python学习资料: http://www.pythondoc.com/pythontutorial3/index.html#
现在开始学习吧~
有活力的思想是永远不会枯竭的。 ——德·叔本华
1. 常用字符类型:int/float
2. 常用运算符:
(1)= (2)+ (3)- (4)* (5)/ (浮点除) (6)// (整除) (7)% (8)** (9)() (10)== (11)!=
3. 使用type()函数查看变量类型
d = 10
type(d)
4. 字符串操作
(1)字符串的转义和连接
str1 = "h\nh"
str2 = "y\\ny"
str3 = r"l\nl"
print(str1+str2+str3)
 (2)字符串的索引和切片
str1 = "hello world"
print(str1+"取str1[0]为"+str1[0])
print(str1+"取str1[1:]为"+str1[1:])
print(str1+"取str1[-1]为"+str1[-1])
print(str1+"取str1[:-1]为"+str1[:-1])
print(str1+"取str1[1::2]为"+str1[1::2])
for i in range(0, len(str1)):
print(str1[i], end="*")
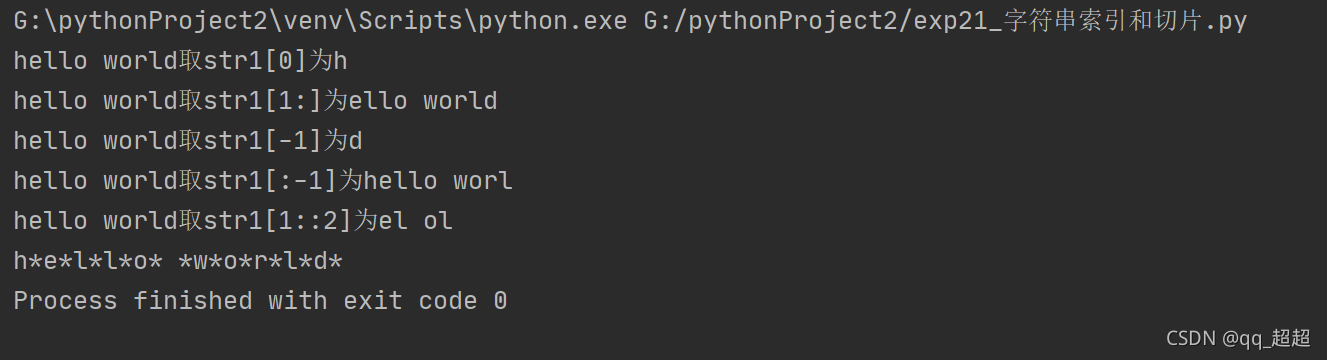 列表的索引和切片同字符串
list1 = [1, 2, 3, "a", "b", "abc"]
print(list1[0])
print(list1[1:])
print(list1[-1])
print(list1[:-1])
print(list1[1::2])
for i in range(0, len(list1)):
print(list1[i], end="*")
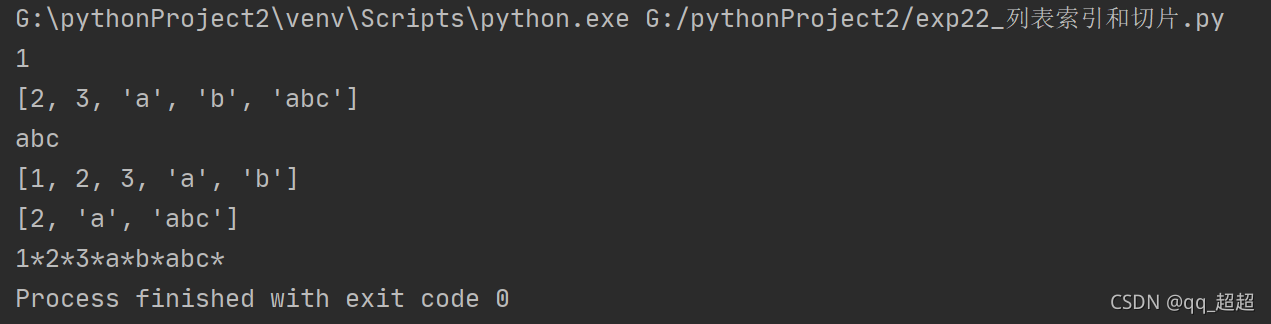
5. 列表、元组、字典、集合
(1)列表常见操作
list1 = [1, 2, 3, 6, 9, 0, 5, 7]
print(list1)
list1.insert(2, 8)
