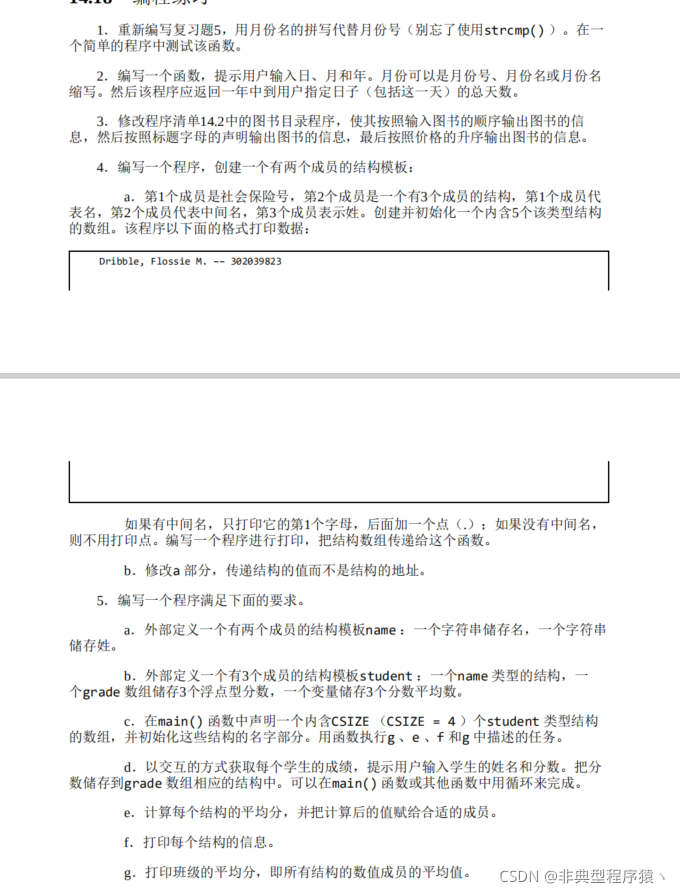 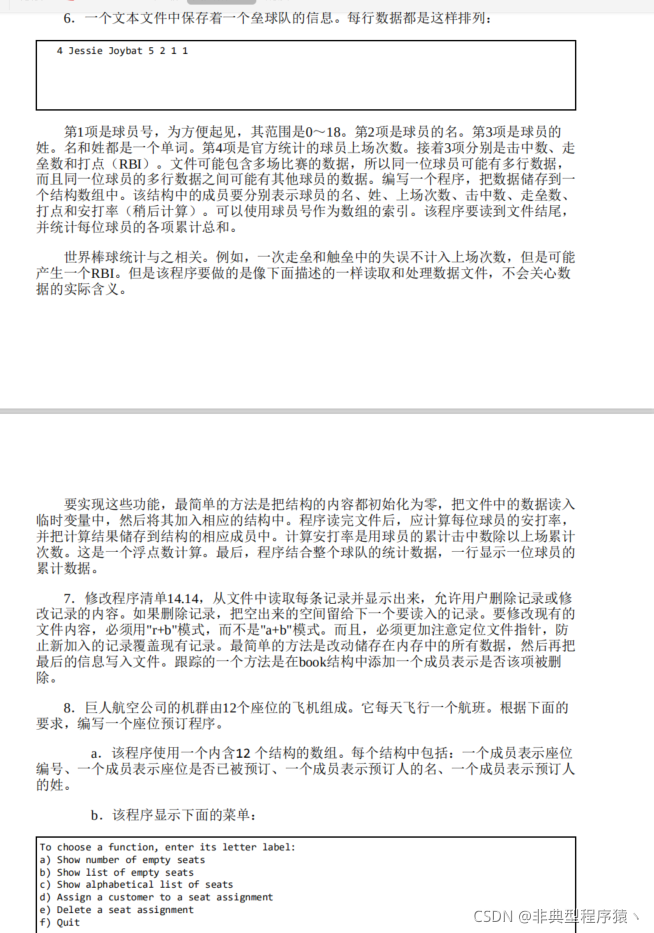 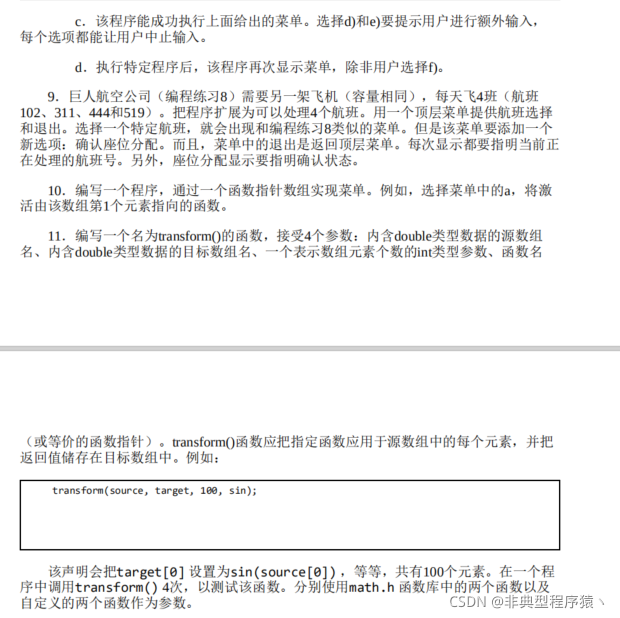 1.
