1 C++回顾
1.1 命名空间
- C++可以重复命名函数,比如好几个
fun() 函数,但是 () 中的传值要不一样,数量要不一样,这样才能进行重复的命名,看下面1.7的函数重载 - C++的大部分定义和函数都在 std 命名空间中,所以使用时一般都会加上
using namespace std;
命名空间:
防止工程过大
所导致的全局变量命名重复
所有的变量甚至是函数都可以写到这个命名空间内部!
可以防止重复命名
命名空间的定义:
命名空间有空间名!
#include <iostream>
using namespace std;
int main()
{
cout << "Hello World";
return 0;
}
1.2 输入输出流
C语言输入输出
三大标准文件:
stdin stdout stderr
一些函数比如下面的两个都是在这里面的
printf();
scanf();
C++
首先可以用C语言的输入输出!
他还可以用
cout
cin输入
注意:
cout 与 cin属于 std空间
所以使用的时候必须声明命名空间才可以使用
1.3 引用
传参,什么情况下传的是 值 ,什么情况下传参,四个函数的使用方法
C++的引用
原则上是给变量起一个别名!
实际上是为了方便传参
举个例子:
int a = 10;
int &b =a;
变量a 他的别名 就是 b
a 和 b 指向的是同一个空间
更多的这种情况是为了传参方便
而不是用指针传参
#include <iostream>
void fun(int num)
{
num++;
}
int fun1(int num)
{
num++;
return num;
}
void fun2(int * num)
{
(* num) ++;
}
void fun3(int &num)
{
num++;
}
int main()
{
int num = 10;
fun(num);
printf("num==%d\r\n",num);
num = fun1(num);
printf("num==%d\r\n",num);
fun2(&num);
printf("num==%d\r\n",num);
fun3(num);
printf("num==%d\r\n",num);
return 0;
}
1.4 bool类型
C语言中有正确错误的概念
正确在C语言中就是 非0->1
错误在C语言中就是 0
C++为了更加方便区分正确和错误
专门搞出来bool
这个bool类型一共有两个值
true false
1 0
1.5 字符串类型
- string 相当于C语言的字符串、数组,甚至是一些函数
- 甚至可以做到C语言用函数才能做到事情等等
- 当然直接使用C语言中的操作也可以
例如:
string str1;
string str2;
str1 = "hello"
str2 = "hello"
str1 == str2
strcmp
str3 = str1+str2;
strcat
1.6 函数默认参数
有些函数会有默认的参数传进去,防止出现一些错误
在C++函数允许有默认传参
大部分的功能、库、外设
都会提供一定的功能;
这些函数需要你传入不同的参数完成不同的功能
open_led(int port);
由于我根本不了解 port-->引脚
open_led(10000);
可能这个函数不但不能点亮LED灯
甚至会造成系统错误。。。
由于可能会出现这样的问题
C++推出这种默认传参的机制
当我不知道该怎么传参的时候
直接调用函数即可
有些函数会有默认的参数
void fun(string str="hello world")
{
cout<<str;
}
很多情况下
函数的功能
Qt、OpenCv函数都会拥有默认传参
默认传参的功能一般都是防止不传参导致的问题
1.7 函数的重载
允许函数名字重复,前提是参数不能重复,如下面的示例
C++函数的重载
正常在C语言中
机会是不能看到一个函数名字重复的函数
但是这种情况在C++中很常见很常见!
在C++中允许函数名字重复
但是前提是名字可以重复你的参数是不能重复的!
这样的函数我们叫做函数的重载!
