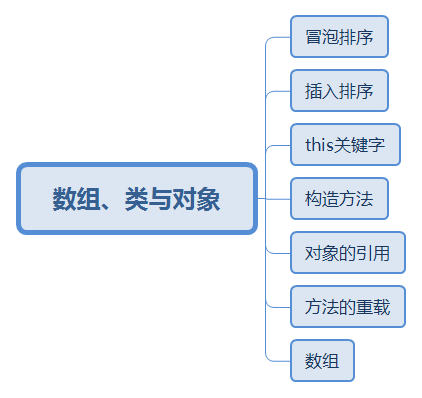
{
int[] str = new int[5];
for (int i = 0; i < str.Length; i++)
{
str[i] = i;
Console.WriteLine(i);
}
string[] st = new string[8];
bool[] bl = new bool[9];
int[] One = new int[6];
int[] Two = { 2, 5, 6, 8, 9 };
int[] Three = new int[] { 3, 5, 7, 6 };
int[] Four = new int[3] { 1, 2, 3 };
Console.WriteLine("------------------");
#region 从一个整数数组中取出最大、最小的整数,以及总和 平均值
#endregion
#region 练习2:计算一个整数数组的所有元素的和
#endregion
#region 练习3: 数组里面都是人的名字,分割成: 例如: 老杨 | 老苏 | 老邹…”
string[] str1 = { "老杨", "老苏", "老邹", "老虎", "老牛", "老王", "老马", "老蒋" };
string str2 = null;
for (int i = 0; i < str1.Length-1; i++)
{
str2 +=str1[i]+"|";
}
Console.WriteLine(str2+str1[str1.Length-1]);
#endregion
#region 练习4:将一个整数数组的每一个元素进行如下的处理:如果元素是正数则将这个位置的元素的值加1,如果元素是负数则将这个位置的元素的值减1,如果元素是0,则不变。
int[] Six = { -5, -6, -7, 0, 5, 3, 1 };
for (int i = 0; i < Six.Length; i++)
{
if (Six[i] > 0)
{
Six[i] += 1;
Console.WriteLine(Six[i]);
}
else if (Six[i] < 0)
{
Six[i] -= 1;
Console.WriteLine(Six[i]);
}
else
{
Console.WriteLine(Six[i]);
}
}
#endregion
#region 练习5:将一个字符串数组的元素的顺序进行反转。{“我”,“是”,”好人”}“好人”,”是”,”我”}。第i个和第length - i - 1个进行交换
string[] s = { "我", "是", "好人" };
for (int i = 0; i < s.Length/2; i++)
{
string temp = s[i];
s[i]= s[s.Length - 1 - i];
s[s.Length - 1 - i] = temp;
}
Console.WriteLine();
for (int i = 0; i <s.Length; i++)
{
Console.WriteLine(s[i]);
}
#endregion
}
}
二、冒泡排序
第一种:
class Program
{
static void Main(string[] args)
{
int[] array = { 5, 9, 4, 6, 3, -1, 0 };
MaoPaoSort(array);
foreach (var item in array)
{
Console.WriteLine(item);
}
}
private static void MaoPaoSort(int[] array)
{
for (int i = 0; i < array.Length - 1; i++)
{
bool isSort = true;
for (int j = 0; j < array.Length - 1; j++)
{
if (array[j] > array[j + 1])
{
int temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
isSort = false;
}
}
if (isSort)
{
break;
}
}
}
}
第二种:
class Program
{
static void Main(string[] args)
{
int[] array = { 2, 5, 9, 4, 6, 1, 3, 7, 8 };
MaoPaoSort(array);
foreach (var item in array)
{
Console.Write(item + ",");
}
Console.ReadKey();
}
private static void MaoPaoSort(int[] array)
{
for (int j = 0; j < array.Length - 1; j++)
{
bool isSort = true;
for (int i = 0; i < array.Length - 1; i++)
{
if (array[i] > array[i + 1])
{
int tamp = array[i];
array[i] = array[i + 1];
array[i + 1] = tamp;
isSort = false;
}
}
if (isSort)
{
break;
}
}
}
}
三、插入排序
class Program
{
static void Main(string[] args) {
int[] array = new int[] { 1, 5, 6, 8, 7, 3, 4 };
InsertSort(array);
foreach (var item in array)
{
Console.Write(item);
}
Console.ReadKey();
}
private static void InsertSort(int[] array)
{
for (int i = 1; i < array.Length; i++)
{
int x = array[i];
bool isInsert = false;
for (int j = i - 1; j >= 0; j--)
{
if (array[j] > x)
{
array[j + 1] = array[j];
}
else
{
array[j + 1] = x;
isInsert = true;
break;
}
}
if (!isInsert)
{
array[0] = x;
}
}
}
}
四、方法的重载
what:方法名相同,参数列表的位置 顺序 类型不同 how:
class Program
{
static void Main(string[] args)
{
}
public static void Test(int a) { }
public static void Test() { }
public static void Test(string a) { }
public static void Test(string a,string b) { }
}
五、练习题
class Program
{
static void Main(string[] args)
{
#region MyRegion
#endregion
#region 水仙花数
#endregion
#region 一个控制台应用程序,求1000之内的所有“完数”。所谓“完数”是指一个数恰好等于它的所有因子之和。例如6是完数,因为6=1+2+3。
#endregion
#region 一个控制台应用程序,要求完成写列功能。
#endregion
#region 要求用户输入5个大写字母,如果用户输入的信息不满足要求,提示帮助信息并要求重新输入。
#endregion
#region 输出1~5的平方值,要求:用for语句实现。用while语句实现。用do-while语句实现。
#endregion
#region 编写一个掷筛子100次的程序,并打印出各种点数的出现次数。
#endregion
#region 编程输出九九乘法表
#endregion
#region 编程输出1000以内的所有素数
#endregion
#region 编程输出1~100中能被3整除但不能被5整除的数,并统计有多少个这样的数。
#endregion
#region 编写一个控制台程序,分别输出1~100之间的平方、平方根。
#endregion
#region 求出1~1000之间的所有能被7整除的数,并计算和输出每5个的和。
#endregion
#region 让用户输入两个整数,然后再输入0-3之间的一个数,0代表加法,1代表减法,2代表乘法,3代表除法,计算这两个数字的结果
#endregion
#region 编写一个程序,对输入的4个整数,求出其中的最大值和最小值,并显示出来。
#endregion
Console.ReadKey();
}
private static bool isSXH(int a)
{
string s = a.ToString();
int sum = 0;
for (int i = 0; i < s.Length; i++)
{
int temp = int.Parse(s[i].ToString());
sum += temp * temp * temp;
}
return a == sum;
}
private static bool isWS(int a)
{
int sum = 0;
for (int i = 1; i < a; i++)
{
if (a % i == 0)
{
sum += i;
}
}
return a == sum;
}
private static bool isZS(int a)
{
for (int i = 2; i < a; i++)
{
if (a % i == 0)
{
return false;
}
}
return true;
}
}
|