print(list1)
list1.remove(5)
print(list1)
i = list1.pop(1)
print(i)
print(list1.index(7))
list1.sort(reverse=True)
print(list1)
list1.sort()
print(list1)
j = [a**2 for a in range(0, 5)]
print(j, end=" ")
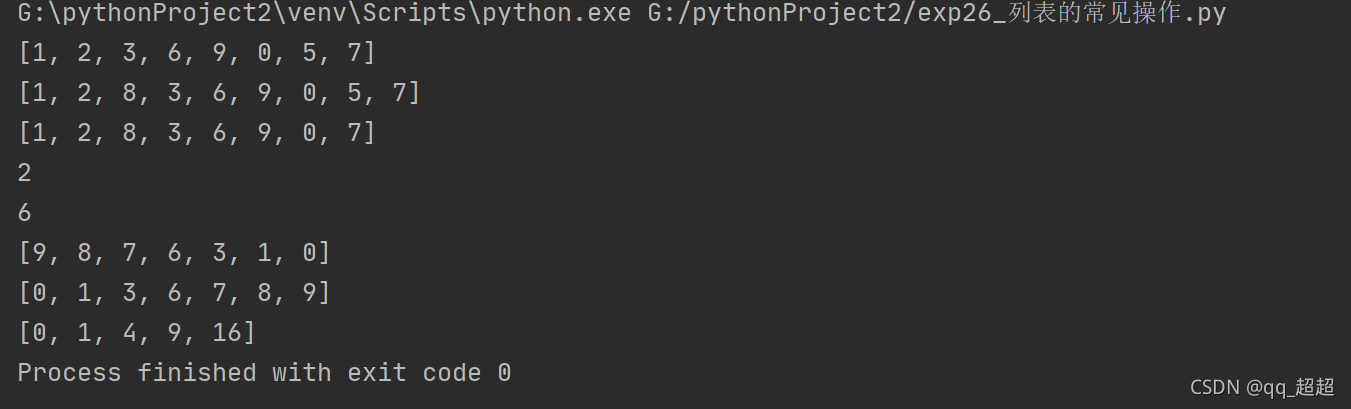 (2)元组
tuple1 = (1, 2, 3, 3)
print(tuple1.count(5))
print(tuple1.index(3))
 (3)字典
dict1 = {"a": 1, "b": 2, "c": 3}
dict2 = dict(e=4, f=5, g=6)
print(dict1)
print(dict2)
print(dict1.keys())
print(dict1.values())
print(dict1.pop("a"))
print(dict1.popitem())
print(dict1)
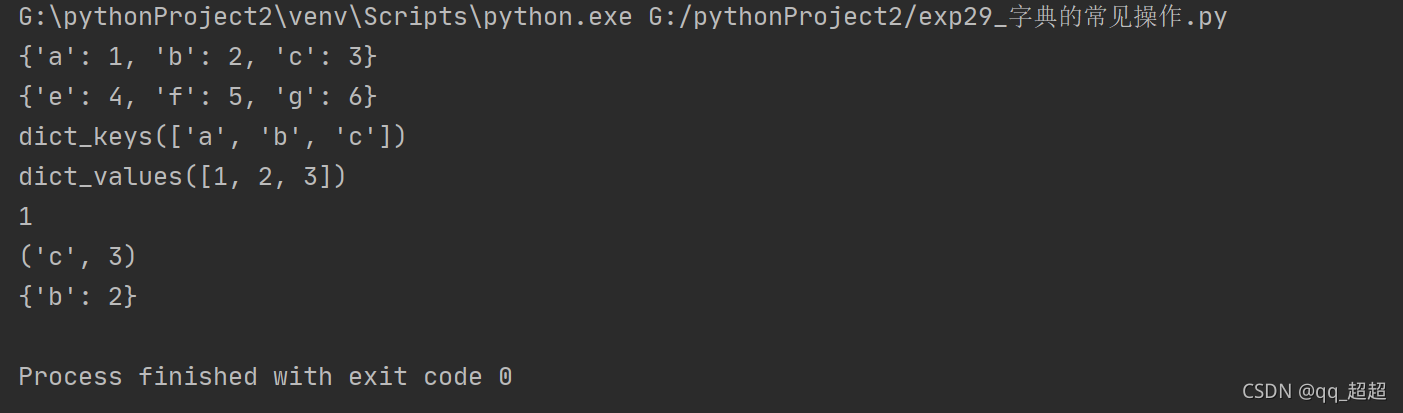 (4)集合
a = {1, 2, 10}
b = set()
c = {1, 2, 3, 4}
print(type(a), type(b))
print(a.union(c))
print(a.intersection(c))
print(a.difference(c))
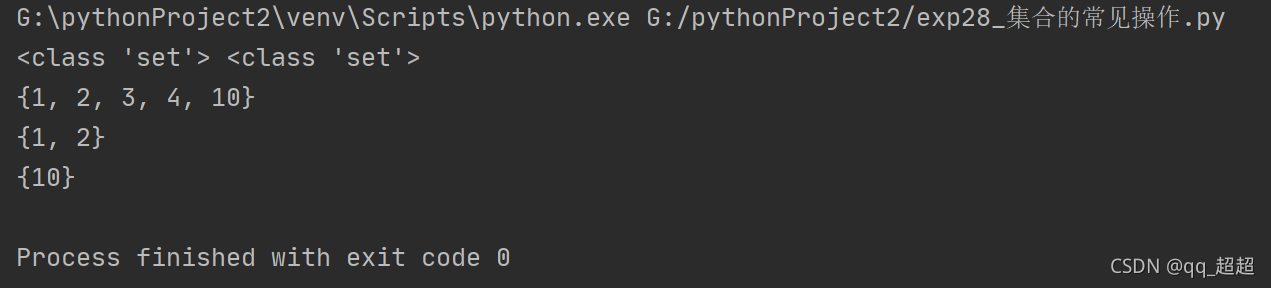
6. 判断语句和循环语句
(1)判断语句 if / elif / else
a = 90
if a < 60:
print("不及格")
elif a < 80:
print("及格但不优秀")
elif a < 90:
print("优秀但不杰出")
else:
print("杰出")
(2)循环语句for / while
for i in range(0, 10, 2):
print(i, end=".")