void fun(void)
{
cout << "i am fun1"<<endl;
}
int fun(int num,int num2)
{
cout << "i am fun2"<<endl;
}
int fun(int num,int num2,int num3)
{
cout << "i am fun3"<<endl;
}
void fun(int num)
{
num++;
cout << "i am fun4"<<endl;
}
2 在Linux中安装VScode
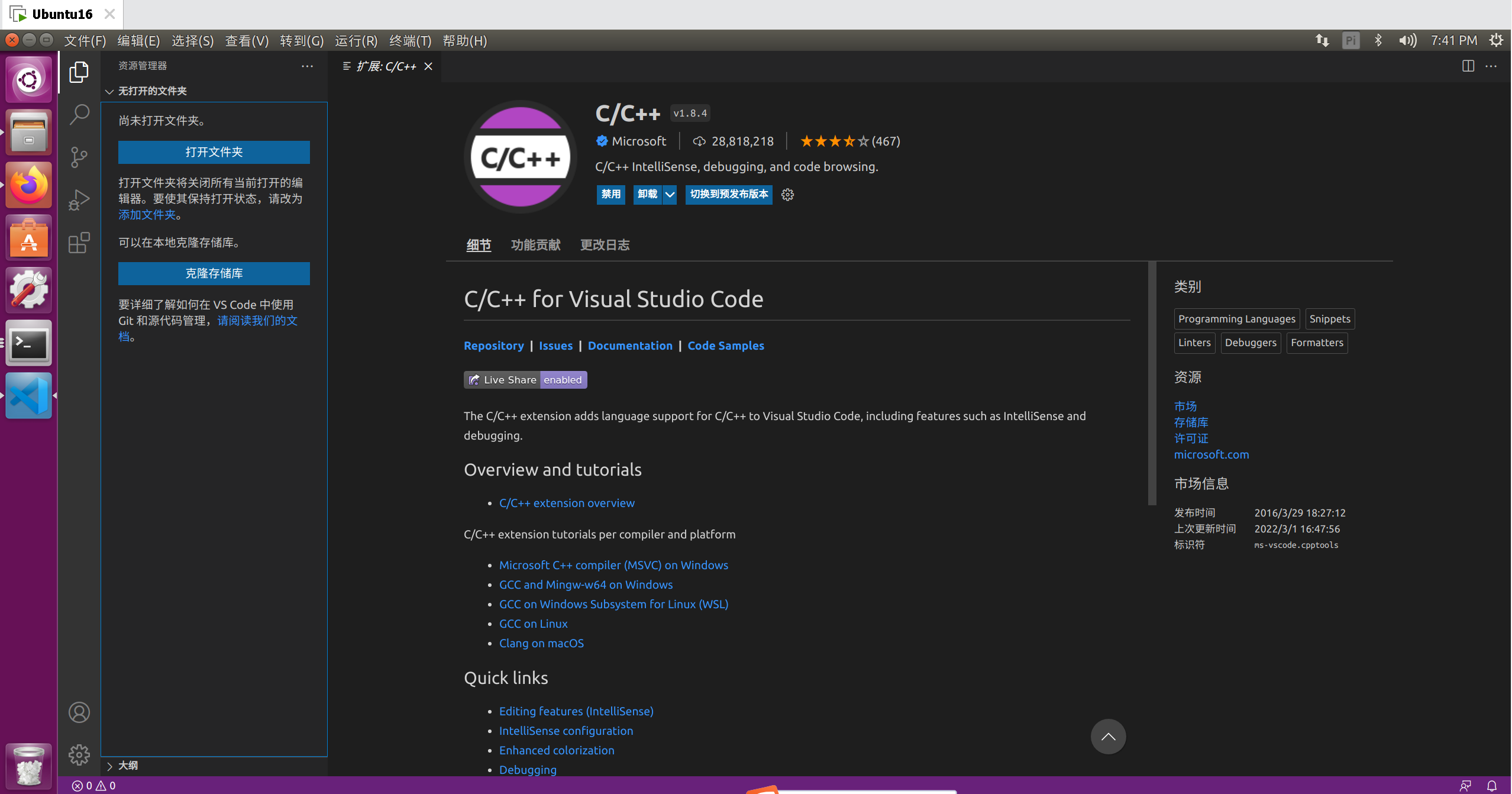
2.1 下载方式
2.1.1 官网下载
https://code.visualstudio.com/
2.1.2 百度网盘
链接:https://pan.baidu.com/s/1tdoHH76BpJins-AGiP4dbw 提取码:7j9t
2.2 下载VScode到Linux中
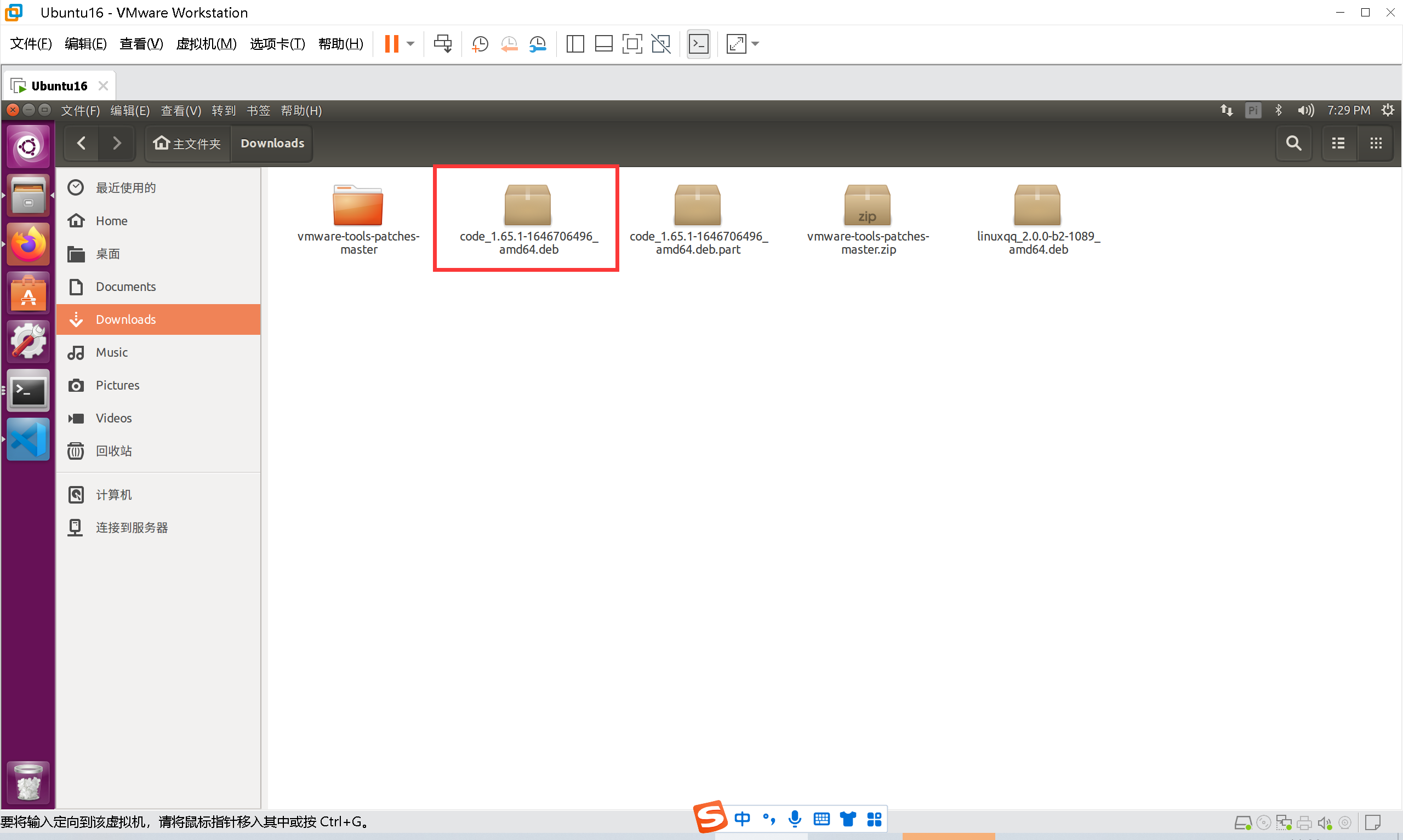
2.3 安装VScode
找到文件位置,在文件的所在位置打开终端,输入 sudo dpkg -i code_1.65.1-1646706496_amd64.deb 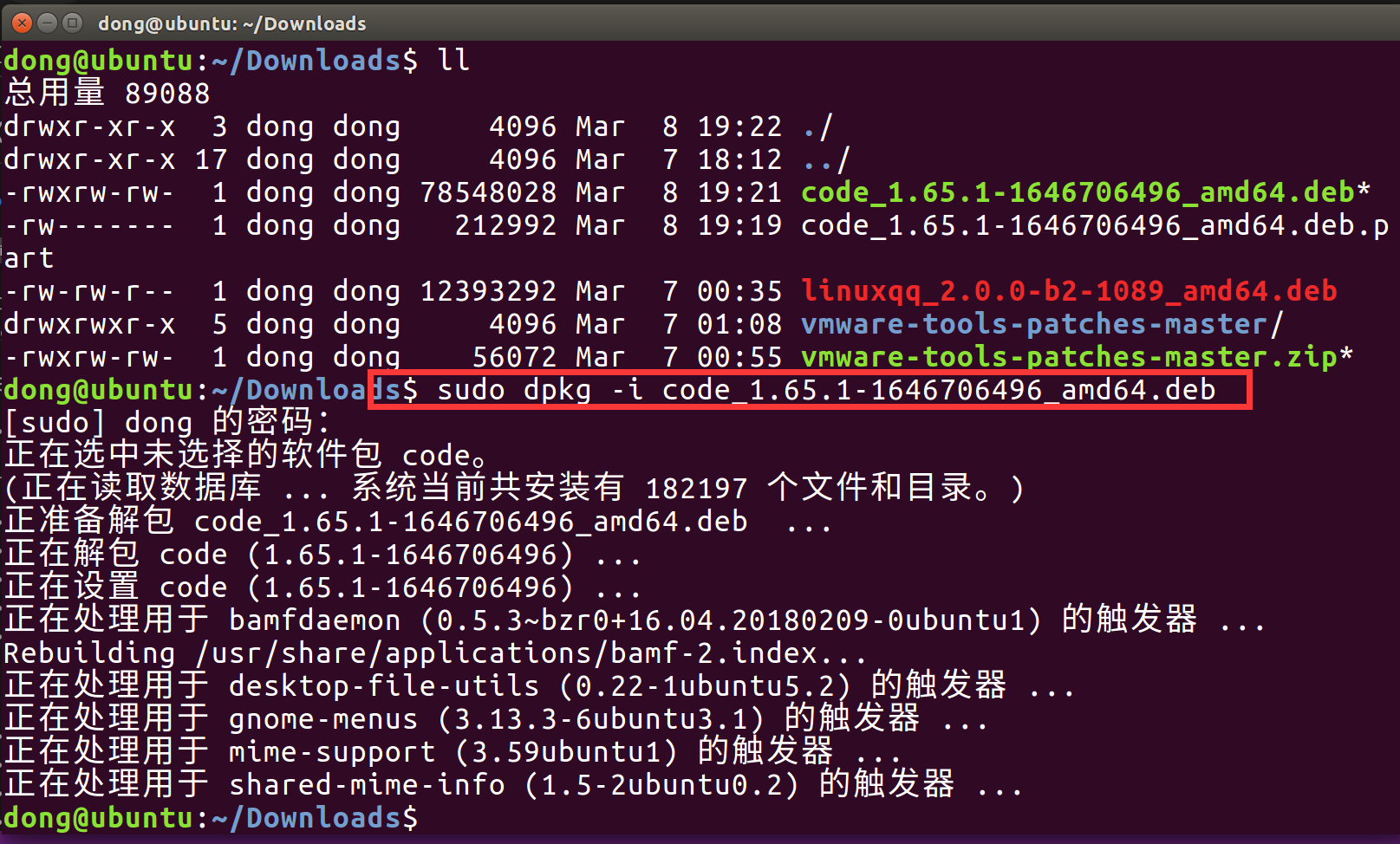
2.4 安装Vscode插件
1)、 C/C++,这个肯定是必须的。 2)、 C/C++ Snippets,即 C/C++重用代码块。 3)、 C/C++ Advanced Lint,即 C/C++静态检测 。 4)、 Code Runner,即代码运行。 5)、 Include AutoComplete,即自动头文件包含。 6)、 Rainbow Brackets,彩虹花括号,有助于阅读代码。 8)、 GBKtoUTF8,将 GBK 转换为 UTF8。 9)、 ARM,即支持 ARM 汇编语法高亮显示。 10)、 Chinese(Simplified),即中文环境。 11)、 vscode-icons, VSCode 图标插件,主要是资源管理器下各个文件夹的图标。 12)、 compareit,比较插件,可以用于比较两个文件的差异。 13)、 DeviceTree,设备树语法插件。
