人生格言
未来犹存,人生当前
阿里云 oss
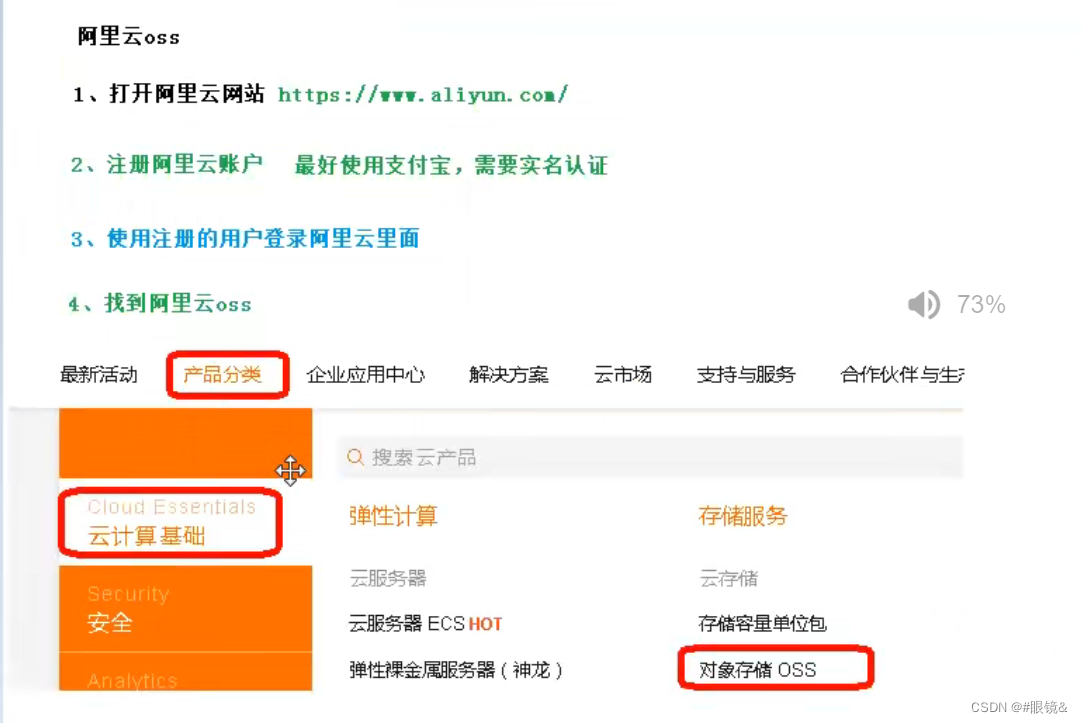
开通对象存储 oss
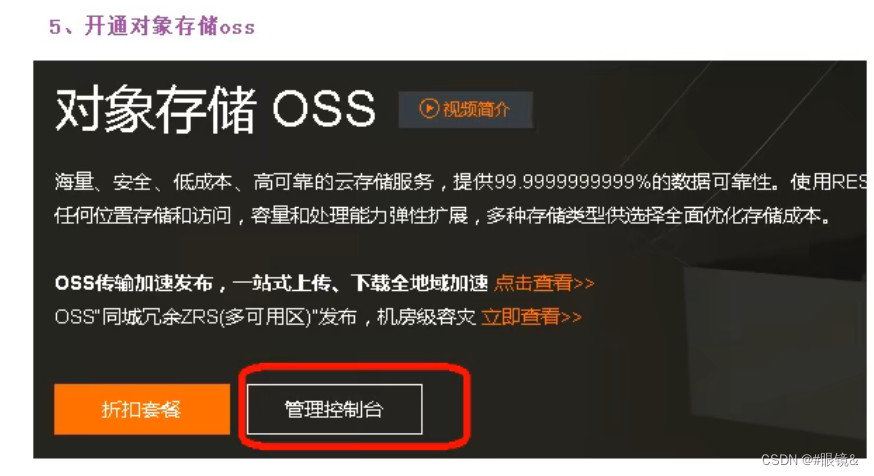
阿里运 oss管理控制台的使用
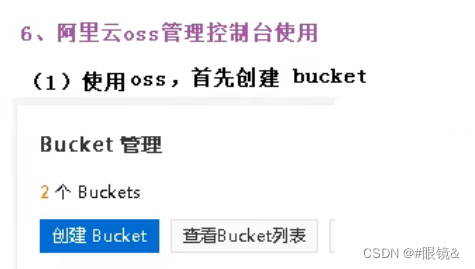 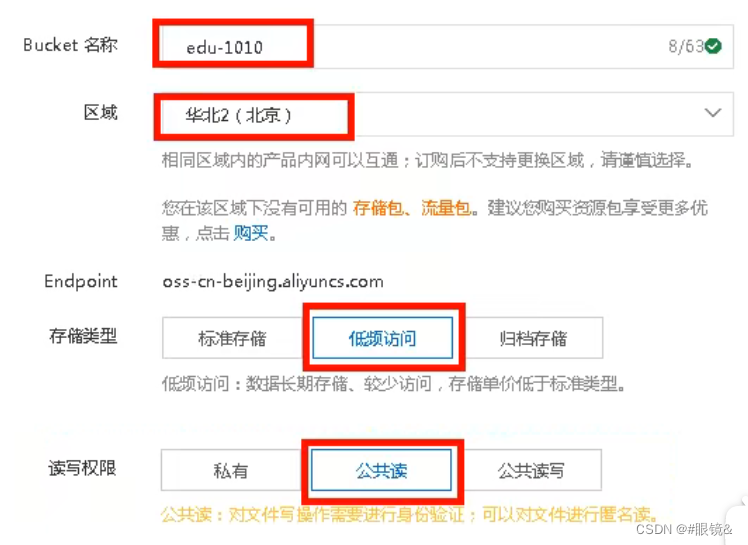 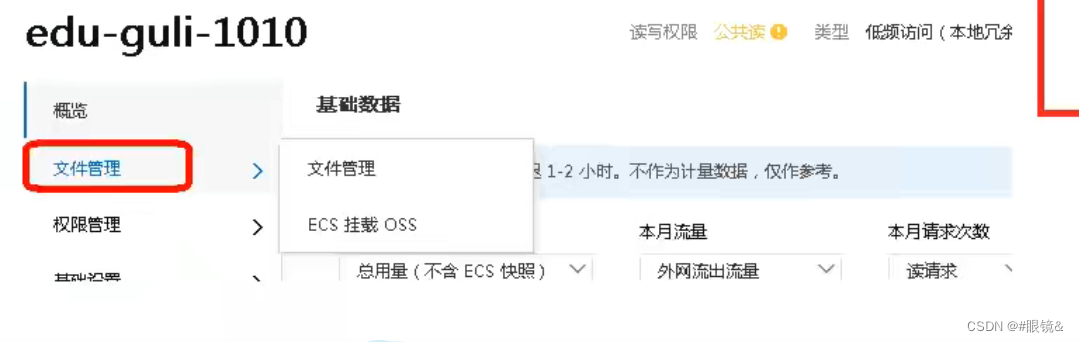
java 代码操作阿里云 oss
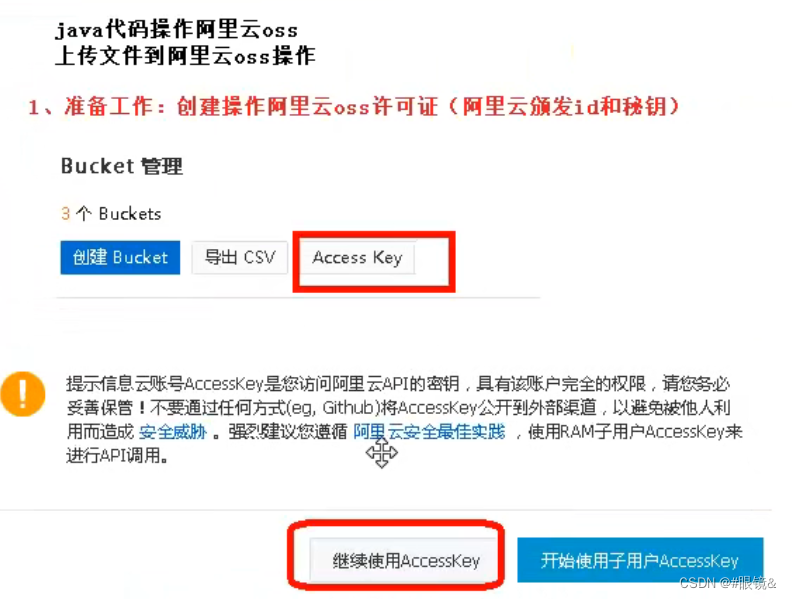 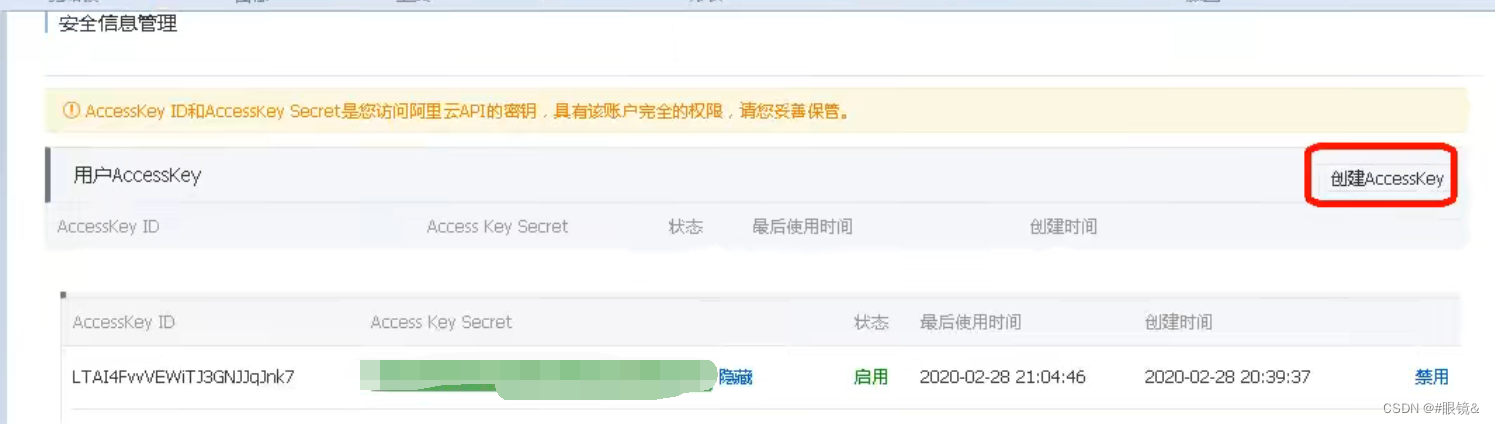 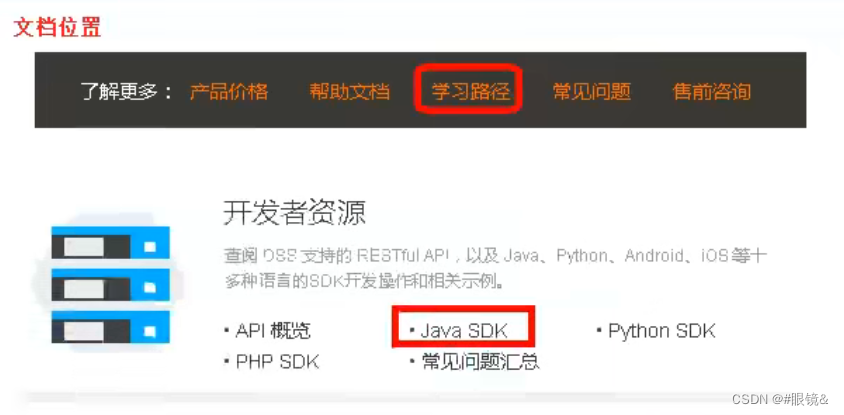 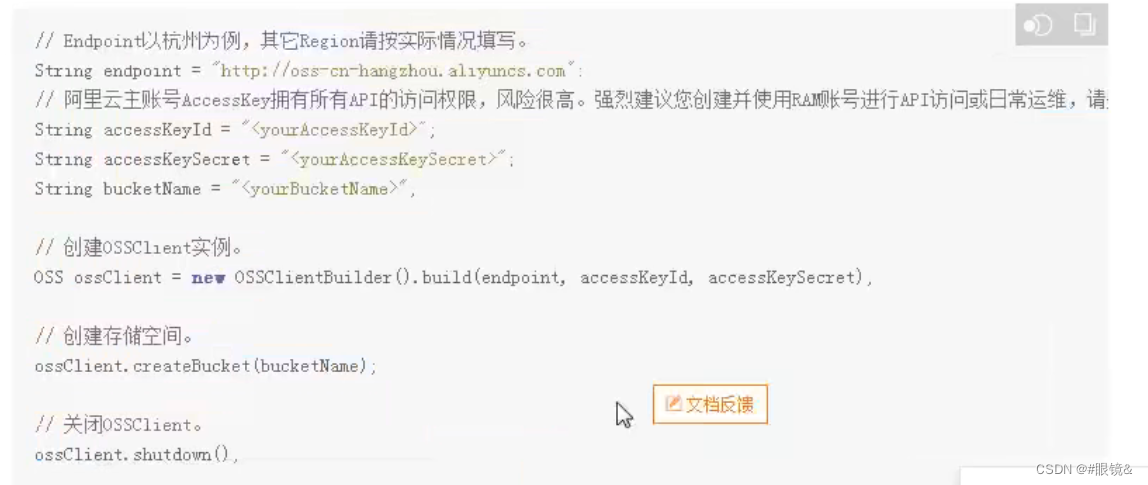
service_oss模块
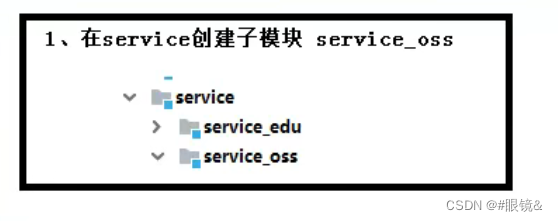 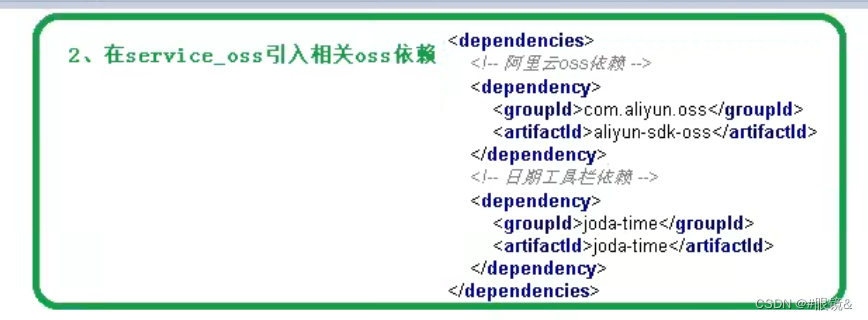 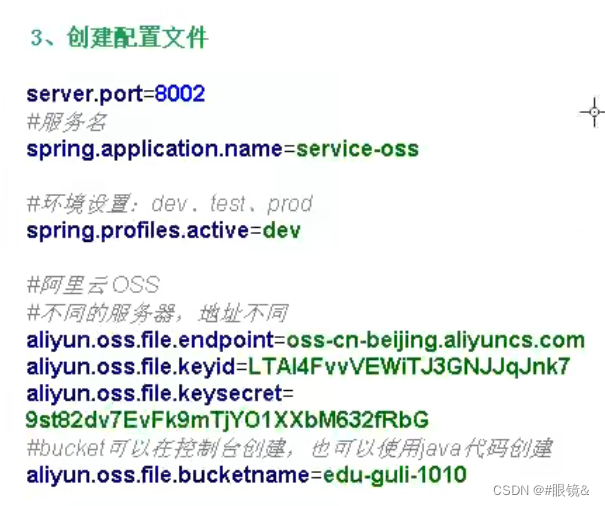 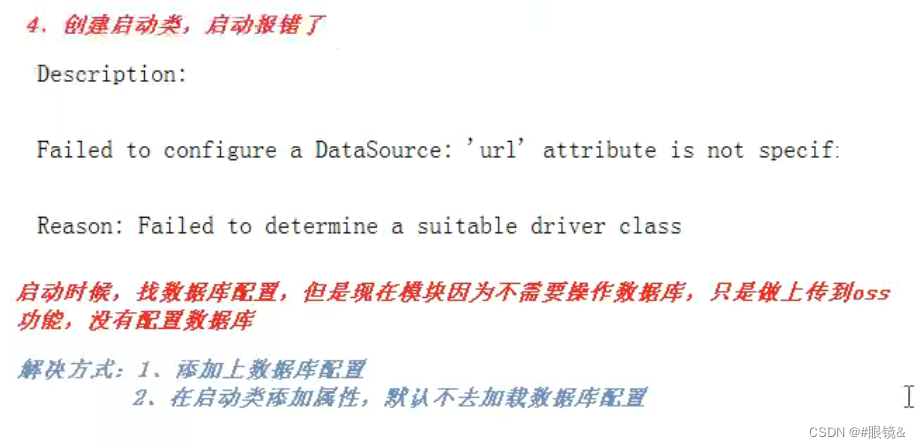 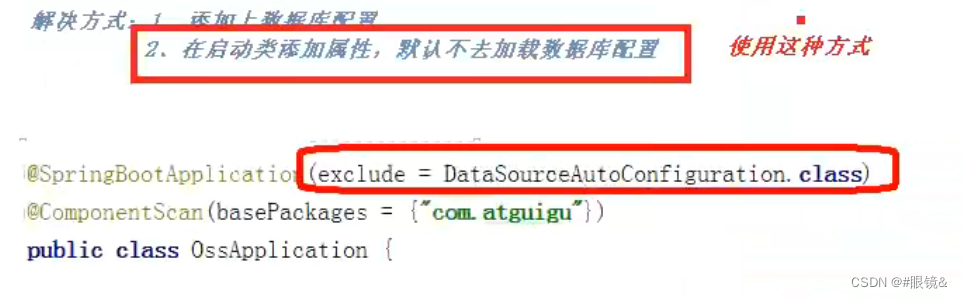
实现二进制文件的上传
在阿里云开通 对象存储服务 oss
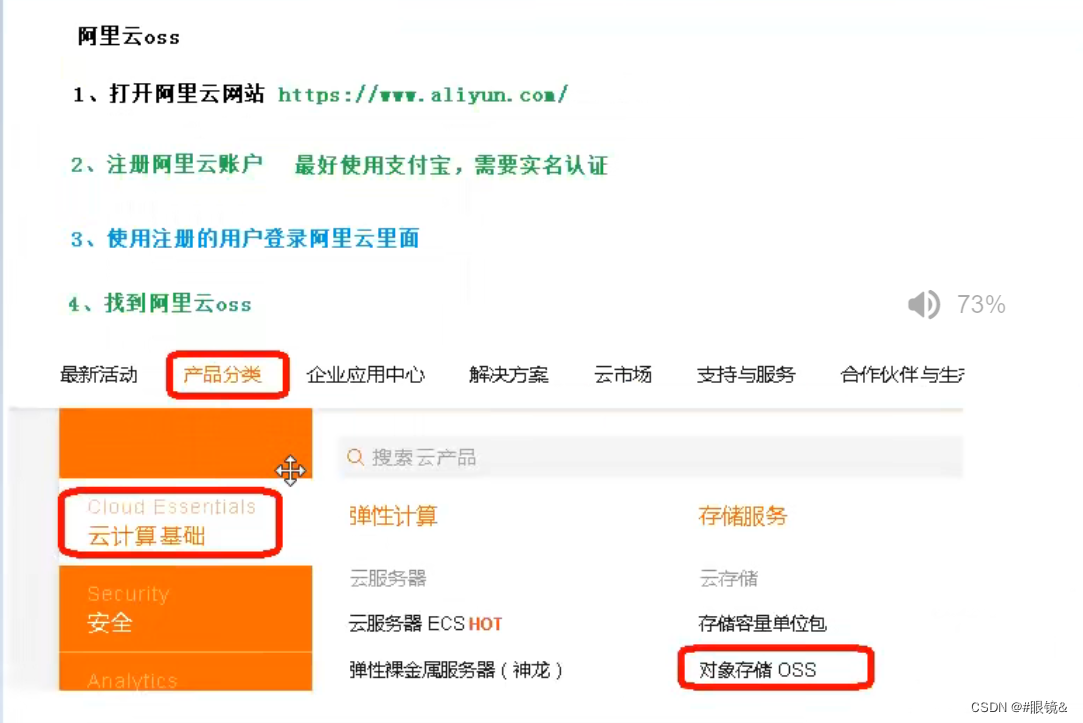 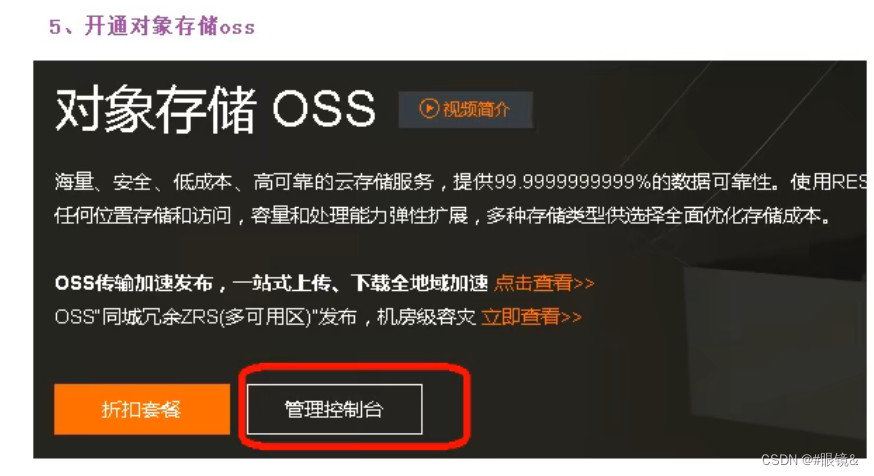
