1.静态类、静态方法、静态属性 2.抽象类 3.接口 4.向上转型和向下转型 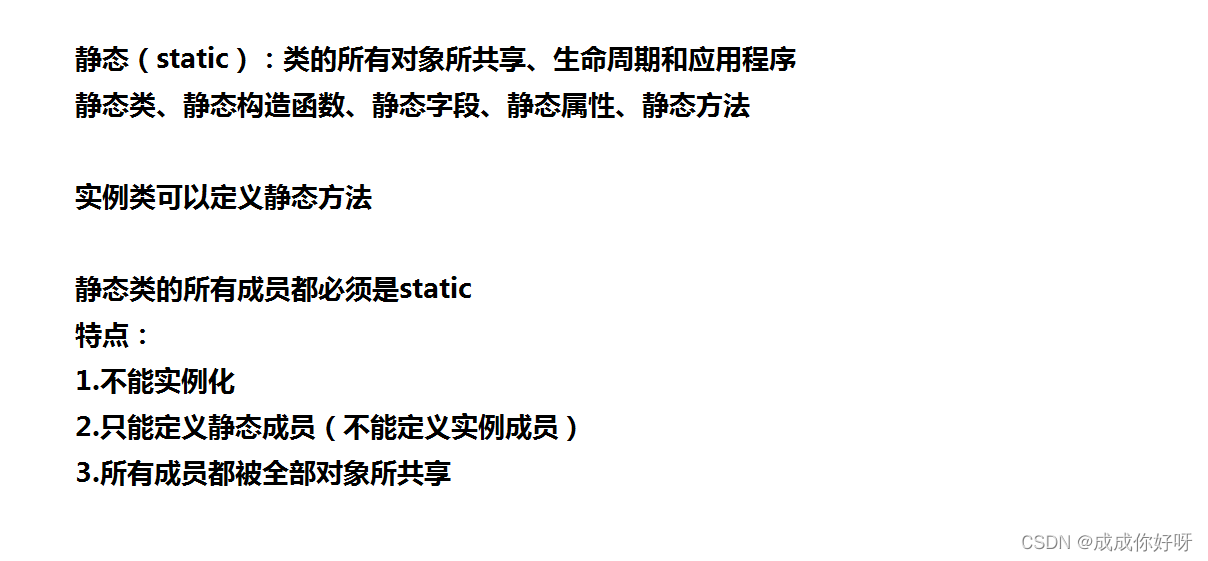
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public class YourClass
private string aa;
public static string bb;
public YourClass( )
{
Console.WriteLine( "实例构造函数...." );
}
static YourClass( )
{
Console.WriteLine( "静态构造函数....");
}
public static void FunctionStatic()
{
Console.WriteLine( "静态方法.....");
}
public void FunctionInstance( )
{
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
class Program
{
static void Main( string[ ] args )
{
YourClass yc1=new YourClass();
YourClass yc2=new YourClass();
YourClass.FunctionStatic();
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public static class StaticClass
{
{
}
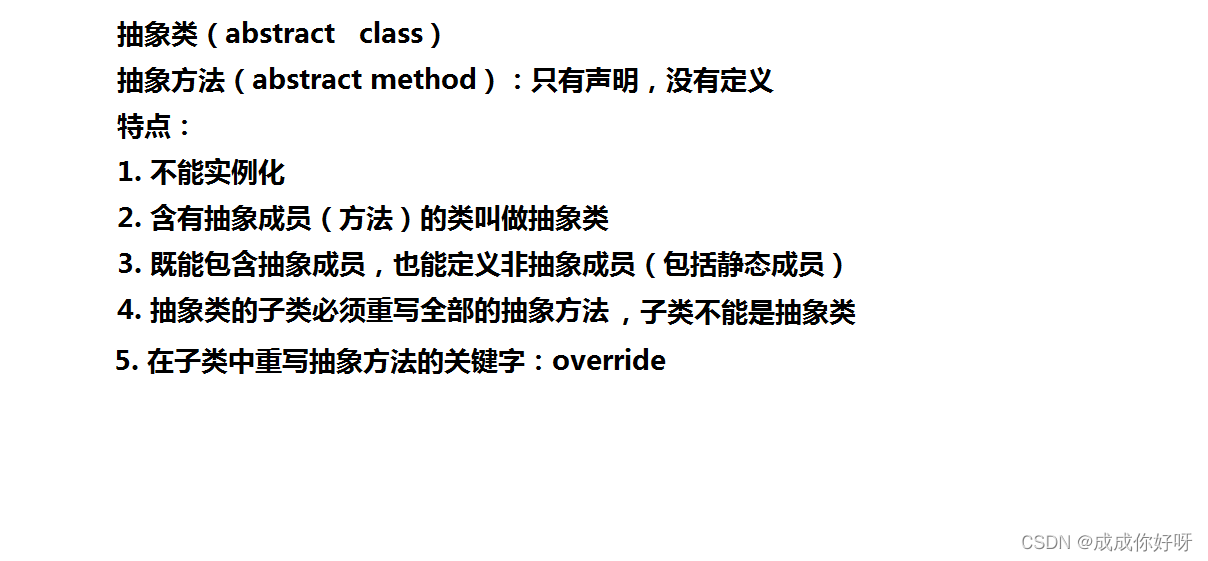 抽象类Graphic.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public abstract class Graphic
{
protected double[ ] bianChange;
public abstract double GetLength( );
public abstract double GetArea( );
public Graphic( int length )
{
bianChange = new double[ length ];
}
public virtual void Show()
{
Console.WriteLine( "这是图形的虚方法.....");
}
}
}
实现类Circle.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public class Circle:Graphic
{
