
}catch(Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn, st, rs);
}
return b2;
}
@Override
public Business getBusinessById(Integer businessId) {
Business b = null;
String sql = "select * from elm_business where business_id = ?";
try {
conn = DBUtil.getConnection();
st = conn.prepareStatement(sql);
st.setInt(1, businessId);
rs = st.executeQuery();
while (rs.next()){
b = new Business();
b.setBusinessId(rs.getInt("business_id"));
b.setPassword(rs.getString("password"));
b.setBusinessName(rs.getString("business_name"));
b.setBusinessAddress(rs.getString("business_address"));
b.setBusinessExplain(rs.getString("business_explain"));
b.setStartPrice(rs.getDouble("start_price"));
b.setDeliveryPrice(rs.getDouble("delivery_price"));
}
} catch (Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn,st,rs);
}
return b;
}
@Override
public List<Business> listBusiness(String businessName) {
ArrayList<Business> list = new ArrayList<>();
StringBuffer sql = new StringBuffer("select * from elm_business where 1=1");
if (businessName !=null && !businessName.equals("")){
sql.append(" and business_name LIKE '%"+businessName+"%'");
}
try {
conn = DBUtil.getConnection();
st = conn.prepareStatement(sql.toString());
rs = st.executeQuery();
while (rs.next()){
Business b = new Business();
b.setBusinessId(rs.getInt("business_id"));
b.setPassword(rs.getString("password"));
b.setBusinessName(rs.getString("business_name"));
b.setBusinessAddress(rs.getString("business_address"));
b.setBusinessExplain(rs.getString("business_explain"));
b.setStartPrice(rs.getDouble("start_price"));
b.setDeliveryPrice(rs.getDouble("delivery_price"));
list.add(b);
}
} catch (Exception e) {
e.printStackTrace();
}
return list;
}
}
FoodDao
package com.neuedu.elm.food.dao;
import java.util.List;
import com.neuedu.elm.business.entity.Business;
import com.neuedu.elm.food.entity.Food;
public interface FoodDao {
public List<Food> listFood(Integer BusinessId);
public int add(Food f);
public int update(Food f);
public int delete(Food f);
public Food listFoodById(Integer foodId);
}
FoodDaoImpl
package com.neuedu.elm.food.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
import com.neuedu.elm.food.entity.Food;
import com.neuedu.elm.util.DBUtil;
public class FoodDaoImpl implements FoodDao{
Connection conn = null;
PreparedStatement st = null;
ResultSet rs = null;
private Scanner sc = new Scanner(System.in);
@Override
public int add(Food f) {
int i = 0;
String sql = "insert into elm_food(food_name,"
+ "food_explain,food_price,business_id)"
+" values( ?, ?, ?, ?)";
try {
conn = DBUtil.getConnection();
st = conn.prepareStatement(sql);
st.setString(1, f.getFoodName());
st.setString(2, f.getFoodExplain());
st.setDouble(3, f.getFoodPrice());
st.setInt(4, f.getBusinessId());
i = st.executeUpdate();
}catch(Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn, st, rs);
}
return i;
}
@Override
public int update(Food f){
int i = 0;
String sql = "update elm_food set food_name= ?,food_explain= ?,food_price= ? where food_id= ? and business_id = ?";
try {
conn = DBUtil.getConnection();
st = conn.prepareStatement(sql);
st.setString(1, f.getFoodName());
st.setString(2, f.getFoodExplain());
st.setDouble(3, f.getFoodPrice());
st.setInt(4, f.getFoodId());
st.setInt(5, f.getBusinessId());
i = st.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
DBUtil.close(conn, st, rs);
}
return i;
}
@Override
public int delete(Food f) {
int i = 0;
String sql = "delete from elm_food where food_id= ? and business_id = ?";
