最近接手了一个项目,其中有个功能是生成PDF格式的合同文件。为此,现将代码分享出来!
效果图如下:
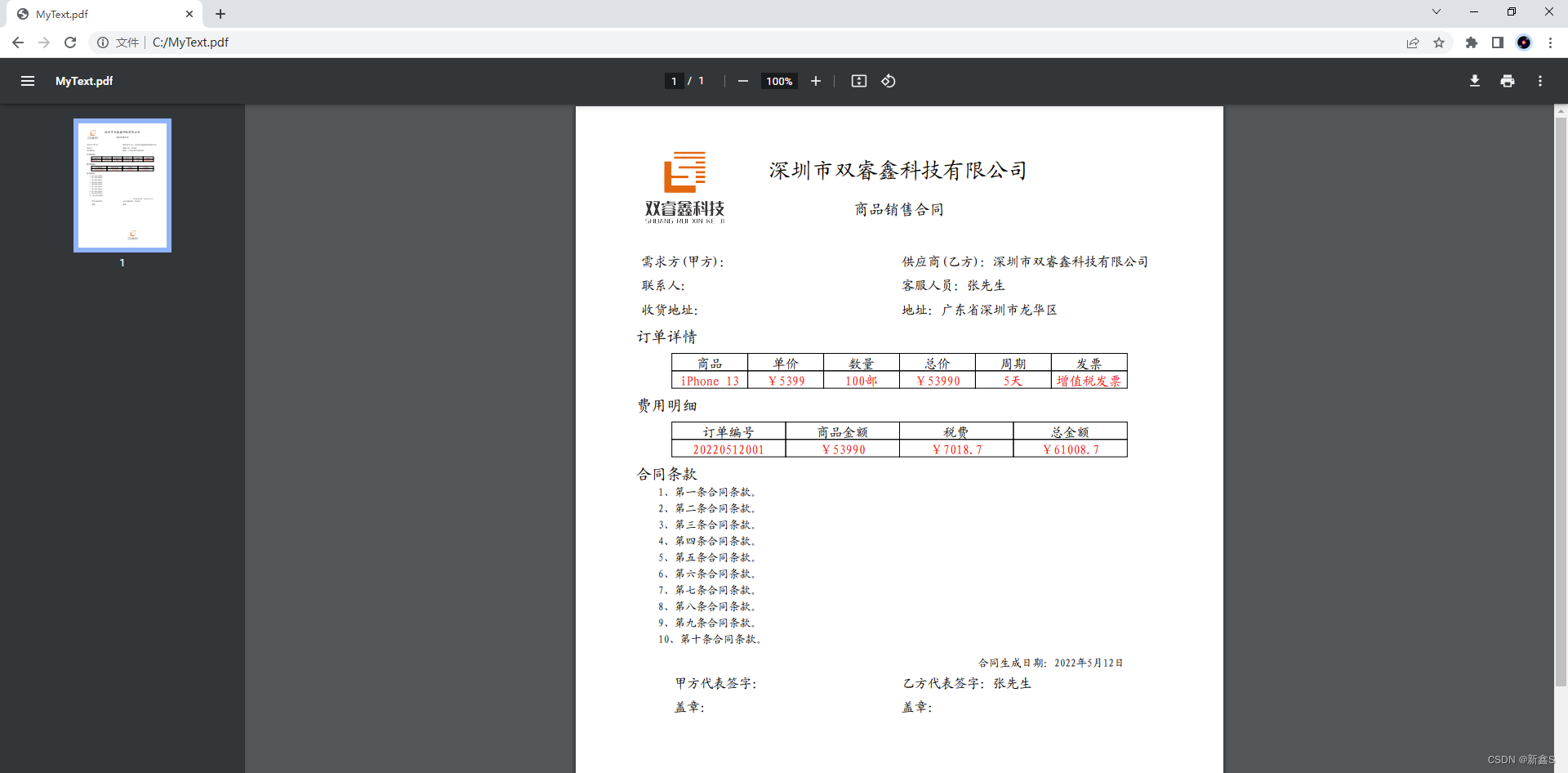
一、准备工作
首先C#代码操作pdf文件,需要引用一个pdf官方提供的两个dll,去网上下载,并将其添加引用到项目即可。 官方下载地址
PDF相关动态库 提取码:0jue 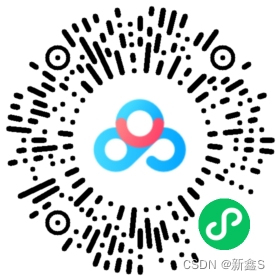
在代码中引用
using iTextSharp.text;
using iTextSharp.text.pdf;
using System.IO;
二、创建PDF文件
private static void Main() {
FileStream pdfFs = new FileStream("C:\\MyText.pdf", FileMode.Create);
Document doc = new Document();
PdfWriter.GetInstance(doc, pdfFs);
doc.Open();
iTextSharp.text.Image image = iTextSharp.text.Image.GetInstance("C:\\logo.jpg");
image.ScaleAbsolute(50, 50);
image.SetAbsolutePosition(50, PageSize.A4.Height-50);
doc.Add(image);
Font font = SetFontSizeColor(20, BaseColor.BLACK);
Paragraph tit = new Paragraph("深圳市双睿鑫科技有限公司", font);
tit.Alignment = 1;
doc.Add(tit);
List<PdfPTable> allTable = new List<PdfPTable>();
allTable.Add(SetTitlePDf());
PdfPTable table = MergeMult(allTable);
doc.Add(table);
font = SetFontSizeColor(14, BaseColor.BLACK);
tit = new Paragraph("订单详情", font);
tit.IndentationLeft = 20;
doc.Add(tit);
allTable = new List<PdfPTable>();
allTable.Add(SetMessPDF());
table = MergeMultTable(allTable);
doc.Add(table);
font = SetFontSizeColor(14, BaseColor.BLACK);
tit = new Paragraph("费用明细", font);
tit.IndentationLeft = 20;
doc.Add(tit);
allTable = new List<PdfPTable>();
allTable.Add(SetInfoPDF());
table = MergeMultTable(allTable);
doc.Add(table);
font = SetFontSizeColor(14, BaseColor.BLACK);
tit = new Paragraph("合同条款", font);
tit.IndentationLeft = 20;
doc.Add(tit);
List<string> str = new List<string>();
str.Add("第一条合同条款。");
str.Add("第二条合同条款。");
str.Add("第三条合同条款。");
str.Add("第四条合同条款。");
str.Add("第五条合同条款。");
str.Add("第六条合同条款。");
str.Add("第七条合同条款。");
str.Add("第八条合同条款。");
str.Add("第九条合同条款。");
str.Add("第十条合同条款。");
doc = contract(str, doc);
allTable = new List<PdfPTable>();
allTable.Add(SetBottomPDf());
table = MergeMultTable(allTable);
doc.Add(table);
image = iTextSharp.text.Image.GetInstance("C://contract.jpg");
image.ScaleAbsolute(150, 150);
image.SetAbsolutePosition(PageSize.A4.Width-300, 10);
doc.Add(image);
doc.Close();
pdfFs.Close();
}
三、写入内容
private static Document contract(List<string> contractList, Document doc)
{
Font font;
Paragraph tit;
for (int i = 0; i < contractList.Count; i++)
{
font = SetFont(10, BaseColor.BLACK);
tit = new Paragraph((i + 1) + "、" + contractList[i], font);
tit.IndentationLeft = 20;
tit.IndentationRight = 20;
tit.FirstLineIndent = 20;
doc.Add(tit);
}
return doc;
}
private static PdfPTable CreateOneRow(List<string> columnNameList, int fontSize)