#include <stdio.h>
#include <string.h>
struct month
{
char name[10];
char abbrev[4];
int days;
int monumb;
};
struct month months[12] =
{
{ "January", "jan", 31, 1 },
{ "February", "feb", 28, 2 },
{ "March", "mar", 31, 3 },
{ "April", "apr", 30, 4 },
{ "May", "may", 31, 5 },
{ "June", "jun", 30, 6 },
{ "July", "jul", 31, 7 },
{ "August", "aug", 31, 8 },
{ "September", "sep", 30, 9 },
{ "October", "oct", 31, 10 },
{ "November", "nov", 30, 11 },
{ "December", "dec", 31, 12 }
};
int allDays(char *temp)
{
int index;
int sum = 0;
for(index = 0; index < 12; index++)
{
if(strcmp(temp, months[index].name) == 0)
{
break;
}
}
if(index != 12)
{
for(int i = 0; i <= index; i++)
{
sum += months[i].days;
}
return sum;
}
else
{
return -1;
}
}
int main(void)
{
int days;
char temp[10];
printf("Please enter a month name(and input q to quit)\n");
while((scanf("%s", temp) == 1) && (temp[0] != 'q'))
{
days = allDays(temp);
if(days > 0)
{
printf("There are %d days altogether\n",days);
printf("Please enter a month name(and input q to quit)\n");
}
else
{
printf("Can't find %s, Please input again\n", temp);
while(getchar() != '\n')
continue;
}
}
return 0;
}
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
struct month
{
char name[10];
char abbrev[4];
int days;
int monumb;
};
struct month months[12] =
{
{ "January", "jan", 31, 1 },
{ "February", "feb", 28, 2 },
{ "March", "mar", 31, 3 },
{ "April", "apr", 30, 4 },
{ "May", "may", 31, 5 },
{ "June", "jun", 30, 6 },
{ "July", "jul", 31, 7 },
{ "August", "aug", 31, 8 },
{ "September", "sep", 30, 9 },
{ "October", "oct", 31, 10 },
{ "November", "nov", 30, 11 },
{ "December", "dec", 31, 12 }
};
int allDays(char *temp, int day)
{
int index;
int sum = 0;
int temp1 = atoi(temp);
if(day < 1 && day > 31)
{
return -1;
}
for(index = 0; index < 12; index++)
{
if((temp1 == months[index].monumb) || (strcmp(temp, months[index].name) == 0) || (strcmp(temp, months[index].abbrev) == 0))
{
return sum += day;
}
else
{
sum += months[index].days;
}
}
return -1;
}
void isLeepYear(int year)
{
if(((year % 4 == 0) && year % 100 != 0) || (year % 400 == 0))
{
months[1].days = 29;
}
else
{
months[1].days = 28;
}
}
int main(void)
{
int day;
int year;
int days;
char temp[12];
printf("Please enter day, month, year(and input q to quit)\n");
while((scanf("%d %11s %d", &day, temp, &year) == 3))
{
isLeepYear(year);
days = allDays(temp, day);
if(days > 0)
{
printf("There are %d days altogether\n",days);
printf("Please enter a month name(and input q to quit)\n");
}
else
{
printf("Can't find %s, Please input again\n", temp);
while(getchar() != '\n')
continue;
}
}
return 0;
}
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <stdbool.h>
#include <string.h>
char * s_gets(char * st,int n);
#define MAXTITL 40
#define MAXAUTL 40
#define MAXBKS 100
struct book{
char title[MAXTITL];
char author[MAXAUTL];
float value;
};
void sort_title(struct book *library[], int count)
{
struct book *temp;
for(int i = 0; i < count - 1; i++)
{
for(int j = i + 1; j < count; j++)
if(strcmp(library[i]->title, library[j]->title) > 0)
{
temp = library[i];
library[i] = library[j];
library[j] = temp;
}
}
}
void sort_value(struct book *library[], int count)
{
struct book *temp;
for(int i = 0; i < count - 1; i++)
{
for(int j = i + 1; j < count; j++)
if(library[i]->value > library[j]->value)
{
temp = library[i];
library[i] = library[j];
library[j] = temp;
}
}
}
int main(void)
{
struct book library[MAXBKS];
struct book *books[MAXBKS];
int count = 0;
int index;
printf("Please enter the book title.\n");
printf("Press [enter] at the start of a line to stop.\n");
while(count < MAXBKS && s_gets(library[count].title,MAXTITL) != NULL && library[count].title[0] != '\0')
{
printf("Now enter the author.\n");
s_gets(library[count].author,MAXAUTL);
printf("Now enter the value.\n");
scanf("%f",&library[count].value);
books[count] = &library[count];
count++;
while(getchar() != '\n')
continue;
if(count < MAXBKS)
{
printf("Enter the next title.\n");
}
}
if(count > 0)
{
printf("Here is the list of your books:\n");
for(index = 0; index < count; index++)