print("\n")
list1 = ["a", "b", "c", "d"]
for j in list1:
print(j, end=".")
print("\n")
a = 3
while a > 0:
print(a, end="*")
a -= 1
print("\n")
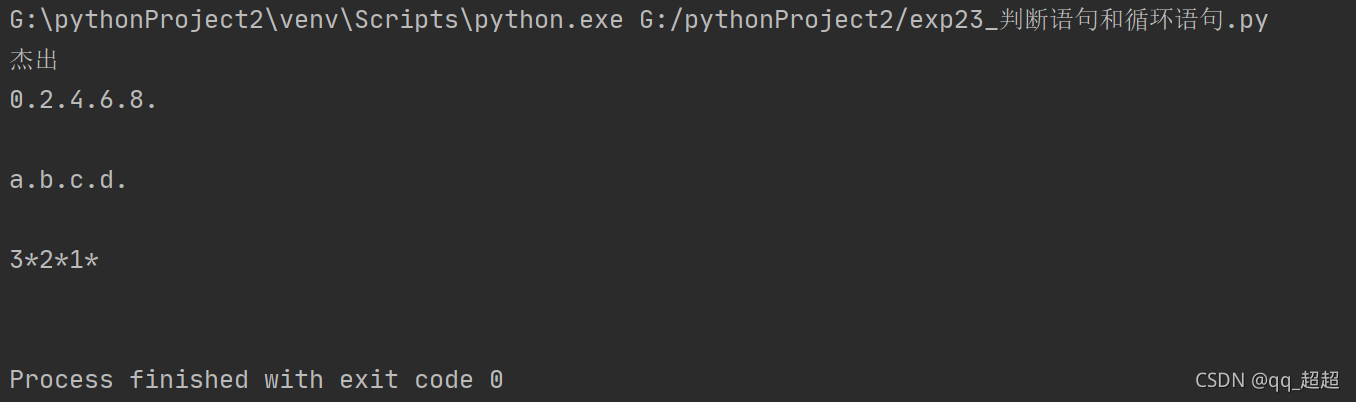
7.函数
(1)函数的定义和调用
"""
函数定义:
以def关键字开头,后面跟上函数名和参数,参数可为空或者默认参数
可以return或者不return,不写return默认返回None
"""
def fun_1(a, b=9):
"""
函数说明
"""
return a + b
"""
函数调用
"""
print(fun_1(3))
print(fun_1(3, 8))
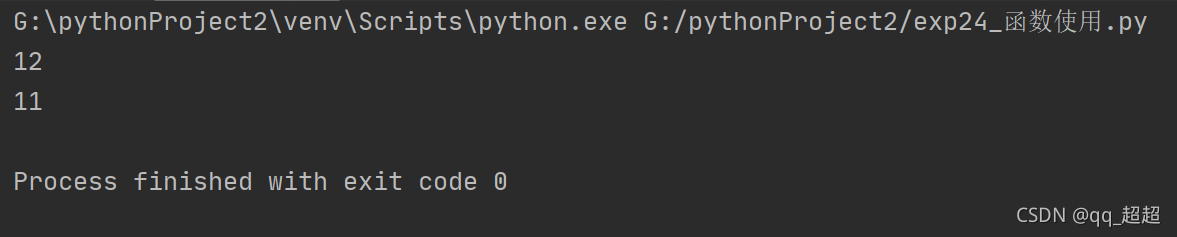
(2)匿名函数(Lambda函数)
"""
匿名函数lambda表达式
用于简单的代码块的封装
让程序看起来更简单
"""
fun_2 = lambda a, b: a * b
print(fun_2(2, 4))

8.python格式化输出的三种方式
name = "wang xiao li"
age = 20
weight = 45
print("My name is %s" % name)
print("My name is %s, my age is %d, my weight is %.2f" % (name, age, weight))
list1 = ["apple", "orange", "peach"]
dict1 = {"class": 301, "teacher": "Zhang san"}
print("My name is {}".format(name))
print("My name is {},My age is {},My weight is {}".format(name, age, weight))
print("My list is {}, My dict is {}".format(list1, dict1))
print("My list is {},{},{}".format(*list1))
print("My dict is {class},{teacher}".format(**dict1))
print(f"My name is {name}")
print(f"My name is {name},My age is {age},My weight is {weight}")
