Python实现虚拟画板
可以自由选择画笔颜色,在电脑上运行,实现画画。 开发环境:Pycharm 作品效果(分为三层,手指的轨迹,图形轨迹层,最终效果呈现层):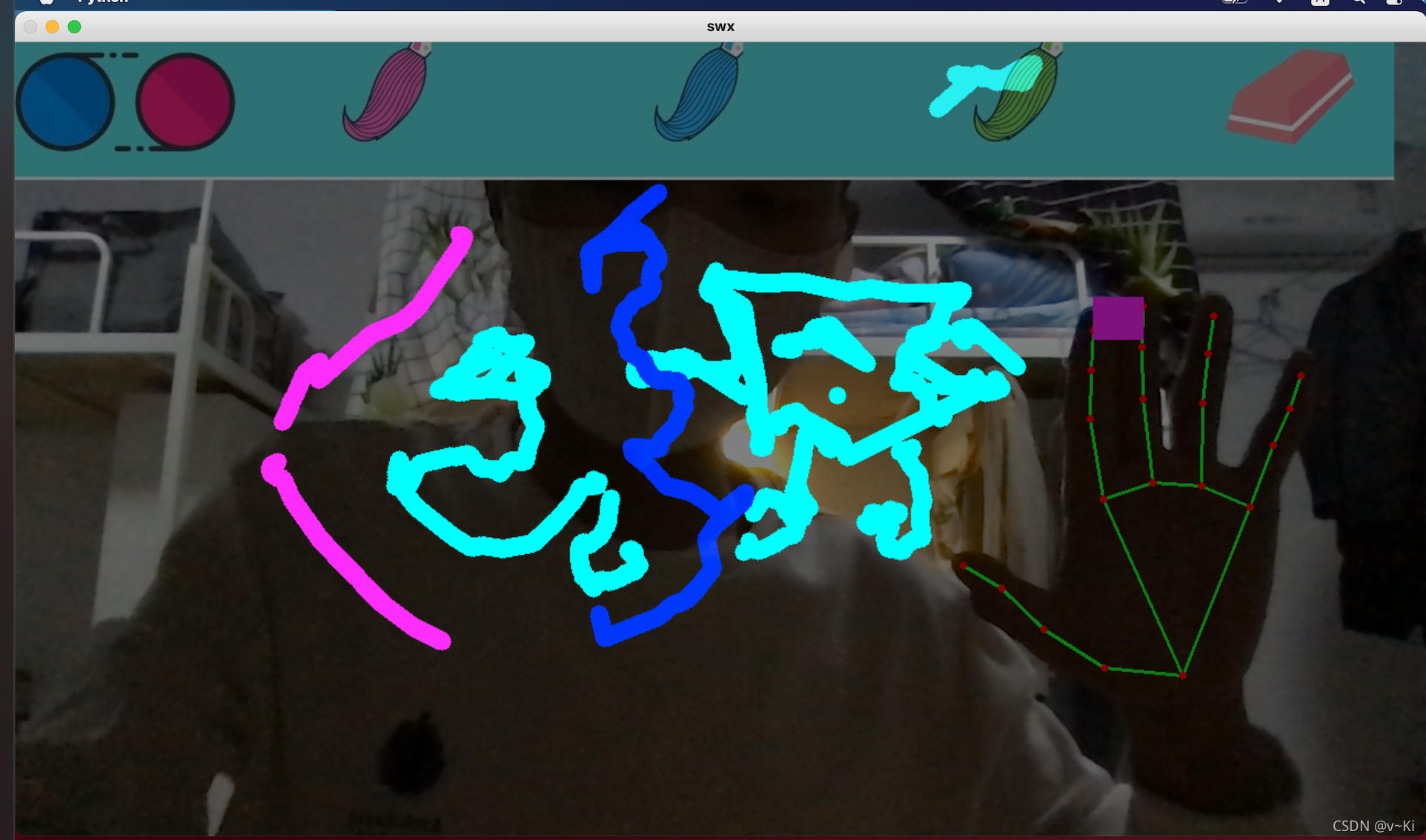
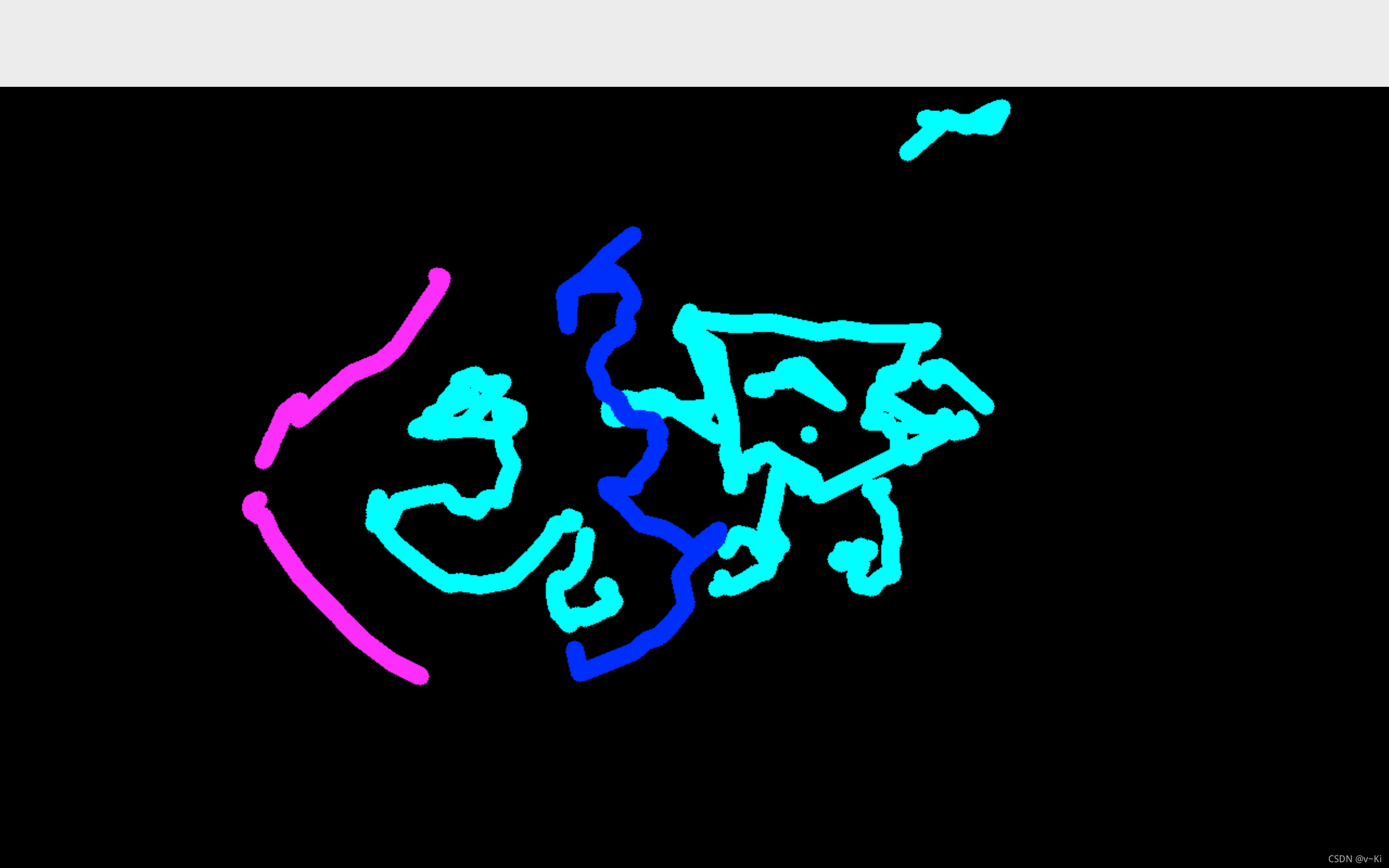
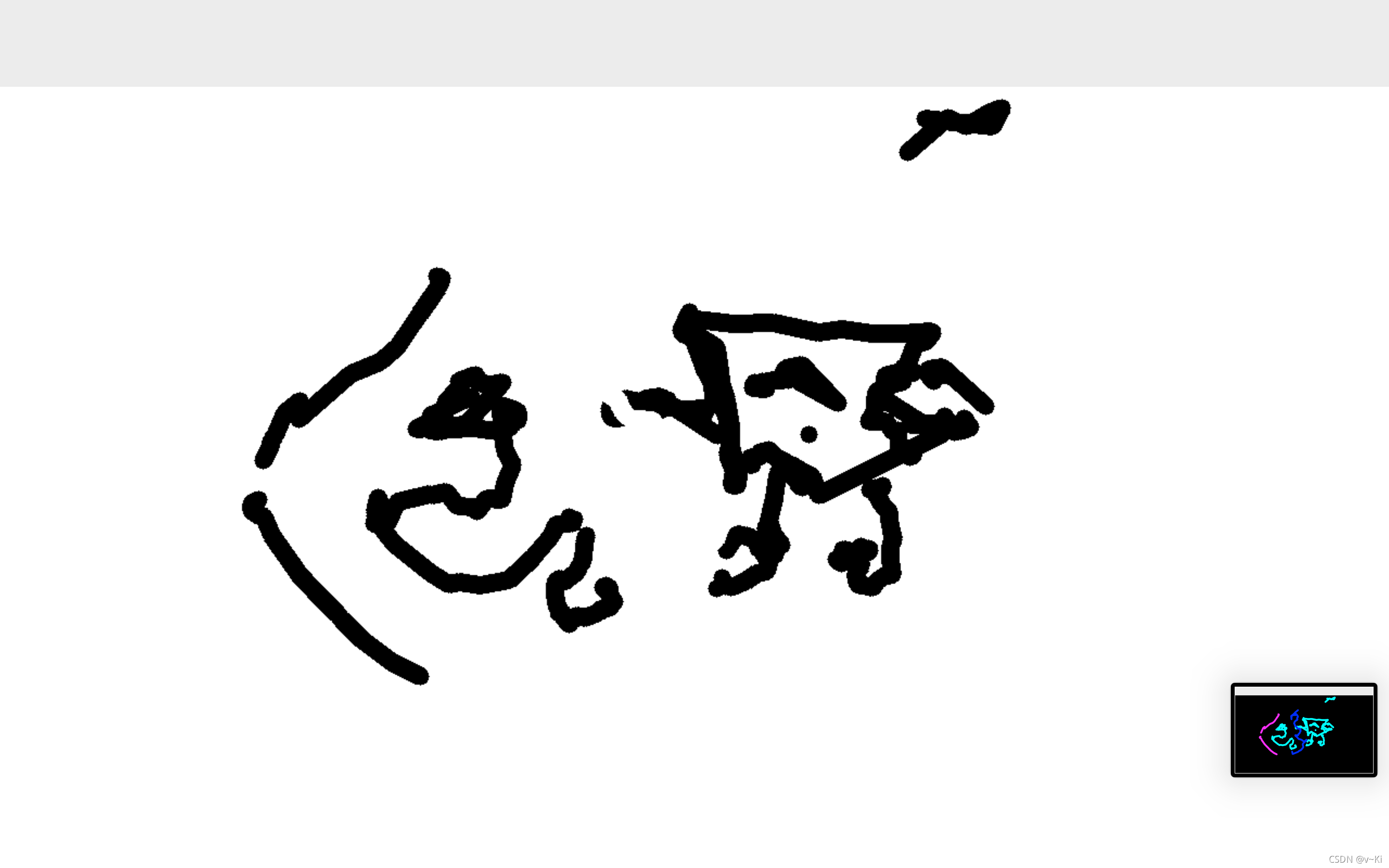
在Pycharm开发环境下需要提前导入以下几个库: cv2, numpy, mediapipe…
程序如下:
import cv2
import numpy as np
import time
import os
import HandTrackingModule as htm
brushThickness = 15
eraserThickness = 100
folderPath = "pic"
myList = os.listdir(folderPath)
print(myList)
overlayList = []
for imPath in myList:
image = cv2.imread(f'{folderPath}/{imPath}')
overlayList.append(image)
print(len(overlayList))
header = overlayList[0]
drawColor = (255,0, 255)
cap = cv2.VideoCapture(0)
cap.set(3, 1280)
cap.set(4, 720)
detector = htm.handDetector(detectionCon=0.85)
xp, yp = 0, 0
imgCanvas = np.zeros((720, 1280, 3), np.uint8)
while True:
success, img = cap.read()
img = cv2.flip(img, 1)
img = detector.findHands(img)
lmList = detector.findPosition(img, draw=False)
if len(lmList)!=0:
x1, y1 = lmList[8][1:]
x2, y2 = lmList[12][1:]
fingers = detector.fingersUp()
if fingers[1] and fingers[2]:
xp, yp = 0, 0
print("选择颜色")
if y1 < 125:
if 250 < x1 < 450:
header = overlayList[0]
drawColor = (255, 0, 255)
elif 550 < x1 < 750:
header = overlayList[1]
drawColor = (255, 0, 0)
elif 800 < x1 < 950:
header = overlayList[2]
drawColor = (255, 255, 0)
elif 1050 < x1 < 1200:
header = overlayList[3]
drawColor = (0, 0, 0)
cv2.rectangle(img, (x1, y1 - 30), (x2, y2 + 30), drawColor, cv2.FILLED)
if fingers[1] and fingers[2] == False:
cv2.circle(img, (x1, y1), 25, drawColor, cv2.FILLED)
print("开始绘图")
if xp == 0 and yp == 0:
xp, yp = x1, y1
if drawColor == (0, 0, 0):
cv2.line(img, (xp, yp), (x1, y1), drawColor, eraserThickness)
cv2.line(imgCanvas, (xp, yp), (x1, y1), drawColor, eraserThickness)
else:
cv2.line(img, (xp, yp), (x1, y1), drawColor, brushThickness)
cv2.line(imgCanvas, (xp, yp), (x1, y1), drawColor, brushThickness)
xp, yp = x1, y1
imgGray = cv2.cvtColor(imgCanvas, cv2.COLOR_BGR2GRAY)
_, imgInv = cv2.threshold(imgGray, 50, 255, cv2.THRESH_BINARY_INV)
imgInv = cv2.cvtColor(imgInv, cv2.COLOR_GRAY2BGR)
img = cv2.bitwise_and(img, imgInv)