try {
conn =DBUtil.getConnection();
st = conn.prepareStatement(sql);
st.setInt(1, f.getFoodId());
st.setInt(2, f.getBusinessId());
i = st.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
DBUtil.close(conn, st, rs);
}
return i;
}
@Override
public List<Food> listFood(Integer BusinessId) {
List<Food> list = new ArrayList<>();
try {
conn = DBUtil.getConnection();
String sql = "select * from elm_food where business_id= ?";
st = conn.prepareStatement(sql);
st.setInt(1, BusinessId);
rs = st.executeQuery();
while(rs.next()) {
Food f = new Food();
f.setFoodId(rs.getInt("food_id"));
f.setFoodName(rs.getString("food_name"));
f.setFoodExplain(rs.getString("food_explain"));
f.setFoodPrice(rs.getDouble("food_price"));
f.setBusinessId(rs.getInt("business_id"));
list.add(f);
}
}catch(Exception e) {
e.printStackTrace();
}finally {
DBUtil.close(conn, st, rs);
}
return list;
}
@Override
public Food listFoodById(Integer foodId) {
Food f = null;
String sql = "select * from elm_food where food_id= ?";
try {
conn = DBUtil.getConnection();
st = conn.prepareStatement(sql);
st.setInt(1, foodId);
rs = st.executeQuery();
while(rs.next()) {
f = new Food();
f.setFoodId(rs.getInt("food_id"));
f.setFoodName(rs.getString("food_name"));
f.setFoodExplain(rs.getString("food_explain"));
f.setFoodPrice(rs.getDouble("food_price"));
f.setBusinessId(rs.getInt("business_id"));
}
} catch (Exception e) {
e.printStackTrace();
} finally {
DBUtil.close(conn, st, rs);
}
return f;
}
}
4.3、view层
AdminView
package com.neuedu.elm.admin.view;
import com.neuedu.elm.admin.entity.Admin;
import com.neuedu.elm.business.entity.Business;
public interface AdminView {
public Admin login();
}
AdminViewImpl
package com.neuedu.elm.admin.view;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.Scanner;
import com.neuedu.elm.admin.dao.AdminDao;
import com.neuedu.elm.admin.dao.AdminDaoImpl;
import com.neuedu.elm.admin.entity.Admin;
import com.neuedu.elm.business.dao.BusinessDao;
import com.neuedu.elm.business.dao.BusinessDaoImpl;
import com.neuedu.elm.business.entity.Business;
import com.neuedu.elm.util.DBUtil;
public class AdminViewImpl implements AdminView{
private AdminDao dao = new AdminDaoImpl();
public Admin login() {
Scanner sc = new Scanner(System.in);
System.out.println("请输入管理员的id:");
int id = sc.nextInt();
System.out.println("请输入管理员的密码:");
String password = sc.next();
Admin a = new Admin(id,null,password);
Admin a2 = dao.login(a);
return a2;
}
}
BusinessView
package com.neuedu.elm.business.view;
import com.neuedu.elm.business.entity.Business;
import java.util.List;
import com.neuedu.elm.admin.entity.Admin;
public interface BusinessView {
public void selectAll();
public void add();
public Business login();
public void delete();
public void update(Integer businessId);
public void updateBusinessPassword(Integer businessId);
public void selectBusiness();
public void selectBusinessById(Integer businessId);
}
BusinessViewImpl
package com.neuedu.elm.business.view;
import java.util.List;
import java.util.Scanner;
import com.neuedu.elm.admin.entity.Admin;
import com.neuedu.elm.business.dao.BusinessDao;
import com.neuedu.elm.business.dao.BusinessDaoImpl;
import com.neuedu.elm.business.entity.Business;
import com.neuedu.elm.food.dao.FoodDao;
import com.neuedu.elm.food.dao.FoodDaoImpl;
import com.neuedu.elm.food.entity.Food;
public class BusinessViewImpl implements BusinessView{
private BusinessDao dao = new BusinessDaoImpl();
private Scanner sc = new Scanner(System.in);
