概述
输出流
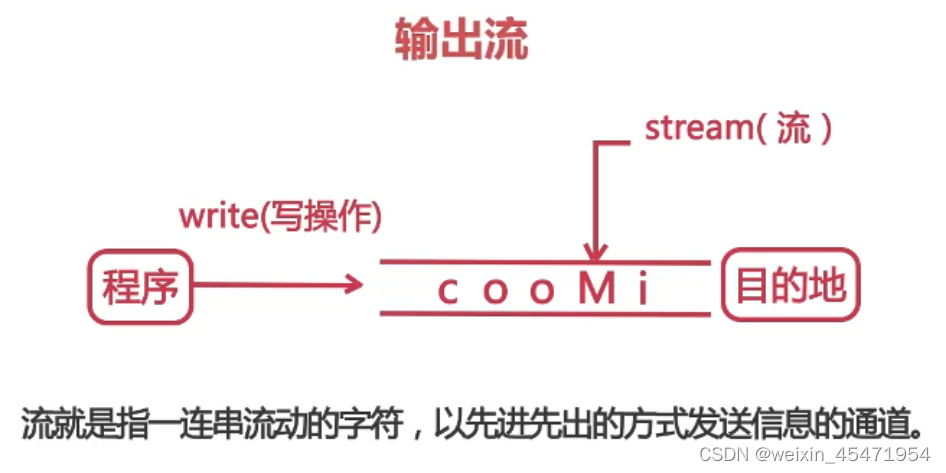
输入流
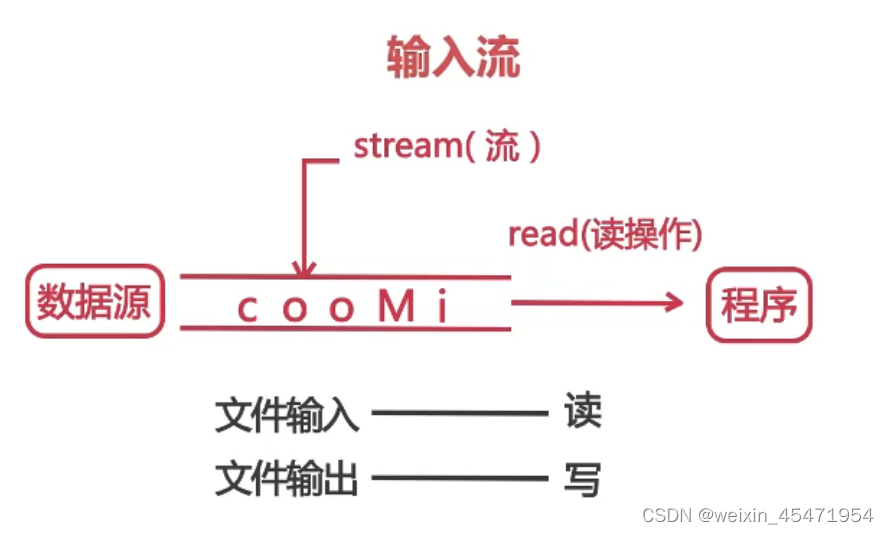
要掌握内容
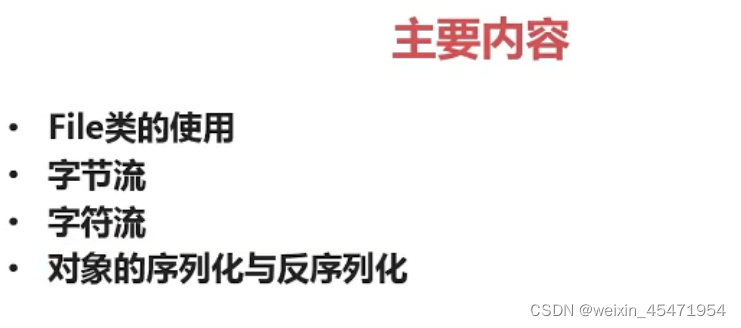
file类概述
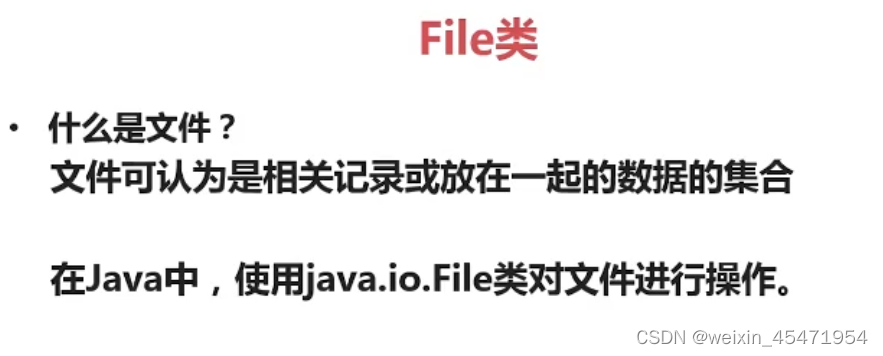
对文件使用一些常用api

public static void main(String[] args) {
File file = new File("d:\\imooc\\set\\io\\score.txt");
System.out.println("是否是目录:"+file.isDirectory());
System.out.println("是否是文件:"+file.isFile());
File file1 = new File("d:\\imooc\\set\\io\\HashSet");
if(!file1.exists()){
file1.mkdir();
}
}
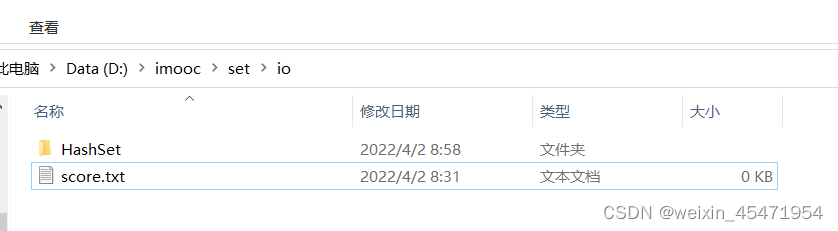
绝对路径和相对路径
绝对路径:从盘符开始的路径 相对路径:从当前路径开始的路径 相对路径理解 java文件和 thread.txt文件处于同一目录下(用命令实现java命令时)
若使用idea或eclipse编译器进行编译时,其会将文件存放于工程目录下面 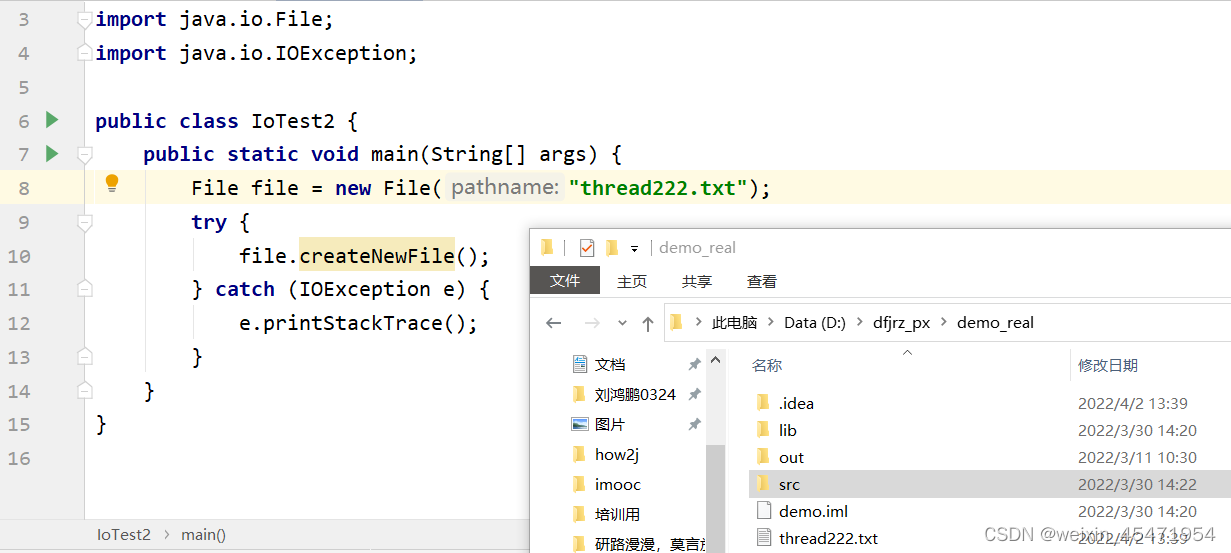
字节流
字节输入和输出流
