一,基础语法
1.1运行一个程序的步骤
-
创建项目 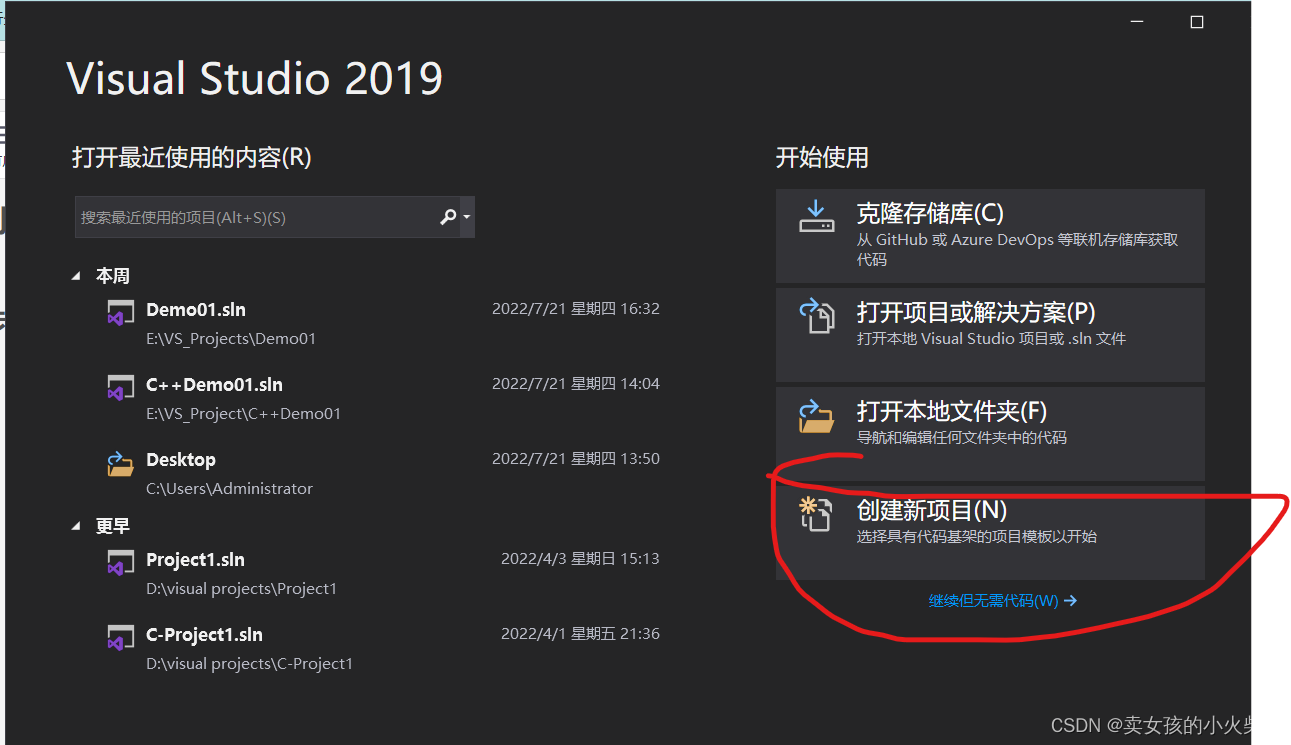 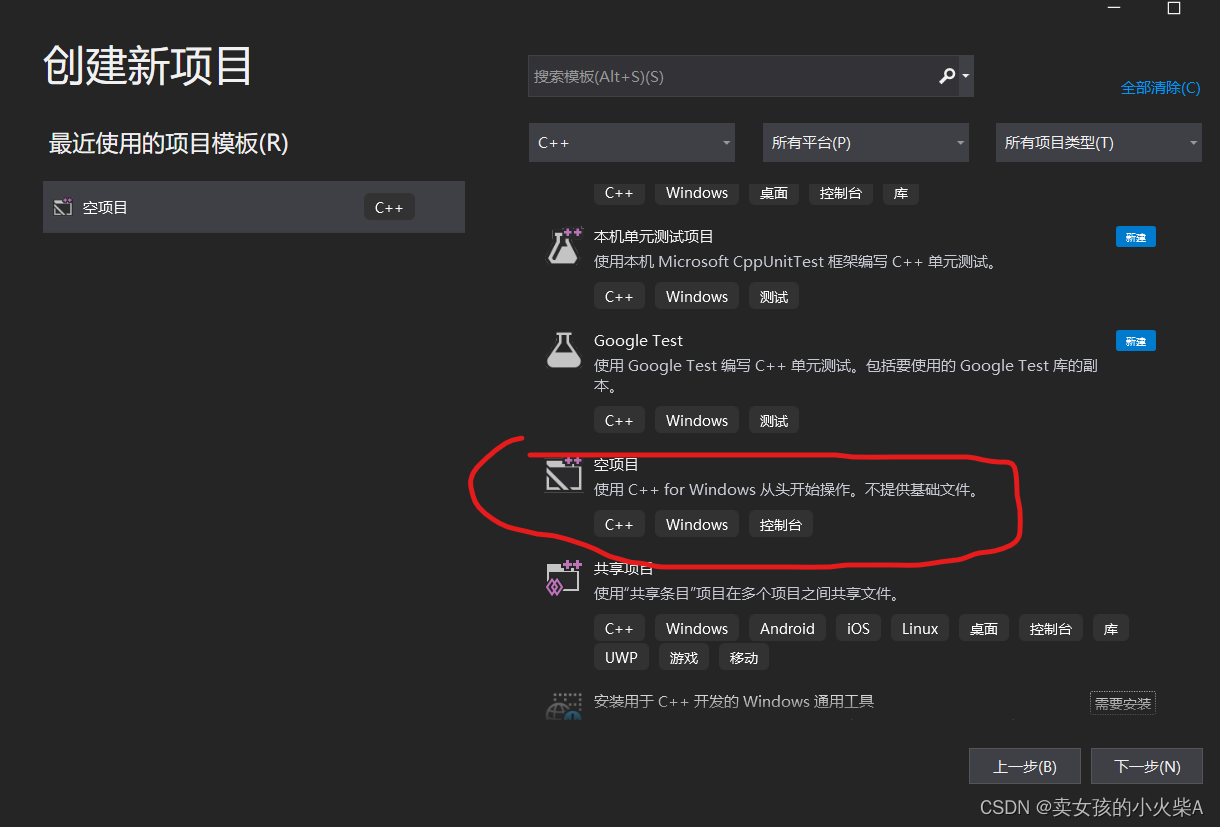 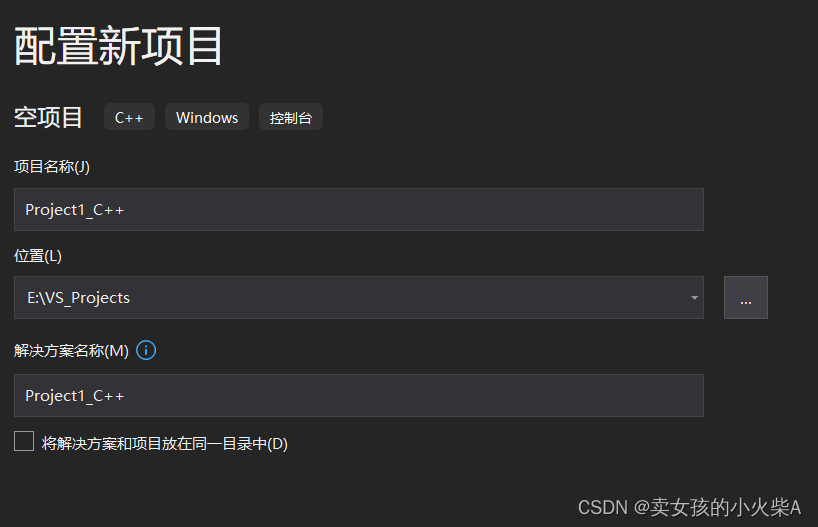 -
创建文件:源文件中添加新建项,在选中C++文件(.cpp) 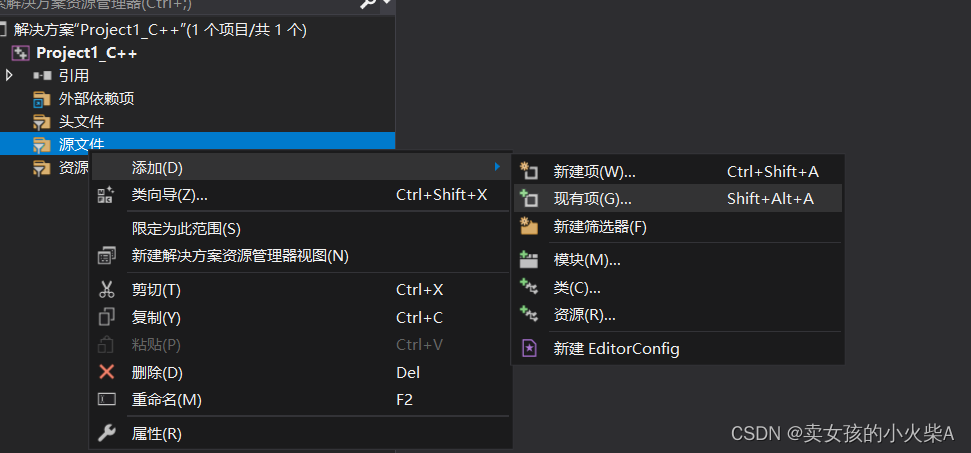 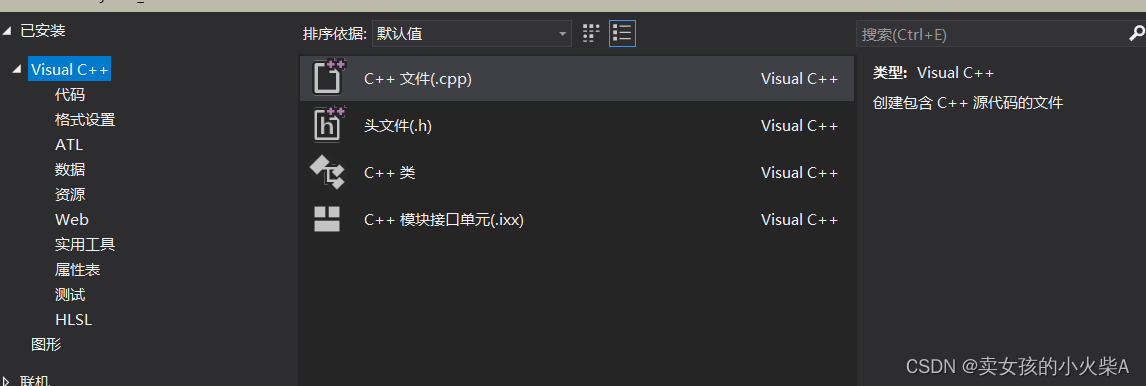 -
编写代码 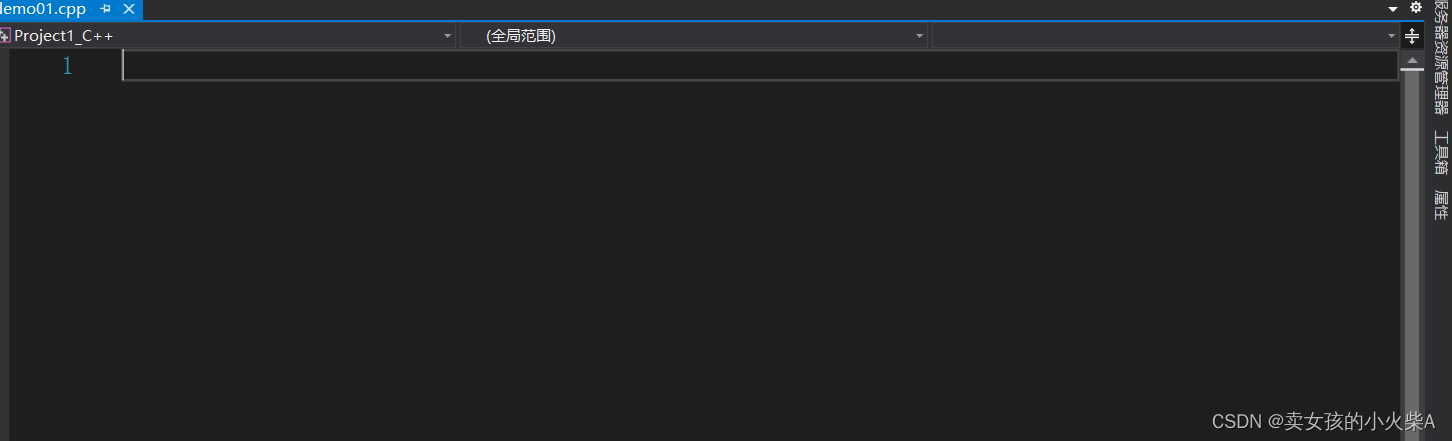 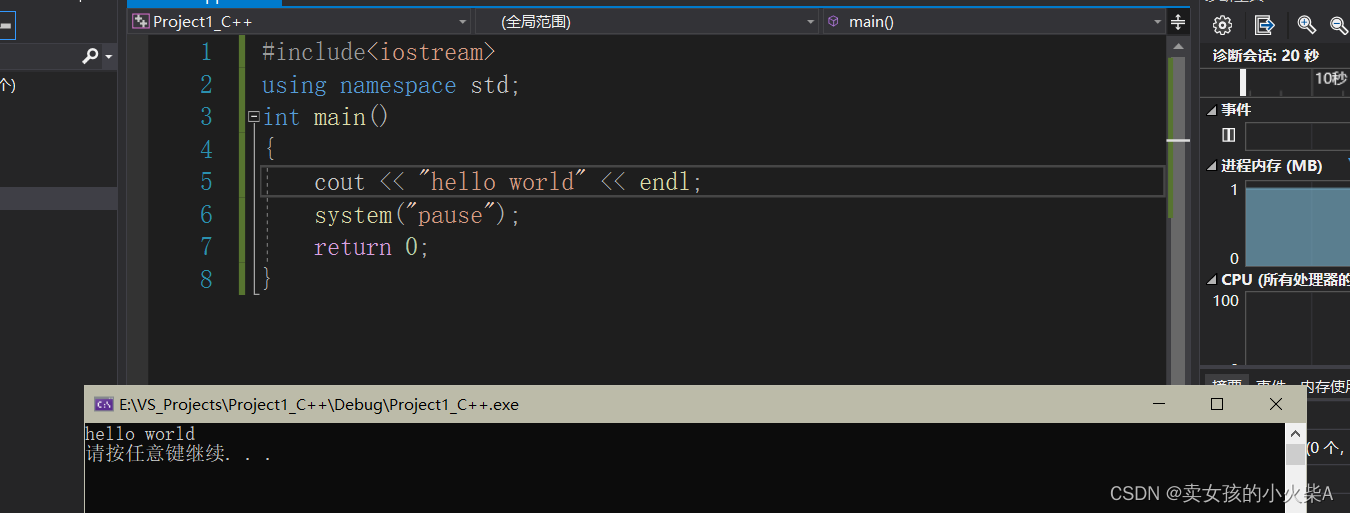
#include<iostream>
using namespace std;