@Override
public void add() {
System.out.println("请输入商家名称:");
String businessName = sc.next();
int id = dao.add(businessName);
if(id>0) {
System.out.println("创建商家成功,商家id为:" + id + ",初始密码为123");
System.out.println("请商家尽快登陆系统,自动修改初始密码!完善信息!");
}else {
System.out.println("创建商家失败!请自行查找原因!");
}
}
@Override
public void selectAll() {
System.out.println("---------------------------------所有商家列表-------------------------------------");
System.out.println("商家id\t|\t密码\t|\t商家名称\t|\t商家地址\t|\t商家描述\t|\t起送费\t|\t配送费");
List<Business> list = dao.selectAll();
for(int i=0;i<list.size();i++) {
Business b2 = list.get(i);
System.out.println("" + (i+1) + b2);
}
System.out.println("-----------------------------------------------------------------------------");
}
@Override
public Business login() {
System.out.println("请输入商家的id:");
int id = sc.nextInt();
System.out.println("请输入商家的密码:");
String password = sc.next();
Business b = new Business(id,password,null, null, null, null, null);
Business b2 = dao.login(b);
return b2;
}
@Override
public void delete() {
Business b =new Business();
System.out.println("请输入要删除的商家id");
int id = sc.nextInt();
b.setBusinessId(id);
BusinessDao dao = new BusinessDaoImpl();
System.out.println("确认要删除吗(y/n)");
if (sc.next().equals("y")){
int i = dao.delete(b);
if (i == 1){
System.out.println("删除商家成功");
}else{
System.out.println("删除商家失败");
}
}
}
@Override
public void update(Integer businessId) {
BusinessDao dao = new BusinessDaoImpl();
Business b = dao.getBusinessById(businessId);
System.out.println("商家id\t|\t密码\t|\t商家名称\t|\t商家地址\t|\t商家描述\t|\t起送费\t|\t配送费");
System.out.println(b);
String str = "";
System.out.println("是否修改商家名称(y/n):");
str = sc.next();
if(str.equals("y")) {
System.out.println("请输入新的商家名称:");
b.setBusinessName(sc.next());
}
System.out.println("是否修改商家地址(y/n):");
str = sc.next();
if(str.equals("y")) {
System.out.println("请输入新的商家地址:");
b.setBusinessAddress(sc.next());
}
System.out.println("是否修改商家介绍(y/n):");
str = sc.next();
if(str.equals("y")) {
System.out.println("请输入新的商家介绍:");
b.setBusinessExplain(sc.next());
}
System.out.println("是否修改起送费(y/n):");
str = sc.next();
if(str.equals("y")) {
System.out.println("请输入新的起送费:");
b.setStartPrice(sc.nextDouble());
}
System.out.println("是否修改配送费(y/n):");
str = sc.next();
if(str.equals("y")) {
System.out.println("请输入新的配送费:");
b.setDeliveryPrice(sc.nextDouble());
}
int i = dao.update(b);
if(i>0) {
System.out.println("修改商家信息成功!");
}else {
System.out.println("修改商家信息失败!");
}
}
@Override
public void updateBusinessPassword(Integer businessId) {
BusinessDao dao = new BusinessDaoImpl();
Business b = dao.getBusinessById(businessId);
System.out.println("请输入旧密码");
String oldPass = sc.next();
System.out.println("请输入新密码");
String newPass = sc.next();
System.out.println("请再次输入新密码");
String beginNewPass = sc.next();
if (!b.getPassword().equals(oldPass)){
System.out.println("你的密码输错了,请重新输入");
}else if (!newPass.equals(beginNewPass)){
System.out.println("两次密码不一致请重新输入");
}else {
int i = dao.updateBusinessPassword(b.getBusinessId(), newPass);
if (i>0){
System.out.println("修改密码成功!");
}else {
System.out.println("修改密码失败!");
}
}
}
@Override
public void selectBusiness() {
String businessName = "";
String str = "";
System.out.println("是否输入商家名称关键词(y/n):");
str = sc.next();
if (str.equals("y")){
System.out.println("请输入商家名称关键词");
businessName = sc.next();
}
BusinessDaoImpl dao = new BusinessDaoImpl();
List<Business> list = dao.listBusiness(businessName);
