1. 打开程序
对于应用程序而言,可以通过ui界面打开程序,也可以通过命令打开程序。在windows下打开一个应用程序只要输入程序的完成路径即可。在window下打开程序的方式有两种:
2. 在终端打开程序和使用python打开程序
在终端打开程序
cd path
example.exe
在python中运行指定的程序
import subprocess
process = subprocess.Popen([r'D:\software\example.exe'])
3. 在终端查找指定名称程序的pid
对于每个正在运行的程序都有一个pid与之对应,可以通过cmd终端和python两种方式查看
在终端查看
for /f "tokens=2 " %a in ('tasklist /fi "imagename eq example.exe" /nh') do echo %a
在python中查看
import subprocess
process = subprocess.run('tasklist /fi "imagename eq example.exe" /nh', stdout=subprocess.PIPE, encoding='gbk')
print("查询到程序的pid为:", process.stdout)
4. 杀死指定名称的程序
在终端
for /f "tokens=2 " %a in ('tasklist /fi "imagename eq example.exe" /nh') do taskkill /f /pid %a
在python中
import subprocess
process = subprocess.run('tasklist /fi "imagename eq example.exe" /nh', stdout=subprocess.PIPE, encoding='gbk')
print("查询到程序的pid为:", process.stdout)
result = re.findall(r'(\d+) Console', process.stdout, re.DOTALL)
print(result)
if result:
for pid in result:
output = subprocess.run('taskkill /f /pid %s' % pid, stdout=subprocess.PIPE, encoding='gbk')
print(output.stdout)
应用
检测指定程序是否运行,如果运行则结束应用程序——炉石传说整活插件!!! 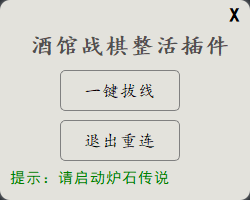 主程序源代码,github项目地址https://gitee.com/sowsophay/battleground.git:
"""
author: sophay
date: 2021/8/30
"""
import ctypes
import sys
import subprocess
from PyQt5.QtCore import Qt, QTimer
from PyQt5.QtGui import QIcon
from PyQt5.QtWidgets import QSystemTrayIcon, QWidget, QApplication, QAction, QMenu, QGraphicsDropShadowEffect
from interface_ui import Ui_Form
import img_rc
class BWidget(QWidget):
def __init__(self, *args, **kwargs):
super(BWidget, self).__init__(*args, **kwargs)
self.setWindowFlags(Qt.FramelessWindowHint | Qt.WindowStaysOnTopHint | Qt.SplashScreen)
self._move_flag = False
self._mouse_x, self._mouse_y, self._origin_x, self._origin_y = None, None, None, None
def mousePressEvent(self, evt):
if evt.button() == Qt.LeftButton:
self._move_flag = True
self._mouse_x, self._mouse_y, self._origin_x, self._origin_y = \
evt.globalX(), evt.globalY(), self.x(), self.y()
def mouseMoveEvent(self, evt):
if self._move_flag:
self.move(self._origin_x + evt.globalX() - self._mouse_x, self._origin_y + evt.globalY() - self._mouse_y)
def mouseReleaseEvent(self, evt):
self._move_flag = False
class MainPanel(BWidget, Ui_Form):
def __init__(self):
super().__init__()
self.setupUi(self)
self.setAttribute(Qt.WA_TranslucentBackground, True)
self.states = {'battle.net': "", 'Hearthstone': ""}
self.init_ui()
qss = """
#radius_widget {
background-color: rgb(227, 226, 220);
border-radius: 10px;
}
#close_btn {
color: black;
outline: none;
}
#close_btn:hover {
color: darkred;
}
#notice_lb {
color: green;
}
#title {
color: #524f4f;
}
#reconnect_btn, #restart_btn {
border: 1px solid gray;
border-radius: 5px;
}
#reconnect_btn:hover, #restart_btn:hover {
border: 1px solid #2f3433;
}
#reconnect_btn:disabled, #restart_btn:disabled {
border: none;
}
"""
self.setStyleSheet(qss)
effect = QGraphicsDropShadowEffect(self)
effect.setBlurRadius(12)
effect.setOffset(0, 0)
effect.setColor(Qt.gray)
self.setGraphicsEffect(effect)
self.system_icon = QIcon(':/logo.ico')
self.tray_icon = QSystemTrayIcon(self)
self.create_tray_icon()
def init_ui(self):
self.reconnect_btn.clicked.connect(self.reconnect_slot)
self.restart_btn.clicked.connect(self.restart_slot)
self.close_btn.clicked.connect(QApplication.instance().quit)
def reconnect_slot(self):
self.query_states()
if all(self.states.values()):
cmd_cut = 'netsh advfirewall firewall add rule name="酒馆战棋" dir=out program="%s" action=block' % \
self.states['Hearthstone']
cmd_connect = 'netsh advfirewall firewall delete rule name="酒馆战棋"'
print("断线中")
self.log("断线中")
subprocess.run(cmd_cut, shell=True, stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
self.reconnect_btn.setEnabled(False)
def connect():
subprocess.run(cmd_connect, shell=True, stdin=subprocess.PIPE, stdout=subprocess.PIPE,
stderr=subprocess.PIPE)
print("重连成功!")