int main()
{
cout << "hello world" << endl;
system("pause");
return 0;
}
- 运行程序
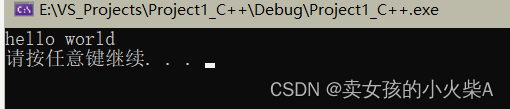
1.2注释
- 单行注释
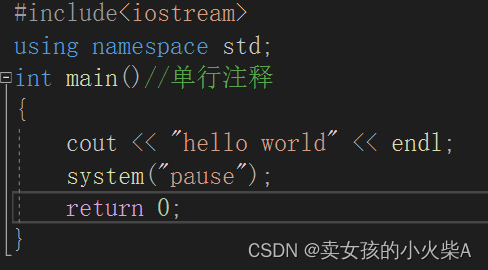
int main()
- 多行注释
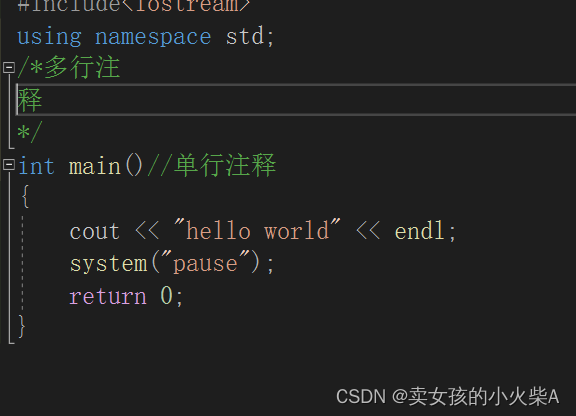
1.3变量
- 变量存在的意义:方便管理内存空间如将变量a指向某空间,操作时只需要使用a来操作,代替了16进制空间编号。
- 创建语法:数据类型 变量名=变量初始值
int a = 10;
1.4常量
- 用于记录程序中不可更改的数据
- 两种定义方法:
- (1)#define 常量名 常量值