printf("%s by %s: $%.2f\n",library[index].title,library[index].author,library[index].value);
sort_title(books, count);
for(index = 0; index < count; index++)
printf("%s by %s: $%.2f\n",books[index]->title,books[index]->author,books[index]->value);
sort_value(books, count);
for(index = 0; index < count; index++)
printf("%s by %s: $%.2f\n",books[index]->title,books[index]->author,books[index]->value);
}
else
{
printf("No books? Too bad.\n");
}
printf("end");
printf("\n");
system("pause");
return 0;
}
char * s_gets(char * st, int n)
{
char * ret_val;
char * find;
ret_val = fgets(st,n,stdin);
if(ret_val)
{
find = strchr(st,'\n');
if(find)
*find = '\0';
else
{
while(getchar() != '\n')
continue;
}
}
return ret_val;
}
4.a
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define LEN 10
struct str1{
char firstName[LEN];
char midName[LEN];
char lastName[LEN];
};
struct str2{
char badInsNum[LEN];
struct str1 name;
};
char* s_gets(char arr[], int n)
{
char *ret1;
char *ret2;
ret1 = fgets(arr, n, stdin);
if(ret1)
{
ret2 = strchr(arr, '\n');
if(ret2)
{
*ret2 = '\0';
}
else
{
while(getchar() != '\n')
continue;
}
}
return ret1;
}
void showData(struct str2 arr[], int n)
{
for(int i = 0; i < n; i++)
{
if(*arr[i].name.midName)
printf("%s, %s %c. -- %s\n", arr[i].name.firstName, arr[i].name.lastName, arr[i].name.midName[0], arr[i].badInsNum);
else
printf("%s, %s -- %s\n", arr[i].name.firstName, arr[i].name.lastName, arr[i].badInsNum);
}
}
int main(void)
{
struct str2 arr[5];
int count = 0;
printf("Please input first name: ");
while((count < 5) && (s_gets(arr[count].name.firstName, LEN) != NULL) && ((arr[count].name.firstName[0]) != '\0'))
{
printf("Please input mid name: ");
s_gets(arr[count].name.midName, LEN);
printf("Please input last name: ");
s_gets(arr[count].name.lastName, LEN);
printf("Please input badInsNum: ");
s_gets(arr[count].badInsNum, LEN);
count++;
if(count < 5)
{
printf("Please input first name: ");
}
}
if(count > 0)
{
showData(arr, count);
}
else
{
printf("No data\n");
}
return 0;
}
4.b
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define LEN 10
struct str1{
char firstName[LEN];
char midName[LEN];
char lastName[LEN];
};
struct str2{
char badInsNum[LEN];
struct str1 name;
};
char* s_gets(char arr[], int n)
{
char *ret1;
char *ret2;
ret1 = fgets(arr, n, stdin);
if(ret1)
{
ret2 = strchr(arr, '\n');
if(ret2)
{
*ret2 = '\0';
}
else
{
while(getchar() != '\n')
continue;
}
}
return ret1;
}
void showData(const struct str2 arr)
{
if(arr.name.midName[0] != '\0')
printf("%s, %s %c. -- %s\n", arr.name.firstName, arr.name.lastName, arr.name.midName[0], arr.badInsNum);
else
printf("%s, %s -- %s\n", arr.name.firstName, arr.name.lastName, arr.badInsNum);
}
int main(void)
{
struct str2 arr[5];
int count = 0;
printf("Please input first name: ");
while((count < 5) && (s_gets(arr[count].name.firstName, LEN) != NULL) && ((arr[count].name.firstName[0]) != '\0'))
{
printf("Please input mid name: ");
s_gets(arr[count].name.midName, LEN);
printf("Please input last name: ");
s_gets(arr[count].name.lastName, LEN);
printf("Please input badInsNum: ");
s_gets(arr[count].badInsNum, LEN);
count++;
if(count < 5)
{
printf("Please input first name: ");
}
}
if(count > 0)
{
for(int i = 0; i < count; i++)
showData(arr[i]);
}
else
{
printf("No data\n");
}
return 0;
}
5
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define LEN 10
#define SIZE 3
#define CSIZE 4
struct name{
char firstName[LEN];
char lastName[LEN];
};
struct student{
struct name names;
double scores[SIZE];
double average;
};
char* s_gets(char arr[], int n)
{
char *ret1;
char *ret2;
ret1 = fgets(arr, n, stdin);
if(ret1)
{
ret2 = strchr(arr, '\n');
if(ret2)
{
*ret2 = '\0';
}
else
{
while(getchar() != '\n')
continue;
}
}
return ret1;
}
void getInformation(struct student students[], int n)
{
int index = 0;
while(index < n)
{
printf("Please input student's first name: ");
s_gets(students[index].names.firstName, LEN);
printf("Please input student's last name: ");
s_gets(students[index].names.lastName, LEN);
printf("Please input student's three scores: ");
scanf("%lf %lf %lf",&(students[index].scores[0]), &(students[index].scores[1]), &(students[index].scores[2]));