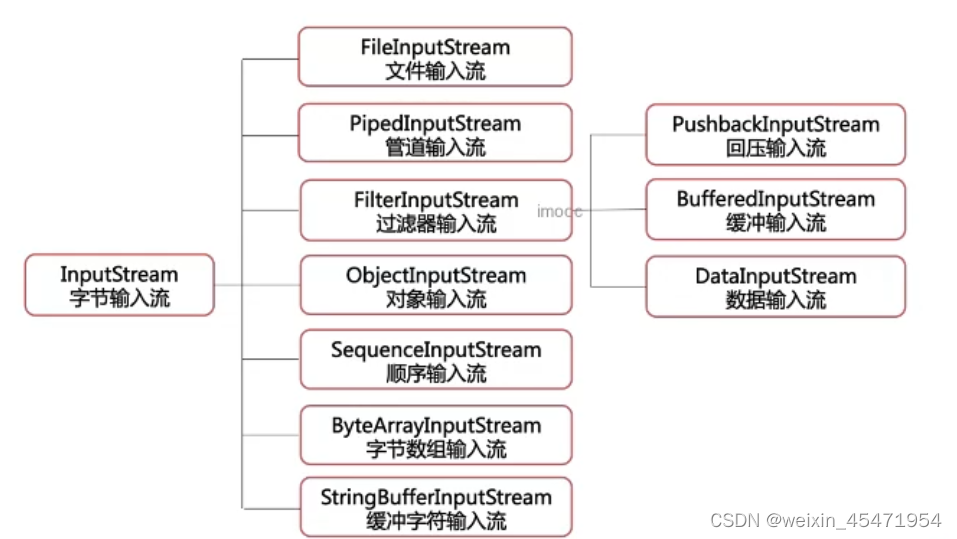 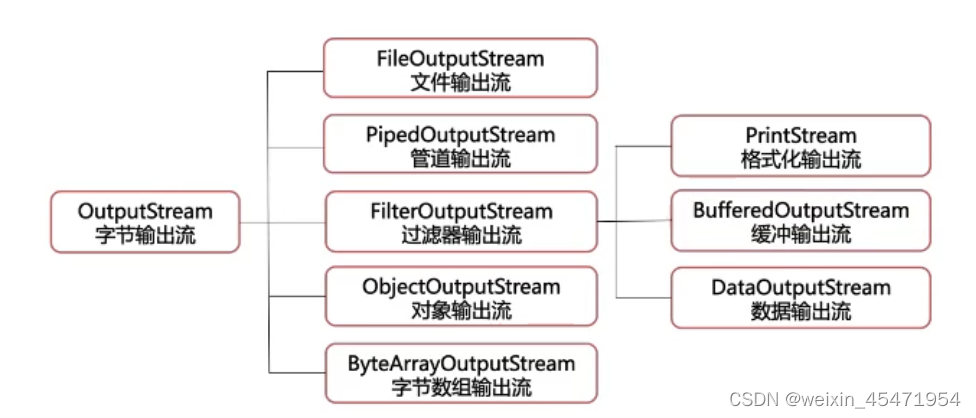
FileInputStream
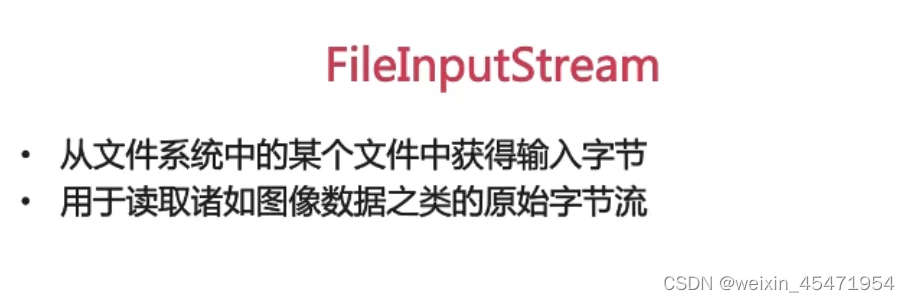
常用api
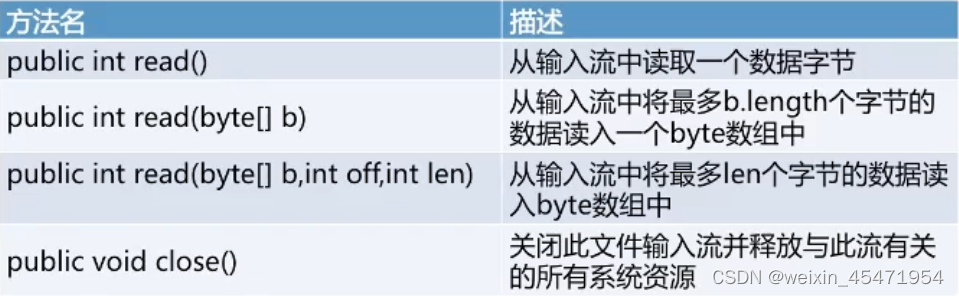 例
public static void main(String[] args) {
FileInputStream file = null;
try {
file = new FileInputStream("hello.txt");
int n =file.read();
while (n!=-1){
System.out.println((char)n);
n=file.read();
}
file.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
该程序的运行结果如下所示
H
e
l
l
o
**说明:**这里read方法返回值为-1,也即读到文件末尾
FileOutputStream
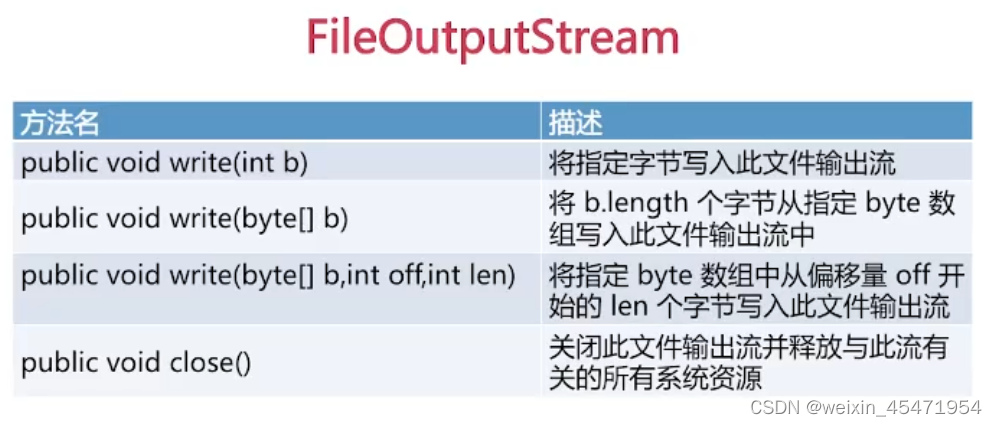 使用方法同FileInputStream **注意:**字节流主要用于跟二进制相关的数据
字符流
概述
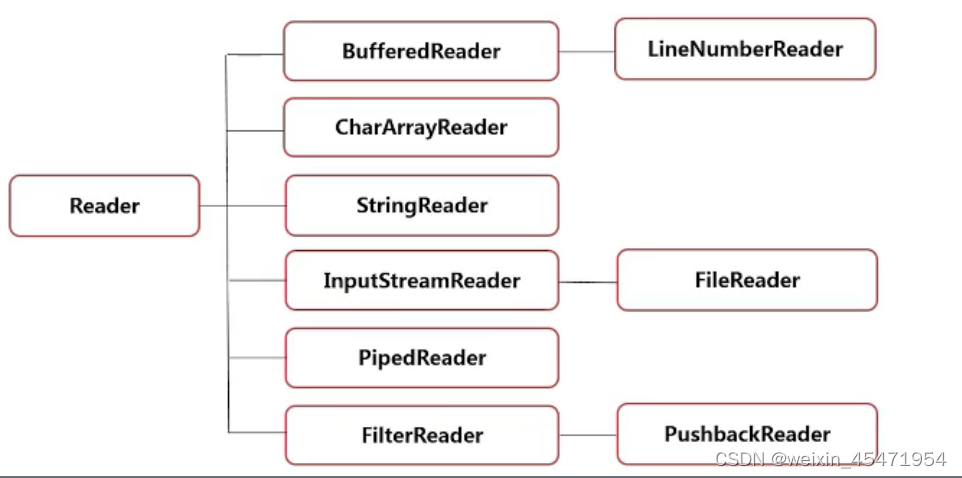 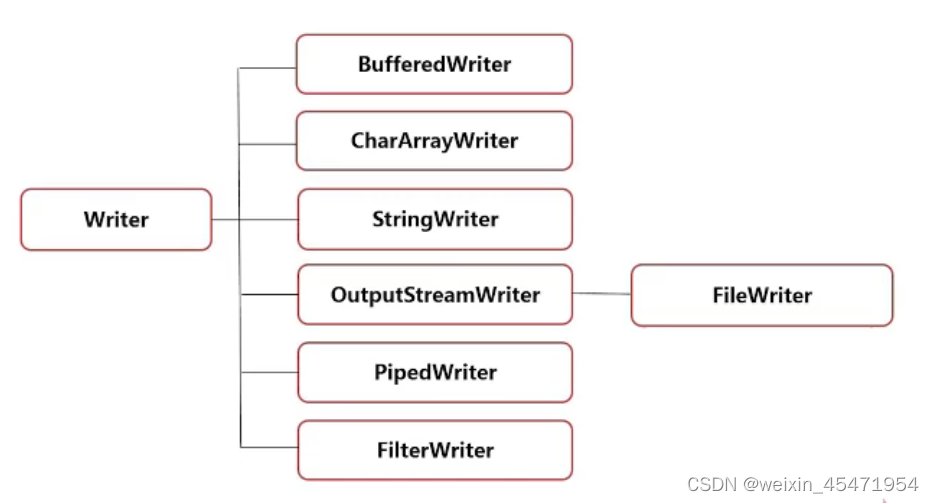 这里注意区分与字节流的区别 字节流:读取和写入 都是需要二进制格式的数据 字符流:qq发消息 发的是字符,网络传输的时候 读取的时候,需要转化为字符