创建 bucket
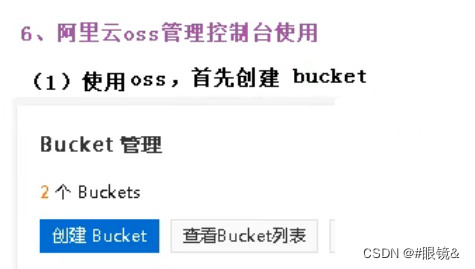
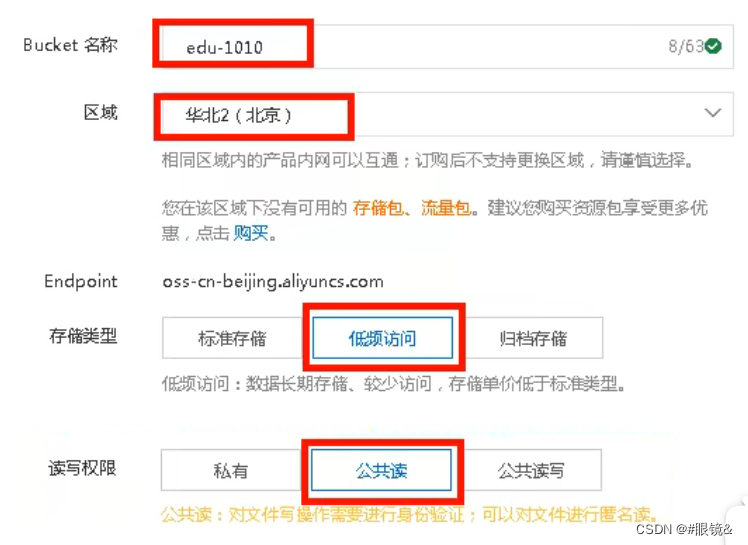
创建 许可证
点击用户头像 
在pom中引入依赖
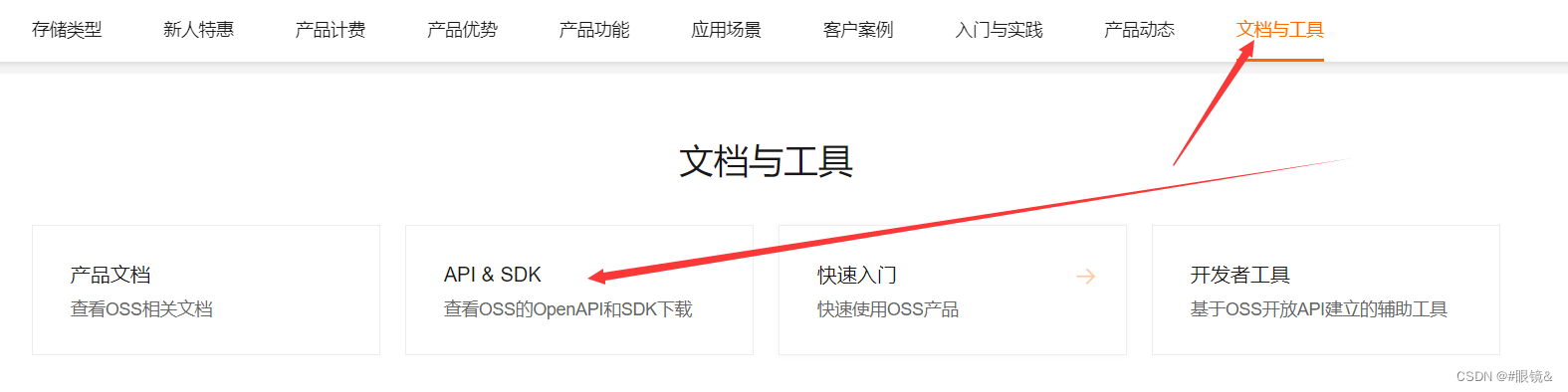 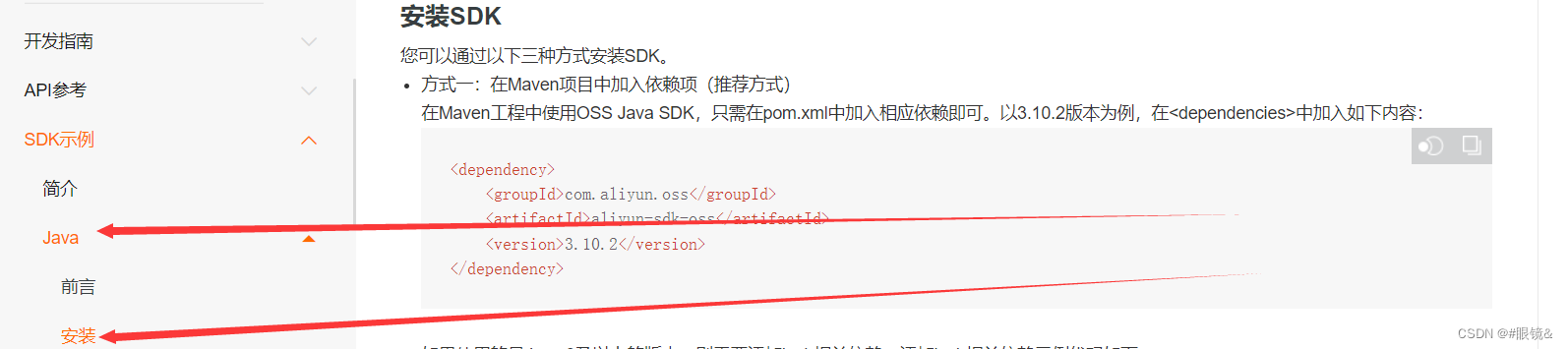
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
<version>3.10.2</version>
</dependency>
<!-- 阿里云oss依赖 -->
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
</dependency>
<!--日期工具栏依赖-->
<dependency>
<groupId>joda-time</groupId>
<artifactId>joda-time</artifactId>
</dependency>
编写配置文件
#服务端口
server.port=8002
#服务名
spring.application.name=service-oss
#环境设置:dev、test、prod
spring.profiles.active=dev
#阿里云 OSS
#不同的服务器,地址不同
aliyun.oss.file.endpoint=
aliyun.oss.file.keyid=
aliyun.oss.file.keysecret=
#bucket可以在控制台创建,也可以使用java代码创建