while(getchar() != '\n')
continue;
index++;
}
}
void calculateAverage(struct student students[], int n)
{
for(int i = 0; i < n; i++)
{
students[i].average = (students[i].scores[0] + students[i].scores[1] + students[i].scores[2]) / 3;
}
}
void showInformation(struct student students[], int n)
{
for(int i = 0; i < n; i++)
{
printf("%s %s: score1: %.2f, score2: %.2f, score3: %.2f, average: %.2f\n",
students[i].names.firstName, students[i].names.lastName, students[i].scores[0],
students[i].scores[1], students[i].scores[2], students[i].average);
}
}
void showAllAverage(struct student students[], int n)
{
double average = 0;
for(int i = 0; i < n; i++)
{
average += students[i].average;
}
average /= 4;
printf("The all source's average is %.2f", average);
}
int main(void)
{
struct student students[CSIZE];
getInformation(students, CSIZE);
calculateAverage(students, CSIZE);
showInformation(students, CSIZE);
showAllAverage(students, CSIZE);
return 0;
}
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define LEN 15
#define SIZE 19
struct name{
char firstName[LEN];
char lastName[LEN];
};
typedef struct softballTeam{
struct name name;
int actionCount;
int hitNumber;
int goHomeAFew;
int RBI;
double dotRate;
}softballTeam;
char* s_gets(char arr[], int n)
{
char *ret1;
char *ret2;
ret1 = fgets(arr, n, stdin);
if(ret1)
{
ret2 = strchr(arr, '\n');
if(ret2)
{
*ret2 = '\0';
}
else
{
while(getchar() != '\n')
continue;
}
}
return ret1;
}
static softballTeam team[SIZE];
int readData(softballTeam team[], int len, FILE *fp)
{
char firstName[LEN], lastName[LEN];
int actionCount, hitNumber, goHomeAFew, RBI, playerID;
double dotRate;
int count;
int ch = 0;
count = fscanf(fp,"%d %18s %18s %d %d %d %d", &playerID, firstName, lastName, &actionCount, &hitNumber, &goHomeAFew, &RBI);
while((count == 7) && ch <= SIZE)
{
if(team[playerID].actionCount == 0)
{
ch++;
strcpy(team[playerID].name.firstName, firstName);
strcpy(team[playerID].name.lastName, lastName);
}
team[playerID].actionCount += actionCount;
team[playerID].hitNumber += hitNumber;
team[playerID].goHomeAFew += goHomeAFew;
team[playerID].RBI += RBI;
team[playerID].dotRate = (double)team[playerID].hitNumber / team[playerID].actionCount;
count = fscanf(fp,"%d %18s %18s %d %d %d %d", &playerID, firstName, lastName, &actionCount, &hitNumber, &goHomeAFew, &RBI);
}
return ch;
}
int main(void)
{
FILE *fp;
int count;
fp = fopen("1.txt", "r");
if(fp == NULL)
{
fprintf(stderr,"Error, Can't open \"1.txt\", please check it!\n");
exit(EXIT_FAILURE);
}
count = readData(team, SIZE, fp);
if(count != 0)
{
printf("There are %d member in this team\n", count);
printf("Here are thre list:\n");
printf("%s %s %s %s %s %s %s %s\n", "ID", "FirstName", "LastName", "actionCount", "hitnumber", "goHomeAFew", "RBI", "dotrate");
for(int i = 0; i < SIZE; i++)
{
if(team[i].actionCount != 0)
{
printf("%2d %9s %8s %11d %9d %10d %3d %7.2f\n", i, team[i].name.firstName, team[i].name.lastName, team[i].actionCount, team[i].hitNumber
,team[i].goHomeAFew, team[i].RBI, team[i].dotRate);
}
}
}
else
{
printf("No member in this team, please check it\n");
}
fclose(fp);
return 0;
}
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <stdbool.h>
#include <string.h>
char * s_gets(char * st,int n);
#define MAXTITL 40
#define MAXAUTL 40
#define MAXBKS 10
struct book{
char title[MAXTITL];
char author[MAXAUTL];
float value;
};
struct pack{
struct book book;
bool delete_me;
};
void eatline(void)
{
while(getchar() != '\n')
continue;
}
int get_choice(const char *pt)
{
int ch = getchar();
eatline();
while(strchr(pt, ch) == NULL)
{
printf("Error, please input right choice. like %s\n", pt);
ch = getchar();
eatline();
}
return ch;
}
void change_book(struct pack *p)
{
char ch;
struct book temp = p->book;
puts("Please according last menu ti continue:");
puts("a)modefy book's title b)modefy book's author");
puts("c)modefy book's value d)save it ");
puts("e)quit it");
while(((ch = get_choice("abcde")) != 'd') && (ch != 'e'))
{
switch(ch)
{
case 'a':
printf("Please input book's title:\n");