字符转换流
用法差不多 直接上例子
public static void main(String[] args) {
FileInputStream fis = null;
try {
fis = new FileInputStream("hello.txt");
InputStreamReader isr = new InputStreamReader(fis);
int n=0;
char[] cbfu = new char[10];
while((n=isr.read())!=-1){
System.out.print((char)n);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
所读入的文件内容为 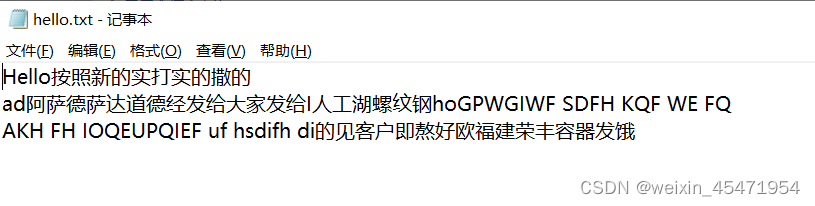
该程序的运行结果如下所示
Hello按照新的实打实的撒的
ad阿萨德萨达道德经发给大家发给I人工湖螺纹钢hoGPWGIWF SDFH KQF WE FQ
AKH FH IOQEUPQIEF uf hsdifh di的见客户即熬好欧福建荣丰容器发饿
输出方式类似,new出字节流对象,然后将其进行输入流转换
其他字符流
BufferedReader 和BufferedWriter 字符缓冲流,增加传输效率
FileInputStream fis = new FileInputStream("hello.txt");
