1.统计当前目录下每个文件类型的文件数
import os
all_file=os.listdir(os.curdir)
type_dict=dict()
for each_file in all_file:
if os.path.isdir(each_file):
type_dict.setdefault("文件夹",0)
type_dict["文件夹"]+=1
else:
type_name=os.path.splitext(each_file)[1]
type_dict.setdefault(type_name,0)
type_dict[type_name]+=1
for each_type in type_dict.keys():
print("该文件夹下文件类型为[%s]的文件有 %d个"%(each_type,type_dict[each_type]))
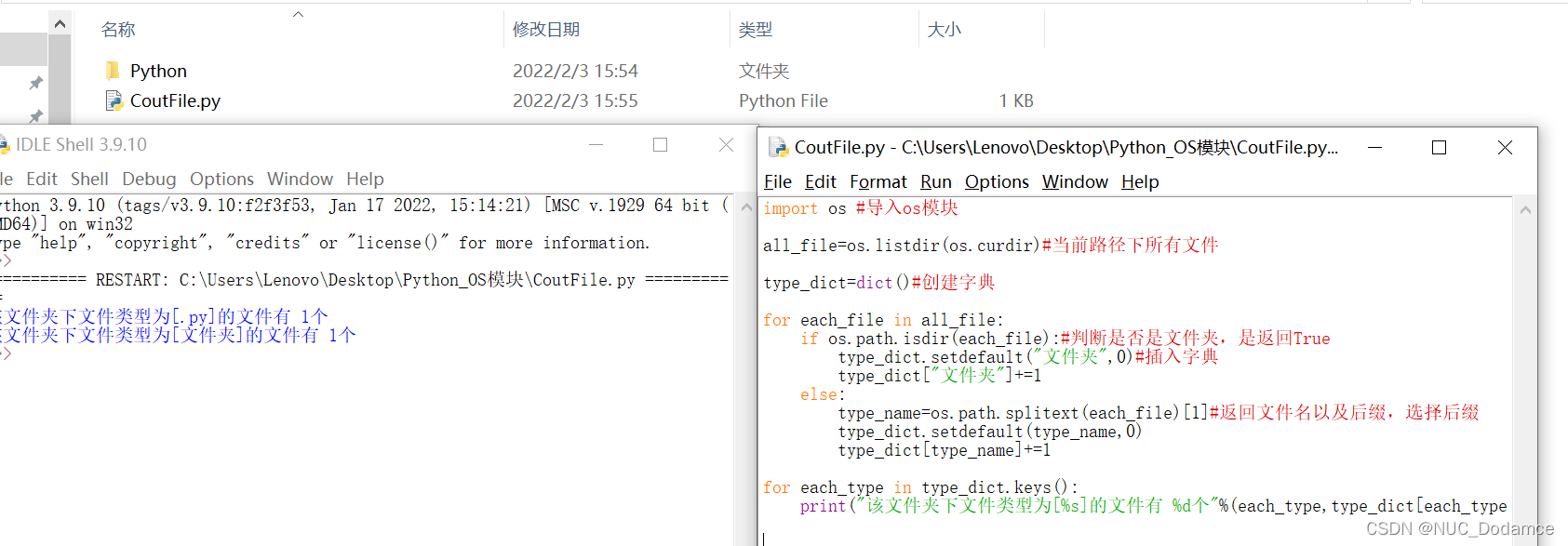
2.计算当前文件夹下所有文件的大小
import os
all_file=os.listdir(os.curdir)
file_dict=dict()
for each_file in all_file:
if os.path.isfile(each_file):
file_size=os.path.getsize(each_file)
