高级流
字符流
- java将流按照读写单位划分为字节流与字符流。
java.io.InputStream 和OutputStream 是所有字节流的超类。- 而
java.io.Reader 和Writer 则是所有字符流的超类,它们和字节流的超类是平级关系。 Reader 和Writer 是两个抽象类,里面规定了所有字符流都必须具备的读写字符的相关方法。- 字符流最小读写单位为字符(char),但是底层实际还是读写字节,只是字符与字节的转换工作由字符流完成。
转换流
java.io.InputStreamReader 和OutputStreamWriter 它们是字符流非常常用的一对实现类同时也是一对高级流,实际开发中我们不直接操作它们,但是它们在流连接中是非常重要的一环。
使用转换输出流向文件中写入文本数据
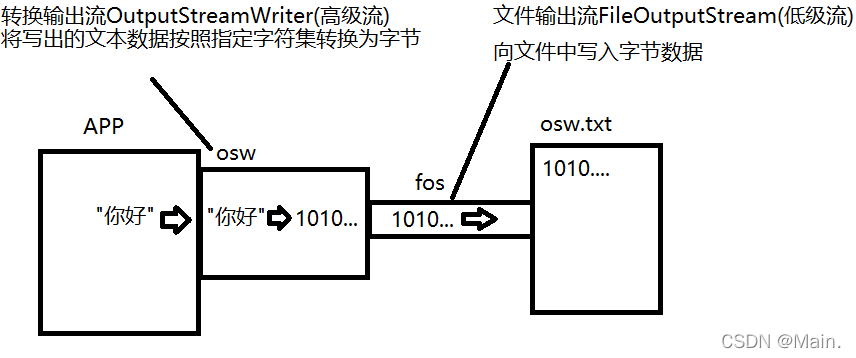
public class OSWDemo {
public static void main(String[] args) throws IOException {
FileOutputStream fos = new FileOutputStream("demo.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos, StandardCharsets.UTF_8);
osw.write("你好");
osw.write("HIEU");
System.out.println("写出完毕");
osw.close();
}
}
使用转换输入流读取文本文件
public class ISRDemo {
public static void main(String[] args) throws IOException {
FileInputStream fis = new FileInputStream("demo.txt");
InputStreamReader isr = new InputStreamReader(fis, StandardCharsets.UTF_8);
int d;
while ((d = isr.read()) != -1) {
System.out.print((char) d);
}
}
}
转换流的意义
实际开发中我们还有功能更好用的字符高级流,但是其他的字符高级流都有一个共通点:不能直接连接在字节流上,而实际操作设备的流都是低级流,同时也都是字节流,因此不能直接在流连接中串联起来。转换流是一对可以连接在字节流上的字符流,其他的高级字符流可以连接在转换流上,在流连接中起到"转换器"的作用(负责字符与字节的实际转换)。
缓冲字符流
java.io.BufferedWriter 和BufferedReader 缓冲字符流内部也有一个缓冲区,读写文本数据以块读写形式加快效率。并且缓冲流有一个特别的功能:可以按行读写文本数据。
缓冲字符输出流:java.io.PrintWriter
java.io.PrintWriter 具有自动行刷新的缓冲字符输出流,实际开发中更常用。它内部总是会自动连接BufferedWriter 作为块写加速使用。 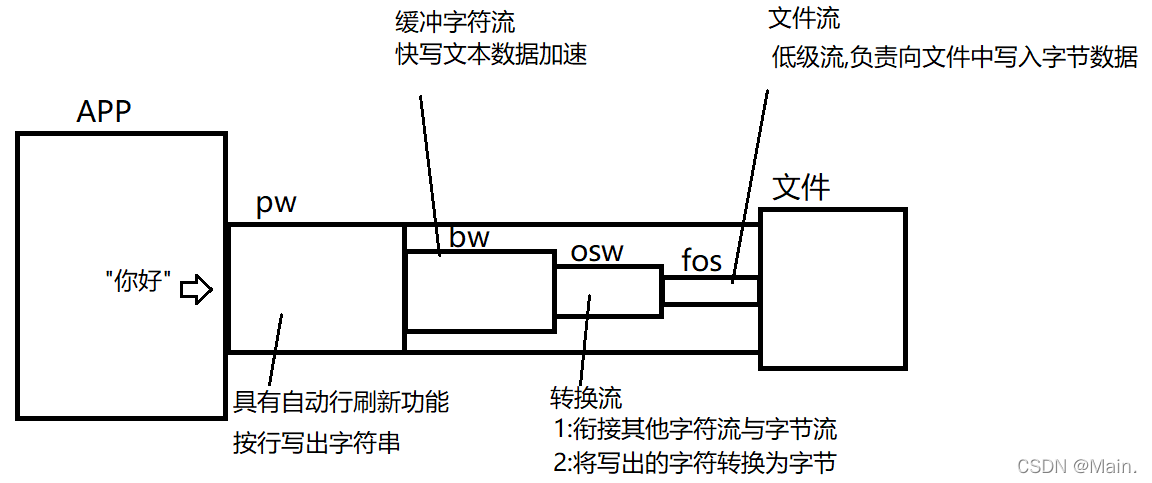
public class PWDemo1 {
public static void main(String[] args)
throws FileNotFoundException, UnsupportedEncodingException {
