捕获异常
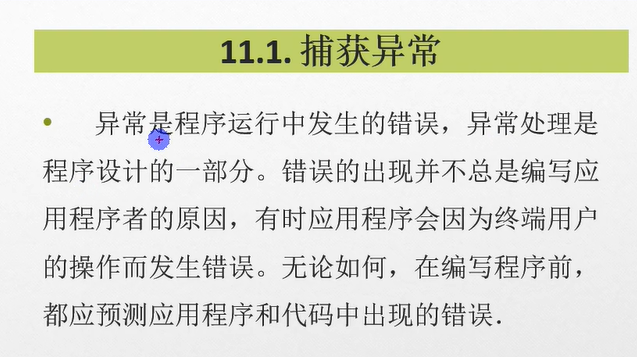 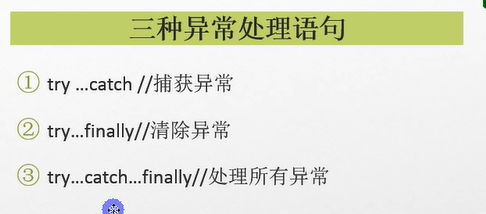 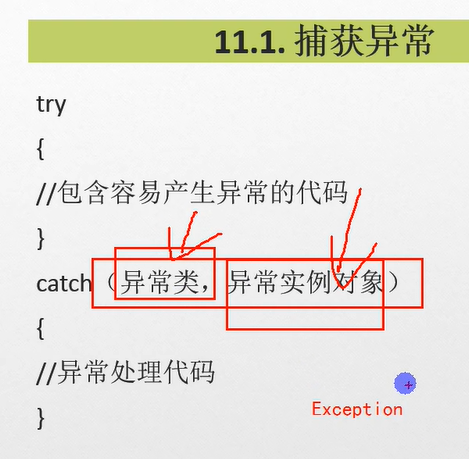 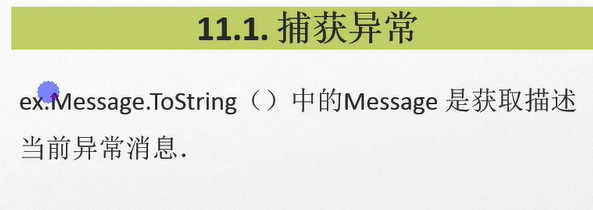
例子: 访问数组,防止越界
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _11._1捕获异常
{
class Program
{
static void Main(string[] args)
{
int[] myint = { 0, 2, 4, 6, 8, 10, 12, 16, 18, 20 };
try
{
for(int i=0;i<=myint.Length;i++)
{
Console.Write(myint[i].ToString() + " ");
}
}
catch(Exception myex)
{
Console.WriteLine(myex.Message.ToString());
}
Console.ReadKey();
}
}
}
结果:
0 2 4 6 8 10 12 16 18 20 索引超出了数组界限。
清除、处理所有异常
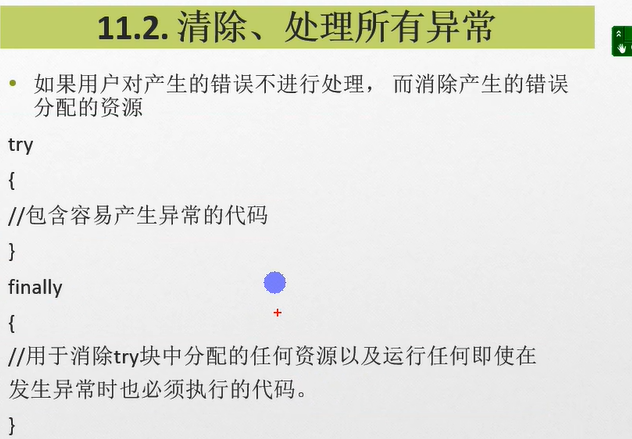 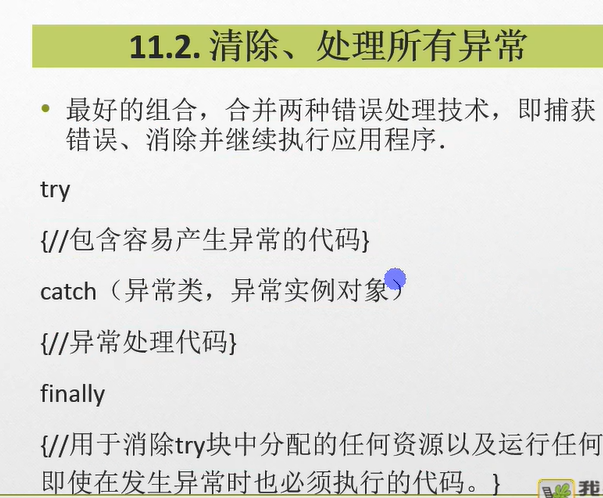 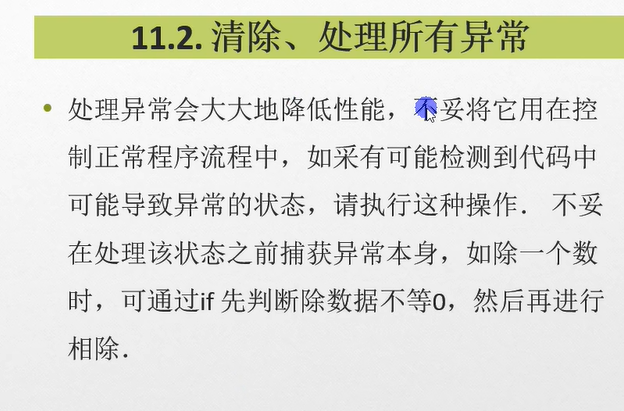
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _11._2清除捕获异常
{
class Program
{
static void Main(string[] args)
{
int[] myint = { 0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20 };
try
{
for (int i = 0; i < myint.Length; i++)
Console.Write("720除以{0}的结果是{1}", myint[i], 720 / myint[i]);
}
catch(Exception myex)
{