file_dict[each_file]=file_size
for each in file_dict.items():
print("%s[%d Bytes]"%(each[0],each[1]))
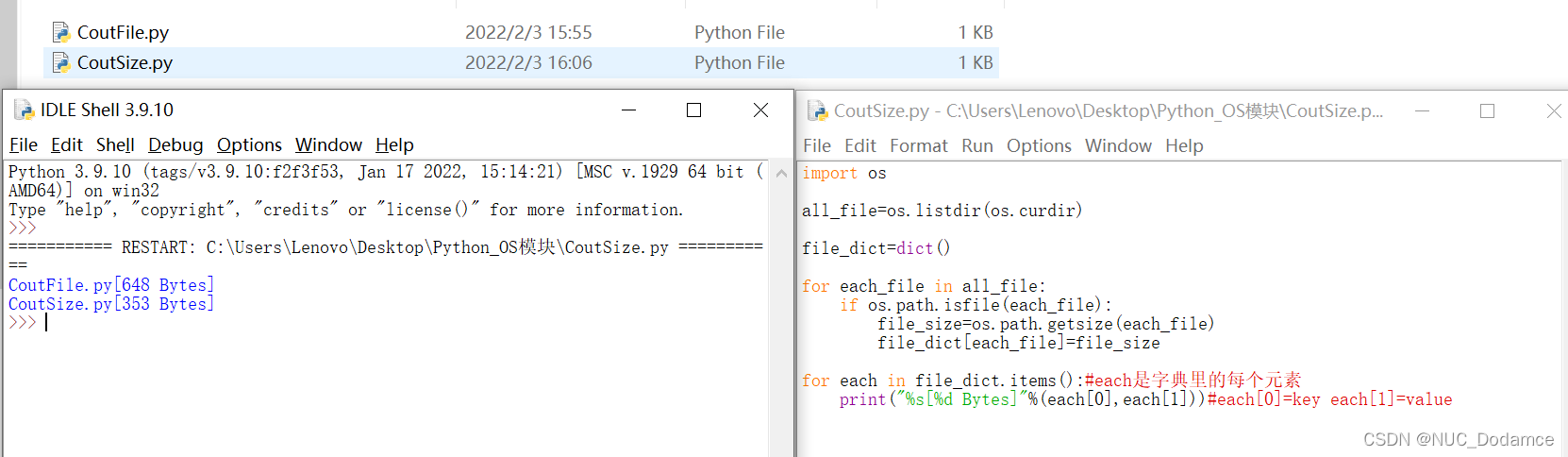
3. 用户输入文件名以及开始搜索的路径,搜索该文件是否存在。如遇到文件夹,则进入文件夹继续搜索
import os
def FindFile(Findir,target):
os.chdir(Findir)
for each_file in os.listdir(os.curdir):
if each_file ==target:
print(os.getcwd()+os.sep+each_file)
elif os.path.isdir(each_file):
FindFile(each_file,target)
os.chdir(os.pardir)
Findir=input("请输入查找目录:")
target=input("请输入要查找的目标文件:")
FindFile(Findir,target)
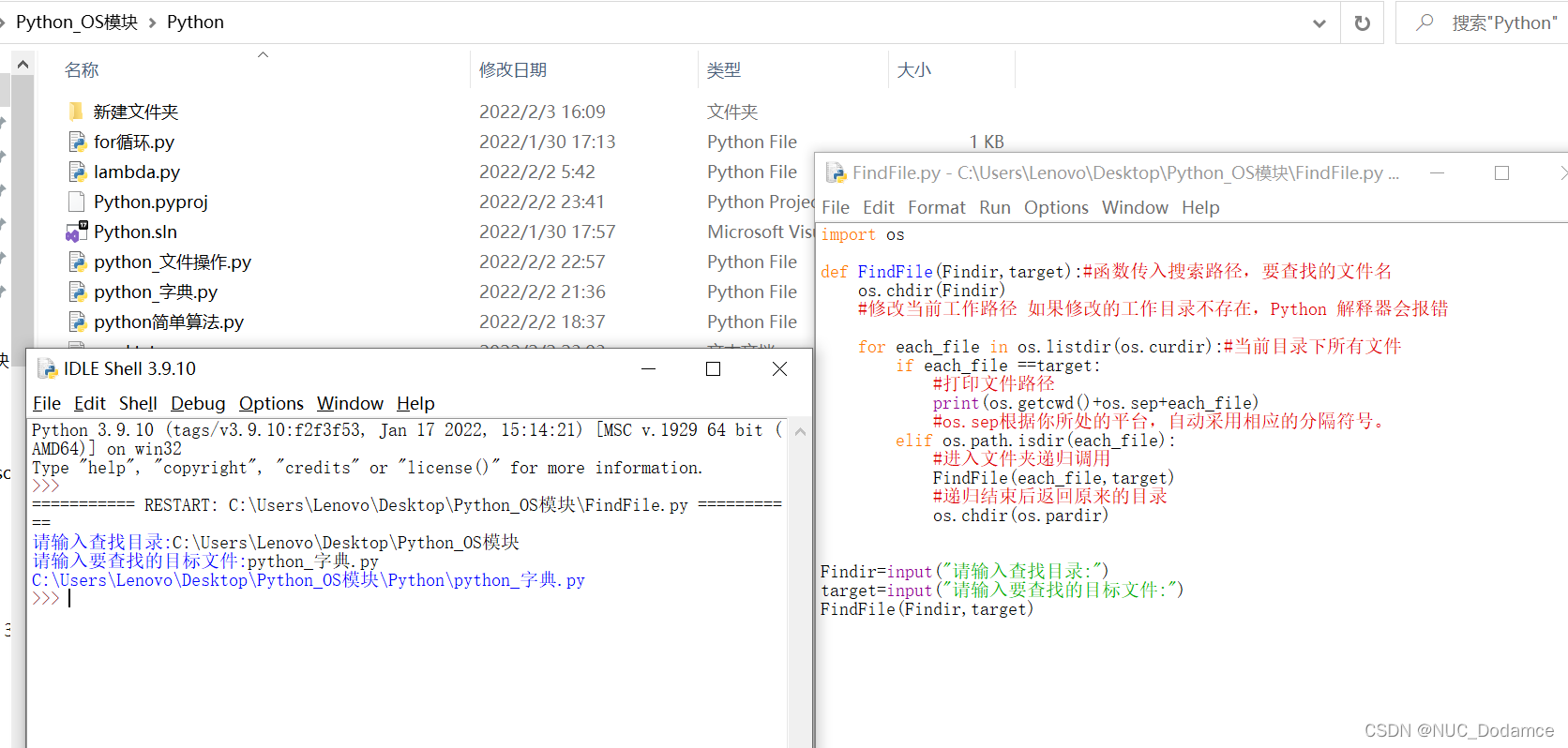
4.用户输入开始搜索的路径,查找该路径下(包含子文件夹内)所有的Python文件以及普通文档(后缀是.py/.txt),并把创建一个文件(py.txt)存放所有找到的文件的路径