System.out.println("---------根据您所输入的关键字,模糊查询匹配到的商家如下:---------");
System.out.println("商家id"+"\t"+"商家名称"+"\t"+"商家地址"+"\t"+"商家描述"+"\t"+"起送费"+"\t"+"配送费");
for (Business b :list){
System.out.println(b.getBusinessId() +"\t"+b.getBusinessName()+"\t"+b.getBusinessAddress()+
"\t"+b.getBusinessExplain()+"\t"+b.getStartPrice()+"\t"+b.getDeliveryPrice());
}
}
@Override
public void selectBusinessById(Integer businessId) {
BusinessDao dao = new BusinessDaoImpl();
Business b = dao.getBusinessById(businessId);
System.out.println("商家id\t|\t密码\t|\t商家名称\t|\t商家地址\t|\t商家描述\t|\t商家起送费\t|\t商家配送费");
System.out.println(b);
}
}
FoodView
package com.neuedu.elm.food.view;
import java.util.List;
import com.neuedu.elm.food.entity.Food;
public interface FoodView {
public void add(Integer businessId);
public void delete(Integer businessId);
public void update(Integer businessId);
public List<Food> selectFood(Integer businessId);
}
FoodViewImpl
package com.neuedu.elm.food.view;
import java.util.List;
import java.util.Scanner;
import com.neuedu.elm.business.dao.BusinessDao;
import com.neuedu.elm.business.dao.BusinessDaoImpl;
import com.neuedu.elm.food.dao.FoodDao;
import com.neuedu.elm.food.dao.FoodDaoImpl;
import com.neuedu.elm.food.entity.Food;
public class FoodViewImpl implements FoodView{
private Scanner sc = new Scanner(System.in);
@Override
public void add(Integer businessId) {
Food f = new Food();
System.out.println("请输入新增食品的名称:");
f.setFoodName(sc.next());
System.out.println("请输入新增食品的描述:");
f.setFoodExplain(sc.next());
System.out.println("请输入新增食品的价格:");
f.setFoodPrice(sc.nextDouble());
f.setBusinessId(businessId);
FoodDao dao = new FoodDaoImpl();
int i = dao.add(f);
if(i>0) {
System.out.println("新增食品成功");
}else {
System.out.println("新增食品失败");
}
}
@Override
public void delete(Integer businessId) {
FoodDao dao = new FoodDaoImpl();
List<Food> list = selectFood(businessId);
if(list.size()==0) {
System.out.println("没有任何可以删除的食品!");
}else {
System.out.println("请选择要删除的食品id:");
int foodId = sc.nextInt();
Food f = dao.listFoodById(foodId);
f.setBusinessId(businessId);
System.out.println("确认要删除吗(y/n):");
if(sc.next().equals("y")) {
int i = dao.delete(f);
if(i>0) {
System.out.println("删除食品成功!");
}else {
System.out.println("删除食品失败!");
}
}
}
}
@Override
public void update(Integer businessId) {
FoodDao dao = new FoodDaoImpl();
List<Food> list = selectFood(businessId);
if(list.size()==0) {
System.out.println("没有任何可以修改的食品!");
}else {
System.out.println("请选择要修改的食品id:");
int foodId = sc.nextInt();
Food food = dao.listFoodById(foodId);
food.setBusinessId(businessId);
System.out.println(food);
String str = "";
System.out.println("是否修改食品名称(y/n):");
str = sc.next();
if("y".equals(str)) {
System.out.println("请输入新的食品名称:");
food.setFoodName(sc.next());
}
System.out.println("是否修改食品描述(y/n):");
str = sc.next();
if("y".equals(str)) {
System.out.println("请输入新的食品描述:");
food.setFoodExplain(sc.next());
}
System.out.println("是否修改食品价格(y/n):");
if("y".equals(sc.next())) {
System.out.println("请输入新的食品价格:");
food.setFoodPrice(sc.nextDouble());
}
int i = dao.update(food);
if(i>0) {
System.out.println("修改食品成功!");
}else {
System.out.println("修改食品失败!");
}
}
}
@Override
public List<Food> selectFood(Integer businessId) {
FoodDao dao = new FoodDaoImpl();
List<Food> list = dao.listFood(businessId);
System.out.println("食品id\t|\t食品名称\t|\t食品描述\t|\t食品价格");
for(Food food : list) {
System.out.println(food.getFoodId()+"\t|\t"+food.getFoodName()
+"\t|\t"+food.getFoodExplain()+"\t|\t"+food.getFoodPrice());
}
return list;
}