{
PdfPTable table = new PdfPTable(columnNameList.Count);
table.SplitLate = false;
table.SplitRows = true;
Font font = SetFontSizeColor(fontSize, BaseColor.BLACK);
PdfPCell cell;
int rowCount = columnNameList.Count;
for (int i = 0; i < rowCount; i++)
{
if (columnNameList[i] != null)
{
cell = new PdfPCell(new Phrase(columnNameList[i], font));
cell.PaddingTop = 20;
cell.PaddingBottom = 30;
cell.HorizontalAlignment = 1;
cell.DisableBorderSide(15);
table.AddCell(cell);
}
}
return table;
}
private static PdfPTable CreateBottomRow(List<string> columnNameList, int fontSize)
{
PdfPTable table = new PdfPTable(columnNameList.Count);
table.SplitLate = false;
table.SplitRows = true;
Font font = SetFontSizeColor(fontSize, BaseColor.BLACK);
PdfPCell cell;
int rowCount = columnNameList.Count;
for (int i = 0; i < rowCount; i++)
{
if (columnNameList[i] != null)
{
cell = new PdfPCell(new Phrase(columnNameList[i], font));
cell.HorizontalAlignment = PdfPCell.ALIGN_RIGHT;
cell.DisableBorderSide(15);
table.AddCell(cell);
}
}
return table;
}
private static PdfPTable CreateOneRowTable(List<string> columnNameList, int fontSize)
{
PdfPTable table = new PdfPTable(columnNameList.Count);
table.SplitLate = false;
table.SplitRows = true;
Font font = SetFontSizeColor(fontSize, BaseColor.BLACK);
PdfPCell cell;
int rowCount = columnNameList.Count;
for (int i = 0; i < rowCount; i++)
{
if (columnNameList[i] != null)
{
cell = new PdfPCell(new Phrase(columnNameList[i], font));
cell.HorizontalAlignment = 1;
table.AddCell(cell);
}
}
return table;
}
private static Font SetFontSizeColor(int size, BaseColor color)
{
BaseFont bfchinese = BaseFont.CreateFont(_WebConfig.GetAppSettingsString("ttf_url"), BaseFont.IDENTITY_H, BaseFont.NOT_EMBEDDED);
Font ChFont = new iTextSharp.text.Font(bfchinese, size, Font.NORMAL, color);
return ChFont;
}
private static Font SetFont(int size, BaseColor color)
{
BaseFont bfchinese = BaseFont.CreateFont(_WebConfig.GetAppSettingsString("ttf_url"), BaseFont.IDENTITY_H, BaseFont.NOT_EMBEDDED);
Font ChFont = new iTextSharp.text.Font(bfchinese, size, Font.NORMAL, color);
return ChFont;
}
public static PdfPTable SetTitlePDf()
{
List<string> text = new List<string>();
text.Add("商品销售合同");
PdfPTable tableMain = CreateOneRow(text, 14);
text = new List<string>();
text.Add("需求方(甲方):");
text.Add("供应商(乙方):深圳市双睿鑫科技有限公司");
text.Add("联系人:");
text.Add("客服人员:张先生");
text.Add("收货地址:");
text.Add("地址:广东省深圳市龙华区");
PdfPTable tableMess = CreateMultRowMultColumn(2, text);
PdfPTable table = MergeTwo(tableMain, tableMess);