import os
FileList=[]
def FindFile(StartDir,target):
os.chdir(StartDir)
for eachFile in os.listdir(os.curdir):
Type=os.path.splitext(eachFile)[1]
if Type in target:
FileList.append(os.getcwd()+os.sep+eachFile+os.linesep)
elif os.path.isdir(eachFile):
FindFile(eachFile,target)
os.chdir(os.pardir)
StartDir=input("输入要查找的起始路径:")
SaveDir=os.getcwd()
target=[".py",".txt"]
FindFile(StartDir,target)
File=open(SaveDir+os.sep+"py.txt",'w')
File.writelines(FileList)
File.close()
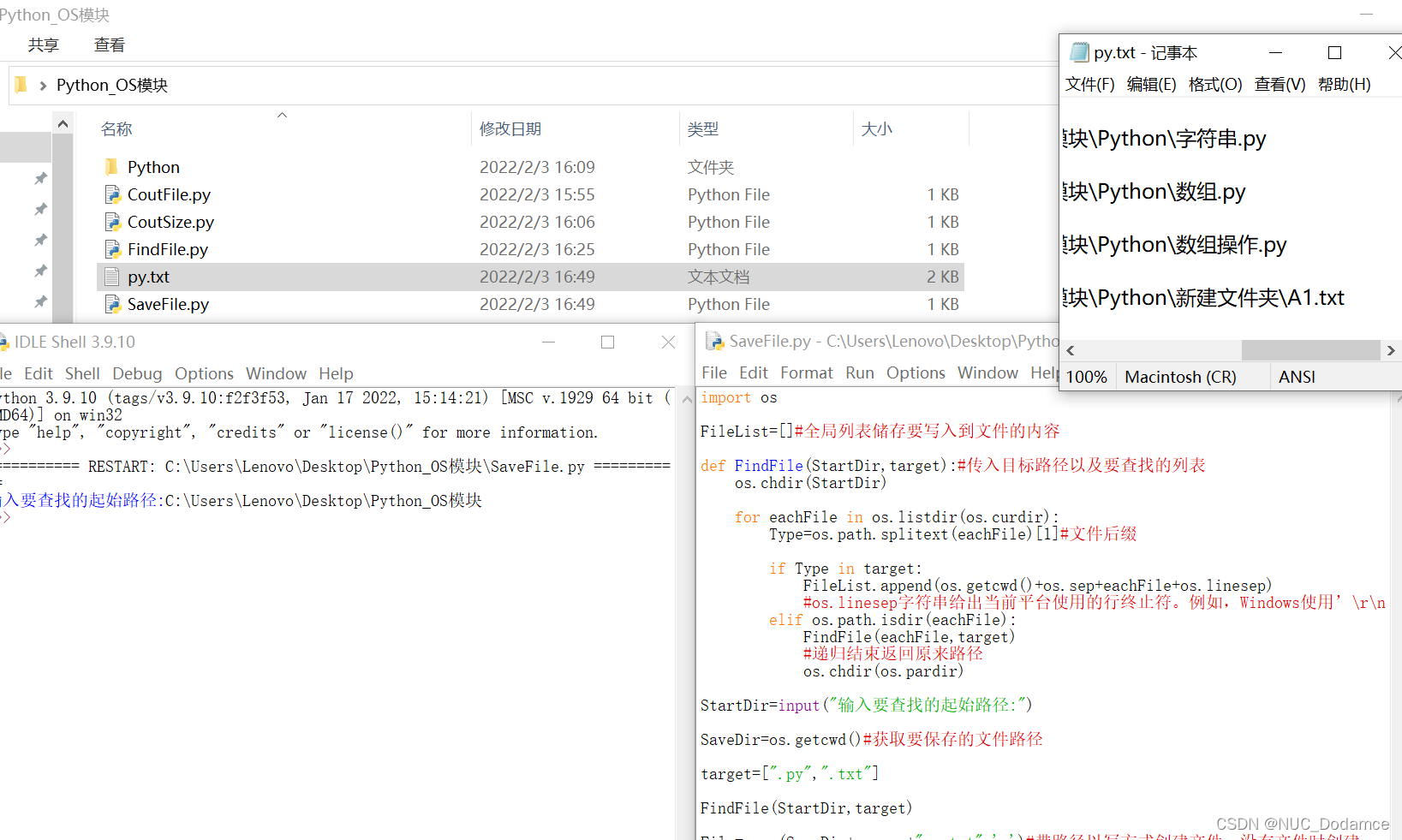