s_gets(temp.title,MAXAUTL);
break;
case 'b':
printf("Please input book's author:\n");
s_gets(temp.author,MAXAUTL);
break;
case 'c':
printf("Please input book's value:\n");
while(scanf("%f",&(temp.value)) != 1)
{
puts("Please input a number:");
scanf("%*s");
}
eatline();
break;
}
puts("Please according last menu ti continue:");
puts("a)modefy book's title b)modefy book's author");
puts("c)modefy book's value d)save it ");
puts("e)quit it");
}
if(ch == 'd')
{
p->book = temp;
}
}
int get_book(struct pack *p)
{
int status = 1;
puts("Please input book's title");
if((s_gets(p->book.title, MAXTITL) == NULL) || (p->book.title[0] == '\0'))
{
status = 0;
}
else
{
printf("Please input book's author:\n");
s_gets(p->book.author,MAXAUTL);
printf("Please input book's value:\n");
while(scanf("%f",&(p->book.value)) != 1)
{
puts("Please input a number:");
scanf("%*s");
}
eatline();
p->delete_me = false;
}
return status;
}
int main(void)
{
struct pack library[MAXBKS];
int count = 0;
int delete_number = 0;
int index,filecount;
FILE * pbooks;
int size = sizeof(struct book);
if((pbooks = fopen("book.dat","r+b")) == NULL)
{
fputs("Can't open book.dat file\n",stderr);
exit(EXIT_FAILURE);
}
while(count < MAXBKS && fread(&library[count],size,1,pbooks) == 1)
{
if(count == 0)
puts("Current contents of book.dat:");
printf("%s by %s: $%.2f\n",library[count].book.title,library[count].book.author,library[count].book.value);
printf("Want to delete or modefy the book?(y/n)\n");
if(get_choice("yn") == 'y')
{
printf("d to delete book, m to modefy the book?(d/m)\n");
if(get_choice("dm") == 'm')
{
change_book(&library[count]);
}
else
{
library[count].delete_me = true;
delete_number++;
puts("Finished deleting book");
}
}
count++;
}
fclose(pbooks);
filecount = count - delete_number;
if(count == MAXBKS)
{
fputs("The book.day file is full.",stderr);
exit(2);
}
printf("Please add new book titles.\n");
printf("Press [enter] at the start of a line to stop.\n");
int open = 0;
while(filecount < MAXBKS)
{
if(filecount < count)
{
while(library[open].delete_me == false)
open++;
if(get_book(&library[open]) == 0)
break;
}
else
{
if(get_book(&library[filecount]) == 0)
break;
}
filecount++;
if(filecount < MAXBKS)
{
puts("Please input new book");
}
}
if((pbooks = fopen("book.dat","w+b")) == NULL)
{
fputs("Can't open book.dat file\n",stderr);
exit(EXIT_FAILURE);
}
if(filecount > 0)
{
printf("Here is the list of your books:\n");
for(index = 0; index < filecount; index++)
{
if(library[index].delete_me == false)
{
printf("%s by %s: $%.2f\n",library[index].book.title,library[index].book.author,library[index].book.value);
fwrite(&library[index].book,size,1,pbooks);
}
}
}
else
{
printf("No books? Too bad.\n");
}
fclose(pbooks);
printf("end");
printf("\n");
system("pause");
return 0;
}
char * s_gets(char * st, int n)
{
char * ret_val;
char * find;
ret_val = fgets(st,n,stdin);
if(ret_val)
{
find = strchr(st,'\n');
if(find)
*find = '\0';
else
{
while(getchar() != '\n')
continue;
}
}
return ret_val;
}
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <stdbool.h>
#include <string.h>
#define LEN 15
#define SIZE 12
char * s_gets(char * st, int n)
{
char * ret_val;
char * find;
ret_val = fgets(st,n,stdin);
if(ret_val)
{
find = strchr(st,'\n');
if(find)
*find = '\0';
else
{
while(getchar() != '\n')
continue;
}
}
return ret_val;
}
struct airline{
int seatId;
bool isOrder;
char firstName[LEN];
char lastName[LEN];
};
void eatLine(void)
{
while(getchar() != '\n')
continue;
}
int getInupt(const char *arr)
{
int ch = getchar();
eatLine();
while(strchr(arr, ch) == NULL)
{
printf("Error, please input %s\n", arr);
ch = getchar();
eatLine();
}
return ch;
}
#define LEN1 7
int showMenu(void)
{
int ch;
const char* pt[LEN1 + 1] = {"To choose a function, enter its letter label: ",
"a) Show number of empty seats",
"b) Show list of empty seats",
"c) Show alphabetical list of seats",
"d) Assign a customer to seat assignment",
"e) Delete a seat assignment",
"f) Quit"};
const char option[LEN1] = "abcdef";
for(int i = 0; i < LEN1; i++)
{
puts(pt[i]);
}
ch = getInupt(option);
return ch;
}
int showNumOfEmptySeat(struct airline seat[], int len)
