C++运算符重载
前言
- 什么是运算符重载
- 运算符重载的实质是什么
- 运算符重载的实质本身就是函数调用
- 运算符重载函数的写法
函数返回值 函数名(函数参数) 函数返回值:运算符运算完成后的值决定的 Complex 函数名 :operator加上重载运算符组成函数名 operator+ 参数 :看运算符的操作数,具体参数个数是要看你重载函数形式是什么 函数体 :学运算符具体想要的操作
提示:以下是本篇文章正文内容,下面案例可供参考
一、友元函数重载运算符
#include <iostream>
using namespace std;
class Complex
{
public:
Complex(int a=0, int b=0) :a(a), b(b)
{
}
void print()
{
cout << a << endl;
cout << b << endl;
}
friend Complex operator+ (Complex one, Complex two);
protected:
int a;
int b;
};
Complex operator+ (Complex one, Complex two)
{
return Complex(one.a + two.a, one.b + two.b);
}
int main()
{
Complex one(1, 1);
Complex two(2, 0);
Complex three;
three = one + two;
three.print();
Complex result;
result = operator+(one, two);
while (1);
return 0;
}
运算结果: 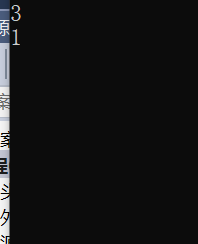
二、类成员函数重载运算符
#include <iostream>
using namespace std;
class Complex
{
public:
Complex(int a=0, int b=0) :a(a), b(b){}
void print()
{
cout << a << endl;
cout << b << endl;
}
friend Complex operator+ (Complex one, Complex two);
bool operator> (Complex object)
{
if (this->a > object.a)
{
return true;
}
else if (this->a == object.a&&this->b > object.b)
{
return true;
}
else
return false;
}
protected:
int a;
int b;
};
Complex operator+ (Complex one, Complex two)
{
return Complex(one.a + two.a, one.b + two.b);
}
int main()
{
Complex one(1, 1);
Complex two(2, 0);
Complex three;
three = one + two;
three.print();
Complex result;
result = operator+(one, two);
if (one > two)
{
cout << "one比较大" << endl;
}
if (one.operator> (two))
{
cout << "one比较大" << endl;
}
while (1);
return 0;
}
运算结果: 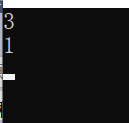
三、特殊运算符重载
1.流运算符重载
- cin类型:istream类的对象
- cout类型:ostream类的对象
- 流运算符>> <<
- 必须采用友元函数的形式重载
#include <iostream>
#include <string>
using namespace std;
class STU
{
public:
STU(string name="", int age=18) :name(name), age(age){}
friend istream& operator>>(istream& in, STU& lisi);
friend ostream& operator<<(ostream& out, STU& lisi);
protected:
string name;
int age;
};
istream& operator>>(istream& in, STU& lisi)
{
in >> lisi.name >> lisi.age;
return in;
}
ostream& operator<<(ostream& out, STU& lisi)
{
out << lisi.name << "\t"<<lisi.age << endl;
return out;
}
int main()
{
string str;
cin >> str;
cout << str;
STU lisi;
cin >> lisi;
cout << lisi;
cin >> str >> lisi;
cout << str << endl << lisi;
while (1);
return 0;
}
运算结果: 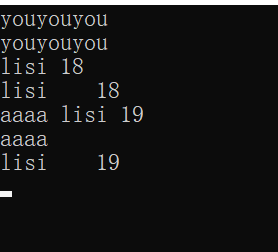
2.++ --运算符重载
- 解决问题:前置和后置的问题:
- 增加无用参数 int去表示当前运算符重载是后置操作
3.文本重载
4.其他运算符
- =()-》[]只能采用类的成员函数形式重载
- 流重载采用友元方式
- . .* ?: :: 不能被重载
#include <iostream>
#include <string>
using namespace std;
class STU
{
public:
STU(string name, int age) :name(name), age(age){}
friend ostream& operator<<(ostream& out, STU& object)
{
out << object.name << "\t" <<object.age<< endl;
return out;
}
STU operator++(int)
{
return STU(name, age++);
}
STU operator++()
{
return STU(name, ++age);
}
protected:
string name;
int age;
};
int main()
{
STU lisi("李四", 18);
cout << lisi << endl;
int num = 1;
int result = num++;
cout << result << "\t" << num << endl;
result = ++num;
cout << result << "\t"<<num << endl;