img = cv2.bitwise_or(img, imgCanvas)
img[0:125, 0:1250] = header
img = cv2.addWeighted(img, 0.5, imgCanvas, 0.5, 0)
cv2.imshow("swx", img)
cv2.imshow("Canvas", imgCanvas)
cv2.imshow("sVINx", imgInv)
cv2.waitKey(1)
import cv2
import mediapipe as mp
import time
class handDetector():
def __init__(self, mode=False, maxHands = 2, detectionCon = 0.5, trackCon = 0.5):
self.mode = mode
self.maxHands = maxHands
self.detectionCon = detectionCon
self.trackCon = trackCon
self.mpHands = mp.solutions.hands
self.hands = self.mpHands.Hands(self.mode, self.maxHands,
self.detectionCon, self.trackCon)
self.mpDraw = mp.solutions.drawing_utils
self.tipIds = [4, 8, 12, 16, 20]
def findHands(self, img, draw=True):
imgRGB = cv2.cv2.cvtColor(img, cv2.COLOR_RGB2BGR)
self.results = self.hands.process(imgRGB)
if self.results.multi_hand_landmarks:
for handLms in self.results.multi_hand_landmarks:
if draw:
self.mpDraw.draw_landmarks(img, handLms, self.mpHands.HAND_CONNECTIONS)
return img
def findPosition(self, img, handNo = 0, draw=True):
self.lmList = []
