easyexcel导出
一、依赖
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>2.2.10</version>
</dependency>
参考文档:语雀easyexcel文档
二、导出
普通导出
public static void exportExcel(HttpServletResponse response, String fileName, String sheetName, List<?> list, Class<?> clazz) {
if (CollectionUtils.isEmpty(list)) {
throw new RuntimeException();
}
if (StringUtils.isEmpty(fileName)) {
fileName = new Date().toString();
}
try {
response.setContentType("application/vnd.ms-excel");
response.setCharacterEncoding("utf-8");
try {
fileName = URLEncoder.encode(fileName, "UTF-8").replaceAll("\\+", "%20");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
response.setHeader("Content-disposition", "attachment;filename=" + fileName + ".xlsx");
EasyExcel.write(response.getOutputStream(), clazz)
.autoCloseStream(Boolean.TRUE).sheet(sheetName)
.doWrite(list);
} catch (Exception e) {
e.printStackTrace();
}
}
实体类
@HeadRowHeight
@HeadStyle(horizontalAlignment = CENTER)
public class ExportAssetModel {
@ColumnWidth(30)
@HeadStyle(fillForegroundColor = 40)
@ContentStyle(wrapped = true)
@ExcelProperty({"基础属性", "制造商\n(可输入数字0-9字母a-zA-Z,下划线_-以及汉字,最大长字符64位)\n(必填)"})
private String manufacturer;
@ColumnWidth(30)
@HeadStyle(fillForegroundColor = 40)
@ContentStyle(wrapped = true)
@ExcelProperty({"基础属性", "型号\n(请输入1-64个字符,可输入中文,数字0-9,字母a-zA-Z,空格,以及字符+._-()()[]:,/)\n(必填)"})
private String model;
@ColumnWidth(30)
@HeadStyle(fillForegroundColor = 40)
@ContentStyle(wrapped = true)
@ExcelProperty({"基础属性", "设备类型\n(请输入1-64个字符,只能输入中文,英文,数字,空格和特殊字符#@_.*-/[]()~′^{}|:;、,‘’)\n(必填)"})
private String deviceTypeStr;
@ColumnWidth(30)
@HeadStyle(fillForegroundColor = 40)
@ContentStyle(wrapped = true)
@ExcelProperty({"基础属性", "状态\n(只能输入使用中、未使用)"})
private String status;
@ColumnWidth(30)
@HeadStyle(fillForegroundColor = 40)
@ContentStyle(wrapped = true)
@ExcelProperty({"基础属性", "备注\n(请输入1-64个字符,只能输入中文,英文,数字,空格和特殊字符#@_.*-/[]()~′^{}|:;、,‘’)"})
private String remark;
@ColumnWidth(30)
@HeadStyle(fillForegroundColor = 53)
@ContentStyle(wrapped = true)
@ExcelProperty({"扩展属性", "高度(U)\n(请输入1-100之间的整数)\n(IT设备、通信设备必填)"})
private String height;
@ColumnWidth(30)
@HeadStyle(fillForegroundColor = 53)
@ContentStyle(wrapped = true)
@ExcelProperty({"扩展属性", "重量(Kg)\n(请输入0-9999.99之间的数)"})
private String weight;
@ColumnWidth(30)
@HeadStyle(fillForegroundColor = 53)
@ContentStyle(wrapped = true)
@ExcelProperty({"扩展属性", "额定功率(W)\n(请输入0-9999999.99之间的数)\n(IT设备、通信设备必填)"})
private String ratedPower;