aliyun.oss.file.bucketname=
String endpoint = "yourEndpoint";
String accessKeyId = "yourAccessKeyId";
String accessKeySecret = "yourAccessKeySecret";
编写controller
package com.gsx.ossservice.controller;
import com.gsx.commonUtils.Result;
import com.gsx.ossservice.service.OssService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
@RestController
@RequestMapping("/eduoss/fileoss")
@CrossOrigin
public class OssController {
@Autowired
private OssService ossService;
@PostMapping
public Result uploadOssFile(MultipartFile file){
String url =ossService.uploadFileAvatar(file);
return Result.success().data("url",url);
}
}
编写service
package com.gsx.ossservice.service;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
public interface OssService {
String uploadFileAvatar(MultipartFile file);
}
编写serviceimpl
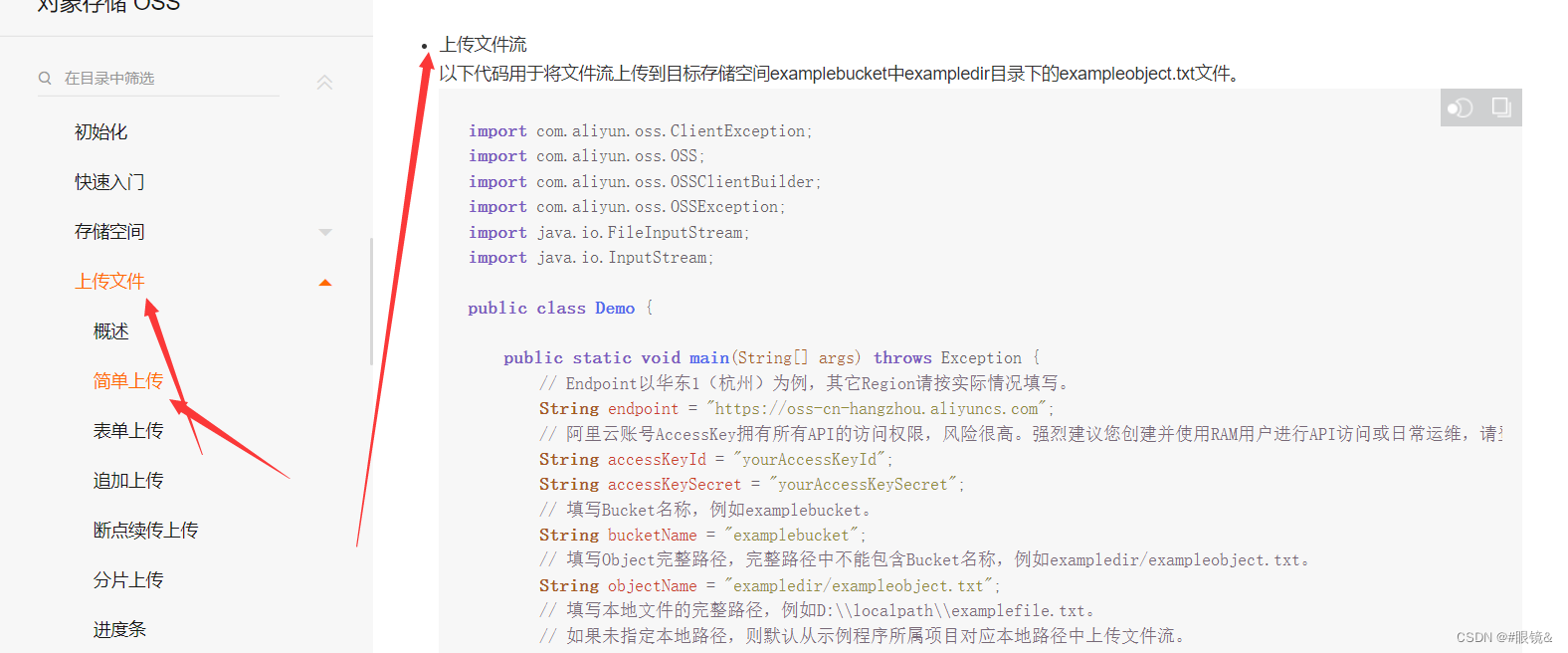
package com.gsx.ossservice.utils;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class ConstantPropertiesUtils implements InitializingBean {
@Value("${aliyun.oss.file.endpoint}")
private String endpoint;
@Value("${aliyun.oss.file.keyid}")
private String keyid;
@Value("${aliyun.oss.file.keysecret}")
private String keysecret;
@Value("${aliyun.oss.file.bucketname}")
private String bucketname;
public static String END_POINT;
public static String KEY_ID;
public static String KEY_SECRET;
public static String BUCKET_NAME;
@Override
public void afterPropertiesSet() throws Exception {
KEY_ID=this.keyid;
KEY_SECRET=this.keysecret;
END_POINT=this.endpoint;
