Java实现单链表+链表反转
Java实现单链表
import java.util.Iterator;
public class LinkList<T> implements Iterable<T> {
private Node head;
private int N;
public LinkList() {
this.head = new Node(null, null);
this.N = 0;
}
public void clear() {
this.head.next = null;
this.N = 0;
}
public boolean isEmpty() {
return N == 0;
}
public int length() {
return N;
}
public T get(int i) {
if (i < 0 || i >= N) {
throw new RuntimeException("位置不合法");
}
Node node = head.next;
for (int index = 0; index < i; index++) {
node = node.next;
}
return (T) node.item;
}
public void insert(T t) {
Node node = new Node(t, null);
Node node1 = head;
while (node1.next != null) {
node1 = node1.next;
}
node1.next = node;
N++;
}
public void insert(int i, T t) {
if (i < 0 || i >= N) {
throw new RuntimeException("位置不合法");
}
Node preNode = head;
for (int index = 0; index < i - 1; index++) {
preNode = preNode.next;
}
Node curr = preNode.next;
Node newNode = new Node(t, curr);
preNode.next = newNode;
N++;
}
public T remove(int i) {
if (i < 0 || i >= N) {
throw new RuntimeException("位置不合法");
}
Node node = head;
for (int index = 0; index < i - 1; index++) {
node = node.next;
}
Node curr = node.next;
node.next = curr.next;
N--;
return (T) curr;
}
public int indexOf(T t) {
Node node = head;
for (int index = 0; node.next != null; index++) {
node = node.next;
if (node.item == t) {
return index;
}
}
return -1;
}
@Override
public Iterator<T> iterator() {
return new SIterator<T>();
}
private class SIterator<T> implements Iterator<T> {
private Node node;
public SIterator() {
this.node = head;
}
@Override
public boolean hasNext() {
return node.next != null;
}
@Override
public T next() {
node = node.next;
return (T) node.item;
}
}
private class Node {
public T item;
public Node next;
public Node(T item, Node next) {
this.item = item;
this.next = next;
}
}
}
链表反转
需求:
原链表中数据为:1->2->3->4
反转后链表中元素为:4->3->2->1
实现方式:使用递归完成反转,递归反转就是从原链表的第一个存储数据的结点开始,依次递归调用反转每一个结点,直到把最后一个结点反转完毕,整个链表就反转完毕了。
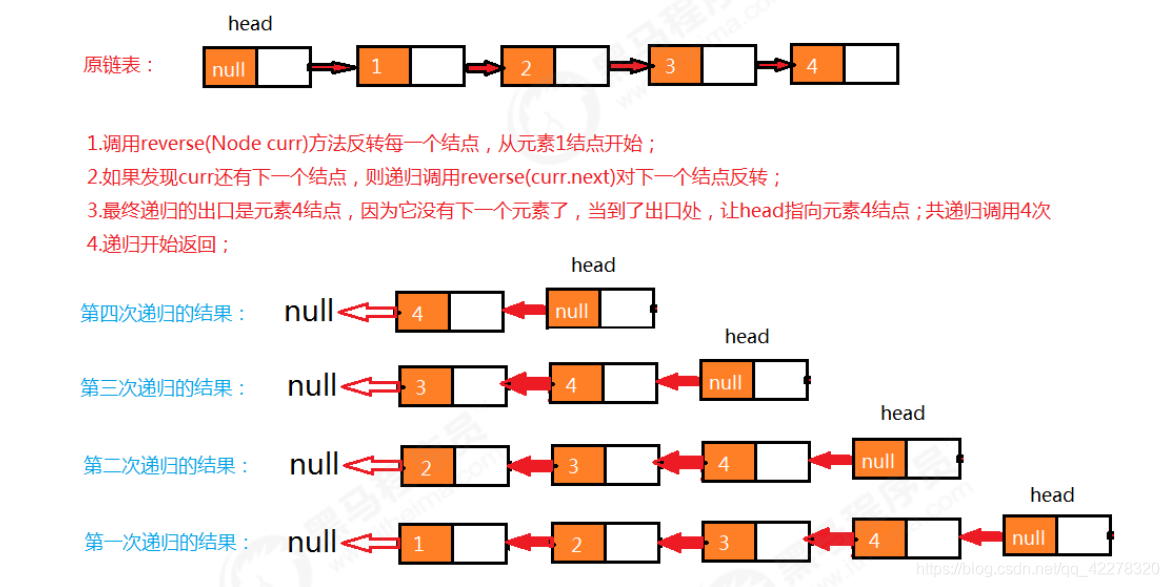
public void reverse(){
if (N==0){
return;
}
reverse(head.next);
}
public Node reverse(Node curr){
if (curr.next==null){
head.next = curr;
return curr;
}
Node pre = reverse(curr.next);
pre.next = curr;
curr.next = null;
return curr;
}
|