print(f"My list is {list1[0]}")
print(f"My dict is {dict1['class']}")
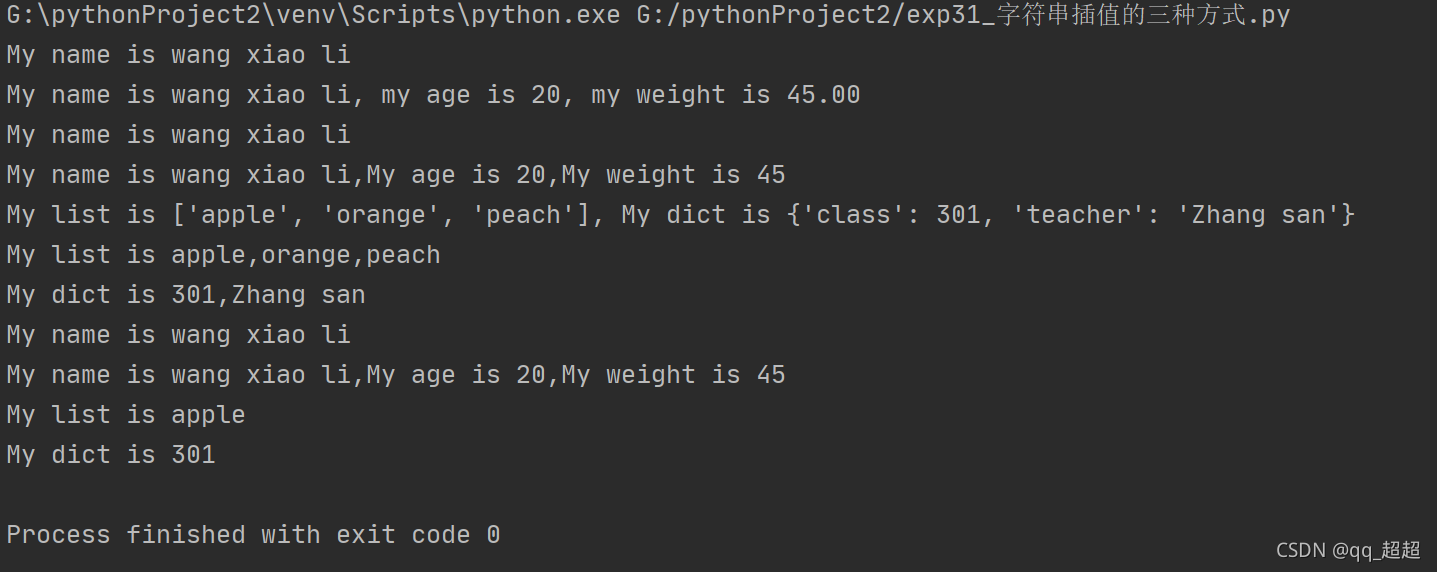
9.文件操作
三个步骤: (1)打开文件 (2)操作文件 (3)关闭文件
f = open("G:\\pythonProject2\\1.txt", "r")
while True:
every = f.readline()
if every != "\n":
print(every, end="")
else:
continue
f.close()
"""
也可以使用with语句块,就不用单独再去编写close语句。执行之后会自动关闭文件
with open("G:\\pythonProject2\\1.txt", "r") as f:
print(f.readlines())
"""
10. json格式和python格式转换
dumps()和loads()函数
import json
data = {
"name": "wangwu",
"age": 5,
"teacher": ["Jerry", "Tom", "Alice"]
}
print(type(data))
data1 = json.dumps(data)
print(data1)
print(type(data1))
data2 = json.loads(data1)
print(data2)
print(type(data2))
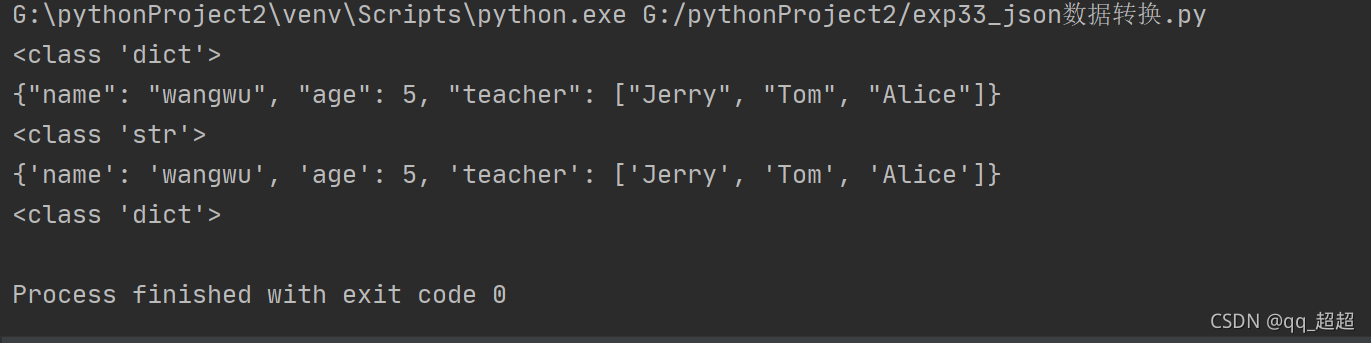
11.python异常处理流程
try:
num1 = int(input())
num2 = int(input())
print(num1 / num2)
except ZeroDivisionError:
print("出现被除数为0的异常")
except ValueError:
print("参数类型错误")
except:
print("遇见任何异常都会执行这个语句块")
else:
print("没有出现异常")
finally:
print("无论是否异常都执行该语句块")
class MyException(Exception):
def __init__(self, value1):
self.value1 = value1
raise MyException("value1")
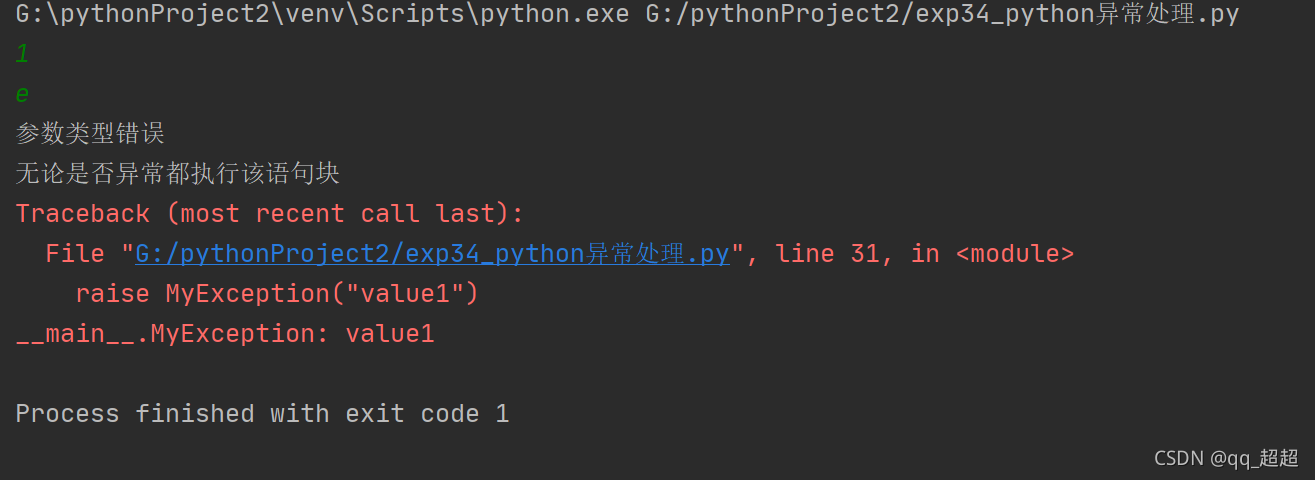
12.面向对象编程:类的定义和使用