- (2)const关键字修饰的变量(通常定义在函数体里面):const 数据类型 常量名 =常量值
#include<iostream>
using namespace std;
#define day 7
int main()
{
cout << "hello world" << endl;
int a = 10;
const int b = 5;
system("pause");
return 0;
}
1.5sizeof关键字
- 作用:统计数据类型/变量所占内存大小
- 语法:sizeof(数据类型/变量)
#include<iostream>
using namespace std;
#define day 7
int main()
{
cout << "hello world" << endl;
int a = 10;
const int b = 5;
cout << "变量a所占内存大小:" << sizeof(a) << endl;
system("pause");
return 0;
}
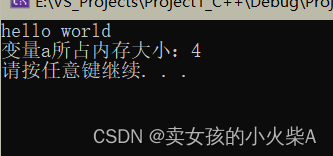
1.6C++输出语句endl与ends
- endl表示end of line此行输出结束并换行
- ends表示输出结束后空一格
#include<iostream>
using namespace std;
#define day 7
int main()
{
cout << "hello world" << ends;
cout << "变量a所占内存大小:" << sizeof(a) << endl;
int a = 10;
const int b = 5;
system("pause");
return 0;
}
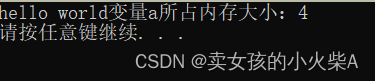
