引言
备忘录模式经常可以遇到,譬如下面这些场景:
备忘录模式(Memento Pattern)
在不破坏封装的前提下,捕获一个对象的内部状态,并在该对象之外保存这个状态,这样可以在以后将对象恢复到原先保存的状态。它是一种对象行为型模式,其别名为Token。
角色
备忘录模式的核心是备忘录类以及用于管理备忘录的负责人类的设计。
说明:如果希望保存多个originator对象的不同时间的状态,也可以,只需要 HashMap <String, 集合>
为什么会出现守护者对象(负责人)?
举个例子说明,下棋软件要提供“悔棋”功能,用户走错棋或操作失误后可恢复到前一个步骤。悔棋可能回到上一步,也有可能回到上上次的状态…因此需要记录多次的状态
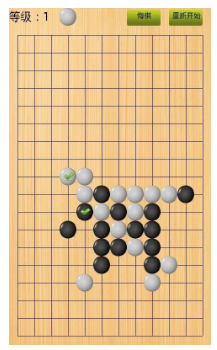
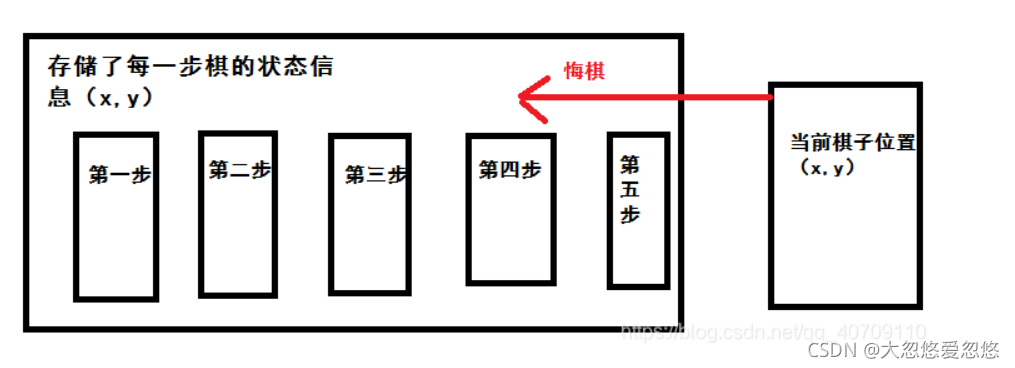 在设计备忘录类时需要考虑其封装性,除了Originator类,不允许其他类来调用备忘录类Memento的构造函数与相关方法 ,如果不考虑封装性,允许其他类调用setState()等方法,将导致在备忘录中保存的历史状态发生改变,通过撤销操作所恢复的状态就不再是真实的历史状态,备忘录模式也就失去了本身的意义。
备忘录模式实现框架
originator : 对象(需要保存状态的对象)
public class Originator {
private String state;
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public Memento saveStateMemento(){
return new Memento(state);
}
public void getStateFromMemento (Memento memento){
state = memento.getState();
}
}
Memento : 备忘录对象,负责保存好记录,即Originator内部状态
public class Memento {
private String state;
public Memento(String state) {
this.state = state;
}
public String getState() {
return state;
}
}
Caretaker: 守护者对象,负责保存多个备忘录对象, 使用集合管理,提高效率
public class Caretaker {
private List<Memento> mementoList = new ArrayList<>();
public void add(Memento memento) {
mementoList.add(memento);
}
public Memento get(int index){
return mementoList.get(index);
}
}
下棋案例
棋子类 Chessman,原发器角色
@Data
@AllArgsConstructor
public class Chessman
{
private String label;
private Integer x,y;
public ChessmanMemento save()
{
return new ChessmanMemento(label,x,y);
}
public void restore(ChessmanMemento chessmanMemento)
{
this.label=chessmanMemento.getLabel();
this.x=chessmanMemento.getX();
this.y=chessmanMemento.getY();
}
public void show()
{
System.out.println(
String.format("棋子: %s ,位置: [%d,%d]",label,x,y)
);
}
}
备忘录角色 ChessmanMemento
@Data
@AllArgsConstructor
public class ChessmanMemento
{
private String label;
private Integer x,y;
}
负责人角色 MementoCaretaker
public class MementoCaretaker
{
Integer index=-1;
List<ChessmanMemento> chessmanMementoLinkedList= Lists.newLinkedList();
public ChessmanMemento getMemento()
{
if(index<=0)
{
throw new IndexOutOfBoundsException("已经无棋可悔了");
}
this.index--;
chessmanMementoLinkedList = chessmanMementoLinkedList.stream()
.limit(this.index+1).collect(Collectors.toList());
return chessmanMementoLinkedList.get(index);
}
public void addMemento(ChessmanMemento chessmanMemento)
{
index++;
chessmanMementoLinkedList.add(chessmanMemento);
}
}
棋子客户端,维护了一个 MementoCaretaker 对象
public class Client
{
MementoCaretaker mementoCaretaker=new MementoCaretaker();
public void play(Chessman chessman)
{
mementoCaretaker.addMemento(chessman.save());
}
public void undo(Chessman chessman)
{
ChessmanMemento memento = mementoCaretaker.getMemento();
chessman.restore(memento);
}
}
测试
public class Test
{
public static void main(String[] args) {
Chessman chessman=new Chessman("车",1,1);
Client client=new Client();
client.play(chessman);
chessman.show();
chessman=new Chessman("马",2,0);
client.play(chessman);
chessman.show();
client.undo(chessman);
chessman.show();
client.undo(chessman);
chessman.show();
}
}
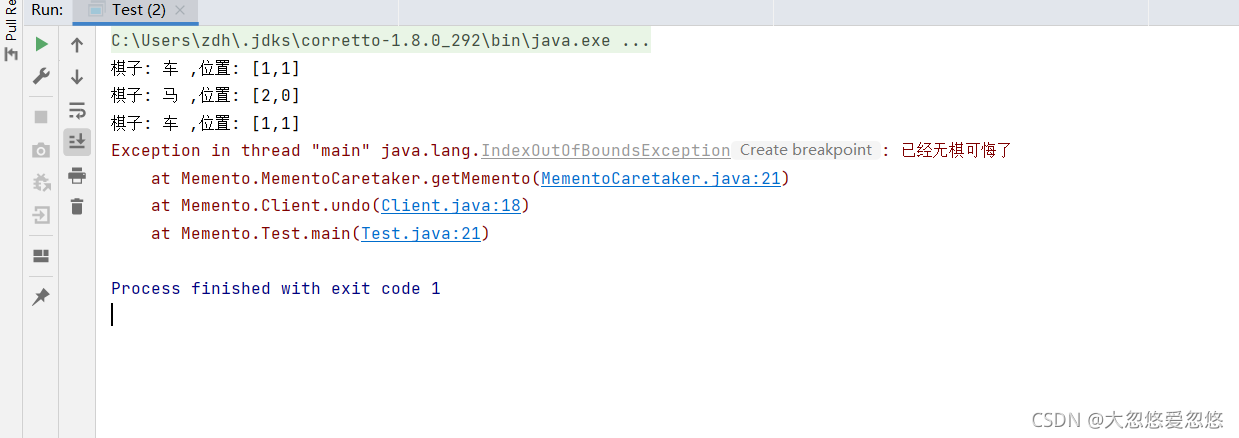
备忘录模式总结
优点
缺点
适用场景
注意细节
参考文章
备忘录模式
|