class Human:
name = "unknown"
age = 0
sex = "unknown"
def __init__(self, name, age, sex):
self.name = name
self.age = age
self.sex = sex
@classmethod
def eat(self):
print("i am eating")
def jump(self):
print("i am jumping")
my_human = Human("zhangsi", 12, "female")
print(f"姓名是{my_human.name}, 年龄是{my_human.age}, 性别是{my_human.sex}")
print(f"姓名是{Human.name}, 年龄是{Human.age}, 性别是{Human.sex}")
print(f"{my_human.eat()}")
print(f"{Human.eat()}")
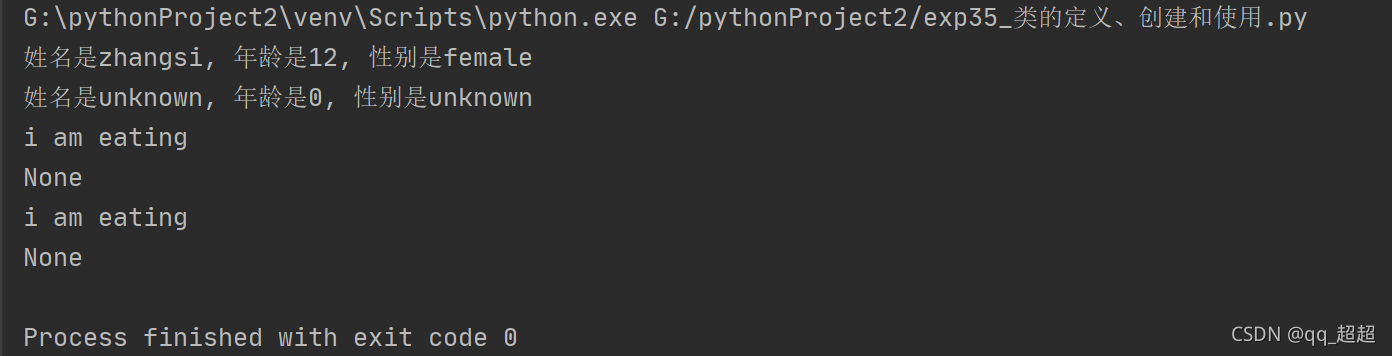 (不知道为什么有None输出)
13.python常用标准库:os \ math \ time \ urllib
import os
import time
import urllib.request
import math
print(math.floor(5.5))
print(math.ceil(5.5))
print(math.sqrt(9))
os.mkdir("testdir")
os.removedirs("testdir")
print(os.listdir("./"))
print(os.getcwd())
print(os.path.exists("test"))
print(time.asctime())
print(time.time())
print(time.sleep(3))
print(time.localtime())
print(time.strftime("%Y-%m-%d %H:%M:%S", time.localtime()))
response = urllib.request.urlopen("http://www.baidu.com")
print(response.status)
print(response.read())
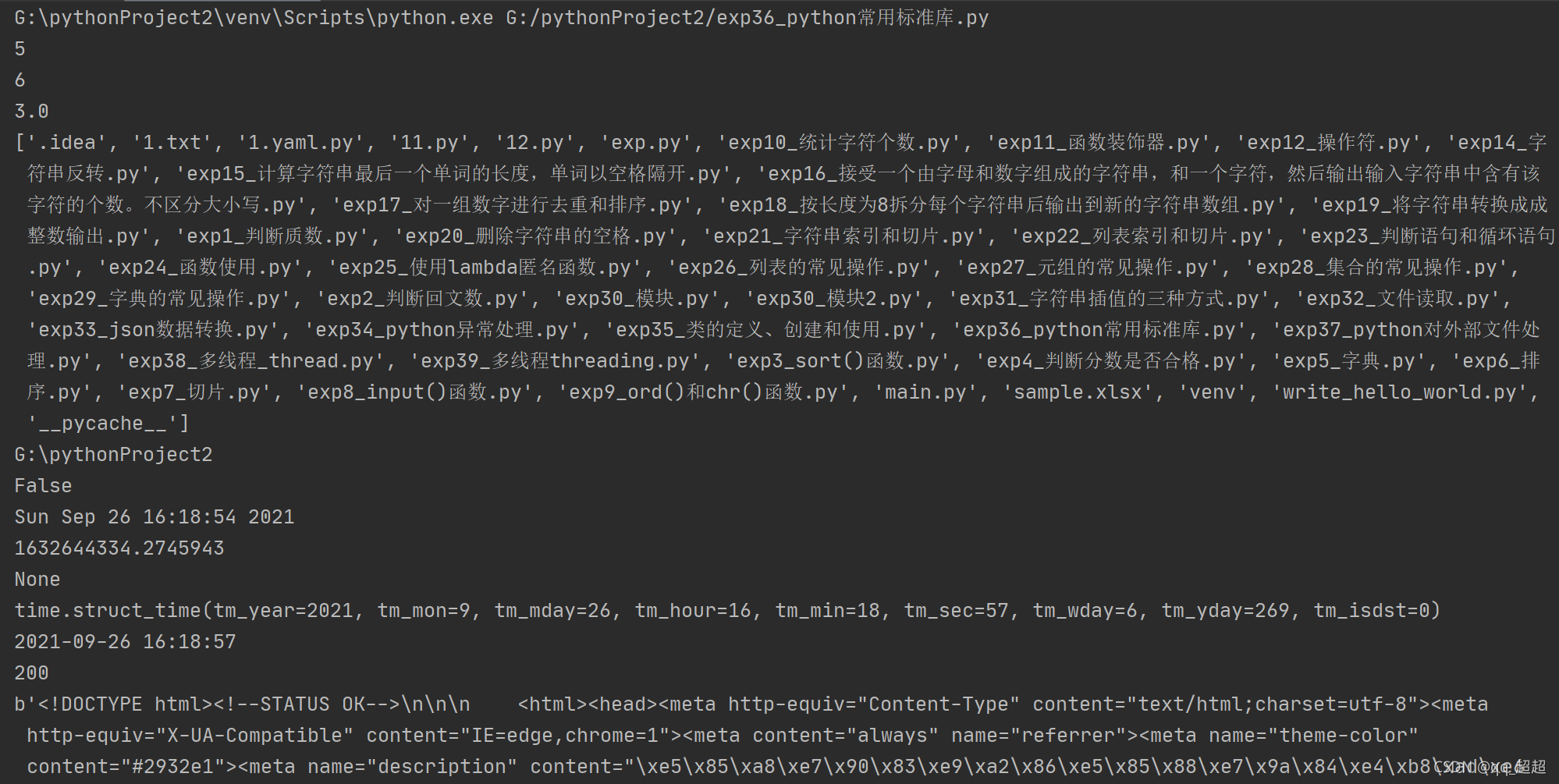
14.python多线程
