1- 登录获取token 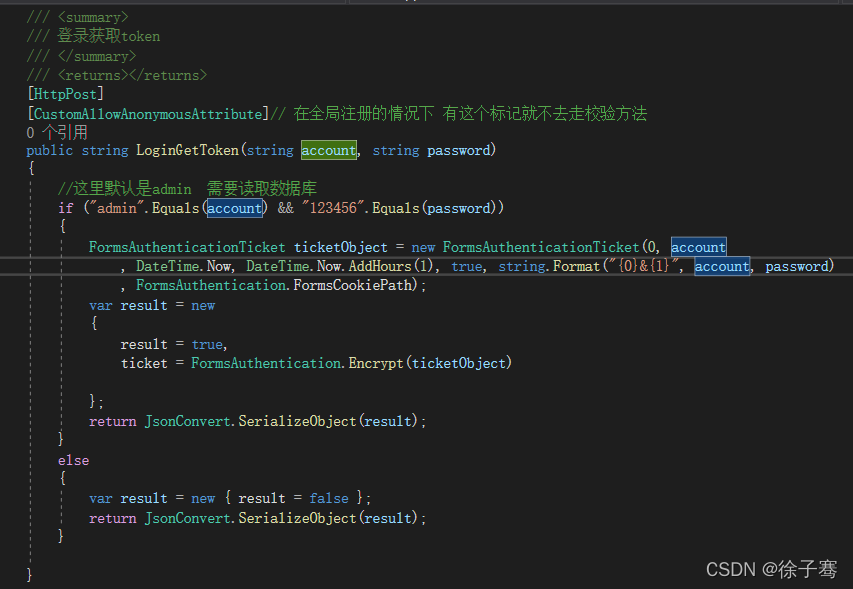
[HttpPost]
[CustomAllowAnonymousAttribute]
public string LoginGetToken(string account, string password)
{
if ("admin".Equals(account) && "123456".Equals(password))
{
FormsAuthenticationTicket ticketObject = new FormsAuthenticationTicket(0, account
, DateTime.Now, DateTime.Now.AddHours(1), true, string.Format("{0}&{1}", account, password)
, FormsAuthentication.FormsCookiePath);
var result = new
{
result = true,
ticket = FormsAuthentication.Encrypt(ticketObject)
};
return JsonConvert.SerializeObject(result);
}
else
{
var result = new { result = false };
return JsonConvert.SerializeObject(result);
}
}
我这里案例是默认的用admin账号,正常开发过程中需要去数据库读取
2-解密验证 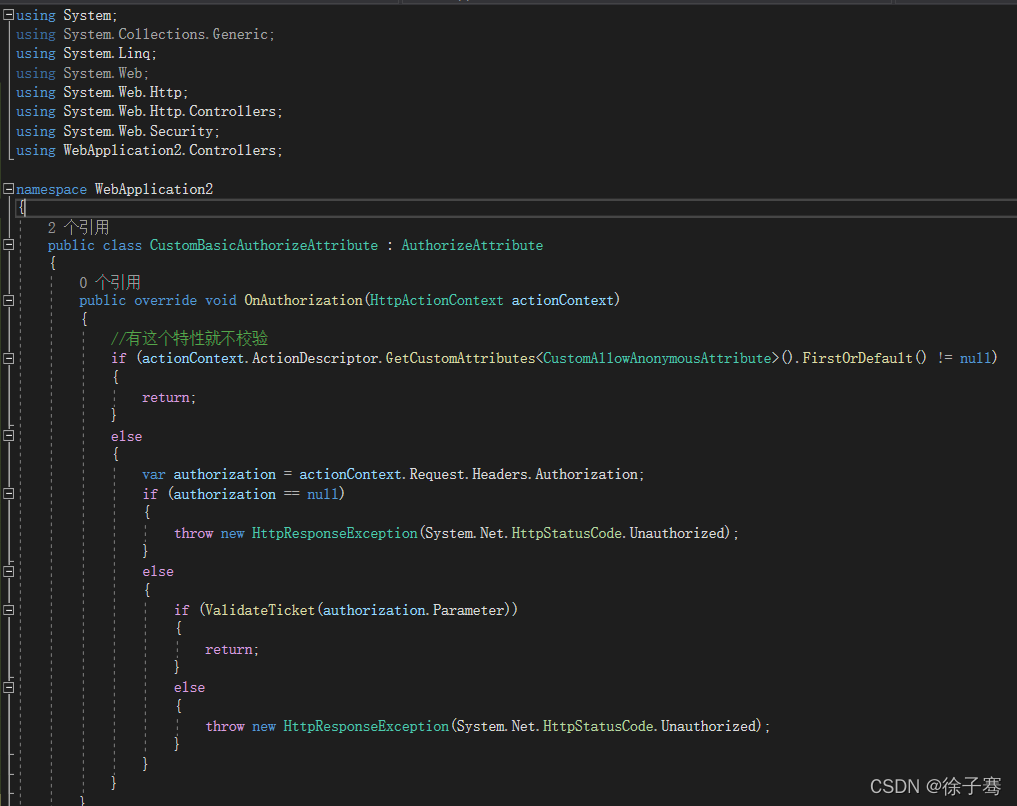 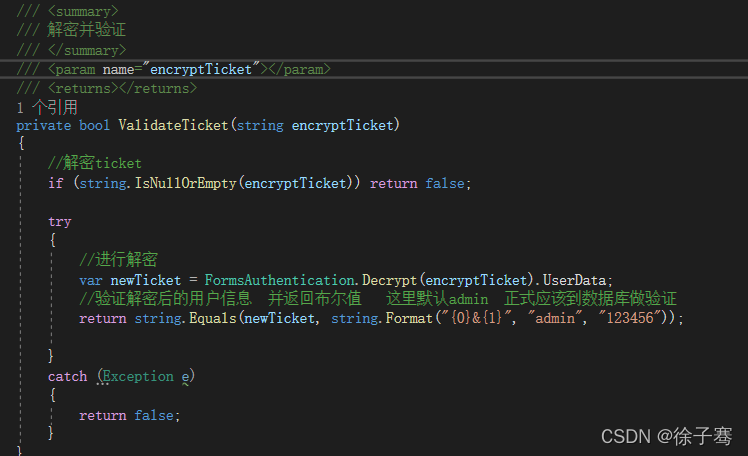
public class CustomBasicAuthorizeAttribute : AuthorizeAttribute
{
public override void OnAuthorization(HttpActionContext actionContext)
{
if (actionContext.ActionDescriptor.GetCustomAttributes<CustomAllowAnonymousAttribute>().FirstOrDefault() != null)
