1.常用快捷键
2.IDEA连接数据库
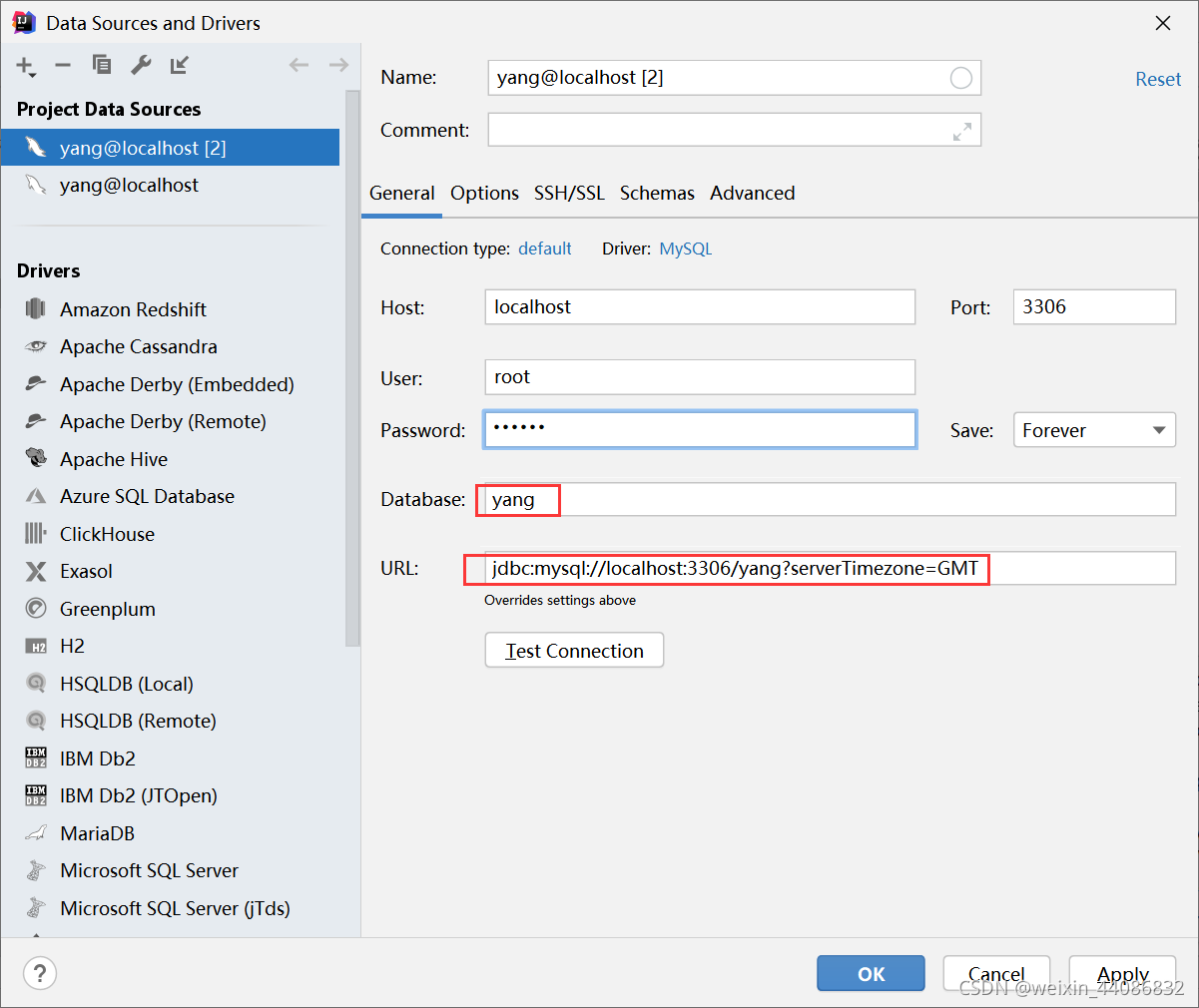
jdbc:mysql://localhost:3306/yang?serverTimezone=GMT
3. Free-MyBatis-plugin
在 **Dao.java 上写新的方法后,Ctrl + 点击可以直接跳转到 **Dao.xml 中,并生成对应的代码
4.EasyCode
下载相应的插件,然后在对应的表上 右键 -> Easy Code -> Generate Code, 可直接生成 entity,dao, service和controller层对应代码
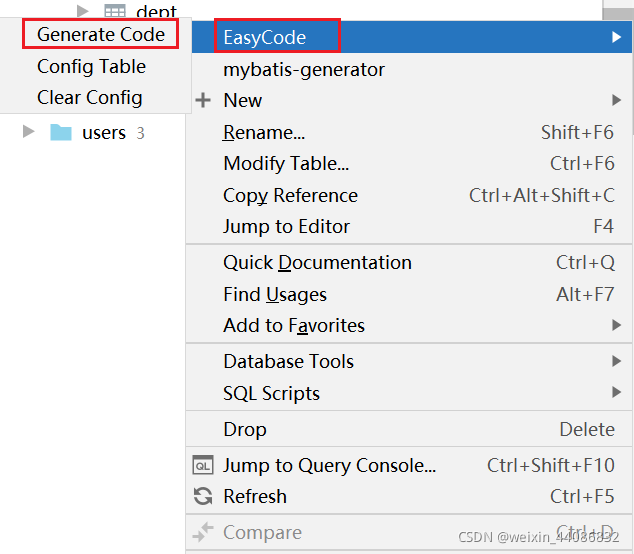
5. 自动生成数据库表对应的实体类以及配置文件
首先需要已经连接数据库, 然后在对应的表上 右键 -> mybatis-generator, 选择对应实体类、Dao.java 和 Dao.xml 的路径 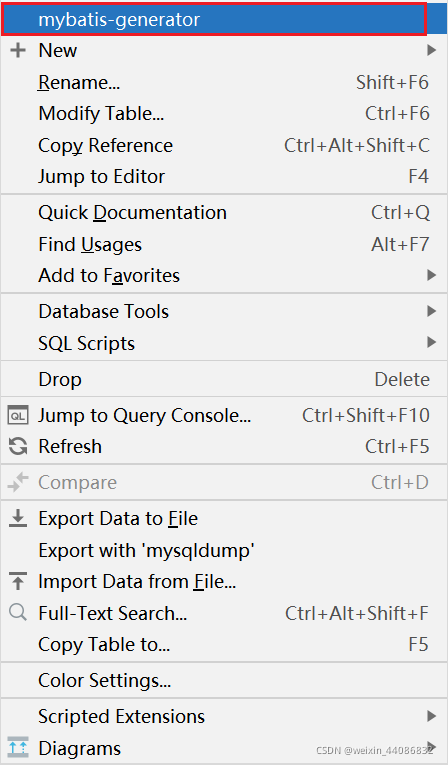 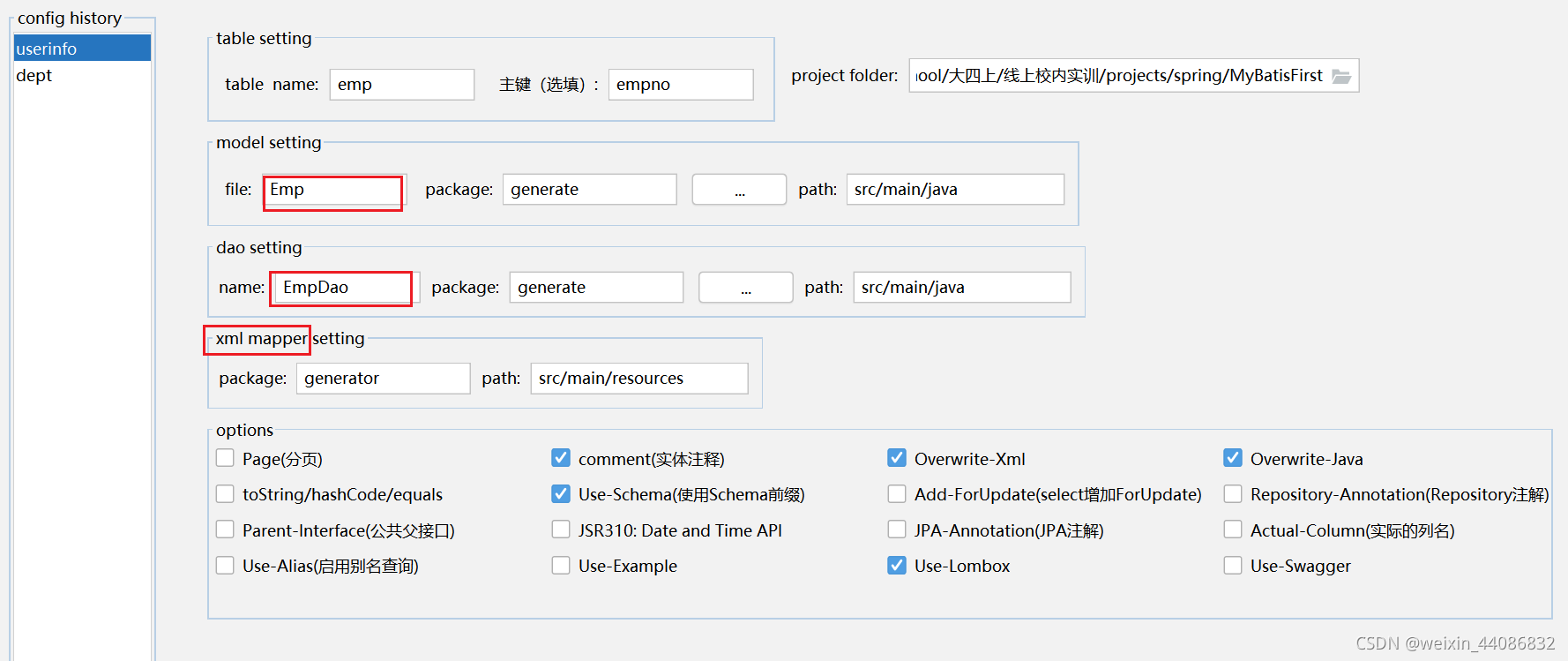
6. 导入lombok jar包,在实体类使用@Data,从而取消get,set,toString方法
pom.xml
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.18</version>
</dependency>
实体类
@Data
public class Dept implements Serializable {
private Integer deptno;
private String dname;
private String loc;
private static final long serialVersionUID = 1L;
}
7. 自动生成测试类
光标移动到 **Dao.java 类名上, 然后 右键 -> generater -> Test
