1.显式接口的应用 2.案例:停车场收费管理平台 3.结构(struct)与类的比较 4.运算符重载 5.索引器
显示接口:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
interface IChinese
{
void Speak( );
}
interface IUSA
{
void Speak( );
}
}
继承接口类:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
class Person: IChinese,IUSA
{
public void Speak( )
{
Console.WriteLine( "#@$%*.....");
}
void IChinese.Speak( )
{
Console.WriteLine( "你好,世界...." );
}
void IUSA.Speak( )
{
Console.WriteLine( "Hello,world...." );
}
}
}
主程序调用:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections;
namespace ConsoleApplication1
{
class Program
{
static void Main( string[ ] args )
{
Person p = new Person( );
p.Speak( );
IChinese ic = new Person( );
ic.Speak( );
IUSA iu = new Person( );
iu.Speak( );
}
}
}
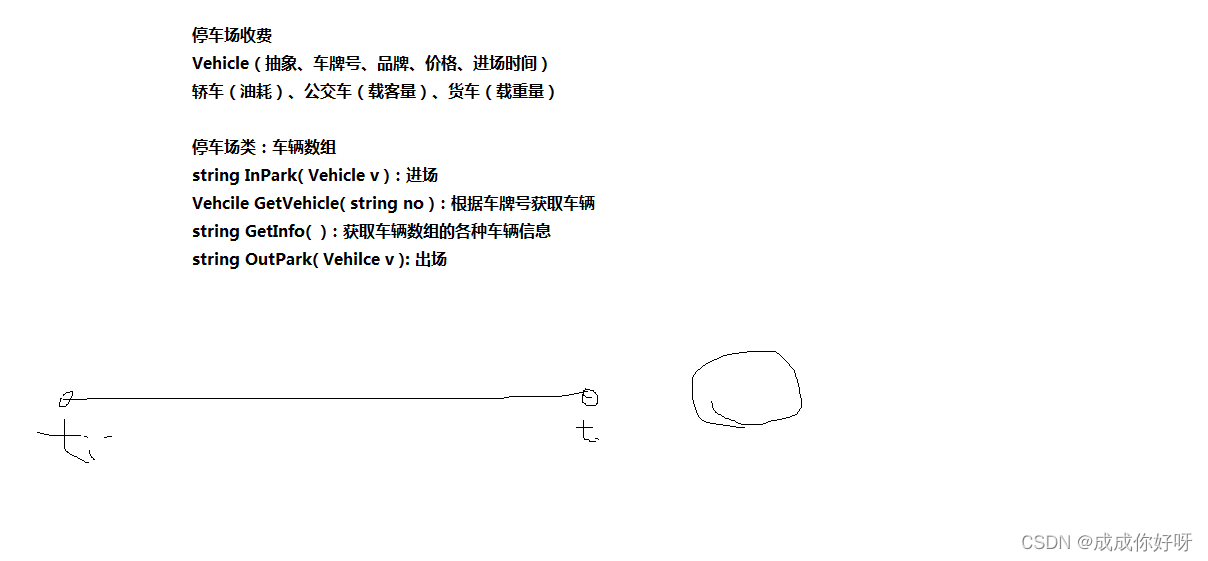
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public enum Brand
{
奔驰,
玛莎拉蒂,
保时捷,
金牛,
金杯
}
public abstract class Vehicle
{
public string No { get; set; }
public Brand VBrand { get; set; }
public int Price { get; set; }
public DateTime InTime { get; set; }
public Vehicle( string no , Brand brand , int price , DateTime intime )
{
this.No = no;
this.VBrand = brand;
this.Price = price;
this.InTime = intime;
}
public Vehicle( )
{
}
}
public class Car : Vehicle
{
public double Oil { get; set; }
public Car( ):base()
{
}
public Car( string no , Brand brand , int price , DateTime intime , double oil )
:base(no,brand,price,intime)
{
this.Oil = oil;
}
public static int operator +( Car c1 , Car c2 )
{
return c1.Price + c2.Price;
}
public static int operator -( Car c1 , Car c2)
{
return c1.Price - c2.Price;
}
}
public class Bus : Vehicle
{
public int Count { get; set; }
public Bus( )
{
}
public Bus( string no , Brand brand , int price , DateTime intime , int count )
: base( no , brand , price , intime )
{
this.Count = count;
}
}
public class Park
{
private Vehicle[ ] vehicles = null;
public Park( int length )
{
vehicles = new Vehicle[ length ];
}
public string InPark( Vehicle v )
{
string info = "很遗憾,车位已满,欢迎下次光临";
for ( int i = 0; i < vehicles.Length; i++ )
{
if ( this.vehicles[i] == null )
{
v.InTime = DateTime.Now;
this.vehicles[ i ] = v;
info = string.Format( "你的车牌号({0})车辆已经在第{1}个车位" , v.No , ( i + 1 ) );
break;
}
}
return info;
}
public Vehicle GetVehicle( string no )
{
Vehicle v = null;
foreach ( var vehicle in vehicles )
{
if ( vehicle != null )
{
if ( vehicle.No.Equals(no))
{
v = vehicle;
break;
}
}
}
return v;
}
public string GetInfo( )
{
string info = "";
int count_car = 0;
int count_bus = 0;
int count_null = 0;
foreach ( Vehicle vv in this.vehicles )
{
if ( vv != null )
{
if ( vv is Car )
{
count_car++;
}
if ( vv is Bus )
{
count_bus++;
}
}
else
{
count_null++;
}
}
info = string.Format( "当前一共有{0}辆轿车\n有{1}辆公交车\n还有{2}个空位" , count_car , count_bus , count_null );
return info;
}
public string OutPark( Vehicle v )
{
string info = "";
long money = ( DateTime.Now.Ticks - v.InTime.Ticks ) * v.Price;
info = "您的车辆需要缴纳" + money + "元";
for ( int i = 0; i < this.vehicles.Length; i++ )
{
if ( this.vehicles[ i ] != null )
{
if ( vehicles[ i ].No.Equals( v.No ) )
{
this.vehicles[ i ] = null;
}
}
}
return info;
}
}
}
主程序:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections;
namespace ConsoleApplication1
{
class Program
{
static void Main( string[ ] args )
{
Car c1 = new Car( "鄂A 888888" , Brand.玛莎拉蒂 , 10 , DateTime.Now , 2.5 );
Bus b1 = new Bus( "鄂A 666666" , Brand.金杯 , 20 , DateTime.Now,30 );
Bus b2 = new Bus( "鄂A 686868" , Brand.金牛 , 20 , DateTime.Now , 30 );
Park park = new Park( 5 );
Console.WriteLine( park.InPark( c1 ) );
Console.WriteLine( park.InPark( b1 ) );
Console.WriteLine( park.InPark( b2 ) );
for ( int i = 0; i < 100000; i++ )
{
}
Vehicle v = park.GetVehicle( "鄂A 888888" );
if ( v !=null )
{
Console.WriteLine( v.VBrand );
}
string info = park.GetInfo( );
info = park.OutPark( b2 );
Console.WriteLine( info );
}
}
}
类:引用类型
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public struct PC
{
private int aa;
public PC(int bb)
{
this.aa = bb;
}
}
}
运算符重载详细见以上主程序代码👆 索引器用的不多
|