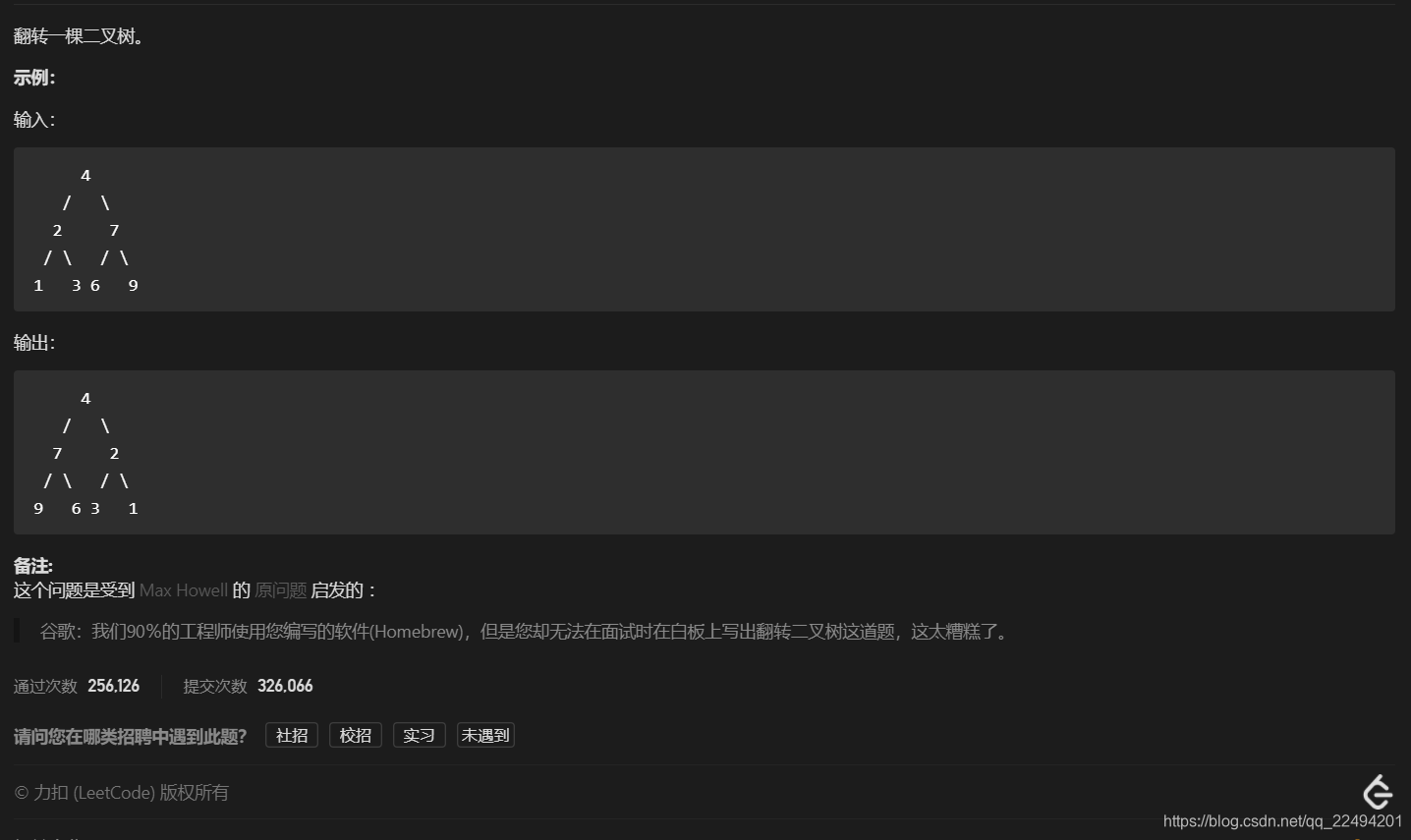 题解: 递归: 递归三部曲: 1、确定递归函数的参数和返回值 参数就是要传入结点的指针,返回值 按照题目要求 返回root结点指针 2、确定终止条件 当当前结点为空时,就返回 3、确定单层逻辑 前序遍历,先交换左右孩子结点,然后反转左子树,反转右子树
class Solution {
public:
TreeNode* invertTree(TreeNode* root) {
if(root == NULL){
return root;
}
swap(root->left,root->right);
invertTree(root->left);
invertTree(root->right);
return root;
}
};
深度优先遍历: 迭代:前序遍历
class Solution {
public:
TreeNode* invertTree(TreeNode* root) {
if(root == NULL){
return root;
}
stack<TreeNode*>st;
st.push(root);
while(!st.empty()){
TreeNode*node = st.top();
st.pop();
swap(node->left,node->right);
if(node->right){
st.push(node->right);
}
if(node->left){
st.push(node->left);
}
}
return root;
}
};
广度优先遍历,即层序遍历
class Solution {
public:
TreeNode* invertTree(TreeNode* root) {
queue<TreeNode*> que;
if(root!=NULL){
que.push(root);
}
while(!que.empty()){
int size = que.size();
for(int i = 0;i<size;i++){
TreeNode* node = que.front();
que.pop();
swap(node->left,node->right);
if(node->left){
que.push(node->left);
}
if(node->right){
que.push(node->right);
}
}
}
return root;
}
};
|