1、简介
<filesystem>是用于访问操作和检索有关路径、文件和目录的信息的类和函数的头文件。
在 Visual Studio 2017 发布时,<filesystem>头文件还不是 C++ 标准。Visual Studio 2017 RTW 中的 C++ 实现了ISO/IEC JTC 1/SC 22/WG 21 N4100中的最终草案标准。Visual Studio 2017 版本 15.7 及更高版本支持新的 C++17<filesystem>标准。这是一个全新的实现,与以前的std::experimental版本不兼容。符号链接支持、错误修复和标准所需行为的更改使其成为必要。在 Visual Studio 2019 版本 16.3 及更高版本中,包括<filesystem>仅提供新的std::filesystem. 包括<experimental/filesystem>仅提供旧的实验性实现。实验性实现将在库的下一个 ABI 破坏版本中删除。
1.1 C/C++标准种类
- ?前C语?的标准有:C89(ANSI C)、C90、C95、C99(ISO C)、C11(C1x)
- ?前C++语?的标准有:C++98、C++03(对98?幅修改)、C++11(全?进化)、C++14、C++17
1.2 VS对C++标准的支持情况
- C++17
VS2017基本支持,VS2015部分支持。 - C++14
VS2017可以完全支持,VS2015基本支持,VS2013部分支持。 - C++11
VS2015及以上完全支持。VS2013基本支持,VS2012部分支持,VS2010及以下版本不支持。 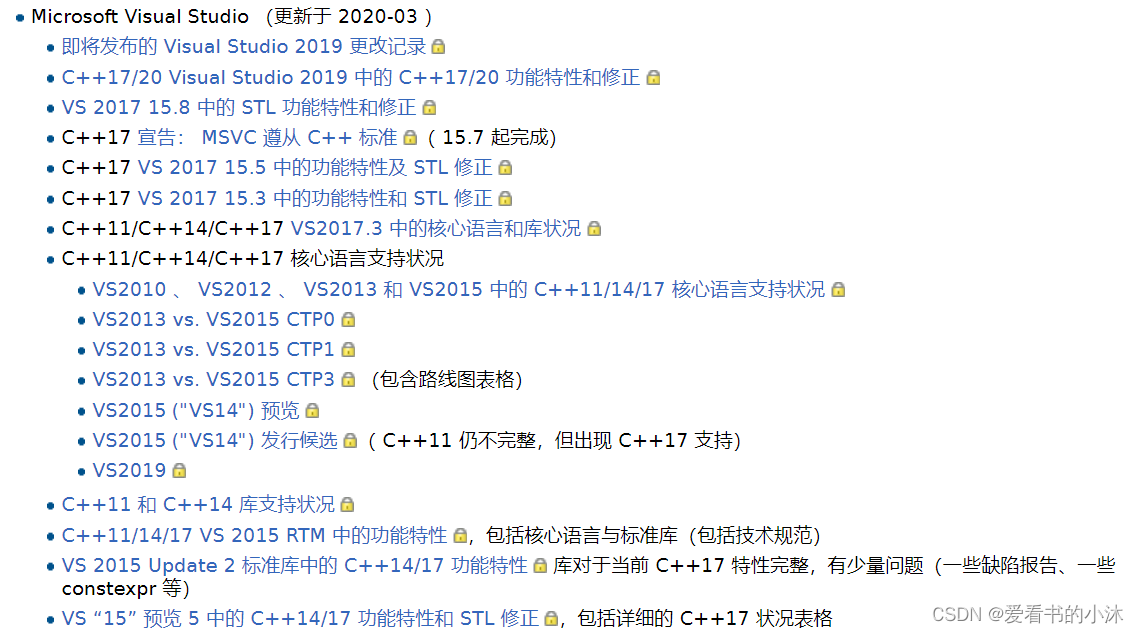
#ifdef __cpp_lib_filesystem
#pragma message("__cpp_lib_filesystem: supported.")
#include <filesystem>
#endif
#ifdef __cpp_lib_experimental_filesystem
#pragma message("__cpp_lib_experimental_filesystem: supported.")
