C#获取指定的WebService接口的所有可调用方法,将其绑定的树图控件(TreeView)中,我们引用天气WebService服务为例,联网情况下均可用。
PS:天气WeatherWebService是一个WebService,而不是WCF。如果按照WCF调用,永远无法调用接口成功
一、新建Windows窗体应用程序WeatherWebServiceDemo
将默认的Form1重命名为FormWeather,窗体FormWeather设计如图
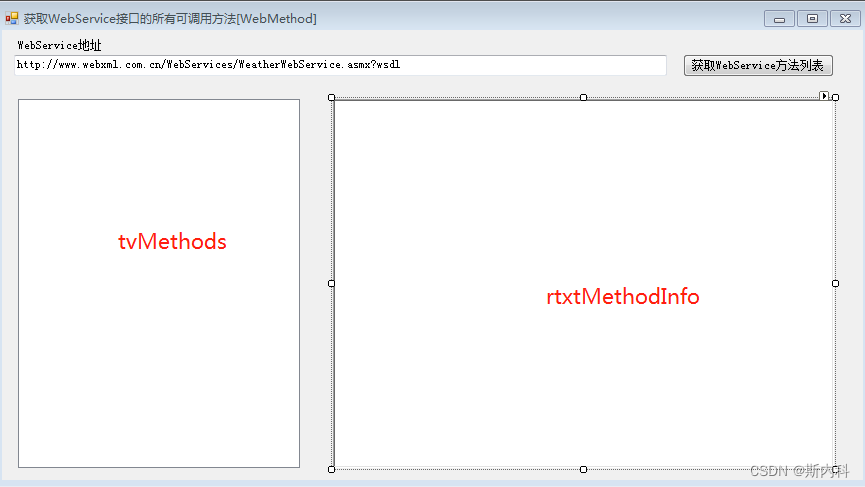
?为树图控件tvAllMethods绑定事件AfterSelect
?二、右键 引用---->添加服务引用
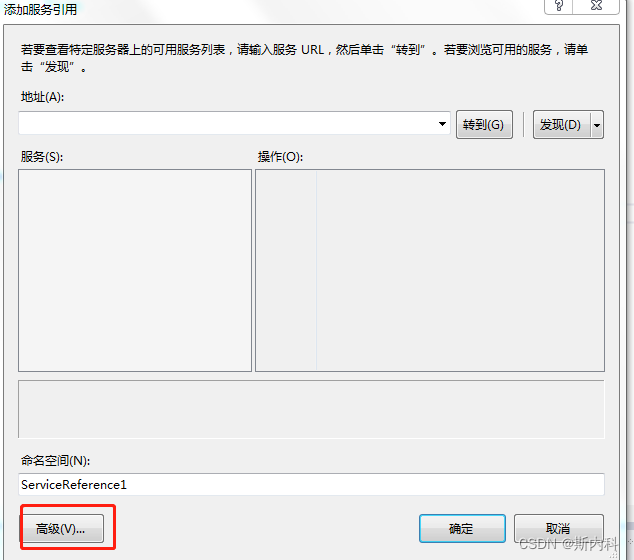
点击“高级” ---->添加Web引用,输入Url为
http://www.webxml.com.cn/WebServices/WeatherWebService.asmx?wsdl
点击转到
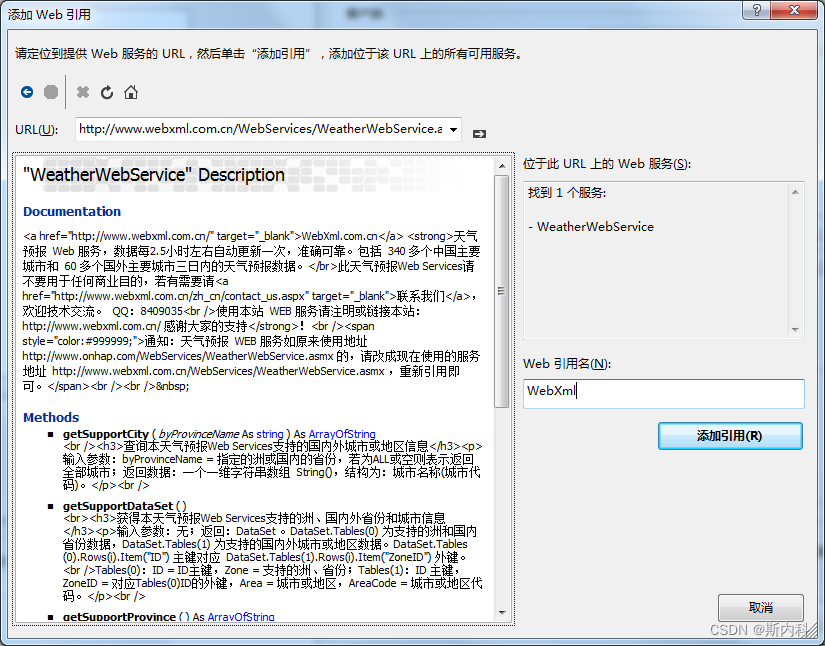
点击“添加引用”即可。?