2.5 用VScode打开项目
一般是以文件夹为单位 比如使用 code ./demo 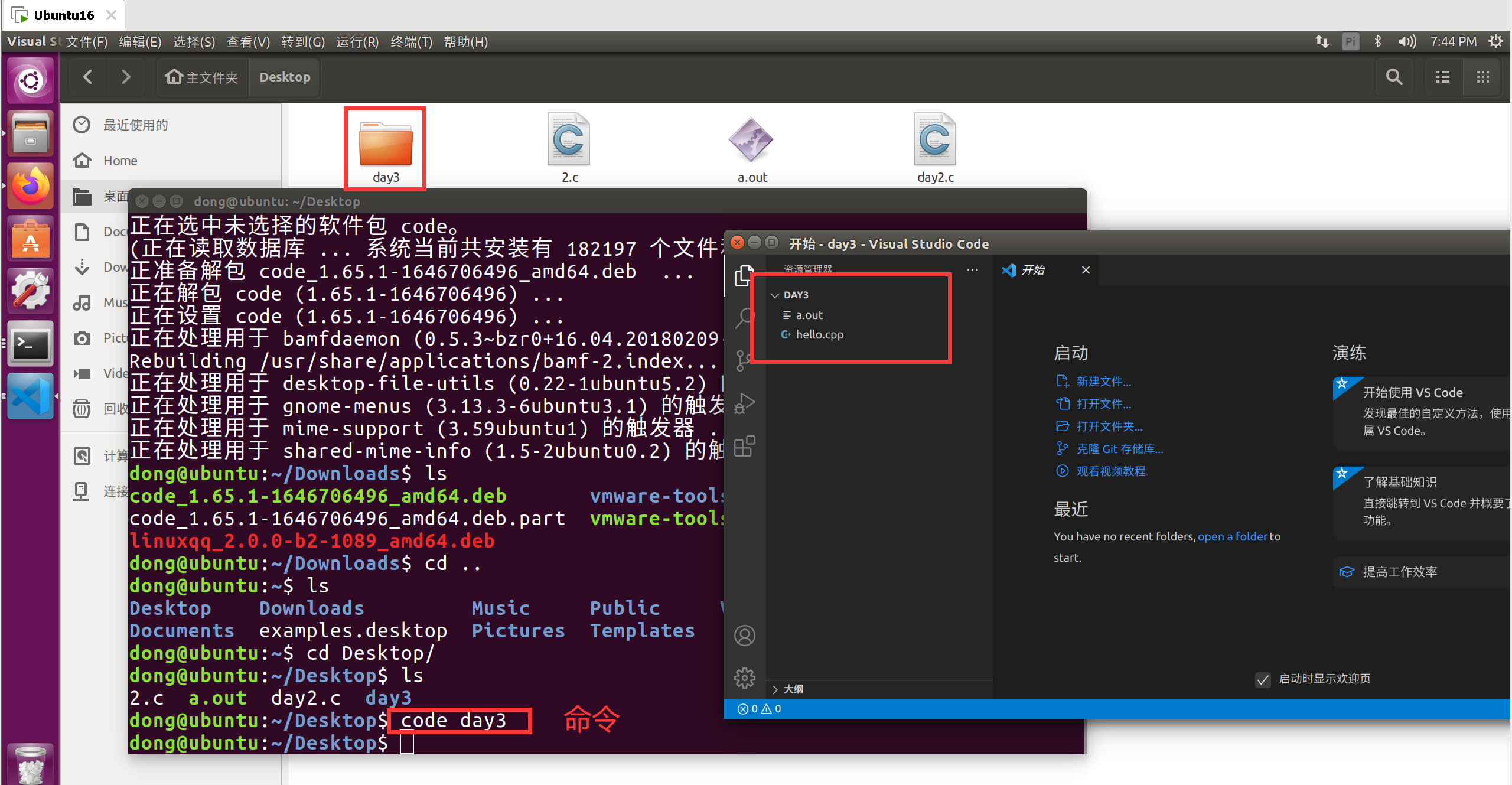
2.6 使用gdb进行Dbug调试
gdb教程: http://c.biancheng.net/gdb/
3 C++的类
类似于C的结构体
- 抽象 -> 描述一条狗,一个人,多种数据集合甚至有函数
- 封装 -> public 等等,公有、隐私等
- 继承 -> 集成其它类的内容,关键字
class - 多态 -> 多态性(polymorphism)可以简单地概括为“一个接口,多种方法”,它是面向对象编程领域的核心概念
3.1 类的实例化
类的定义:
class student
{
int age;
string name;
string birth;
};
class student tmp;
tmp.age;
tmp.name;
tmp.birth
3.2 类的其他特点
C++ 类访问修饰符——菜鸟教程
类内部的所有成员都会有自己的属性,属性决定了成员的权限 类的成员有三个属性 -> public private protected
public:
#include<iostream>
#include<assert.h>
using namespace std;
class A{
public:
int a;
A(){
a1 = 1;
a2 = 2;
a3 = 3;
a = 4;
}
void fun(){
cout << a << endl;
cout << a1 << endl;
cout << a2 << endl;
cout << a3 << endl;
}
public:
int a1;
protected:
int a2;