{
int number = 0;
for(int i = 0; i < len; i++)
{
if(seat[i].isOrder == false)
number++;
}
return number;
}
void makeList(struct airline seat[], int len, char *pt, bool flag)
{
pt[0] = '\0';
char temp[5];
for(int i = 0; i < len; i++)
{
if(seat[i].isOrder == flag)
{
sprintf(temp, "%d ",seat[i].seatId);
strcat(pt, temp);
}
}
}
void showListOfEmptySeat(struct airline seat[], int len)
{
char temp[SIZE * 3];
if(showNumOfEmptySeat(seat, len) == 0)
{
printf("The seat is full\n");
}
else
{
puts("The following is avaiable:");
makeList(seat, len, temp, false);
puts(temp);
}
}
void sort_seat(struct airline *ptr[], int len)
{
struct airline *temp;
for(int i = 0; i < len - 1; i++)
{
for(int j = i + 1; j < len; j++)
{
if(strcmp(ptr[i]->firstName, ptr[j]->firstName) > 0)
{
temp = ptr[i];
ptr[i] = ptr[j];
ptr[j] = temp;
}
}
}
}
void showAlphabeticalListOfSeat(struct airline *ptr[], int len)
{
if(showNumOfEmptySeat(*ptr, len) == SIZE)
{
puts("The seat is empty, please check it");
}
else
{
sort_seat(ptr, len);
for(int i = 0; i < len; i++)
{
if(ptr[i]->isOrder == true)
{
printf("%s %s order %d\n",ptr[i]->firstName, ptr[i]->lastName, ptr[i]->seatId);
}
}
}
}
void assignAcustomerToaSeatAssignment(struct airline seat[], int len)
{
char ch;
bool status = false;
int number;
char temp[SIZE * 3];
if(showNumOfEmptySeat(seat, len) == 0)
{
printf("The seat is full\n");
}
else
{
puts("These seats are available");
makeList(seat, len, temp, false);
puts(temp);
printf("Please choose number of seat above:\n");
do
{
while(scanf("%d", &number) != 1)
{
scanf("%*s");
}
if(number <= 0 || number > SIZE || seat[number - 1].isOrder == true)
{
puts("Input error, please input again");
puts(temp);
status = false;
}
else
{
status = true;
}
} while (status == false);
eatLine();
puts("Please input your first name:");
s_gets(seat[number - 1].firstName, LEN);
puts("Please input your last name:");
s_gets(seat[number - 1].lastName, LEN);
puts("Please input c to cancel, s to save it");
ch = getInupt("cs");
if(ch == 's')
{
seat[number - 1].isOrder = true;
puts("Passenger assigned to seat");
}
else
{
puts("Passenger not assigned to seat");
}
}
}
void deleteAseatassignment(struct airline seat[], int len)
{
char ch;
bool status = false;
int number;
char temp[SIZE * 3];
if(showNumOfEmptySeat(seat, len) == SIZE)
{
printf("The seat is full\n");
}
else
{
puts("These seats can be deleted");
makeList(seat, len, temp, true);
puts(temp);
printf("Please choose number of seat above:\n");
do
{
while(scanf("%d", &number) != 1)
{
scanf("%*s");
}
if(number <= 0 || number > SIZE || seat[number - 1].isOrder == false)
{
puts("Input error, please input again");
puts(temp);
status = false;
}
else
{
status = true;
}
} while (status == false);
eatLine();
puts("Please input c to cancel, s to save it");
ch = getInupt("cs");
if(ch == 's')
{
seat[number - 1].isOrder = false;
puts("Passenger dropped");
}
else
{
puts("Passenger retained");
}
}
}
int main(void)
{
struct airline seat[SIZE], *ptr[SIZE];
FILE *fp;
size_t size = sizeof(struct airline);
int ch;
if((fp = fopen("mesage.dat","rb")) == NULL)
{
for(int i = 0; i < SIZE; i++)
{
seat[i].isOrder = false;
seat[i].seatId = i + 1;
}
}
else
{
fread(seat, size, SIZE, fp);
fclose(fp);
}
for(int i = 0; i < SIZE; i++)
{
ptr[i] = &seat[i];
}
while((ch = showMenu()) != 'f')
{
switch(ch)
{
case 'a':
printf("There have %d seats in our plane\n", showNumOfEmptySeat(seat, SIZE));
break;
case 'b':
showListOfEmptySeat(seat, SIZE);
break;
case 'c':
showAlphabeticalListOfSeat(ptr, SIZE);
break;
case 'd':
assignAcustomerToaSeatAssignment(seat, SIZE);
break;
case 'e':
deleteAseatassignment(seat, SIZE);
break;
}
}
if((fp = fopen("mesage.dat","wb")) == NULL)
{
puts("Failed to open masage.dat!");
}
else
{
fwrite(seat, size, SIZE, fp);
fclose(fp);
}
return 0;
}
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <stdbool.h>
#include <string.h>
#define LEN 15
#define SIZE 12
#define NUMS 4
char * s_gets(char * st, int n)
{
char * ret_val;
char * find;
ret_val = fgets(st,n,stdin);
if(ret_val)
{
find = strchr(st,'\n');
if(find)
*find = '\0';
else
{