@ColumnWidth(30)
@HeadStyle(fillForegroundColor = 53)
@ContentStyle(wrapped = true)
@ExcelProperty({"扩展属性", "配电端口数\n(请输入0-2048之间的整数)"})
private String distributionPort;
@ColumnWidth(30)
@HeadStyle(fillForegroundColor = 53)
@ContentStyle(wrapped = true)
@ExcelProperty({"扩展属性", "网络端口数\n(请输入0-2048之间的整数)"})
private String networkPort;
}
导出效果

自定义表头导出
public static void customHeadExportExcel(HttpServletResponse response, String fileName, String sheetName, List<List<Object>> list, List<List<String>> head) {
if (CollectionUtils.isEmpty(list)) {
throw new RuntimeException();
}
if (CollectionUtils.isEmpty(head)) {
throw new RuntimeException();
}
if (StringUtils.isEmpty(fileName)) {
fileName = new Date().toString();
}
try {
response.setContentType("application/vnd.ms-excel");
response.setCharacterEncoding("utf-8");
try {
fileName = URLEncoder.encode(fileName, "UTF-8").replaceAll("\\+", "%20");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
response.setHeader("Content-disposition", "attachment;filename=" + fileName + ".xlsx");
EasyExcel.write(response.getOutputStream())
.head(head)
.registerWriteHandler(myHorizontalCellStyleStrategy())
.registerWriteHandler(new SimpleColumnWidthStyleStrategy(25))
.autoCloseStream(Boolean.TRUE)
.sheet(sheetName)
.doWrite(list);
} catch (Exception e) {
e.printStackTrace();
}
}
private static HorizontalCellStyleStrategy myHorizontalCellStyleStrategy() {
WriteCellStyle headWriteCellStyle = new WriteCellStyle();
headWriteCellStyle.setHorizontalAlignment(HorizontalAlignment.CENTER);
headWriteCellStyle.setFillForegroundColor(IndexedColors.PALE_BLUE.getIndex());
WriteFont headWriteFont = new WriteFont();
headWriteFont.setBold(true);
headWriteFont.setFontName("宋体");
headWriteFont.setFontHeightInPoints((short) 12);
headWriteCellStyle.setWriteFont(headWriteFont);
List<WriteCellStyle> listCntWritCellSty = new ArrayList<>();
WriteCellStyle contentWriteCellStyle = new WriteCellStyle();
WriteFont contentWriteFont = new WriteFont();
contentWriteFont.setFontName("宋体");
contentWriteFont.setFontHeightInPoints((short) 11);
contentWriteCellStyle.setWriteFont(contentWriteFont);
contentWriteCellStyle.setWrapped(true);
contentWriteCellStyle.setVerticalAlignment(VerticalAlignment.CENTER);
contentWriteCellStyle.setFillPatternType(FillPatternType.SOLID_FOREGROUND);
contentWriteCellStyle.setFillForegroundColor(IndexedColors.YELLOW.getIndex());