1.7数据类型
-
操作系统根据变量的数据类型来分配内存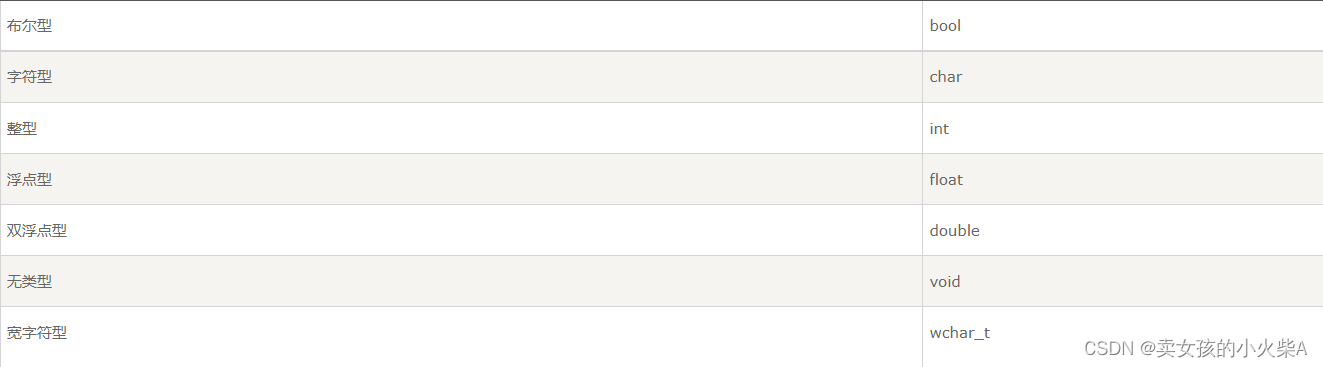 -
各种变量类型在内存中存储值时需要占用的内存 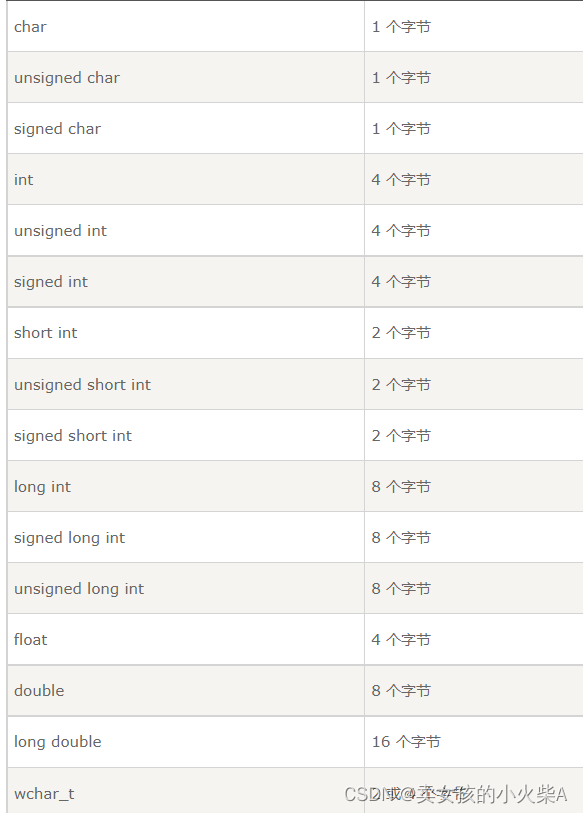 -
我们可以使用typedef来为已经存在的数据类型定义别名
typedef int f;
f a=3;
1.8枚举类型
- 枚举类型(enumeration)是C++中的一种派生数据类型,它是由用户定义的若干枚举常量的集合。
- enum 枚举名{
标识符[=整型常数], 标识符[=整型常数], … 标识符[=整型常数] } 枚举变量;
enum color {
red,
green,
blue
} c;
c = blue;
- 变量 c 的类型为 color。最后,c 被赋值为 “blue”。
|