(1)进程:指的是执行中的程序,拥有独立的地址空间、内存、数据栈,由操作系统管理。多进程可以并行。 进程之间通过IPC通信。 (2)线程:同一进程下执行,多线程可以并发进行(并发,指的是同一时刻只有一个线程执行),且多个线程的资源是共享的。数据需要同步原语(比如锁、GIL),来保证多个线程间安全进行。 (3)GIL保证了同一时刻只有一个线程执行。 (4)python如何实现多线程:_thread和threading。 ○ _thread:基本的线程和锁,使用_thread包实现多线程,需额外自己写锁的代码。 ○ threading:更高级的操作,进行了一些封装。比如不需要自己额外写锁的代码。 (5)锁的意义:执行完成后再kill掉线程
(1)使用_thread包 实现多线程
import _thread
import time
from time import sleep
import logging
logging.basicConfig(level=logging.INFO)
def loop(nloop, nsleep, lock):
logging.info(f"{nloop} start at {time.asctime()}")
sleep(nsleep)
logging.info(f"{nloop} end at {time.asctime()}")
lock.release()
def main():
logging.info(f"start all at {time.asctime()}")
locks = []
loop_time = [2, 4]
nloop = range(len(loop_time))
for i in nloop:
lock = _thread.allocate_lock()
lock.acquire()
locks.append(lock)
for i in nloop:
_thread.start_new_thread(loop, (i, loop_time[i], locks[i]))
for i in nloop:
while locks[i].locked():
pass
logging.info(f"end all at {time.asctime()}")
if __name__ == "__main__":
main()
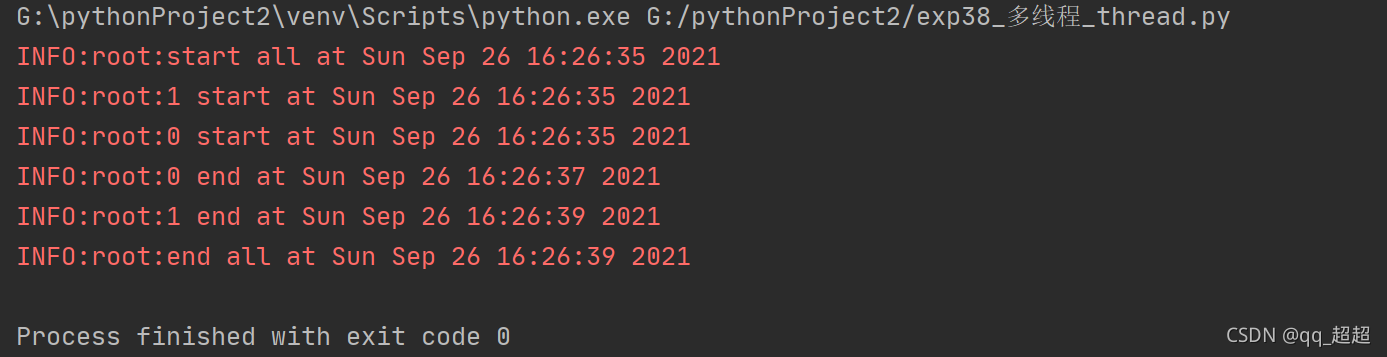 (2)使用threading包 实现多线程(创建了MyThread类,继承父类Thread)
import _thread
import threading
import time
from time import sleep
import logging
logging.basicConfig(level=logging.INFO)