contentWriteCellStyle.setHorizontalAlignment(HorizontalAlignment.LEFT);
setBorderStyle(contentWriteCellStyle);
listCntWritCellSty.add(contentWriteCellStyle);
WriteCellStyle contentWriteCellStyle2 = new WriteCellStyle();
contentWriteCellStyle2.setFillPatternType(FillPatternType.SOLID_FOREGROUND);
contentWriteCellStyle2.setFillForegroundColor(IndexedColors.GREEN.getIndex());
contentWriteCellStyle2.setVerticalAlignment(VerticalAlignment.CENTER);
contentWriteCellStyle2.setHorizontalAlignment(HorizontalAlignment.LEFT);
setBorderStyle(contentWriteCellStyle2);
listCntWritCellSty.add(contentWriteCellStyle2);
return new HorizontalCellStyleStrategy(headWriteCellStyle, listCntWritCellSty);
}
private static void setBorderStyle(WriteCellStyle contentWriteCellStyle) {
contentWriteCellStyle.setBorderLeft(BorderStyle.THIN);
contentWriteCellStyle.setBorderTop(BorderStyle.THIN);
contentWriteCellStyle.setBorderRight(BorderStyle.THIN);
contentWriteCellStyle.setBorderBottom(BorderStyle.THIN);
}
多sheet导出
public static void exportMoreExcel(HttpServletResponse response, String fileName,
String sheetNameOne,String sheetNameTwo,String sheetNameThree,
List<List<Object>> list, List<List<String>> head,
List<?> listTwo,Class<?> clazzTwo, List<?> listThree,Class<?> clazzThree) {
if (CollectionUtils.isEmpty(list)) {
throw new RuntimeException();
}
if (CollectionUtils.isEmpty(head)) {
throw new RuntimeException();
}
if (StringUtils.isEmpty(fileName)) {
fileName = new Date().toString();
}
try {
response.setContentType("application/vnd.ms-excel");
response.setCharacterEncoding("utf-8");
try {
fileName = URLEncoder.encode(fileName, "UTF-8").replaceAll("\\+", "%20");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
response.setHeader("Content-disposition", "attachment;filename=" + fileName + ".xlsx");
ExcelWriter excelWriter = EasyExcel.write(response.getOutputStream()).build();
WriteSheet writeSheet = EasyExcel.writerSheet(1, sheetNameOne)
.head(head)
.registerWriteHandler(myHorizontalCellStyleStrategy())
.registerWriteHandler(new SimpleColumnWidthStyleStrategy(25))
.build();
excelWriter.write(list, writeSheet);
writeSheet = EasyExcel.writerSheet(2, sheetNameTwo).head(clazzTwo).build();
excelWriter.write(listTwo, writeSheet);
writeSheet = EasyExcel.writerSheet(3, sheetNameThree).head(clazzThree).build();
excelWriter.write(listThree, writeSheet);
excelWriter.finish();
} catch (Exception e) {
e.printStackTrace();
}
}
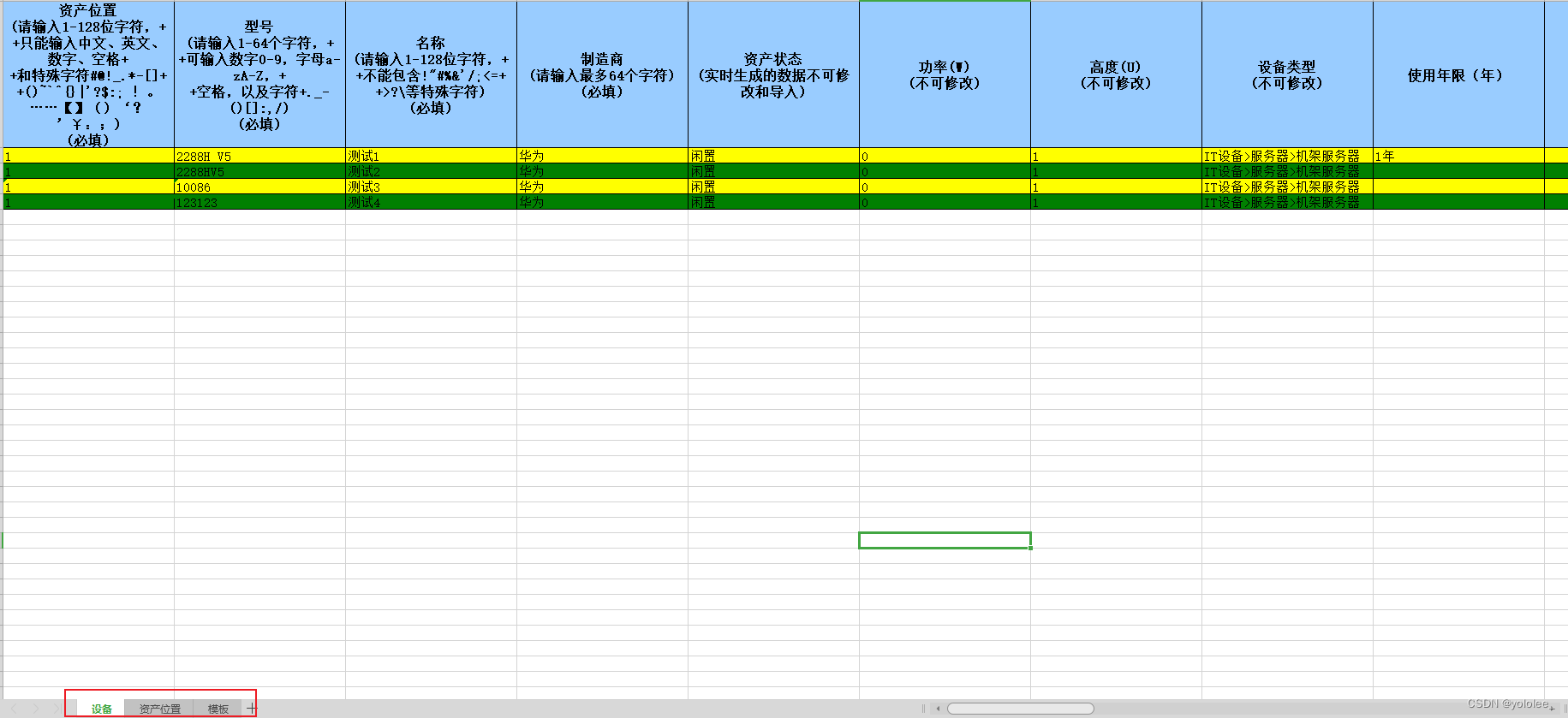
|