}
4.4、程序入口
AdminEnter
package com.neuedu.elm;
import java.util.Scanner;
import com.neuedu.elm.admin.entity.Admin;
import com.neuedu.elm.admin.view.AdminView;
import com.neuedu.elm.admin.view.AdminViewImpl;
import com.neuedu.elm.business.view.BusinessView;
import com.neuedu.elm.business.view.BusinessViewImpl;
public class AdminEnter {
public static void main(String[] args) {
new AdminEnter().work();
}
public void work() {
System.out.println("========================饿了么管理员登录========================");
AdminView aview = new AdminViewImpl();
BusinessView view = new BusinessViewImpl();
Scanner sc = new Scanner(System.in);
Admin a = aview.login();
if(a!=null) {
int num = 0;
while(num!=5) {
System.out.println("请选择进行哪种操作:>>>>>>>>>>>>>>>>>");
System.out.println("\t1-显示所有商家列表"
+ "\t 2-根据商家名称查询商家"
+ "\t 3-创建商家"
+ "\t 4-删除商家"
+ "\t 5-退出系统");
num = sc.nextInt();
switch(num) {
case 1:
view.selectAll();
break;
case 2:
view.selectBusiness();
break;
case 3:
view.add();
break;
case 4:
view.delete();
break;
case 5:
System.out.println("========================退出系统,欢迎下次再使用========================");
break;
default:
System.out.println("没有这个选项!");
break;
}
}
}else {
System.out.println("登录失败!请检查id和密码是否正确!");
}
}
}
BusinessEnter
package com.neuedu.elm;
import java.util.Scanner;
import com.neuedu.elm.admin.view.AdminView;
import com.neuedu.elm.admin.view.AdminViewImpl;
import com.neuedu.elm.business.entity.Business;
import com.neuedu.elm.business.view.BusinessView;
import com.neuedu.elm.business.view.BusinessViewImpl;
import com.neuedu.elm.food.view.FoodViewImpl;
public class BusinessEnter {
public static void main(String[] args) throws Exception {
run();
}
public static void run() throws Exception {
System.out.println("===================================================================");
System.out.println("----------------------------------饿了么商家自主管理系统---------------------------");
System.out.println("===================================================================");
Scanner sc = new Scanner(System.in);
AdminView aview = new AdminViewImpl();
BusinessView view = new BusinessViewImpl();
Business business = view.login();
if (business != null){
System.out.println(">>>>>>>商家#"+ business.getBusinessName() +"#欢迎您回来!>>>>>>>");
int num = 0;
while (num != 5){
System.out.println(">>>>>>> 一级菜单\t 1-查看商家信息\t 2-修改商家信息\t 3-修改密码\t 4-所属商品管理\t 5-退出系统");
System.out.println("请输入你要选择的序号:");
num = sc.nextInt();
switch (num){
case 1:
view.selectBusinessById(business.getBusinessId());
break;
case 2:
view.update(business.getBusinessId());
break;
case 3:
view.updateBusinessPassword(business.getBusinessId());
break;
case 4:
new BusinessEnter().foodManager(business.getBusinessId());
break;
case 5:
System.out.println("----------------------------------欢迎下次登录----------------------------------");
break;
default:
System.out.println("没有这个选项,请重新输入");
break;
}
}
}else {
System.out.println("登录失败,用户名密码错误!");
}
}
public void foodManager(Integer businessId) throws Exception{
FoodViewImpl view = new FoodViewImpl();
int num = 0;
while(num != 5) {
System.out.println(">>>>>>> 二级菜单\t 1-查看食品信息\t 2-修改食品信息\t 3-新增食品信息\t 4-删除食品信息\t 5-返回上一级菜单");
System.out.println("请输入你要选择的序号:");
Scanner sc = new Scanner(System.in);
num = sc.nextInt();
switch(num) {
case 1:
view.selectFood(businessId);
break;
case 2:
view.update(businessId);
break;
case 3:
view.add(businessId);
break;
case 4:
view.delete(businessId);
break;
case 5:
break;
default :
System.out.println("没有这个选项,请重新输入");
break;
}
}
}
}
|