深度拷贝(已封装为一个泛型通用方法复制即用):
public static List<T> DeepCopyList<T>(IList<T> list1 , out List<T> list2)
{
MemoryStream ms = new MemoryStream();
BinaryFormatter bf = new BinaryFormatter();
bf.Serialize(ms, list1);
ms.Position = 0;
list2 = (List<T>)bf.Deserialize(ms);
return list2;
}
使用到的类(类需要标记可序列化特性):
[Serializable]
public class Student
{
public int Id { get; set; }
public int Age { get; set; }
}
测试方法:
static void Main(string[] args)
{
var list1 = new List<Student>();
Student stu1 = new Student();
stu1.Id = 1;
stu1.Age = 12;
list1.Add(stu1);
Student stu2 = new Student();
stu2.Id = 2;
stu2.Age = 21;
list1.Add(stu2);
Student stu3 = new Student();
stu3.Id = 3;
stu3.Age = 23;
list1.Add(stu3);
var list2 = new List<Student>();
list2 = DeepCopyList<Student>(list1,out list2).Where(s => s.Id == 1).ToList();
var ss = list2.Select(s => s.Age = 33).ToList();
}
结果: 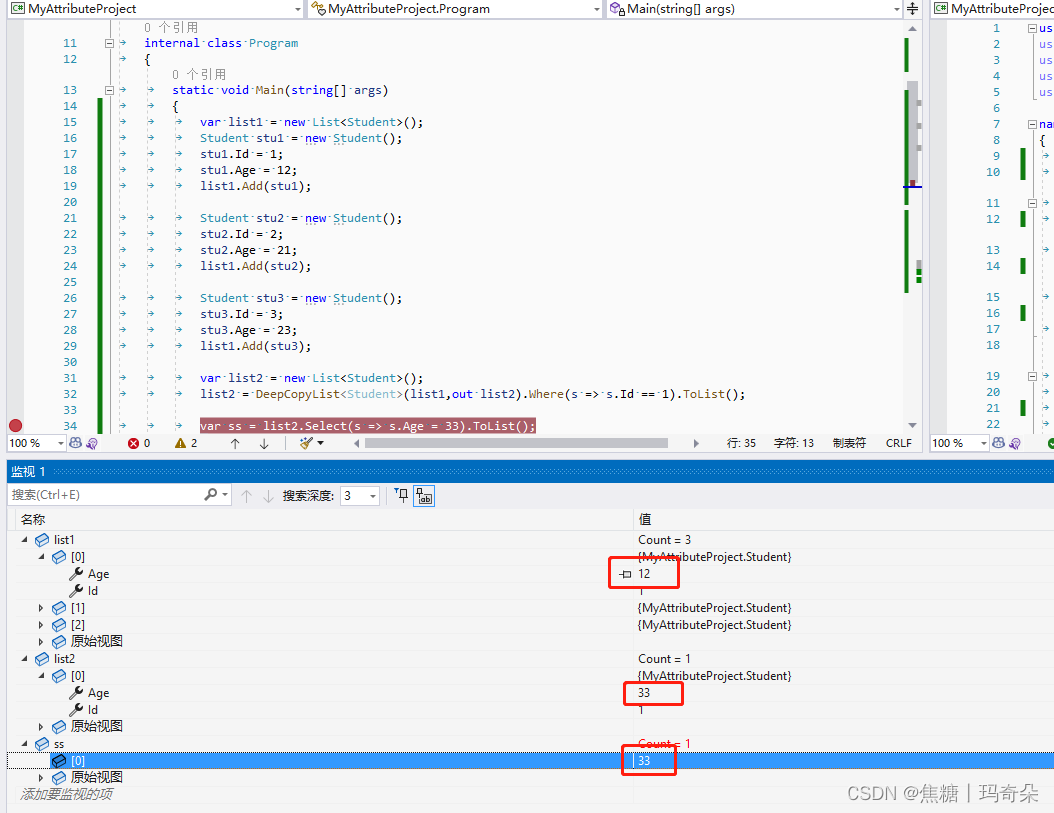
转json
public static List<T> Clone<T>(this List<T> list) where T : new()
{
var str = JsonConvert.SerializeObject(list);
return JsonConvert.DeserializeObject<List<T>>(str);
}
使用到的类(类需要标记可序列化特性):
[Serializable]
public class Student
{
public int Id { get; set; }
public int Age { get; set; }
}
测试方法:
static void Main(string[] args)
{
var list1 = new List<Student>();
Student stu1 = new Student();
stu1.Id = 1;
stu1.Age = 12;
list1.Add(stu1);
Student stu2 = new Student();
stu2.Id = 2;
stu2.Age = 21;
list1.Add(stu2);
Student stu3 = new Student();
stu3.Id = 3;
stu3.Age = 23;
list1.Add(stu3);
var list2 = new List<Student>();
list2 = Clone<Student>(list1).Where(s => s.Id == 1).ToList();
var ss = list2.Select(s => s.Age = 33).ToList();
}
结果: 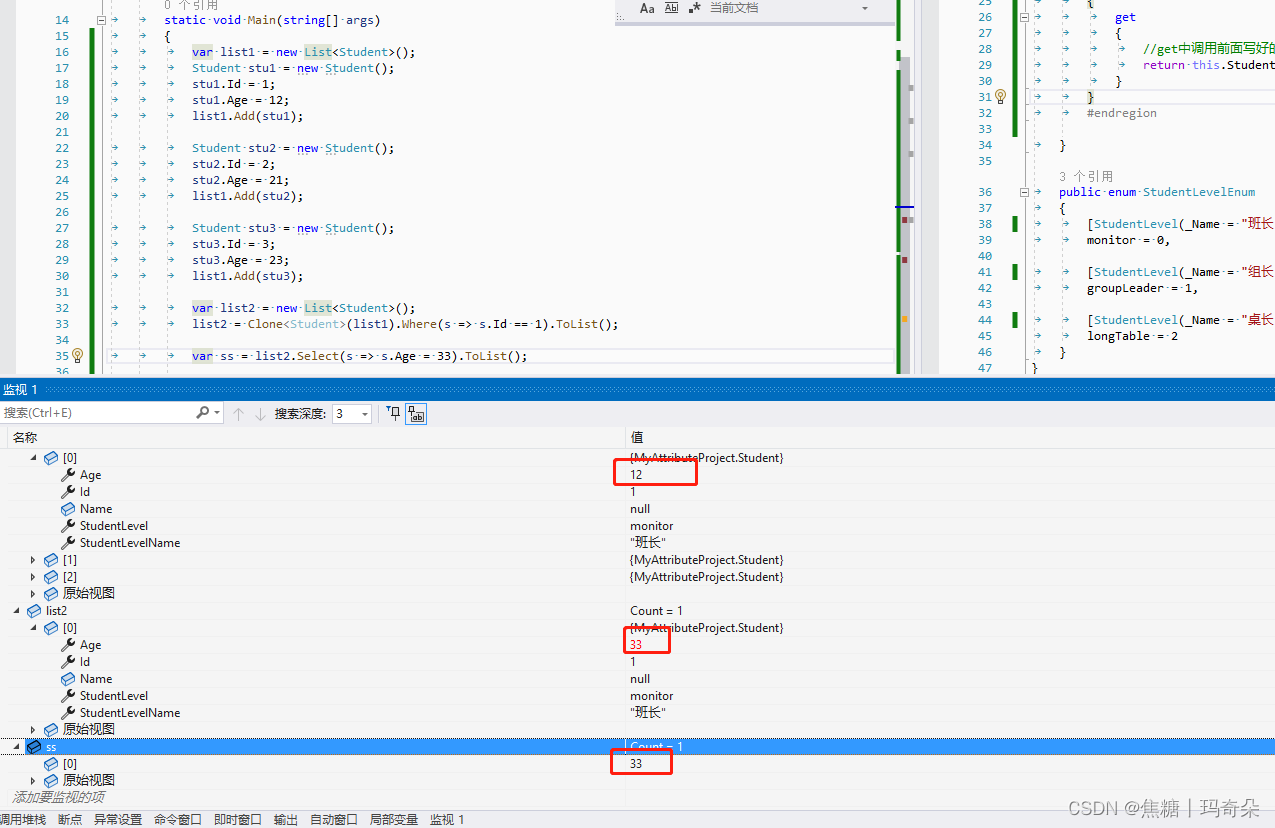
|