while(getchar() != '\n')
continue;
}
}
return ret_val;
}
struct airline{
int seatId;
bool isOrder;
char firstName[LEN];
char lastName[LEN];
};
struct flight{
struct airline air[SIZE];
};
struct flightPtr{
struct airline *air[SIZE];
};
void eatLine(void)
{
while(getchar() != '\n')
continue;
}
int getInupt(const char *arr)
{
int ch = getchar();
eatLine();
while(strchr(arr, ch) == NULL)
{
printf("Error, please input %s\n", arr);
ch = getchar();
eatLine();
}
return ch;
}
#define LEN1 8
int showMenu(void)
{
int ch;
const char* pt[LEN1] = {"To choose a function, enter its letter label: ",
"a) Show number of empty seats",
"b) Show list of empty seats",
"c) Show alphabetical list of seats",
"d) Assign a customer to seat assignment",
"e) Delete a seat assignment",
"f) Confirm seat",
"g) Quit"};
const char option[LEN1] = "abcdefg";
for(int i = 0; i < LEN1; i++)
{
puts(pt[i]);
}
ch = getInupt(option);
return ch;
}
#define LEN2 6
int showflight(void)
{
int ch;
const char* pt[LEN2] = {"To choose a flight, enter its letter label: ",
"a) choose 102 flight",
"b) choose 311 flight",
"c) choose 444 flights",
"d) choose 519 flight",
"e) Quit"};
const char option[LEN2] = "abcde";
for(int i = 0; i < LEN2; i++)
{
puts(pt[i]);
}
ch = getInupt(option);
return ch;
}
int showNumOfEmptySeat(struct airline seat[], int len)
{
int number = 0;
for(int i = 0; i < len; i++)
{
if(seat[i].isOrder == false)
number++;
}
return number;
}
void makeList(struct airline seat[], int len, char *pt, bool flag)
{
pt[0] = '\0';
char temp[5];
for(int i = 0; i < len; i++)
{
if(seat[i].isOrder == flag)
{
sprintf(temp, "%d ",seat[i].seatId);
strcat(pt, temp);
}
}
}
void showListOfEmptySeat(struct airline seat[], int len)
{
char temp[SIZE * 3];
if(showNumOfEmptySeat(seat, len) == 0)
{
printf("The seat is full\n");
}
else
{
puts("The following is avaiable:");
makeList(seat, len, temp, false);
puts(temp);
}
}
void sort_seat(struct airline *ptr[], int len)
{
struct airline *temp;
for(int i = 0; i < len - 1; i++)
{
for(int j = i + 1; j < len; j++)
{
if(strcmp(ptr[i]->firstName, ptr[j]->firstName) > 0)
{
temp = ptr[i];
ptr[i] = ptr[j];
ptr[j] = temp;
}
}
}
}
void showAlphabeticalListOfSeat(struct airline *ptr[], int len)
{
if(showNumOfEmptySeat(*ptr, len) == SIZE)
{
puts("The seat is empty, please check it");
}
else
{
sort_seat(ptr, len);
for(int i = 0; i < len; i++)
{
if(ptr[i]->isOrder == true)
{
printf("%s %s order %d\n",ptr[i]->firstName, ptr[i]->lastName, ptr[i]->seatId);
}
}
}
}
void assignAcustomerToaSeatAssignment(struct airline seat[], int len)
{
char ch;
bool status = false;
int number;
char temp[SIZE * 3];
if(showNumOfEmptySeat(seat, len) == 0)
{
printf("The seat is full\n");
}
else
{
puts("These seats are available");
makeList(seat, len, temp, false);
puts(temp);
printf("Please choose number of seat above:\n");
do
{
while(scanf("%d", &number) != 1)
{
scanf("%*s");
}
if(number <= 0 || number > SIZE || seat[number - 1].isOrder == true)
{
puts("Input error, please input again");
puts(temp);
status = false;
}
else
{
status = true;
}
} while (status == false);
eatLine();
puts("Please input your first name:");
s_gets(seat[number - 1].firstName, LEN);
puts("Please input your last name:");
s_gets(seat[number - 1].lastName, LEN);
puts("Please input c to cancel, s to save it");
ch = getInupt("cs");
if(ch == 's')
{
seat[number - 1].isOrder = true;
puts("Passenger assigned to seat");
}
else
{
puts("Passenger not assigned to seat");
}
}
}
void deleteAseatassignment(struct airline seat[], int len)
{
char ch;
bool status = false;
int number;
char temp[SIZE * 3];
if(showNumOfEmptySeat(seat, len) == SIZE)
{
printf("The seat is full\n");
}
else
{
puts("These seats can be deleted");
makeList(seat, len, temp, true);
puts(temp);
printf("Please choose number of seat above:\n");
do
{
while(scanf("%d", &number) != 1)
{
scanf("%*s");
}
if(number <= 0 || number > SIZE || seat[number - 1].isOrder == false)
{
puts("Input error, please input again");
puts(temp);
status = false;
}
else
{
status = true;
}
} while (status == false);
eatLine();
puts("Please input c to cancel, s to save it");