InputStreamReader isr = new InputStreamReader(fis);
BufferedReader br = new BufferedReader(isr);
简单来说,就是对转过来的字符在套上一层缓冲,其他一样 **疑惑:**为什么需要通过字节流读取数据? 这是因为 这里模仿的是 数据从网络上传输过来(二进制),实际上,字符流也有自己的读取文件类。
对象序列化
场景:将多条信息进行封装成一个对象,进行传输过去
实现步骤
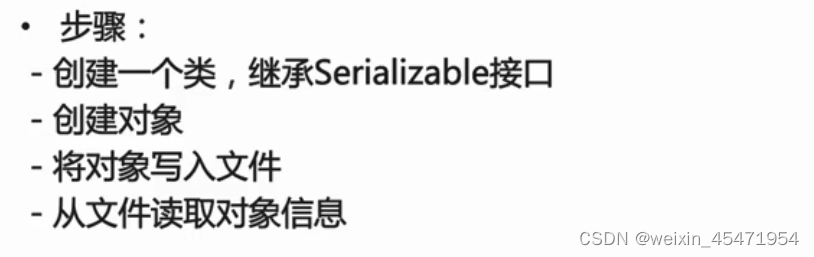
涉及的类
 1.创建goods类 实现接口
public class Goods implements Serializable {
private String bianhao;
private String name;
private double price;
public String getBianhao() {
return bianhao;
}
@Override
public String toString() {
return "Goods{" +
"bianhao='" + bianhao + '\'' +
", name='" + name + '\'' +
", price=" + price +
'}';
}
public void setBianhao(String bianhao) {
this.bianhao = bianhao;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public Goods(String bianhao, String name, double price) {
this.bianhao = bianhao;
this.name = name;
this.price = price;
}
}
2.创建测试类
public class GoodsTest {
public static void main(String[] args) {
Goods goods = new Goods("g500", "电脑", 3000);
try {
FileOutputStream fis = new FileOutputStream("mooc.txt");
ObjectOutputStream oos = new ObjectOutputStream(fis);
FileInputStream fos = new FileInputStream("mooc.txt");
ObjectInputStream ois = new ObjectInputStream(fos);
oos.writeObject(goods);
oos.flush();
Goods goods1 = (Goods) ois.readObject();
System.out.println(goods1);
fis.close();
oos.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
该程序的运行结果如下所示
Goods{bianhao='g500', name='电脑', price=3000.0}
|