public Circle( int length )
: base( length )
{
base.bianChange[ 0 ] = 10;
}
public override double GetArea( )
{
return base.bianChange[ 0 ] * base.bianChange[ 0 ] * Math.PI;
}
public override double GetLength( )
{
return base.bianChange[ 0 ] * 2 * Math.PI;
}
public override void Show( )
{
Console.WriteLine( "圆形的重写方法.....");
}
}
}
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
class Program
{
static void Main( string[ ] args )
{
Circle circle = new Circle( 1 );
double mj = circle.GetArea( );
Console.WriteLine( "面积:{0}平方米" , mj );
}
}
}
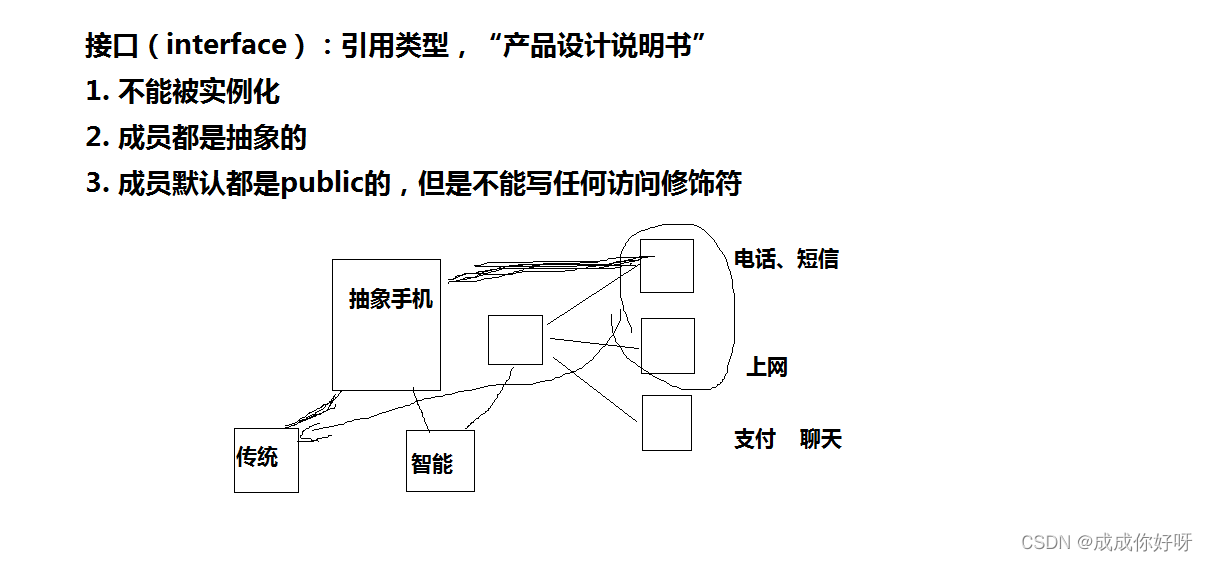 接口是通过类实现的,如同产品要根据产品说明书进行设计实现。两者有关联但不相同。
接口:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public interface IUSB
{
void Write( );
void Read( );
}
}
实现类:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public class MP3:IUSB
{
public void Write( )
{
Console.WriteLine( "下载歌曲");
}
public void Read( )
{
Console.WriteLine( "播放歌曲" );
}
}
}
主程序:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
class Program
{
static void Main( string[ ] args )
{
IUSB usb = new MP3( );
usb.Read( );
}
}
}
eg: 接口:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public interface IMobile
{
void Call( );