self.log("重连成功!")
self.reconnect_btn.setEnabled(True)
QTimer.singleShot(3000, connect)
def restart_slot(self):
self.query_states()
if all(self.states.values()):
p_kill = subprocess.run(
'taskkill /F /IM "Hearthstone.exe" /T', shell=True, stdin=subprocess.PIPE, stdout=subprocess.PIPE,
stderr=subprocess.PIPE, encoding='gbk')
print("终止程序!", p_kill.stdout)
self.log("终止炉石传说成功")
self.restart_btn.setEnabled(False)
def restart():
print("重启")
self.log("正在重启炉石传说")
cmd = r'%s Launcher.exe --exec="launch WTCG"' % self.states['battle.net'][:-4]
print(cmd)
subprocess.run(cmd, shell=True, stdin=subprocess.PIPE, stdout=subprocess.PIPE,
stderr=subprocess.PIPE, encoding='gbk')
self.restart_btn.setEnabled(True)
self.log("重启成功")
QTimer.singleShot(5000, restart)
def query_states(self):
translate = {'battle.net': '战网', 'Hearthstone': '炉石传说'}
for name in self.states.keys():
get_p_path = subprocess.run(
r'wmic process where name="%s.exe" get executablepath' % name, shell=True, stdin=subprocess.PIPE,
stdout=subprocess.PIPE, stderr=subprocess.PIPE, encoding='gbk')
path = get_p_path.stdout.split('\n')
if path:
if "ExecutablePath" in path[0]:
path = path[2].strip(' ')
print("查询到%s运行路径为:" % translate[name], path)
self.states[name] = path
if not self.states[name]:
print("请启动%s" % translate[name])
self.log("请启动%s" % translate[name])
print(self.states)
def log(self, msg):
self.notice_lb.setText("提示:%s" % msg)
self.notice_lb.adjustSize()
def create_tray_icon(self):
restore_menu = QAction('恢复(&R)', self, triggered=self.showNormal)
quit_menu = QAction('退出(&Q)', self, triggered=QApplication.instance().quit)
menu = QMenu(self)
menu.addAction(restore_menu)
menu.addAction(quit_menu)
self.tray_icon.setIcon(self.system_icon)
self.tray_icon.setContextMenu(menu)
self.tray_icon.show()
if __name__ == '__main__':
debug = False
def is_admin():
try:
return ctypes.windll.shell32.IsUserAnAdmin()
except (PermissionError, ValueError):
return False
if debug:
app = QApplication(sys.argv)
win = MainPanel()
win.show()
sys.exit(app.exec_())
else:
if is_admin():
app = QApplication(sys.argv)
win = MainPanel()
win.show()
sys.exit(app.exec_())
else:
if sys.version_info[0] == 3:
ctypes.windll.shell32.ShellExecuteW(None, "runas", sys.executable, __file__, None, 1)
|