ch = getInupt("cs");
if(ch == 's')
{
seat[number - 1].isOrder = false;
puts("Passenger dropped");
}
else
{
puts("Passenger retained");
}
}
}
int showWhichFlight(int ch)
{
switch(ch)
{
case 'a':printf("You are operating 102 flight\n");return 0;
case 'b':printf("You are operating 311 flight\n");return 1;
case 'c':printf("You are operating 444 flight\n");return 2;
case 'd':printf("You are operating 519 flight\n");return 3;
}
}
void confirmSeat(struct airline seat[], int len)
{
puts("Seats assignment list:");
for(int i = 0; i < len; i++)
{
if(seat[i].isOrder == true)
{
printf("Seat %d: assignment\n",seat[i].seatId);
}
else
{
printf("Seat %d: unassignment\n",seat[i].seatId);
}
}
}
int main(void)
{
struct flight flight[NUMS];
struct flightPtr ptr[NUMS];
FILE *fp;
size_t size = sizeof(struct flight);
int ch;
int ch2;
if((fp = fopen("mesage.dat","rb")) == NULL)
{
for(int i = 0; i < NUMS; i++)
{
for(int j = 0; j < SIZE; j++)
{
flight[i].air[j].isOrder = false;
flight[i].air[j].seatId = j + 1;
}
}
}
else
{
fread(flight, size, NUMS, fp);
fclose(fp);
}
for(int i = 0; i < NUMS; i++)
{
for(int j = 0; j < SIZE; j++)
{
ptr[i].air[j] = &(flight[i].air[j]);
}
}
while((ch2 = showflight()) != 'e')
{
int num = showWhichFlight(ch2);
while((ch = showMenu()) != 'g')
{
showWhichFlight(ch2);
switch(ch)
{
case 'a':
printf("There have %d seats in our plane\n", showNumOfEmptySeat(flight[num].air, SIZE));
break;
case 'b':
showListOfEmptySeat(flight[num].air, SIZE);
break;
case 'c':
showAlphabeticalListOfSeat(ptr[num].air, SIZE);
break;
case 'd':
assignAcustomerToaSeatAssignment(flight[num].air, SIZE);
break;
case 'e':
deleteAseatassignment(flight[num].air, SIZE);
break;
case 'f':
confirmSeat(flight[num].air, SIZE);
break;
}
showWhichFlight(ch2);
}
}
if((fp = fopen("mesage.dat","wb")) == NULL)
{
puts("Failed to open masage.dat!");
}
else
{
fwrite(flight, size, NUMS, fp);
fclose(fp);
}
return 0;
}
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <stdbool.h>
#include <string.h>
void sum(int a, int b)
{
printf("%d + %d = %d\n", a, b, a + b);
}
void sub(int a, int b)
{
printf("%d - %d = %d\n", a, b, a - b);
}
void mult(int a, int b)
{
printf("%d * %d = %d\n", a, b, a * b);
}
void divide(int a, int b)
{
printf("%d / %d = %f\n", a, b, (double)(a) / b);
}
void eatLine(void)
{
while(getchar() != '\n')
continue;
}
int getInupt(const char *arr)
{
int ch = getchar();
eatLine();
while(strchr(arr, ch) == NULL)
{
printf("Error, please input %s\n", arr);
ch = getchar();
eatLine();
}
return ch;
}
#define LEN1 6
int showMenu(void)
{
int ch;
const char* pt[LEN1] = {"To choose a function, enter its letter label: ",
"a) +",
"b) -",
"c) *",
"d) /",
"e) Quit"};
const char option[LEN1] = "abcde";
for(int i = 0; i < LEN1; i++)
{
puts(pt[i]);
}
ch = getInupt(option);
return ch;
}
int main(void)
{
int num1, num2;
int ch;
void (*pFun[4])(int a, int b) = {sum, sub, mult, divide};
printf("Please input 2 num\n");
scanf("%d %d", &num1, &num2);
eatLine();
while((ch = showMenu()) != 'e')
{
switch(ch)
{
case 'a':pFun[0](num1, num2);break;
case 'b':pFun[1](num1, num2);break;
case 'c':pFun[2](num1, num2);break;
case 'd':pFun[3](num1, num2);break;
}
}
return 0;
}
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <stdbool.h>
#include <string.h>
#include <math.h>
#define SIZE 100
void transform(double source[], double target[], int size, double (*pFun)(double x))
{
for(int i = 0; i < size; i++)
{
target[i] = pFun(source[i]);
}
}
double fun1(double x)
{
return x * x;
}
double fun2(double x)
{
return x * x * x;
}
int main(void)
{
double source[SIZE];
double target[SIZE];
for(int i = 0; i < SIZE; i++)
{
source[i] = i;
}
transform(source, target, SIZE, sin);
for(int i = 0; i < SIZE; i++)
{
printf("%.2f ", target[i]);
}
printf("\n\n");
transform(source, target, SIZE, cos);
for(int i = 0; i < SIZE; i++)
{
printf("%.2f ", target[i]);
}
printf("\n\n");
transform(source, target, SIZE, fun1);
for(int i = 0; i < SIZE; i++)
{
printf("%.2f ", target[i]);
}
printf("\n\n");
transform(source, target, SIZE, fun2);
for(int i = 0; i < SIZE; i++)
{
printf("%.2f ", target[i]);
}
printf("\n\n");
return 0;
}
|