void Message( );
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public interface INet
{
void Internet( );
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public interface IIntellMobile
{
void Pay( );
void Chat( );
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public interface IShouJi : INet , IMobile , IIntellMobile
{
}
}
实现:可继承多个接口
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public abstract class Mobile: IMobile
{
public void Call( )
{
Console.WriteLine( "打电话....");
}
public void Message( )
{
Console.WriteLine( "发短信...." );
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public class TraditionMobile:Mobile,INet
{
public void Internet( )
{
Console.WriteLine( "网上冲浪.....");
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
public class IntellMobile:Mobile,IShouJi
{
public void Pay( )
{
Console.WriteLine("支付宝......");
}
public void Chat( )
{
Console.WriteLine( "聊天......");
}
public void Internet( )
{
Console.WriteLine( "网址冲浪....");
}
}
}
主程序:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
class Program
{
static void Main( string[ ] args )
{
IntellMobile im = new IntellMobile( );
im.Chat( );
}
}
}
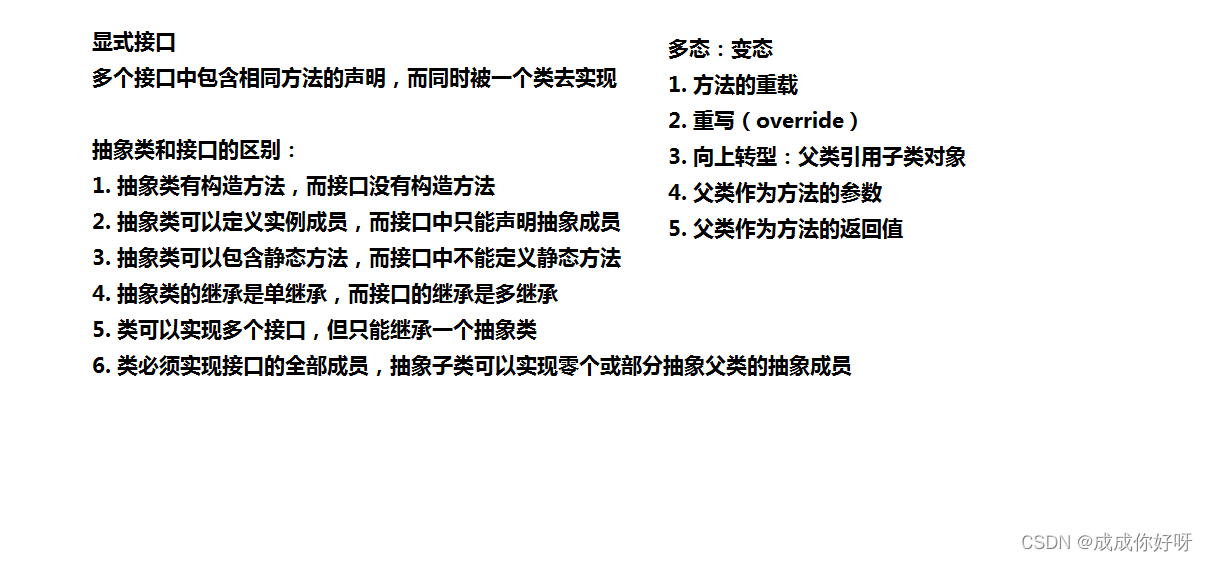
|