阿里云oss实现_添加讲师上传头像功能(后端)
1、在service模块下创建子模块service_oss
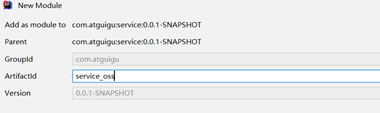 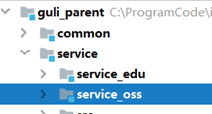
2、添加依赖
<dependencies>
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
</dependency>
<dependency>
<groupId>joda-time</groupId>
<artifactId>joda-time</artifactId>
</dependency>
</dependencies>
3、添加配置文件application.properties
#服务端口
server.port=8002
#服务名
spring.application.name=service-oss
#环境设置:dev、test、prod
spring.profiles.active=dev
4、创建启动类
@SpringBootApplication
@ComponentScan(basePackages = {"com.atguigu"})
public class OssApplication {
public static void main(String[] args) {
SpringApplication.run(OssApplication.class,args);
}
}
5、启动报错
由于该模块不需要数据库,所以排除注解就行 exclude = DataSourceAutoConfiguration.class
添加注解参数,解决问题
@SpringBootApplication(exclude = DataSourceAutoConfiguration.class)
@ComponentScan(basePackages = {"com.atguigu"})
public class OssApplication {
public static void main(String[] args) {
SpringApplication.run(OssApplication.class,args);
}
}
7、实现controller
@Api(description="文件管理")
@RestController
@RequestMapping("/ossservice/fileoss")
@CrossOrigin
public class FileController {
@Autowired
private FileService fileService;
@ApiOperation(value = "上传文件")
@PostMapping("uploadFile")
public R uploadFile(MultipartFile file){
String url = fileService.uploadFileOSS(file);
return R.ok().data("url",url);
}
}
## 8、实现service
@Service
public class FileServiceImpl implements FileService {
@Override
public String uploadFileOSS(MultipartFile file) {
String endpoint = "oss-cn-beijing.aliyuncs.com";
String accessKeyId = "LTAI3buexRAagkdy";
String accessKeySecret = "A6hpWJbF3Zz6wj3jxuBe40Mwryt1Zz";
String bucketName = "guli-file201021";
String fileName = file.getOriginalFilename();
OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
try {
InputStream inputStream = file.getInputStream();
ossClient.putObject(bucketName, fileName, inputStream);
ossClient.shutdown();
String url ="https://"+bucketName+"."+endpoint+"/"+fileName;
return url;
} catch (IOException e) {
e.printStackTrace();
throw new GuliException(20001,"上传失败");
}
}
}
添加讲师上传头像接口(完善)
1、提出跟环境相关参数
(1)添加配置信息
#阿里云 OSS
#不同的服务器,地址不同
aliyun.oss.file.endpoint=oss-cn-beijing.aliyuncs.com
aliyun.oss.file.keyid=LTAI3buexRAagkdy
aliyun.oss.file.keysecret=A6hpWJbF3Zz6wj3jxuBe40Mwryt1Zz
#bucket可以在控制台创建,也可以使用java代码创建
aliyun.oss.file.bucketname=guli-file201021
(2)创建工具类,读取配置信息
@Component
public class ConstantPropertiesUtil implements InitializingBean {
@Value("${aliyun.oss.file.endpoint}")
private String endpoint;
@Value("${aliyun.oss.file.keyid}")
private String keyId;
@Value("${aliyun.oss.file.keysecret}")
private String keySecret;
@Value("${aliyun.oss.file.bucketname}")
private String bucketName;
public static String END_POINT;
public static String ACCESS_KEY_ID;
public static String ACCESS_KEY_SECRET;
public static String BUCKET_NAME;
@Override
public void afterPropertiesSet() throws Exception {
END_POINT = endpoint;
ACCESS_KEY_ID = keyId;
ACCESS_KEY_SECRET = keySecret;
BUCKET_NAME = bucketName;
}
(3)改造接口方法
String endpoint = ConstantPropertiesUtil.END_POINT;
String accessKeyId = ConstantPropertiesUtil.ACCESS_KEY_ID;
String accessKeySecret = ConstantPropertiesUtil.ACCESS_KEY_SECRET;
String bucketName = ConstantPropertiesUtil.BUCKET_NAME;
String fileName = file.getOriginalFilename();
(4)测试
2、优化文件名,不能重复
fileName = UUID.randomUUID().toString()+fileName;
3、优化文件存储路径
String path = new DateTime().toString("yyyy/MM/dd");
fileName = path+"/"+fileName;
4、测试
|