PrintWriter pw = new PrintWriter("demo.txt", "UTF-8");
pw.println("hi");
pw.println("你好");
System.out.println("写出完毕");
pw.close();
}
}
在流链接中使用pw
public class PWDemo2 {
public static void main(String[] args) throws IOException {
FileOutputStream fos = new FileOutputStream("demo.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos, StandardCharsets.UTF_8);
BufferedWriter bw = new BufferedWriter(osw);
PrintWriter pw = new PrintWriter(bw);
pw.println("你好");
pw.println("再见");
System.out.println("写出完毕");
pw.close();
}
}
PrintWriter的自动行刷新功能
如果实例化PW时第一个参数传入的是一个流,则此时可以再传入一个boolean型的参数,此值为true时就打开了自动行刷新功能。 即: 每当我们用PW的println方法写出一行字符串后会自动flush。
public class PWDemo3 {
public static void main(String[] args) throws IOException {
PrintWriter pw = new PrintWriter(
new BufferedWriter(
new OutputStreamWriter(
new FileOutputStream("demo.txt")
)
), true);
Scanner scanner = new Scanner(System.in);
System.out.println("请输入内容,单独输入exit停止");
while (true) {
String line = scanner.nextLine();
if ("exit".equals(line)) {
break;
}
pw.println(line);
}
System.out.println("再见!");
pw.close();
}
}
缓冲字符输入流:java.io.BufferedReader
是一个高级的字符流,特点是块读文本数据,并且可以按行读取字符串。
public class BRDemo {
public static void main(String[] args) throws IOException {
FileInputStream fis =
new FileInputStream("demo.txt");
InputStreamReader isr = new InputStreamReader(fis, StandardCharsets.UTF_8);
BufferedReader br = new BufferedReader(isr);
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
br.close();
}
}
Java IO总结
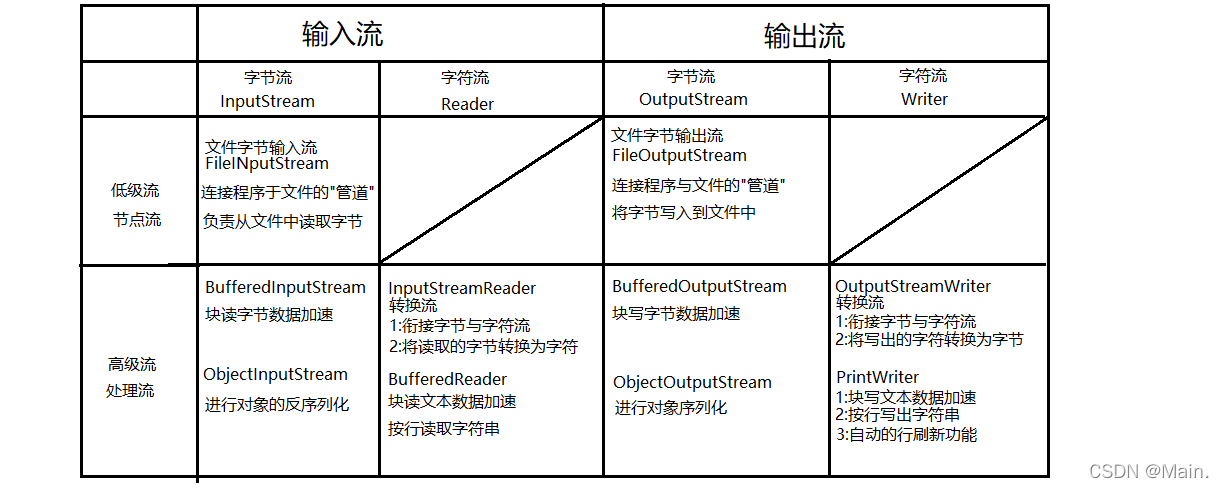
|