{
return;
}
else
{
var authorization = actionContext.Request.Headers.Authorization;
if (authorization == null)
{
throw new HttpResponseException(System.Net.HttpStatusCode.Unauthorized);
}
else
{
if (ValidateTicket(authorization.Parameter))
{
return;
}
else
{
throw new HttpResponseException(System.Net.HttpStatusCode.Unauthorized);
}
}
}
}
private bool ValidateTicket(string encryptTicket)
{
if (string.IsNullOrEmpty(encryptTicket)) return false;
try
{
var newTicket = FormsAuthentication.Decrypt(encryptTicket).UserData;
return string.Equals(newTicket, string.Format("{0}&{1}", "admin", "123456"));
}
catch (Exception e)
{
return false;
}
}
}
3-在配置文件中全局注册 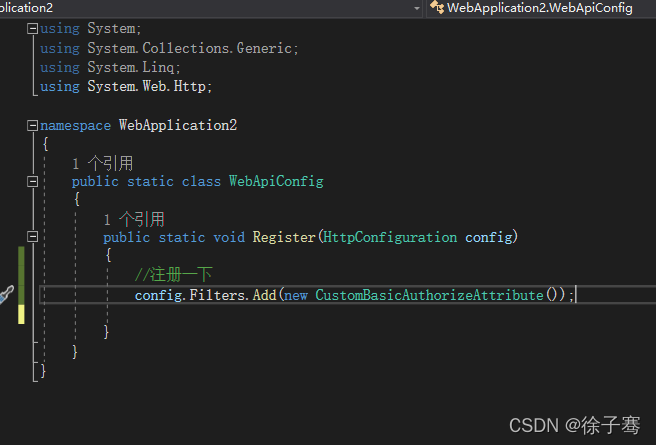
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
config.Filters.Add(new CustomBasicAuthorizeAttribute());
}
}
4-新建特性自定义类 继承Attribute就可以无需其他操作 目的是在全局注册的情况下 有这个标记就不去走校验方法 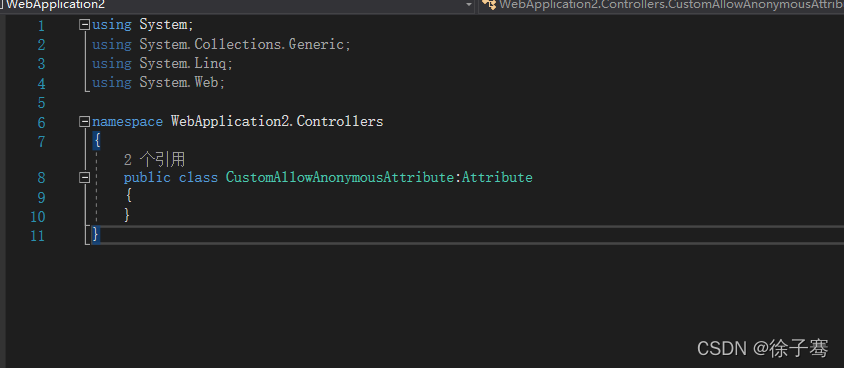
public class CustomAllowAnonymousAttribute:Attribute
{
}
|