选择需要进行测试的方法
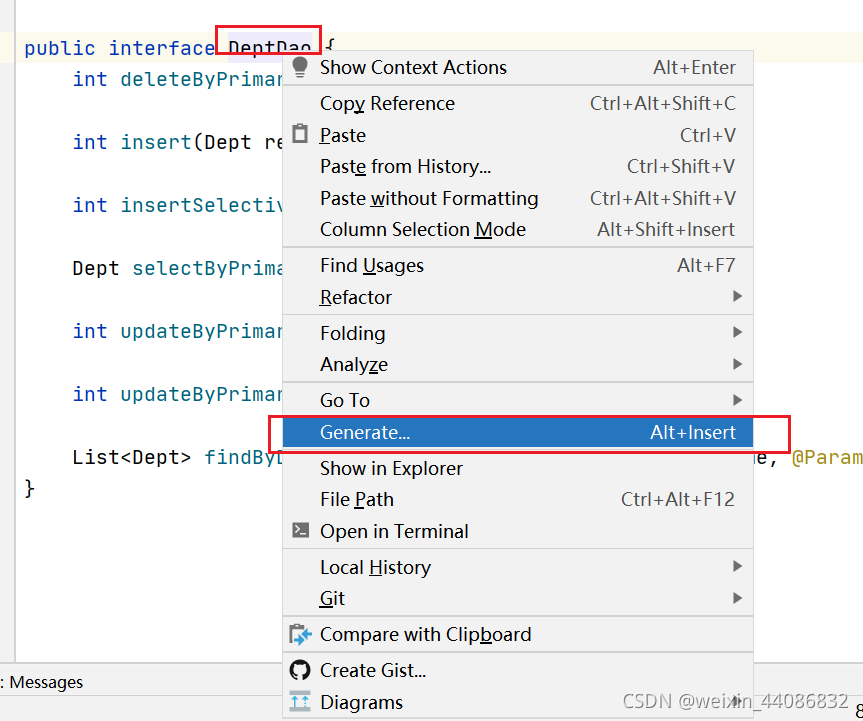 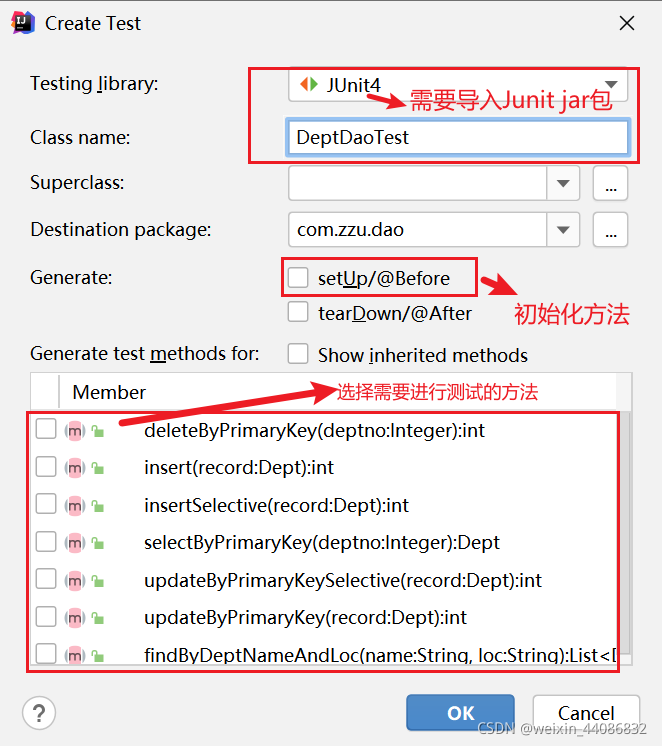
8. 多个条件查询
- 使用注解的方式(推荐使用)
**Dao.java
List<Dept> findByDeptNameAndLoc(@Param("name") String name, @Param("loc") String loc);
****Dao.xml
<select id="findByDeptNameAndLoc" resultType="com.zzu.entity.Dept">
select * from dept where dname=#{name} and loc=#{loc}
</select>
-
使用内置变量 param1,param2的方式 -
使用内置变量 arg0, arg1的方式 -
使用Map封装变量
9. resultType与resultMap
<resultMap id="BaseResultMap" type="com.zzu.entity.Dept">
<id column="deptno" jdbcType="INTEGER" property="deptno" />
<result column="dname" jdbcType="VARCHAR" property="dname" />
<result column="loc" jdbcType="VARCHAR" property="loc" />
</resultMap>
10. 一对一,一对多
1. 一对一,assocation
<resultMap id="EmpDeptMap" type="com.zzu.entity.Emp" autoMapping="true">
<id property="empno" column="empno"/>