if self.results.multi_hand_landmarks:
myHand = self.results.multi_hand_landmarks[handNo]
for id, lm in enumerate(myHand.landmark):
h, w, c = img.shape
cx, cy = int(lm.x * w), int(lm.y * h)
self.lmList.append([id, cx, cy])
if draw:
cv2.circle(img, (cx, cy), 5, (255, 0, 0), cv2.FILLED)
return self.lmList
def fingersUp(self):
fingers = []
if self.lmList[self.tipIds[0]][1] < self.lmList[self.tipIds[0] - 1][1]:
fingers.append(1)
else:
fingers.append(0)
for id in range(1, 5):
if self.lmList[self.tipIds[id]][2] < self.lmList[self.tipIds[id] - 2][2]:
fingers.append(1)
else:
fingers.append(0)
return fingers
def main():
cTime = 0
pTime = 0
cap = cv2.VideoCapture(0)
detector = handDetector()
while True:
success, img = cap.read()
img = detector.findHands(img)
lmList = detector.findPosition(img)
if len(lmList) !=0:
print(lmList[4])
cTime = time.time()
fps = 1 / (cTime - pTime)
pTime = cTime
cv2.putText(img, str(int(fps)), (10, 70), cv2.FONT_HERSHEY_PLAIN, 3,
(0, 0, 255), 3)
cv2.imshow("svinx", img)
cv2.waitKey(1)
if __name__ == "__main__":
main()
|