Console.WriteLine(myex.Message.ToString());
}
finally
{
Console.WriteLine("我什么时候都会执行,无论发生异常与否");
}
Console.ReadKey();
}
}
}
结果:
尝试除以零。
我什么时候都会执行,无论发生异常与否
引发异常
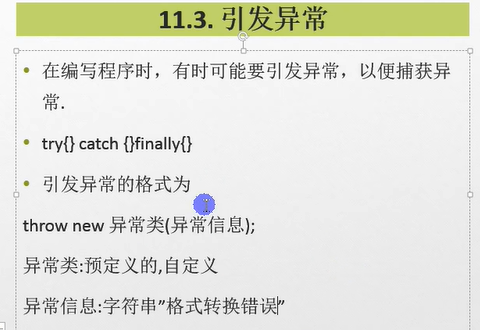 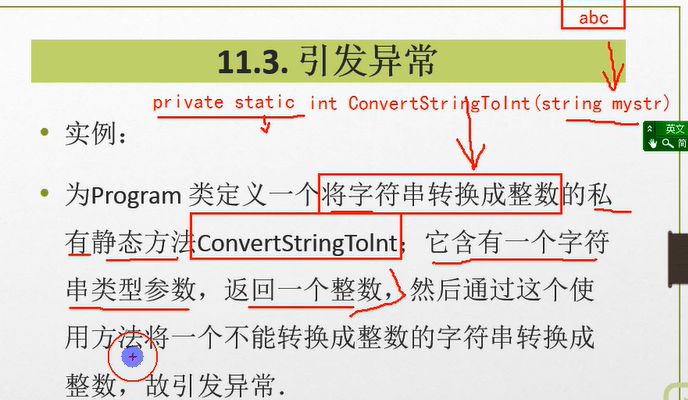
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _11._3引发异常
{
class Program
{
private static int ConvertStringToInt(string mystr)
{
int outnum = 0;
try
{
outnum = Convert.ToInt32(mystr);
return outnum;
}
catch
{
throw new FormatException("格式转换不正确(由我要自学网制作)");
}
}
static void Main(string[] args)
{
string mystr = "51zxw";
try
{
int myint;
myint = Program.ConvertStringToInt(mystr);
Console.WriteLine(myint);
}
catch(FormatException exf)
{
Console.WriteLine(exf.Message.ToString());
}
Console.ReadKey();
}
}
}
结果:
格式转换不正确(由我要自学网制作)
预定义异常类
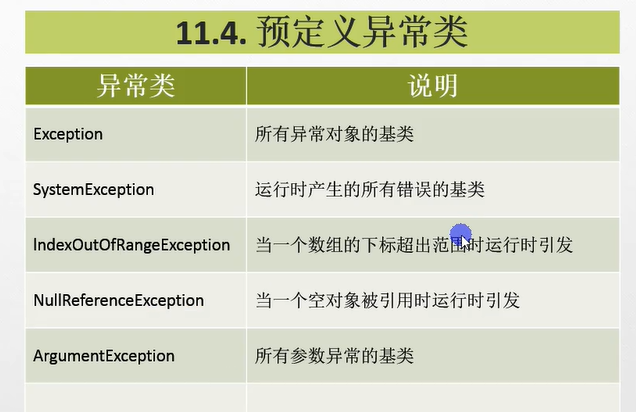 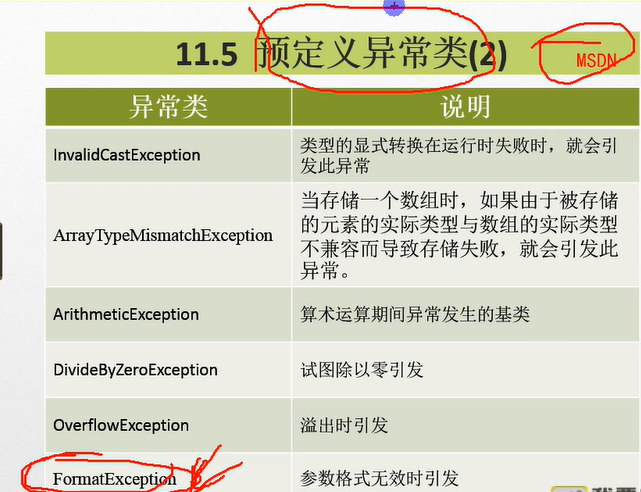
自定义异常类
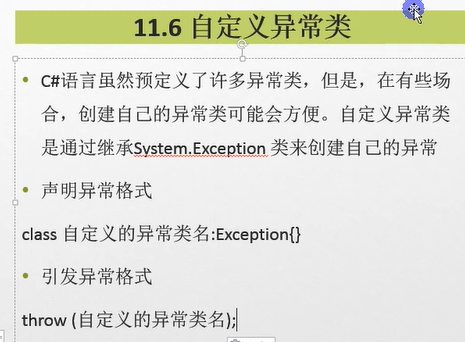 自定义的异常类
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _11._6自定义异常类
{
class MyException:Exception
{