<result property="deptno" column="deptno"/>
<association property="dept" javaType="com.zzu.entity.Dept" column="deptno" autoMapping="true">
<id column="deptno" property="deptno"/>
<result column="dname" property="dname"/>
<result column="loc" property="loc"/>
</association>
</resultMap>
<select id="findAllEmp" resultMap="EmpDeptMap">
select e.*, d.*
from emp e left join dept d on e.deptno = d.deptno
</select>
<select id="findAllEmp2" resultMap="EmpDeptMap">
select e.*, d.*
from emp e, dept d
where e.deptno = d.deptno
</select>
2. 一对多,collection
<resultMap id="deptEmpMap" type="com.zzu.entity.Dept" autoMapping="true">
<id property="deptno" column="deptno"></id>
<collection property="emps" ofType="com.zzu.entity.Emp" autoMapping="true">
<id property="empno" column="empno"/>
</collection>
</resultMap>
<select id="findAllDeptEmp" resultMap="deptEmpMap">
select d.*, e.*
from dept d inner join emp e on d.deptno = e.deptno
</select>
11. 动态查询
1. <if>
<select id="findByDeptNameAndLoc" resultMap="BaseResultMap">
select *
from dept
where 1=1
<if test="name!='' and name!=null">
and dname=#{name}
</if>
<if test="loc!='' and loc!=null">
and loc=#{loc}
</if>
</select>
2. <when> <otherwise>
对应java中的 switch
3. <where>
12. 批量操作
for each 批量操作
**Dao.java
int addDeptBatch(@Param("depts") List<Dept> depts);
int deleteDeptBatch(@Param("ids") List<Integer> ids);
**Dao.xml
<delete id="deleteDeptBatch">
delete from dept where deptno in
<foreach collection="ids" separator="," open="(" close=")" item="id">
#{id}
</foreach>
</delete>
<insert id="addDeptBatch" keyProperty="deptno" useGeneratedKeys="true">
insert into dept(dname, loc) values
<foreach collection="depts" item="dept" separator=",">
(#{dept.dname}, #{dept.loc})
</foreach>
</insert>
对应查询语句: 

|