一、分析
首先用postman测试对应的接口,测试如下
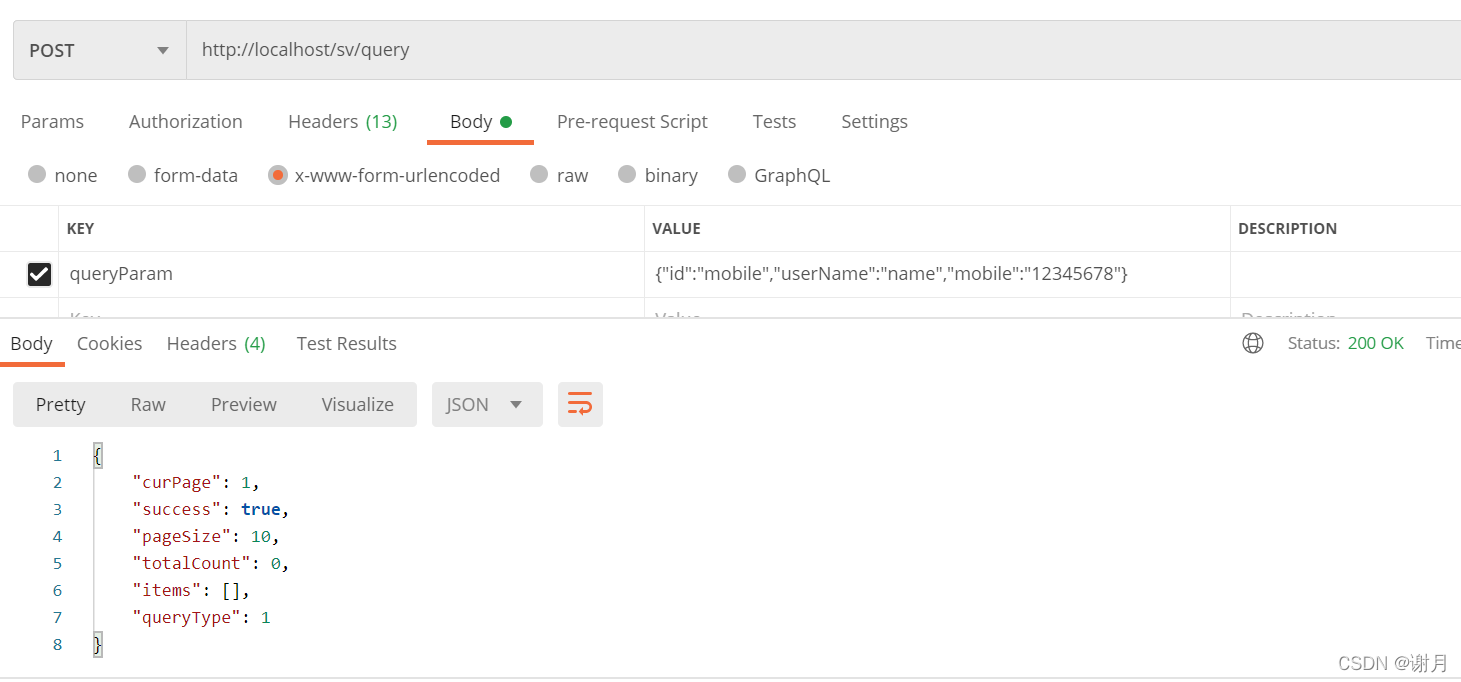
?其中请求头中content-type为application/x-www-form-urlencoded
参数是:queryParam:{"id":"mobile","userName":"name","mobile":"12345678"}
注意:我们进行API调用时,参数需要转为String类型,平时我们调用get请求application/x-www-form-urlencoded参数都是直接拼接在url后面,? ? ? ? ? ? ? ? ? ? ? ? ? ??url?字段名=值&字段名=值,所以这里用同样的方式进行参数处理。
二、代码样例
public String getNameCode(String name,String phone) throws Exception {
// 1.组装数据,以及请求头
Map header = new HashMap();
String newParam = "queryParam={\"id\":\"mobile\",\"userName\":\""+name+"\",\"mobile\":\""+phone+"\"}";
header.put("content-type","application/x-www-form-urlencoded");
// 3.调用接口查询
String testRst = HttpUtil.doPost("http://localhost/sv/query",newParam,header);
if(testRst==null){
throw new Exception("bomc接口响应失败,请稍后重试");
}
// 4.解析结果集
JSONObject json = JSONObject.parseObject(testRst);
JSONArray item = json.getJSONArray("items");
JSONObject obj = item.getJSONObject(0);
String account = (String) obj.get("userAccount");
return account;
}
public static String doPost(String url, String params, Map header) throws Exception {
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);// 创建httpPost
logger.info("POST请求url:" + url);
for (Iterator iter = header.keySet().iterator(); iter.hasNext(); ) {
String key = String.valueOf(iter.next());
String value = String.valueOf(header.get(key));
httpPost.setHeader(key, value);
}
RequestConfig requestConfig = RequestConfig.custom().setConnectionRequestTimeout(20000)
.setSocketTimeout(20000).setConnectTimeout(20000).build();
httpPost.setConfig(requestConfig);
//设置参数
logger.info("POST请求参数:" + params);
StringEntity entity = new StringEntity(params, "utf-8");
entity.setContentEncoding("UTF-8");
entity.setContentType("application/json");
httpPost.setEntity(entity);
CloseableHttpResponse response = null;
try {
response = httpclient.execute(httpPost);
StatusLine status = response.getStatusLine();
int state = status.getStatusCode();
if (state == HttpStatus.SC_OK) {
HttpEntity responseEntity = response.getEntity();
String jsonString = EntityUtils.toString(responseEntity,"UTF-8");
return jsonString;
} else {
logger.error("请求返回:" + state + "(" + url + ")");
}
} finally {
if (response != null) {
try {
response.close();
} catch (IOException e) {
e.printStackTrace();
}
}
try {
httpclient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return null;
}
|