public MyException(string message):base(message)
{
}
}
}
主程序调用
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _11._6自定义异常类
{
class Program
{
static void Main(string[] args)
{
try
{
Console.WriteLine("这行代码在引发异常前被执行");
string mystr = "这是我自己定义的异常,由我要自学网制作";
throw new MyException(mystr);
Console.WriteLine("由于引发了异常,这行代码不会被执行");
}
catch(MyException ex)
{
Console.WriteLine(ex.Message.ToString());
}
Console.ReadKey();
}
}
}
结果:
这行代码在引发异常前被执行
这是我自己定义的异常,由我要自学网制作
本章小结及任务实施
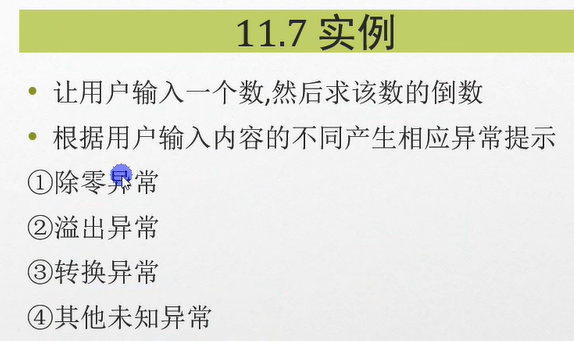
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _11._7本章小结及任务实施
{
class Program
{
static void Main(string[] args)
{
Console.Write("请输入一个整数:");
try
{
int myint = int.Parse(Console.ReadLine());
double mydouble = 1.0 / myint;
Console.WriteLine("该数的倒数是:" + mydouble);
}
catch(DivideByZeroException)
{
Console.WriteLine("产生除零异常");
}
catch(OverflowException)
{
Console.WriteLine("溢出异常");
}
catch(FormatException)
{
Console.WriteLine("转换异常");
}
catch(Exception)
{
Console.WriteLine("其他异常");
}
Console.ReadKey();
}
}
}
请输入一个整数:589455125696552
溢出异常
|