.
官网:http://rapidjson.org/zh-cn/ 开发环境:VS code 2019
一、环境配置
1、下载rapidjson
下载网址:https://github.com/Tencent/rapidjson/ 下载完成之后,打开目录,可以看到以下文件,其中include是主要文件,也是最重要的文件 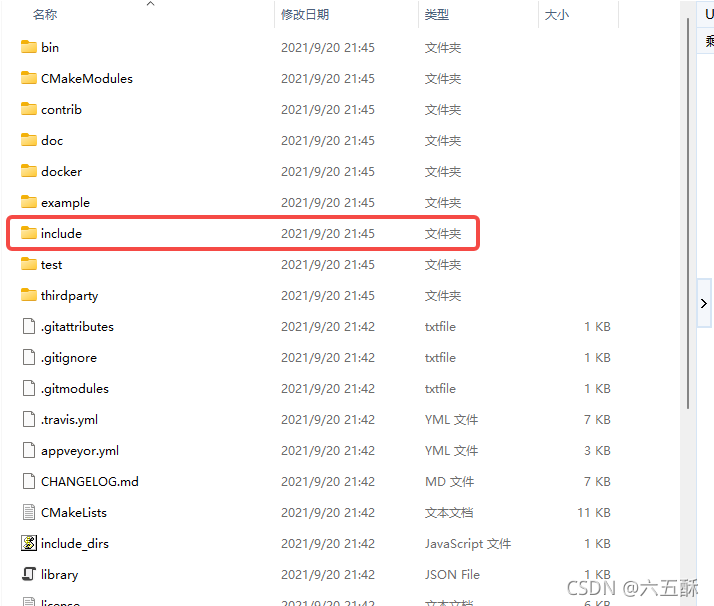 可以将文件移动到工程项目里面
2、添加库的链接
打开调试属性 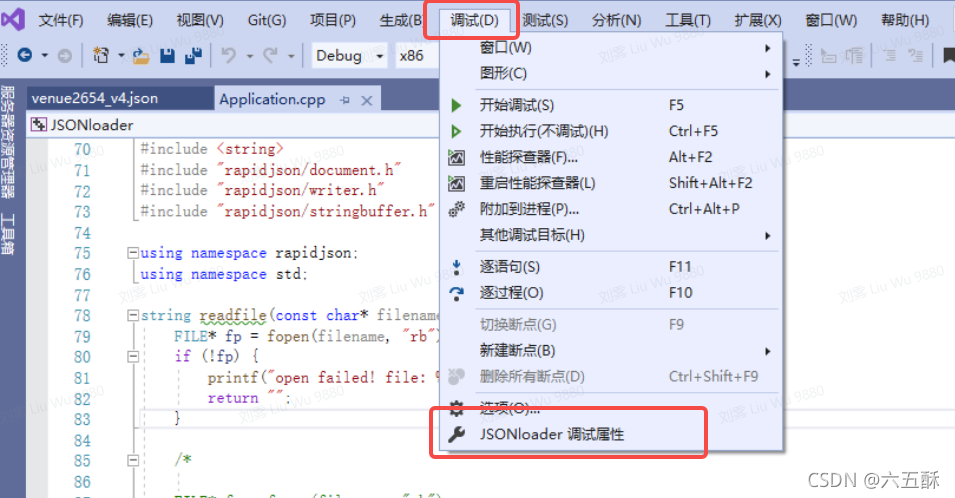 选择VC++目录->包含目录 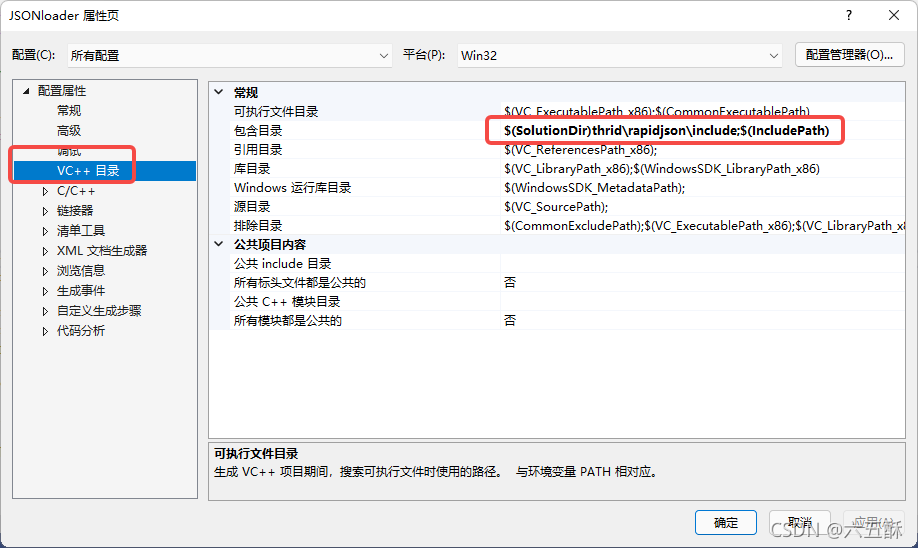 将上面的include目录包含进来,即可使用了,$(SolutionDir)表示当前的工程目录 $(SolutionDir)thrid\rapidjson\include 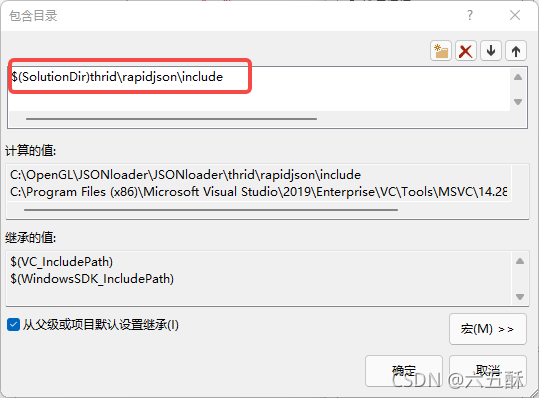
二、rapidjson例程
读取JSON文件,获取对应元素
#include <iostream>
#include <string>
#include "rapidjson/document.h"
#include "rapidjson/writer.h"
#include "rapidjson/stringbuffer.h"
using namespace rapidjson;
using namespace std;
string readfile(const char* filename) {
FILE* fp = fopen(filename, "rb");
if (!fp) {
printf("open failed! file: %s", filename);
return "";
}
/*
FILE* fp = fopen(filename, "rb");
if (!fp) {
printf("open failed! file: %s", filename);
return "";
}
char* buf = new char[1024 * 16];
int n = fread(buf, 1, 1024 * 16, fp);
fclose(fp);
string result;
if (n >= 0) {
result.append(buf, 0, n);
}
delete[]buf;
*/
char buf[1024 * 16]; //新建缓存区
string result;
/*循环读取文件,直到文件读取完成*/
while (int n = fgets(buf, 1024 * 16, fp) != NULL)
{
//int len = strlen(buf);
//buf1[len - 1] = '\0'; /*去掉换行符*/
//printf("%s %d \n", buf, len - 1);
result.append(buf);
//cout << buf << endl;
}
fclose(fp);
//cout << result << endl;
return result;
}
int parseJSON(const char* jsonstr) {
Document d;
if (d.Parse(jsonstr).HasParseError()) {
printf("parse error!\n");
return -1;
}
if (!d.IsObject()) {
printf("should be an object!\n");
return -1;
}
if (d.HasMember("errorCode")) {
Value& m = d["errorCode"];
int v = m.GetInt();
printf("errorCode: %d\n", v);
}
printf("show numbers: \n");
if (d.HasMember("numbers")) {
Value& m = d["numbers"];
if (m.IsArray()) {
for (int i = 0; i < m.Size(); i++) {
Value& e = m[i];
int n = e.GetInt();
printf("%d,", n);
}
}
}
return 0;
}
int parseJSON2(const char* jsonstr) {
Document d;
if (d.Parse(jsonstr).HasParseError()) {
throw string("parse error!\n");
}
if (!d.IsObject()) {
throw string("should be an object!\n");
}
/*判断是否存在Joints*/
if (!d.HasMember("Joints")) {
throw string("'Joints' no found!");
}
/*查看Joints的个数和数据类型*/
Value& j = d["Joints"];
cout << typeid(j).name() << endl;
cout << j.Size() << endl;
