1.异常
(1)try…(一个)except
try:
print('step1')
a = 3/0
print('step2')
except BaseException as e:
print('step3,异常')
print('产生的异常对象:',e)
print('e的类型:',type(e))
print('step3:异常处理结束后,继续执行后面的代码')
'''结果
step1
step3,异常
产生的异常对象: division by zero
e的类型: <class 'ZeroDivisionError'>
step3:异常处理结束后,继续执行后面的代码
'''
小例子:
try:
while True:
a = int(input('输入数字:'))
print(a)
if a == 88:
print('退出程序')
break
except BaseException as e:
print(e)
print('输入的不是数字')
'''结果
输入数字:q
invalid literal for int() with base 10: 'q'
输入的不是数字
'''
(2)try…(多个)except
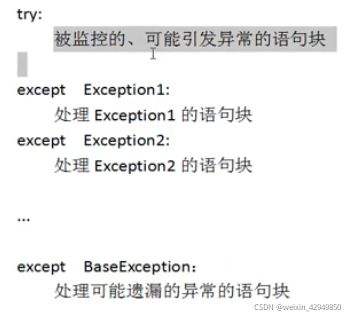
try:
a = input('输入被除数:')
b = input('输入除数:')
c = float(a)/float(b)
print(c)
except ZeroDivisionError:
print('异常,0不能做除数')
except ValueError:
print('异常,不能将字符串转为数字')
except BaseException:
print('其他异常')
(3). try…except… esle结构
try 之后有异常except,无异常else。再跳出这个模块执行后面的语句
(4)try…except…[else]… finally 结构
 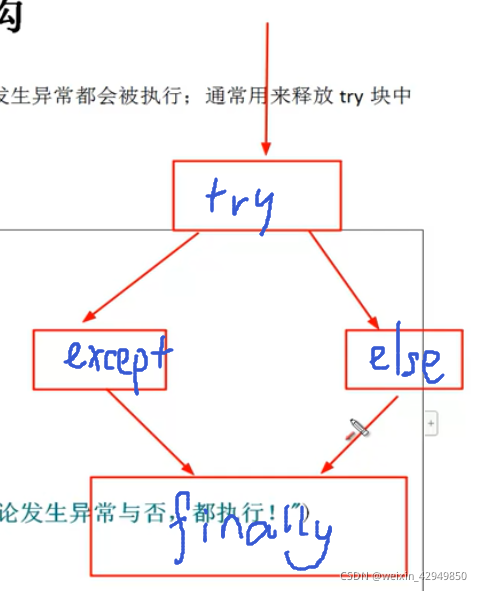
try:
f = open('G:\\huhu_boundary\\2019-3.txt','r')
content = f.readline()
print(content)
except:
print('no file')
finally:
try:
f.close()
except BaseException as e:
print(e)
print('程序结束')
(5)常见异常
SyntaxError:语法错误 NameError:尝试访问一个没有什么的变量 ZeroDivisionError:除数为0错误 ValueError:数值错误 TypeError:类型错误 AttributeError:访问对象的不存在属性 IndexError:索引越界异常 KeyError:字典的关键字不存在
2.with上下文管理器
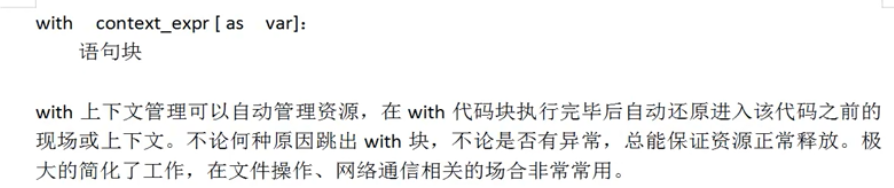
with open('G:\\huhu_boundary\\2019-3.txt') as f:
content = f.readline()
print(content)
print('程序结束')
3.traceback 模块
import traceback
try:
print('step1')
num = 1/0
except:
traceback.print_exc()
try:
print('step1')
num = 1/0
except:
with open('d:/a.txt','a') as f:
traceback.print_exc(file=f)
4.自定义异常类
通常继承Exception,命名用Error后缀
class AgeError(Exception):
def __init__(self,errorInfo):
Exception.__init__(self)
self.errorInfo = errorInfo
def __str__(self):
return str(self.errorInfo)+',年龄错误'
if __name__=='__main__':
age = int(input(' age:'))
if age<1 or age>100:
raise AgeError(age)
else:
print('无异常')
5.pycharm的调试模式
step over:跳过这个函数 step into:若当前是一个函数,则会进入这个函数 step out:若当前在函数内,则跳出函数 run to cursor:运行到光标处
|