STU object = lisi++;
cout << object;
object = ++lisi;
cout << object<<lisi;
while (1);
return 0;
}
运行结果: 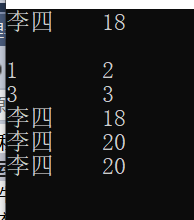
四、对象隐式转换
#include <iostream>
using namespace std;
class STU
{
public:
operator int()
{
return age;
}
STU(string name, int age):name(name), age(age){}
protected:
string name;
int age;
};
int main()
{
STU lisi("李四", 18);
int lisiage = lisi;
cout << lisiage << endl;
while (1);
return 0;
}
运行结果:
 在这里插入图片描述](https://img-blog.csdnimg.cn/4fc44c73b0ef4c9dafef44a71f970ff3.png)
五、重载综合案例
#include <iostream>
#include <string>
#include <functional>
#include <memory>
using namespace std;
class Int
{
public:
Int(int num) :num(num) {}
int& data()
{
return num;
}
string tostr()
{
return to_string(num);
}
Int operator+(const Int& value)
{
return Int(this->num + value.num);
}
friend Int operator-(const Int& one, const Int& two)
{
return Int(one.num - two.num);
}
Int operator+=(const int& value)
{
return Int(this->num + value);
}
Int operator+=(const Int& value)
{
return Int(this->num + value.num);
}
Int operator++(int)
{
return Int(this->num++);
}
Int operator++()
{
return Int(++this->num);
}
Int operator&(const Int& value)
{
return Int(this->num & value.num);
}
bool operator!()
{
return !this->num;
}
Int operator-()
{
return Int(-this->num);
}
friend ostream& operator<<(ostream& out, const Int& object)
{
out << object.num << endl;
return out;
}
friend istream& operator>>(istream& in, Int& object)
{
in >> object.num;
return in;
}
int* operator&()
{
return &this->num;
}
bool operator>(const Int& object)
{
return this->num > object.num;
}
protected:
int num;
};
void print(const int& num)
{
cout << num << endl;
}
class myvector
{
public:
myvector(int size)
{
base = new int[size] {0};
}
int& operator[](int index)
{
return base[index];
}
protected:
int *base;
};
class Function
{
typedef void(*PF)();
public:
Function(PF pf) :pf(pf) {}
void operator()()
{
pf();
}
protected:
PF pf;
};
struct MM
{
string name;
int age;
MM(string name, int age) :name(name), age(age) {}
};
class Auto_ptr
{
public:
Auto_ptr(int* ptr) :ptr(ptr) {}
Auto_ptr(MM* ptr) :ptrMM(ptr) {}
int& operator*()
{
return *ptr;
}
MM* operator->()
{
return ptrMM;
}
~Auto_ptr()
{
if (ptrMM)
{
delete ptr;
ptr = nullptr;
}
if (ptrMM)
{
delete ptrMM;
ptrMM = nullptr;
}
}
protected:
int* ptr;
MM* ptrMM;
};
void testAuto_ptr()
{
Auto_ptr ptr(new int(18));
cout << *ptr << endl;
Auto_ptr ptrMM(new MM("mm", 19));
cout << ptrMM->name<< endl;
cout << ptrMM->age << endl;
auto_ptr<int> autoPtr(new int(18));
cout << *autoPtr << endl;
auto_ptr<MM> autoPtrMM(new MM("mm", 19));
cout << autoPtrMM->name << endl;
cout << autoPtrMM->age << endl;
}
void print()
{
cout << "测试函数" << endl;
}
int max(int a, int b)
{
return a + b;
}
int main()
{
print(1);
int a = 1;
print(a);
Int num(10);
cout << num;
cout << -num;
myvector vec(5);
#if 0
for (int i = 0; i < 5; i++)
{
cin >> vec[i];
}
for (int i = 0; i < 5; i++)
{
cout << vec[i];
}
#endif
Function p(print);
p();
testAuto_ptr();
while (1);
return 0;
}
运行结果: 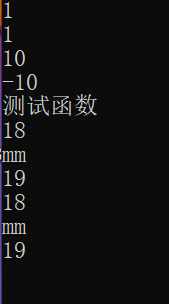
|