private:
int a3;
};
class B : public A{
public:
int a;
B(int i){
A();
a = i;
}
void fun(){
cout << a << endl;
cout << a1 << endl;
cout << a2 << endl;
cout << a3 << endl;
}
};
int main(){
B b(10);
cout << b.a << endl;
cout << b.a1 << endl;
cout << b.a2 << endl;
cout << b.a3 << endl;
system("pause");
return 0;
}
private:
- 私有成员变量或函数在类的外部是不可访问的,甚至是不可查看的。只有类和友元函数可以访问私有成员
- 默认情况下,类的所有成员都是私有的
#include<iostream>
#include<assert.h>
using namespace std;
class A{
public:
int a;
A(){
a1 = 1;
a2 = 2;
a3 = 3;
a = 4;
}
void fun(){
cout << a << endl;
cout << a1 << endl;
cout << a2 << endl;
cout << a3 << endl;
}
public:
int a1;
protected:
int a2;
private:
int a3;
};
class B : private A{
public:
int a;
B(int i){
A();
a = i;
}
void fun(){
cout << a << endl;
cout << a1 << endl;
cout << a2 << endl;
cout << a3 << endl;
}
};
int main(){
B b(10);
cout << b.a << endl;
cout << b.a1 << endl;
cout << b.a2 << endl;
cout << b.a3 << endl;
system("pause");
return 0;
}
protected:
- protected(受保护)成员变量或函数与私有成员十分相似,但有一点不同,protected(受保护)成员在派生类(即子类)中是可访问的
#include<iostream>
#include<assert.h>
using namespace std;
class A{
public:
int a;
A(){
a1 = 1;
a2 = 2;
a3 = 3;
a = 4;
}
void fun(){
cout << a << endl;
cout << a1 << endl;
cout << a2 << endl;
cout << a3 << endl;
}
public:
int a1;
protected:
int a2;
private:
int a3;
};
class B : protected A{
public:
int a;
B(int i){
A();
a = i;
}
void fun(){
cout << a << endl;
cout << a1 << endl;
cout << a2 << endl;
cout << a3 << endl;
}
};
int main(){
B b(10);
cout << b.a << endl;
cout << b.a1 << endl;
cout << b.a2 << endl;
cout << b.a3 << endl;
system("pause");
return 0;
}
3.3 C++的函数
类内实现:比较常见 类外实现:在类内声明,在类外实现(函数体在类外),Qt比较常用,比如Qt的槽函数
class student
{
public:
int a;
void fun(void)
{
b = b+1;
c = c+1;
}
void test(void);
private:
int b;
protected:
int c;
};
void student::test(void)
{
cout<<"hello i am student test!\r\n";
}
3.4 构造函数和析构函数
构造函数(和类名相同):实例化(创建对象)的时候必然要调用的初始化函数,对象创建时自动调用 析构函数(~ 加上类名):对象摧毁的时候回调的释放的函数,对象被摧毁的时候自动调用(return的时候)
class Student
{
private:
public:
Student();
~Student();
};
Student::Student()
{
}
Student::~Student()
{
}
3.5 类的继承
直接继承父类的内容
格式 继承的权限
采用公共继承的情况下:
class student
{
public:
int a;
private:
int b;
protected:
int c;
};
class newstudent:public student
{
public:
int d;
void fun(void)
{
c =20;
}
};
公共的继承
class newstudent:public student
相当于
class newstudent
{
public:
int d;
int a;
void fun(void)
{
c =20;
}
protected:
int c;
}
受保护继承
class newstudent:protected student
class newstudent
{
public:
int d;
void fun(void)
{
c =20;
}
protected:
int a;
int c;
}
私有继承
class newstudent:private student
相当于:
class newstudent
{
public:
int d;
void fun(void)
{
c =20;
}
private:
int a;
int c;
}
|