class MyThread(threading.Thread):
def __init__(self, func, args, name=""):
threading.Thread.__init__(self)
self.func = func
self.args = args
self.name = name
def run(self):
self.func(*self.args)
def loop(nloop, nsleep):
logging.info(f"{nloop} start at {time.asctime()}")
sleep(nsleep)
logging.info(f"{nloop} end at {time.asctime()}")
def main():
logging.info(f"start all at {time.asctime()}")
threads = []
loop_time = [2, 4]
nloop = range(len(loop_time))
for i in nloop:
t = MyThread(loop, (i, loop_time[i]), loop.__name__)
threads.append(t)
for i in nloop:
threads[i].start()
for i in nloop:
threads[i].join()
logging.info(f"end all at {time.asctime()}")
if __name__ == "__main__":
main()
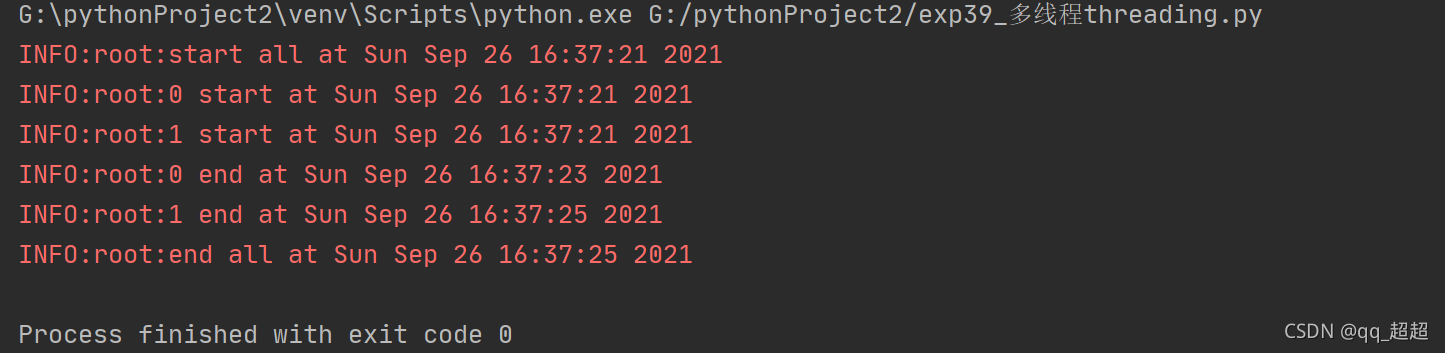
15.python对外部数据源文件处理
(1)YAML https://pyyaml.org/wiki/PyYAMLDocumentation
(2)EXCEL https://openpyxl.readthedocs.io/en/stable/usage.html#write-a-workbook
16.装饰器
(1)优点:在不修改原来业务逻辑的情况下对代码进行扩展,常用于权限校验、用户认证、日志记录、性能测试、事务处理、缓存等。 (2)结构:装饰器是一个带有函数作为参数并返回一个新函数的闭包。本质上也是函数。 (3)语法糖@
以打印场景为例,对比没有使用装饰器和使用装饰器时,对代码量的影响。 (1)不使用装饰器,打印每个函数的开始运行时间和结束运行时间。
import time
from time import sleep
def fun_1():