/*查看Joints下第一个Joints的ID*/
Value& m = d["Joints"][0];
m.HasMember("ID");
if (m.IsObject()){
cout << "ID" << endl;
if (m["ID"].IsInt()) {
int ID = m["ID"].GetInt();
cout << ID << endl;
}
}
//string v = m.GetString();
//printf("errorCode: %d\n", v);
//printf("show numbers:\n");
/*
if (d.HasMember("numbers")) {
Value& m = d["numbers"];
if (m.IsArray()) {
for (int i = 0; i < m.Size(); i++) {
Value& e = m[i];
int n = e.GetInt();
printf("%d", n);
}
}
}
*/
return 0;
}
/*
//path="/Users/macname/Desktop/example.json"
{
"errorCode":0,
"reason":"OK",
"result":{"userId":10086,"name":"中国移动"},
"numbers":[110,120,119,911]
}
*/
int main() {
string jsonstr = readfile("C:/OpenGL/JSONloader/JSONloader/JSONloader/venue2654_v4.json");
//string jsonstr = readfile("C:/OpenGL/JSONloader/JSONloader/JSONloader/test.txt");
//parseJSON(jsonstr.c_str());
try {
parseJSON2(jsonstr.c_str());
}
catch (string e) {
printf("error: %s \n", e.c_str());
}
getchar();
return 0;
}
简洁版
#include <iostream>
#include <string>
#include "rapidjson/document.h"
#include "rapidjson/writer.h"
#include "rapidjson/stringbuffer.h"
using namespace rapidjson;
using namespace std;
string readfile(const char* filename) {
FILE* fp = fopen(filename, "rb");
if (!fp) {
printf("open failed! file: %s", filename);
return "";
}
char buf[1024 * 16]; //新建缓存区
string result;
/*循环读取文件,直到文件读取完成*/
while (int n = fgets(buf, 1024 * 16, fp) != NULL)
{
result.append(buf);
//cout << buf << endl;
}
fclose(fp);
return result;
}
int parseJSON2(const char* jsonstr) {
Document d;
if (d.Parse(jsonstr).HasParseError()) {
throw string("parse error!\n");
}
if (!d.IsObject()) {
throw string("should be an object!\n");
}
/*判断是否存在Joints*/
if (!d.HasMember("Joints")) {
throw string("'Joints' no found!");
}
/*查看Joints的个数和数据类型*/
Value& j = d["Joints"];
cout << typeid(j).name() << endl;
cout << j.Size() << endl;
/*查看Joints下第一个Joints的ID*/
Value& m = d["Joints"][0];
m.HasMember("ID");
if (m.IsObject()){
cout << "ID" << endl;
if (m["ID"].IsInt()) {
int ID = m["ID"].GetInt();
cout << ID << endl;
}
}
return 0;
}
int main() {
string jsonstr = readfile("C:/OpenGL/JSONloader/JSONloader/JSONloader/venue2654_v4.json");
try {
parseJSON2(jsonstr.c_str());
}
catch (string e) {
printf("error: %s \n", e.c_str());
}
return 0;
}
|