BUCKET_NAME=this.bucketname;
}
}
package com.gsx.ossservice.service.impl;
import com.aliyun.oss.OSS;
import com.aliyun.oss.OSSClientBuilder;
import com.gsx.ossservice.service.OssService;
import com.gsx.ossservice.utils.ConstantPropertiesUtils;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.io.InputStream;
@Service
public class OssServiceImpl implements OssService {
@Override
public String uploadFileAvatar(MultipartFile file) {
String endpoint = ConstantPropertiesUtils.END_POINT;
String accessKeyId = ConstantPropertiesUtils.KEY_ID;
String accessKeySecret = ConstantPropertiesUtils.KEY_SECRET;
String bucketName = ConstantPropertiesUtils.BUCKET_NAME;
InputStream inputStream = null;
try {
OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
inputStream = file.getInputStream();
String fileName = file.getOriginalFilename();
ossClient.putObject(bucketName, fileName, inputStream);
ossClient.shutdown();
String url = "http://"+bucketName+"."+endpoint+"/"+fileName ;
return url;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
}
官方参考
import com.aliyun.oss.ClientException;
import com.aliyun.oss.OSS;
import com.aliyun.oss.OSSClientBuilder;
import com.aliyun.oss.OSSException;
import java.io.FileInputStream;
import java.io.InputStream;
public class Demo {
public static void main(String[] args) throws Exception {
String endpoint = "https://oss-cn-hangzhou.aliyuncs.com";
String accessKeyId = "yourAccessKeyId";
String accessKeySecret = "yourAccessKeySecret";
String bucketName = "examplebucket";
