java异常
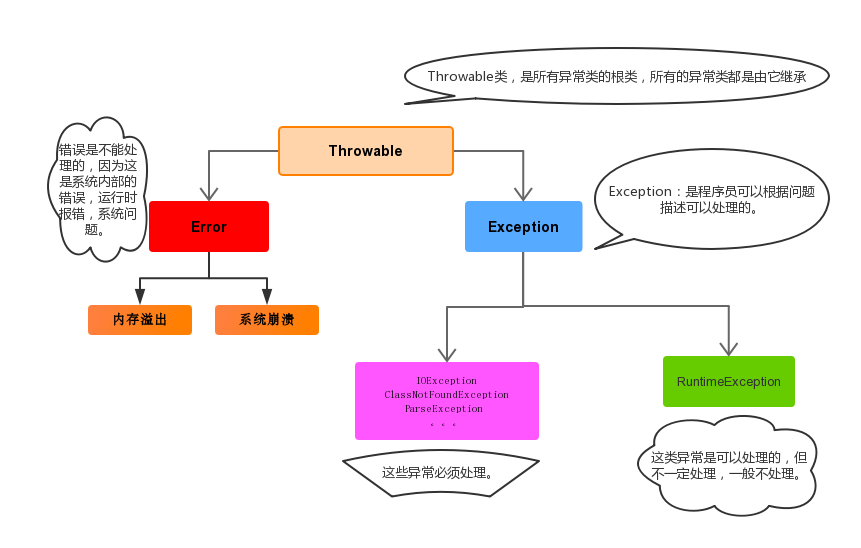
小结
RuntimeException和Exception有什么区别:
Exception属于编译时异常,编译器在编译时会检测该异常是否异常的处理方案 ,如果没有处理方案,编译不能通过。
RuntimeException属于运行时异常,编译器不会检测该异常是否有异常的处理方案,不需要声明。
说明:在Exception的所有子类异常中,只有RuntimeException不是编译异常,是运行时异常,其他子类都是编译异常。
编译时期异常
一定要用try catch或者tthrows处理,否则程序报错,无法生成字节码文件
checked异常。在编译时期,就会检查,如果没有处理异常,则编译失败。(如日期格式化异常) 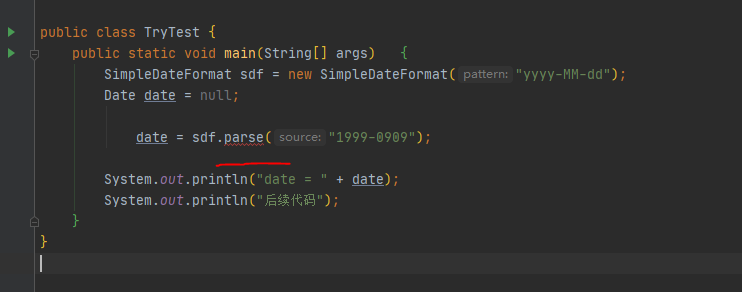
2种解决方式
throws(不推荐,没法运行后续代码)
package cn.itcast.test;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class TryTest {
public static void main(String[] args) throws ParseException {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Date date = null;
date = sdf.parse("1999-0909");
System.out.println("date = " + date);
System.out.println("后续代码");
}
}
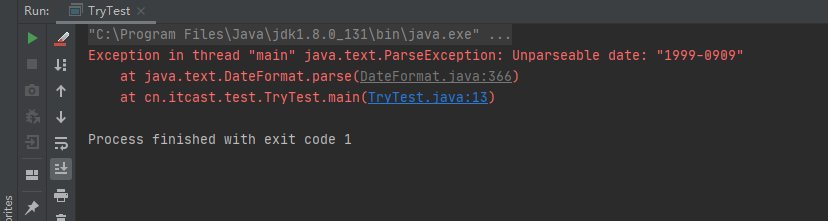
try catch(推荐,可以运行后续代码)
package cn.itcast.test;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class TryTest {
public static void main(String[] args) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Date date = null;
try {
date = sdf.parse("1999-0909");
} catch (ParseException e) {
e.printStackTrace();
}
System.out.println("date = " + date);
System.out.println("后续代码");
}
}
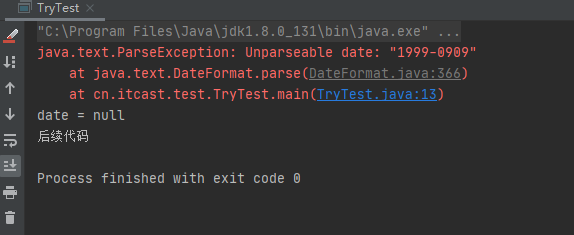
运行时期异常
不一定要用try catch或者throws处理,因为程序不报错
runtime异常。在运行时期,检查异常.在编译时期,运行异常不会让编译器检测(不报错)。(如数学异常) 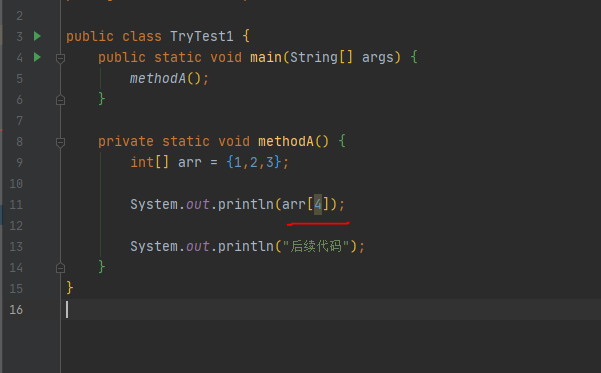
运行时期时异常:ArrayIndexOutOfBoundsException,NullPointerException
运行时期异常(RuntimeException)在方法上不用throws抛出
当 throw new RuntimeException的时候,不用throws
package cn.itcast.test;
public class TryTest {
public static void main(String[] args) {
int[] arr = {2,4,52,2};
int index = 4;
int element = getElement(arr, index);
System.out.println(element);
System.out.println("over");
}
public static int getElement(int[] arr,int index){
if(index<0 || index>arr.length-1){
throw new ArrayIndexOutOfBoundsException("哥们,角标越界了~~~");
}
int element = arr[index];
return element;
}
}
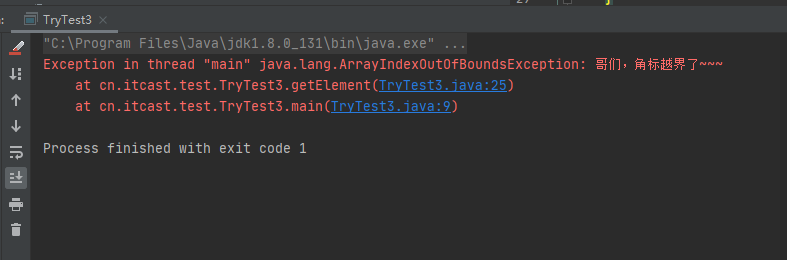
|