#include <experimental/filesystem>
namespace std {
namespace filesystem = experimental::filesystem;
}
#endif
/Zc:__cplusplus
std::cout << __cplusplus << std::endl;
1.3 gcc对C++标准的支持情况
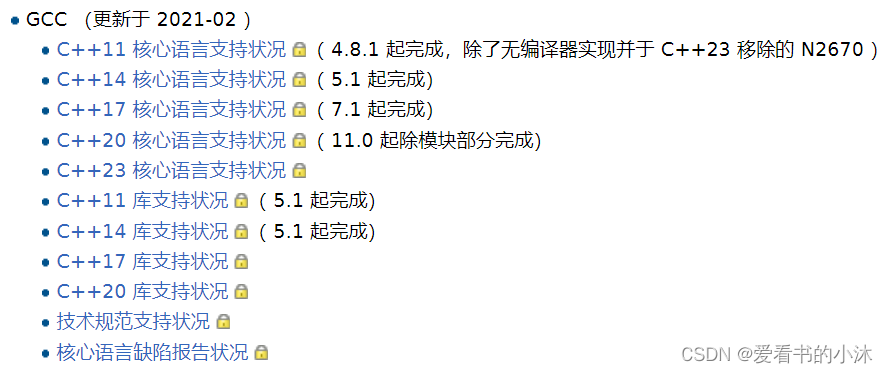
2、头文件<filesystem>介绍
https://docs.microsoft.com/en-us/cpp/standard-library/filesystem-functions?view=msvc-170
2.1 类(Classes)
Name | Description |
---|
directory_entry class | Describes an object that is returned by a directory_iterator or a recursive_directory_iterator and contains a path. | directory_iterator class | Describes an input iterator that sequences through the file names in a file-system directory. | filesystem_error class | A base class for exceptions that are thrown to report a low-level system overflow. | path class | Defines a class that stores an object of template type String that is suitable for use as a file name. | recursive_directory_iterator class | Describes an input iterator that sequences through the file names in a file-system directory. The iterator can also descend into subdirectories. | file_status class | Wraps a file_type. |
2.2 结构(Structs)
Name | Description |
---|
space_info structure | Holds information about a volume. |
struct space_info
{
uintmax_t capacity;
uintmax_t free;
uintmax_t available;
};
2.3 函数(Functions)
https://docs.microsoft.com/en-us/cpp/standard-library/filesystem-functions?view=msvc-170
2.4 操作符(Operators)
https://docs.microsoft.com/en-us/cpp/standard-library/filesystem-operators?view=msvc-170
Name | Description |
---|
operator== | bool operator==(const path& left, const path& right) noexcept; | operator!= | bool operator!=(const path& left, const path& right) noexcept; | operator< | bool operator<(const path& left, const path& right) noexcept; | operator<= | bool operator<=(const path& left, const path& right) noexcept; | operator> | bool operator>(const path& left, const path& right) noexcept; | operator>= | bool operator>=(const path& left, const path& right) noexcept; | operator/ | path operator/(const path& left, const path& right); | operator<< | template <class Elem, class Traits> basic_ostream<Elem, Traits>& operator<<(basic_ostream<Elem, Traits>& os, const path& pval); | operator>> | template <class Elem, class Traits> basic_istream<Elem, Traits>& operator<<(basic_istream<Elem, Traits>& is, const path& pval); |
2.5 枚举(Enumerations)
Name | Description |
---|
copy_options | An enumeration that is used with copy_file and determines behavior if a destination file already exists. | directory_options | An enumeration that specifies options for directory iterators. | file_type | An enumeration for file types. | perm_options | Enumerates options for the permissions function. | perms | A bitmask type used to convey permissions and options to permissions |
3、头文件<filesystem>的函数
函数名 | 功能 |
---|
void copy(const path& from, const path& to) | 目录复制 | path absolute(const path& pval, const path& base = current_path()) | 获取相对于base的绝对路径 | bool create_directory(const path& pval) | 当目录不存在时创建目录 | bool create_directories(const path& pval) | 形如/a/b/c这样的,如果都不存在,创建目录结构 | uintmax_t file_size(const path& pval) | 返回目录的大小 | file_time_type last_write_time(const path& pval) | 返回目录最后修改日期的file_time_type对象 | bool exists(const path& pval) | 用于判断path是否存在 | bool remove(const path& pval) | 删除目录 | uintmax_t remove_all(const path& pval) | 递归删除目录下所有文件,返回被成功删除的文件个数 | void rename(const path& from, const path& to) | 移动文件或者重命名 |
#include <iostream>
#ifdef __cpp_lib_filesystem
#pragma message("__cpp_lib_filesystem: supported.")
