需求
系统是多用户使用。以公司为主体,公司分为管理员和运营人员。
系统管理员可以控制系统菜单,公司管理员可控制系统菜单的显示与否。
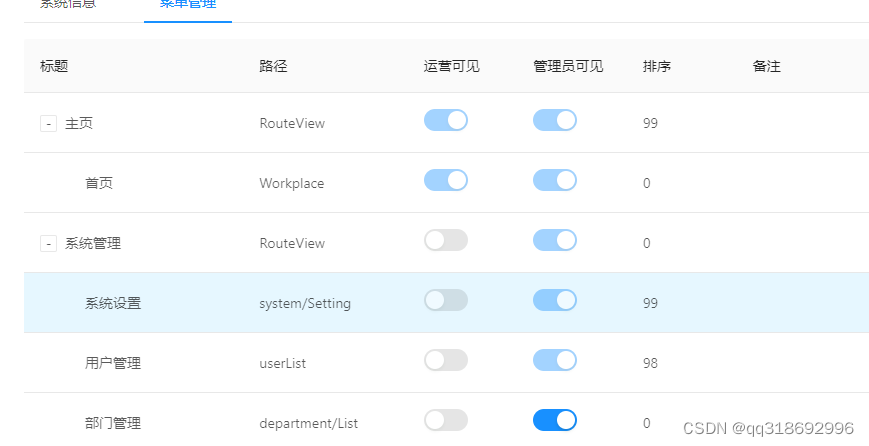
user1=管理员
user2=运营人员
建表,储存菜单
CREATE TABLE `app_nav` (
`id` int NOT NULL AUTO_INCREMENT,
`title` varchar(50) CHARACTER SET utf8 COLLATE utf8_general_ci DEFAULT NULL,
`parentId` int DEFAULT '0',
`icon` varchar(50) DEFAULT NULL,
`name` varchar(50) CHARACTER SET utf8 COLLATE utf8_general_ci DEFAULT NULL,
`show` tinyint(1) DEFAULT '0',
`redirect` varchar(50) DEFAULT NULL,
`component` varchar(50) DEFAULT NULL,
`sort` int DEFAULT '0' COMMENT '排序',
`state` tinyint(1) DEFAULT '0',
`is_edit` tinyint(1) DEFAULT '0' COMMENT '是否可编辑',
`is_admin` tinyint(1) DEFAULT '0' COMMENT '是否是管理员功能',
`is_user2` tinyint(1) DEFAULT '0' COMMENT '运营可见',
`is_user1` tinyint(1) DEFAULT '0' COMMENT '管理员可见',
`desc` varchar(50) DEFAULT NULL COMMENT '备注',
`type` tinyint DEFAULT '0' COMMENT '0是系统,1定制',
`store_id` int DEFAULT '0' COMMENT '0是系统,非0是自定义',
`dtime` int DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=44 DEFAULT CHARSET=utf8;
建表,储存用户所对应的菜单
CREATE TABLE `app_nav_item` (
`id` int NOT NULL AUTO_INCREMENT,
`navId` int DEFAULT NULL,
`store_id` int DEFAULT NULL,
`ctime` int DEFAULT NULL,
`is_user1` tinyint(1) DEFAULT '0',
`is_user2` tinyint(1) DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8;
思路:判断子表对应的菜单是否存在,存在使用子表的状态的。
// 公司管理员获取菜单
$data = NavModel::alias('n')
->join(["(select * from app_nav_item where store_id = {$this->store_id})"=>'nt'],'nt.navId=n.id','left')
->order('n.parentId asc,n.sort desc,n.id asc')
->field('n.*,ifnull(nt.is_user1,n.is_user1) is_user1,ifnull(nt.is_user2,n.is_user2) is_user2')//如果子表为空,则使用系统显示状态。如果不空则使用用户自定义的显示状态
->where("n.store_id = 0 or n.store_id = {$this->store_id}")// 用户可以自定义菜单
->where('n.state = 1')
->select()->toArray();
// 公司管理员编辑菜单
public function editValue($id=0,$field='',$value='')
{
if (empty($id)) {
$this->error("id不能空");
}
$info = NavModel::find($id);
if (empty($info)) {
$this->error("菜单id已不存在");
}
$ItemInfo = NavItemModel::where(['navId' => $id, 'store_id' => $this->store_id])->find();
switch ($field) {
/* case 'is_admin':
$value = !empty($value) ? 1 : 0;
break;*/
case 'is_user1':
$value = !empty($value) ? 1 : 0;
break;
case 'is_user2':
$value = !empty($value) ? 1 : 0;
break;
/* case 'is_edit':
$value = !empty($value) ? 1 : 0;
break;*/
default:
$this->error("field参数有误");
}
if (empty($ItemInfo)) {
// 新记录
$sql = [
'store_id' => $this->store_id,
'navId' => $id,
'is_user1' => $info['is_user1'],
'is_user2' => $info['is_user2'],
'ctime' => time()
];
$sql[$field] = $value;
NavItemModel::insert($sql);
}else{
// 编辑
$ItemInfo = $ItemInfo->toArray();
$info = $info->toArray();
$ItemInfo[$field] = $value;
if ($info['is_user1'] === $ItemInfo['is_user1'] && $info['is_user2'] === $ItemInfo['is_user2']) {
// 跟系统菜单状态显示一致就删除记录
NavItemModel::where('id', $ItemInfo['id'])->delete();
}else{
$sql[$field] = $value;
NavItemModel::where('id', $ItemInfo['id'])->update($sql);
}
}
//NavModel::where('id', $id)->update($sql);
$this->success();
}
// 登录用户获取菜单
$data = NavModel::alias('n')
->join(["(select * from app_nav_item where store_id = {$this->store_id})" => 'nt'], 'nt.navId=n.id', 'left')
->order('n.parentId asc,n.sort desc,n.id asc')
->field('n.*')
->cache(60)
->where("n.store_id = 0 or n.store_id = {$this->store_id}")
->where('n.state = 1')
->where("if(nt.id is null,n.is_user{$this->userType} = 1, nt.is_user{$this->userType}=1)")//如果子表不存在数据,则使用系统菜单默认状态
->select()->toArray();
|