三、新建类文件SoapUtil.cs
SoapUtil源程序如下:
using System;
using System.Collections.Generic;
using System.CodeDom;
using System.CodeDom.Compiler;
using System.IO;
using System.Net;
using System.Reflection;
using System.Web.Services.Description;
using System.Xml.Serialization;
namespace WeatherWebServiceDemo
{
/// <summary>
/// 动态获取WebService的所有方法
/// 斯内科 2022-06-08
/// </summary>
public class SoapUtil
{
/// <summary>
/// 获取WebService接口的所有WebMethod方法
/// 通过WebService方法的特性为【System.Web.Services.Protocols.SoapDocumentMethodAttribute】
/// 根据特性SoapDocumentMethodAttribute来筛选出所有WebMethod方法
/// </summary>
/// <param name="url"></param>
public static List<MethodInfo> GetAllWebMethodsFromService(string url, out string className)
{
className = "SoapWebService";
if (!url.EndsWith("?wsdl", StringComparison.CurrentCultureIgnoreCase))
{
url = url + "?wsdl";
}
string tempUrl = url;
if (url.EndsWith("?wsdl", StringComparison.CurrentCultureIgnoreCase))
{
tempUrl = url.Substring(0, url.Length - 5);
}
className = Path.GetFileNameWithoutExtension(tempUrl);
// 1. 使用 WebClient 下载 WSDL 信息。
WebClient web = new WebClient();
Stream stream = web.OpenRead(url);
// 2. 创建和格式化 WSDL 文档。
ServiceDescription description = ServiceDescription.Read(stream);
// 3. 创建客户端代理代理类。
ServiceDescriptionImporter importer = new ServiceDescriptionImporter();
// 指定访问协议。
importer.ProtocolName = "Soap";
// 生成客户端代理。
importer.Style = ServiceDescriptionImportStyle.Client;
importer.CodeGenerationOptions = CodeGenerationOptions.GenerateProperties | CodeGenerationOptions.GenerateNewAsync;
// 添加 WSDL 文档。
importer.AddServiceDescription(description, null, null);
// 4. 使用 CodeDom 编译客户端代理类。
// 为代理类添加命名空间,缺省为全局空间。
CodeNamespace nmspace = new CodeNamespace();
CodeCompileUnit unit = new CodeCompileUnit();
unit.Namespaces.Add(nmspace);
ServiceDescriptionImportWarnings warning = importer.Import(nmspace, unit);
CodeDomProvider provider = CodeDomProvider.CreateProvider("CSharp");
CompilerParameters parameter = new CompilerParameters();
parameter.GenerateExecutable = false;
parameter.GenerateInMemory = true;//在内存中生成输出
// 可以指定你所需的任何文件名。
parameter.OutputAssembly = AppDomain.CurrentDomain.BaseDirectory + className + ".dll";
parameter.ReferencedAssemblies.Add("System.dll");
parameter.ReferencedAssemblies.Add("System.XML.dll");
parameter.ReferencedAssemblies.Add("System.Web.Services.dll");
parameter.ReferencedAssemblies.Add("System.Data.dll");
// 生成dll文件,并会把WebService信息写入到dll里面