String objectName = "exampledir/exampleobject.txt";
String filePath= "D:\\localpath\\examplefile.txt";
OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
try {
InputStream inputStream = new FileInputStream(filePath);
ossClient.putObject(bucketName, objectName, inputStream);
} catch (OSSException oe) {
System.out.println("Caught an OSSException, which means your request made it to OSS, "
+ "but was rejected with an error response for some reason.");
System.out.println("Error Message:" + oe.getErrorMessage());
System.out.println("Error Code:" + oe.getErrorCode());
System.out.println("Request ID:" + oe.getRequestId());
System.out.println("Host ID:" + oe.getHostId());
} catch (ClientException ce) {
System.out.println("Caught an ClientException, which means the client encountered "
+ "a serious internal problem while trying to communicate with OSS, "
+ "such as not being able to access the network.");
System.out.println("Error Message:" + ce.getMessage());
} finally {
if (ossClient != null) {
ossClient.shutdown();
}
}
}
}
测试
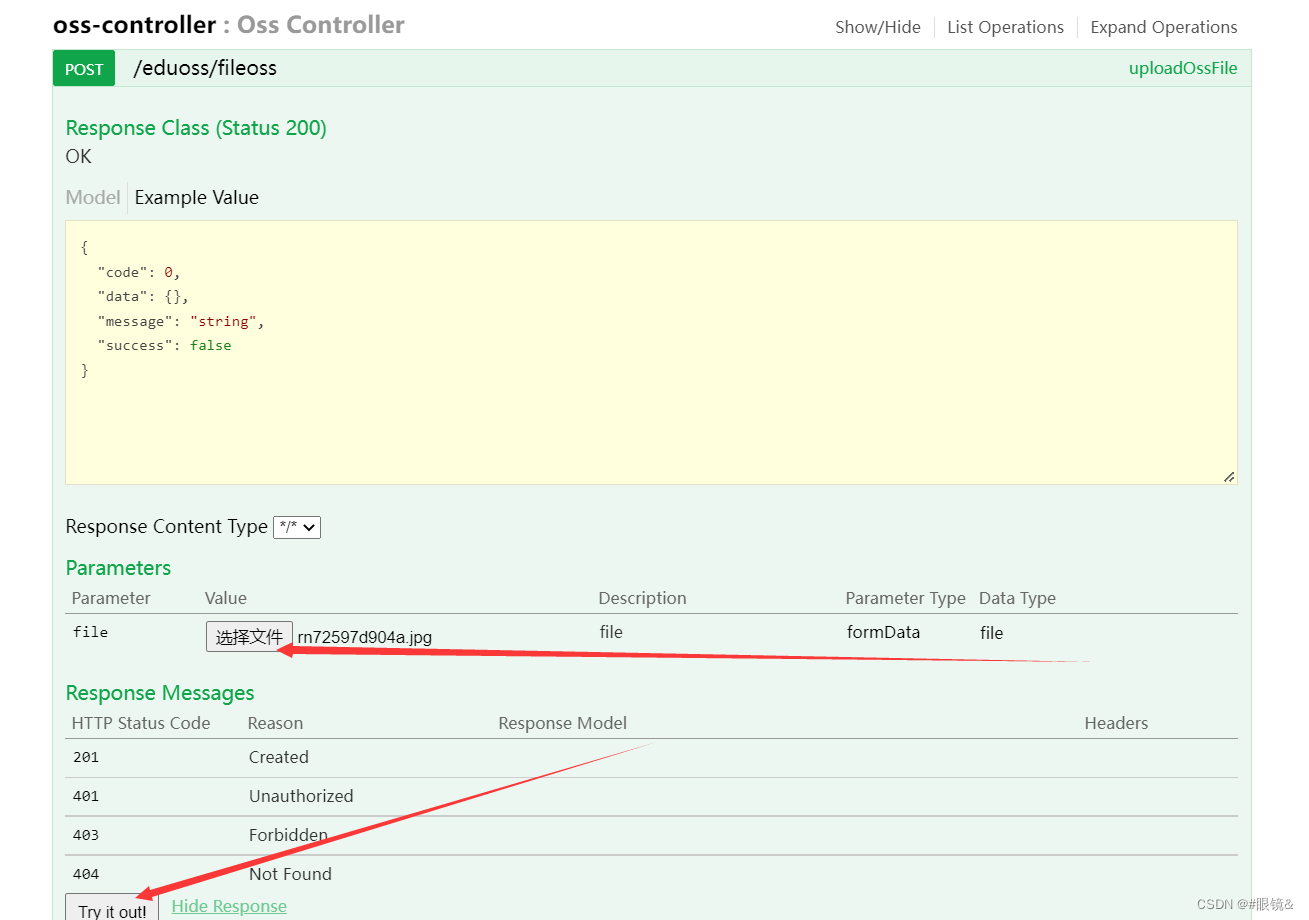
测试结果
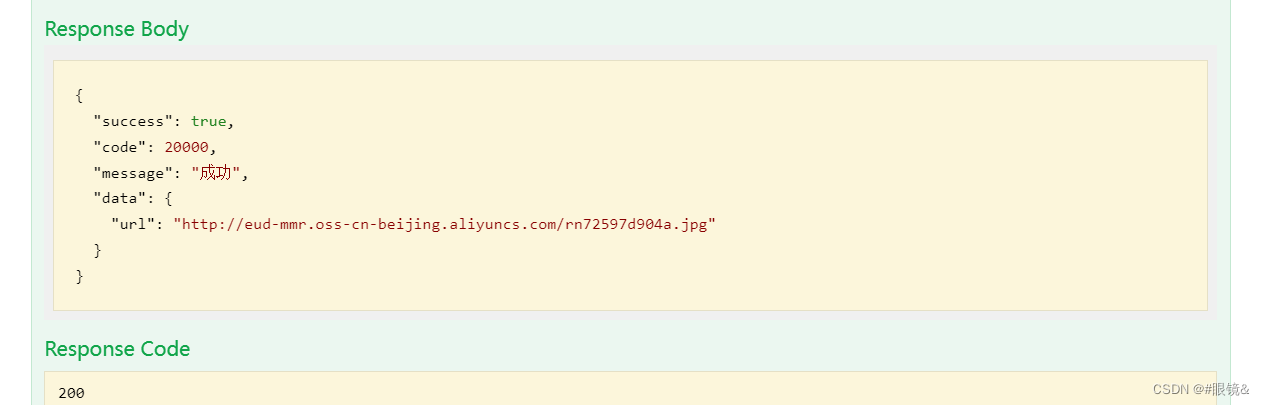  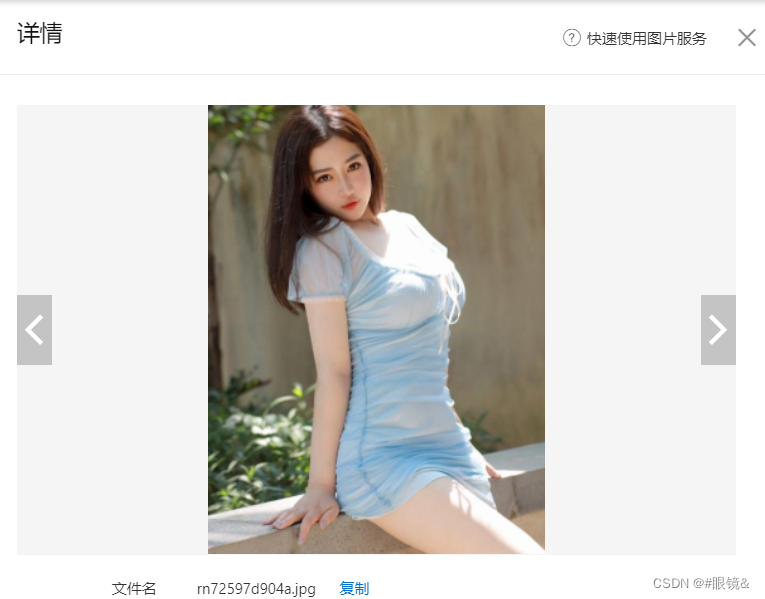
解决以下问题
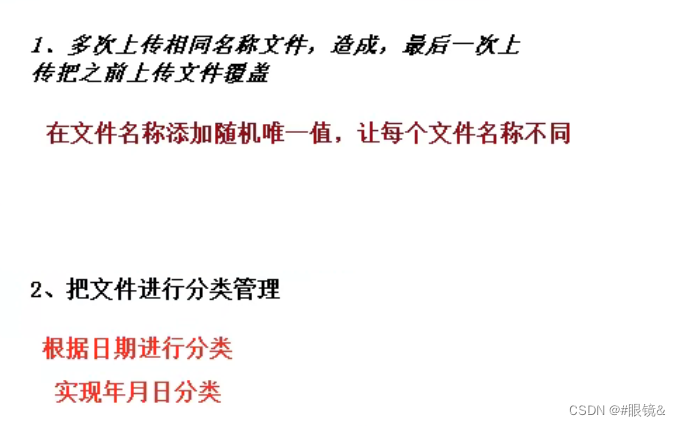
产生不同的文件名
String fileName = file.getOriginalFilename();
String uuid = UUID.randomUUID().toString().replaceAll("-","");
fileName=uuid+fileName;
实现文件的分类存储
//把文件按照日期进行分类
//获取当前的日期 使用工具类
String datePath= new DateTime().toString("yyyy/MM/dd");
//拼接 2022/5/4/filename
fileName=datePath+"/"+fileName;
|