5.用户输入关键字,查找当前文件夹内(如果当前文件夹内包含文件夹,则进入文件夹继续搜索)所有含有该关键字的文本文件(.txt后缀),要求显示该文件所在的位置以及关键字在文件中的具体位置(第几行第几个字符)
UnicodeDecodeError: ‘gbk’ codec can’t decode byte错误解决
解决方法连接
import os
def PrintPos(Key_Pos):
keys=Key_Pos.keys()
keys=sorted(keys)
for each_key in keys:
print("关键字出现在第%s行,第%s个位置"%(each_key,str(Key_Pos[each_key])))
def PosInLine(line,Key):
Pos=[]
begin=line.find(Key)
while begin!=-1:
Pos.append(begin+1)
begin=line.find(Key,begin+1)
return Pos
def SearchTxT(FileName,Key):
File=open(FileName,'r',encoding='gbk',errors='ignore')
row=0
Key_Pos=dict()
for each_line in File:
row+=1
if Key in each_line:
Pos=PosInLine(each_line,Key)
Key_Pos[row]=Pos
File.close()
return Key_Pos
def FindFile(Key,flag):
Files=os.walk(os.getcwd())
TxT=[]
for i in Files:
for File in i[2]:
if os.path.splitext(File)[1] ==".txt":
File=os.path.join(i[0],File)
TxT.append(File)
for eachTxT in TxT:
Key_Pos=SearchTxT(eachTxT,Key)
if Key_Pos:
print("======================================================")
print("在文件[%s]中找到关键字(%s)"%(eachTxT,Key))
if flag in ["YES","Yes","yes"]:
PrintPos(Key_Pos)
print("请将该脚本放入要查找关键字的文件夹中")
Key=input("请输入要查找的关键字:")
flag=input("是否需要打印关键字[%s]在文件的具体位置(Yes/No):"%Key)
FindFile(Key,flag)
Stop=input()
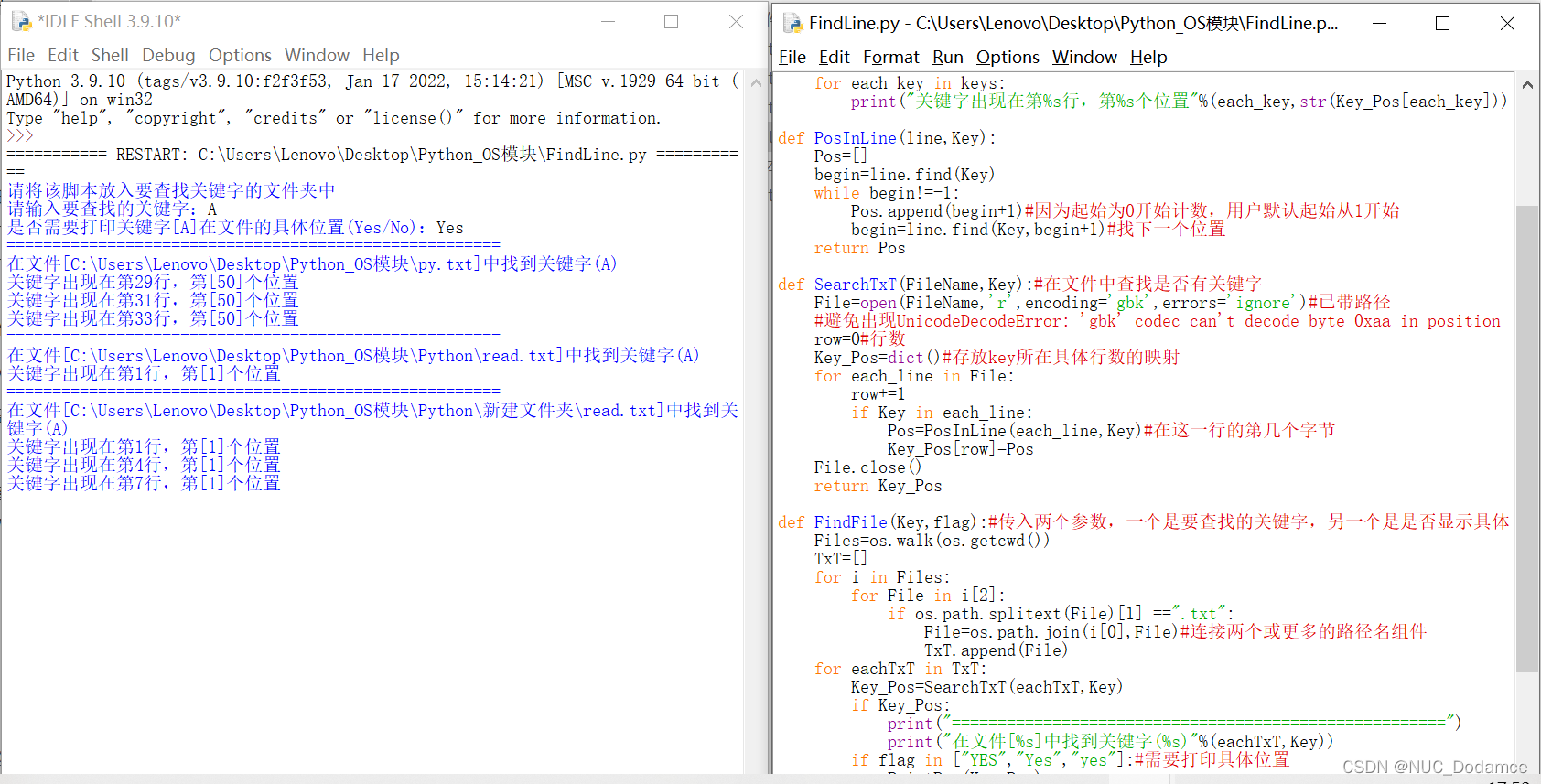
|