CompilerResults result = provider.CompileAssemblyFromDom(parameter, unit);
Assembly assembly = result.CompiledAssembly;
Type type = assembly.GetType(className);
List<MethodInfo> methodInfoList = new List<MethodInfo>();
MethodInfo[] methodInfos = type.GetMethods();
for (int i = 0; i < methodInfos.Length; i++)
{
MethodInfo methodInfo = methodInfos[i];
//WebMethod方法的特性为:System.Web.Services.Protocols.SoapDocumentMethodAttribute
Attribute attribute = methodInfo.GetCustomAttribute(typeof(System.Web.Services.Protocols.SoapDocumentMethodAttribute));
if (methodInfo.MemberType == MemberTypes.Method && attribute != null)
{
methodInfoList.Add(methodInfo);
}
}
return methodInfoList;
}
}
}
四、窗体FormWeather的主要程序如下:
(忽略设计器自动生成的部分类代码)
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WeatherWebServiceDemo
{
public partial class FormWeather : Form
{
public FormWeather()
{
InitializeComponent();
}
private void btnGetWebMethod_Click(object sender, EventArgs e)
{
tvAllMethods.Nodes.Clear();
string url = txtUrl.Text.Trim();
string className;
try
{
List<MethodInfo> methodInfos = SoapUtil.GetAllWebMethodsFromService(url, out className);
TreeNode rootNode = new TreeNode("服务名:" + className);//以类名作为根节点
tvAllMethods.Nodes.Add(rootNode);
for (int i = 0; i < methodInfos.Count; i++)
{
MethodInfo methodInfo = methodInfos[i];
TreeNode treeNode = new TreeNode($"{methodInfo.Name}");
//设置Tag标签为方法信息
treeNode.Tag = methodInfo;
rootNode.Nodes.Add(treeNode);
}
tvAllMethods.ExpandAll();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "出错");
}
}
private void tvAllMethods_AfterSelect(object sender, TreeViewEventArgs e)
{
rtxtMethodInfo.Clear();
if (e.Node.Level == 0)
{
//不考虑根节点:服务类名
return;
}
MethodInfo methodInfo = e.Node.Tag as MethodInfo;
if (methodInfo == null)
{
return;
}
ParameterInfo[] parameterInfos = methodInfo.GetParameters();
rtxtMethodInfo.AppendText($"{methodInfo.ToString()}\n");
rtxtMethodInfo.AppendText($"接口方法名:【{methodInfo.Name}】\n");
rtxtMethodInfo.AppendText($"接口返回类型:【{methodInfo.ReturnType}】\n");
rtxtMethodInfo.AppendText($"接口参数个数:【{parameterInfos.Length}】\n");
for (int i = 0; i < parameterInfos.Length; i++)
{
rtxtMethodInfo.AppendText($" --->参数【{i + 1}】:【{parameterInfos[i].ToString()}】\n");
}
rtxtMethodInfo.AppendText($"接口整体描述:\n{methodInfo.ReturnType} {methodInfo.Name}({string.Join(",", parameterInfos.Select(p => p.ToString()))})");
}
}
}
五、程序测试如图:
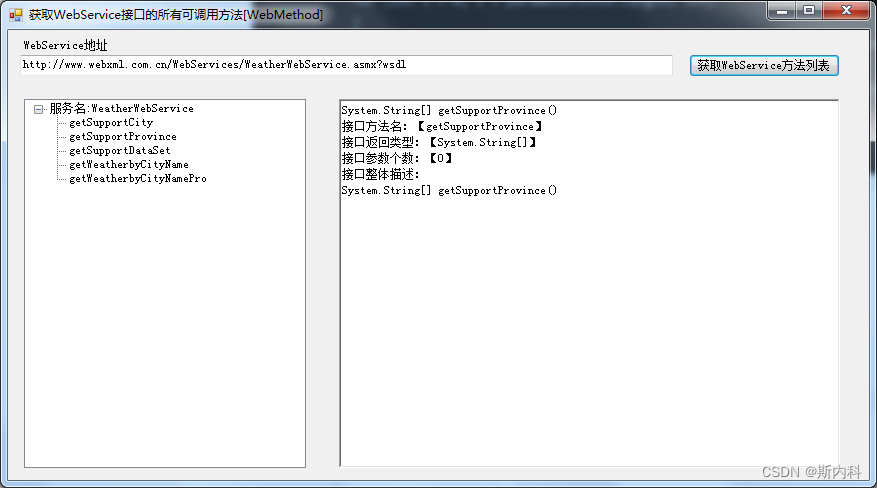
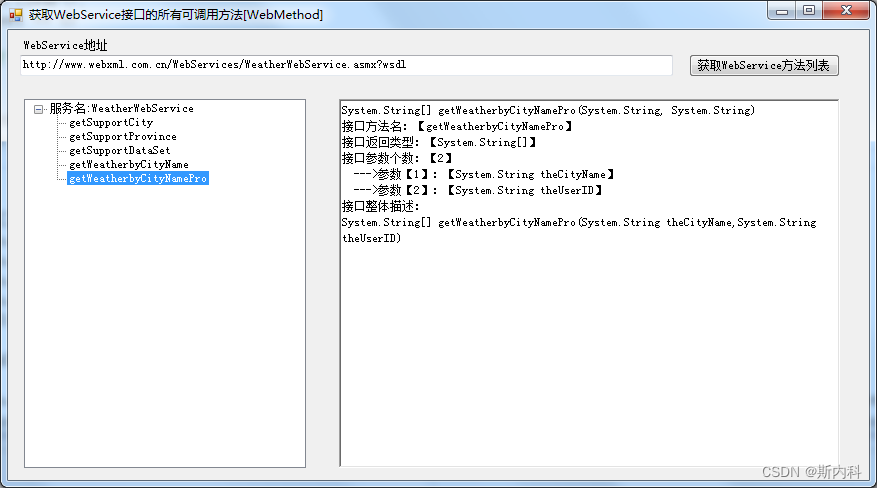
?
?
?
|