写TS比写JS多了一步就是编译。
在ts中声明变量时可以指定变量的类型
尚硅谷官方文档
一、安装并测试自动编译
1.命令行安装自动编译命令
tsc --init
2.修改配置中的输出js存储位置和关闭严格模式
"outDir": "./js",
"outDir": "./js",
3.启动监视,自动编译ts为js
启动监视任务: 终端 -> 运行任务 -> 监视tsconfig.json 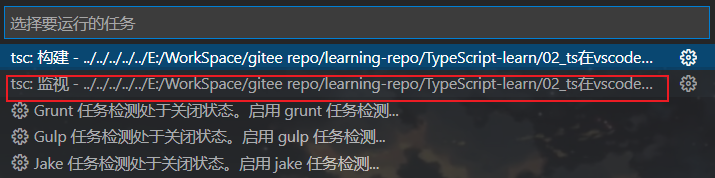
1.自动编译测试
1.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>自动编译成果</title>
</head>
<body>
<script src="./js/index.js"></script>
</body>
</html>
2.ts:
(() => {
function sayHello(name) {
return name + ',你好啊!'
}
let name = "通天教主"
console.log(sayHello(name))
})();
3.自动编译的js
(() => {
function sayHello(name) {
return name + ',你好啊!';
}
let name = "通天教主";
console.log(sayHello(name));
})();
结果: 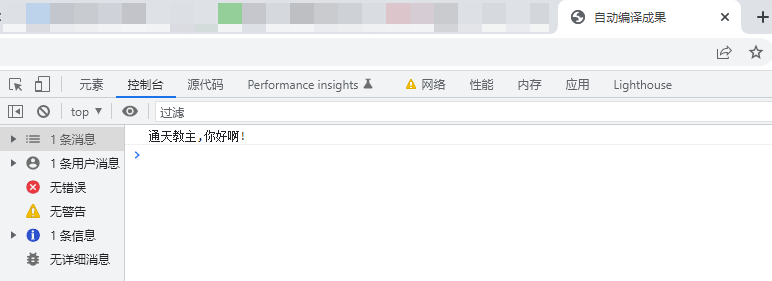
二、ts类型注解
约束函数和变量, 在对应变量加上限制:
变量:变量类型
ts:
(() => {
function sayHello(name: string) {
return name + ',你好啊!'
}
let name = "通天教主";
console.log(sayHello(name))
})();
三、ts接口
接口其实就是告诉我们一个类实现了哪些方法。
接口描述了类的公共部分,在 TS 里接口一般指这个变量拥有什么东西
(() => {
interface IPerson {
firstName: string
lastName: string
}
function showFullName(person: IPerson) {
return "在下" + person.lastName + '_' + person.firstName + '.';
}
let user: IPerson = {
lastName: '东方',
firstName: '不败'
}
console.log(showFullName(user))
})();

四、类
在自运行函数输出各个基本类型
(() => {
interface IPerson {
firstName: string
lastName: string
}
class Person {
firstName: string
lastName: string
fullName: string
constructor(lastName, firstName) {
this.firstName = firstName
this.lastName = lastName
this.fullName = this.lastName + '·' + this.firstName
}
}
function showFullName(person: IPerson) {
return person.lastName + '·' + person.firstName
}
let person = new Person("东方", '不败')
console.log(showFullName(person))
})()
五、基础类型
(() => {
let flag: boolean = true
console.log("布尔值 boolean")
console.log(flag)
console.log('--------------------------------------------------')
console.log("浮点数 number")
let num1: number = 10
let num2: number = 0b1010
let num3: number = 0o12
let num4: number = 0xa
console.log(num1)
console.log(num2)
console.log(num3)
console.log(num4)
console.log('--------------------------------------------------')
console.log("字符串 string")
let str1: string = "一切命运的馈赠"
let str2: string = "早已明码标价"
console.log(`${str1},${str2}.`)
let num: number = 1
let str: string = '命运的重量'
console.log(str + num)
})()
undefined 和 null
修改 tsconfig.json 如果关闭strictNullChecks ,则 null 和 undefined 两种类型是任意类型的子类型, 自编译运行不会报错
"strictNullChecks": false,
五.基础类型代码补充
str = undefined
num = null
console.log(str, num)
|