#include <filesystem>
#endif
#ifdef __cpp_lib_experimental_filesystem
#pragma message("__cpp_lib_experimental_filesystem: supported.")
#include <experimental/filesystem>
#endif
namespace fs = std::filesystem;
int main() {
std::cout << __cplusplus << std::endl;
fs::current_path(fs::temp_directory_path());
fs::create_directories("test2022/1/2/a");
fs::create_directory("test2022/1/2/b");
fs::permissions("test2022/1/2/b", fs::perms::others_all, fs::perm_options::remove);
fs::create_directory("test2022/1/2/c", "test2022/1/2/b");
std::system("tree test2022");
fs::remove_all("test2022");
return 0;
}
4、头文件<filesystem>的path类
函数名 | 功能 |
---|
path& append(const _Src& source) | 在path末尾加入一层结构 | path& assign(string_type& source) | 赋值(字符串) | void clear() | 清空 | int compare(const path& other) | 进行比较 | bool empty() | 空判断 | path filename() | 返回文件名(有后缀) | path stem() | 返回文件名(不含后缀) | path extension() | 返回文件后缀名 | path is_absolute() | 判断是否为绝对路径 | path is_relative() | 判断是否为相对路径 | path relative_path() | 返回相对路径 | path parent_path() | 返回父路径 | path& replace_extension(const path& replace) | 替换文件后缀 |
#include <iostream>
#include <set>
#ifdef __cpp_lib_filesystem
#pragma message("__cpp_lib_filesystem: supported.")
#include <filesystem>
#endif
#ifdef __cpp_lib_experimental_filesystem
#pragma message("__cpp_lib_experimental_filesystem: supported.")
#include <experimental/filesystem>
#endif
namespace fs = std::filesystem;
int main() {
std::cout << __cplusplus << std::endl;
fs::path src_dir("D:\\test");
std::set<string> dir_set;
for (fs::directory_iterator end, ite(src_dir); ite != end; ++ite)
{
if (!fs::is_directory(ite->path()))
dir_set.insert(ite->path().filename().string());
};
return 0;
}
5、to_string函数
string to_string (int val);
string to_string (long val);
string to_string (long long val);
string to_string (unsigned val);
string to_string (unsigned long val);
string to_string (unsigned long long val);
string to_string (float val);
string to_string (double val);
string to_string (long double val)
结语
如果您觉得该方法或代码有一点点用处,可以给作者点个赞,或打赏杯咖啡; ╮( ̄▽ ̄)╭ 如果您感觉方法或代码不咋地//(ㄒoㄒ)//,就在评论处留言,作者继续改进; o_O??? 如果您需要相关功能的代码定制化开发,可以留言私信作者; (????) 感谢各位童鞋们的支持! ( ′ ▽′ )ノ ( ′ ▽′)っ!!!
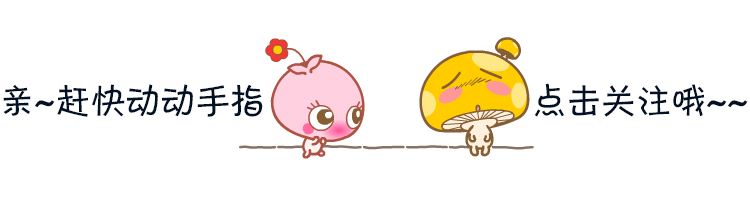
|