print(f"fun_1 start运行的时间{time.asctime()}")
sleep(2)
print(f"fun_1 end运行的时间{time.asctime()}")
def fun_2():
print(f"fun_2 start运行的时间{time.asctime()}")
sleep(2)
print(f"fun_2 end运行的时间{time.asctime()}")
def fun_3():
print(f"fun_3 start运行的时间{time.asctime()}")
sleep(2)
print(f"fun_3 end运行的时间{time.asctime()}")
def main():
fun_1()
fun_2()
fun_2()
if __name__ == "__main__":A
main()
(2)使用装饰器
import time
from time import sleep
def outer(func):
def inner():
print(f"{func.__name__} start运行的时间{time.asctime()}")
func()
print(f"{func.__name__} end运行的时间{time.asctime()}")
return inner
def fun_1():
sleep(2)
def fun_2():
sleep(2)
def fun_3():
sleep(2)
def main():
outer(fun_1)()
outer(fun_2)()
outer(fun_3)()
if __name__ == "__main__":
main()
(3)使用语法糖@
import time
from time import sleep
def outer(func):
def inner():
print(f"{func.__name__} start运行的时间{time.asctime()}")
func()
print(f"{func.__name__} end运行的时间{time.asctime()}")
return inner
@outer
def fun_1():
sleep(2)
@outer
def fun_2():
sleep(2)
@outer
def fun_3():
sleep(2)
def main():
fun_1()
fun_2()
fun_3()
if __name__ == "__main__":
main()
以上3种情况的运行结果都一样: 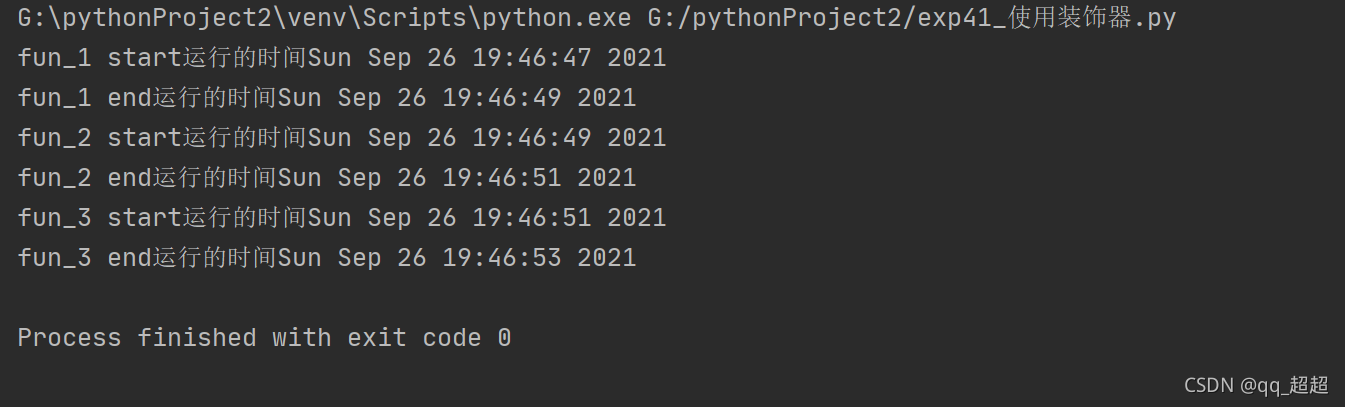
|