return table;
}
public static PdfPTable SetBottomPDf()
{
List<string> text = new List<string>();
text.Add("合同生成日期:" + DateTime.Now.ToLongDateString().ToString());
PdfPTable tableMain = CreateBottomRow(text, 10);
text = new List<string>();
text.Add("甲方代表签字:");
text.Add("乙方代表签字:张先生");
text.Add("盖章:");
text.Add("盖章:");
PdfPTable tableMess = CreateMultRowMultColumn(2, text);
PdfPTable table = MergeTwo(tableMain, tableMess);
return table;
}
public static PdfPTable MergeTwoTable(PdfPTable table1, PdfPTable table2)
{
PdfPTable table = new PdfPTable(1);
PdfPCell cell;
table.SplitLate = false;
table.SplitRows = true;
int rowCount = 2;
for (int i = 0; i < rowCount; i++)
{
switch (i)
{
case 0:
cell = new PdfPCell(table1);
cell.Padding = 0;
cell.DisableBorderSide(15);
table.AddCell(cell);
break;
case 1:
cell = new PdfPCell(table2);
cell.Padding = 0;
cell.DisableBorderSide(15);
table.AddCell(cell);
break;
default:
break;
}
}
return table;
}
public static PdfPTable MergeTwo(PdfPTable table1, PdfPTable table2)
{
PdfPTable table = new PdfPTable(1);
PdfPCell cell;
table.SplitLate = false;
table.SplitRows = true;
int rowCount = 2;
for (int i = 0; i < rowCount; i++)
{
switch (i)
{
case 0:
cell = new PdfPCell(table1);
cell.Padding = 0;
cell.DisableBorderSide(15);
table.AddCell(cell);
break;
case 1:
cell = new PdfPCell(table2);
cell.Padding = 0;
cell.DisableBorderSide(15);
table.AddCell(cell);
break;
default:
break;
}
}
return table;
}
public static PdfPTable SetMessPDF()
{
List<string> checkNameList = new List<string>();
checkNameList.Add("商品");
checkNameList.Add("单价");
checkNameList.Add("数量");
checkNameList.Add("总价");
checkNameList.Add("周期");
checkNameList.Add("发票");
PdfPTable tableTitle = CreateOneRowTable(checkNameList, 12);
checkNameList = new List<string>();
checkNameList.Add("iPhone 13");
checkNameList.Add("¥5399");
checkNameList.Add("100部");
checkNameList.Add("¥53990");
checkNameList.Add("5天");
checkNameList.Add("增值税发票");
PdfPTable tableMess = CreateMultRowMultColumnTable(6, checkNameList);
PdfPTable tableOverall = MergeTwoTable(tableTitle, tableMess);
return tableOverall;
}
private static PdfPTable CreateRowTitleRowDataTable(string columnTitleName, PdfPTable messPDFTable, int columnCount)
{
PdfPTable table = new PdfPTable(2);
table.SetWidths(new int[] { 1, columnCount });
table.SplitLate = false;
table.SplitRows = true;
Font font = SetFontSizeColor(15, BaseColor.BLUE);
PdfPCell cell;
int rowCount = 2;
for (int i = 0; i < rowCount; i++)
{
switch (i)
{
case 0:
cell = new PdfPCell(new Phrase(columnTitleName, font));
cell.HorizontalAlignment = 1;
table.AddCell(cell);
break;
case 1:
cell = new PdfPCell(messPDFTable);
cell.Padding = 0;
table.AddCell(cell);
break;
default:
break;
}
}
return table;
}
private static PdfPTable CreateMultRowMultColumn(int column, List<string> columnDataNameList)
{
PdfPTable table = new PdfPTable(column);
Font font = SetFontSizeColor(12, BaseColor.BLACK);
table.SplitLate = false;
table.SplitRows = true;
PdfPCell cell;
int rowColumn = columnDataNameList.Count;
for (int i = 0; i < rowColumn; i++)
{
cell = new PdfPCell(new Phrase(columnDataNameList[i], font));
cell.PaddingTop = 5;
cell.PaddingBottom = 5;
cell.DisableBorderSide(15);
table.AddCell(cell);
}
return table;
}
private static PdfPTable CreateMultRowMultColumnTable(int column, List<string> columnDataNameList)
{
PdfPTable table = new PdfPTable(column);
Font font = SetFontSizeColor(12, BaseColor.RED);
table.SplitLate = false;
table.SplitRows = true;
PdfPCell cell;
int rowColumn = columnDataNameList.Count;
for (int i = 0; i < rowColumn; i++)
{
cell = new PdfPCell(new Phrase(columnDataNameList[i], font));
cell.HorizontalAlignment = 1;
table.AddCell(cell);
}
return table;
}
public static PdfPTable SetInfoPDF()
{
List<string> checkNameList = new List<string>();
checkNameList.Add("订单编号");
checkNameList.Add("商品金额");
checkNameList.Add("税费");
checkNameList.Add("总金额");
PdfPTable tableTitle = CreateOneRowTable(checkNameList, 12);
checkNameList = new List<string>();
checkNameList.Add("20220512001");
checkNameList.Add("¥53990");
checkNameList.Add("¥7018.7");
checkNameList.Add("¥61008.7");
PdfPTable tableMess = CreateMultRowMultColumnTable(4, checkNameList);
PdfPTable tableOverall = MergeTwoTable(tableTitle, tableMess);
return tableOverall;
}
public static PdfPTable MergeMultTable(List<PdfPTable> pdfList)
{
PdfPTable table = new PdfPTable(1);
PdfPCell cell;
table.SplitLate = false;
table.SplitRows = true;
foreach (PdfPTable pdf in pdfList)
{
cell = new PdfPCell(pdf);
cell.Padding = 0;
cell.PaddingTop = 10;
cell.DisableBorderSide(15);
table.AddCell(cell);
}
return table;
}
public static PdfPTable MergeMult(List<PdfPTable> pdfList)
{
PdfPTable table = new PdfPTable(1);
PdfPCell cell;
table.SplitLate = false;
table.SplitRows = true;
foreach (PdfPTable pdf in pdfList)
{
cell = new PdfPCell(pdf);
cell.PaddingLeft = -30;
cell.PaddingRight = -30;
cell.DisableBorderSide(15);
table.AddCell(cell);
}
return table;
}
四、总结
C#生成PDF文件无非就是表格类PdfPTable与单元格类PdfPCell互相配合使用而已!根据自己的需求封装函数创建表格返回就行。
|