强烈推荐,刷PTA的朋友都认识一下柳神–PTA解法大佬
本文由参考于柳神博客写成
柳神的CSDN博客,这个可以搜索文章
柳神的个人博客,这个没有广告,但是不能搜索
还有就是非常非常有用的 算法笔记 全名是
算法笔记 上级训练实战指南 //这本都是PTA的题解算法笔记
PS 今天也要加油鸭
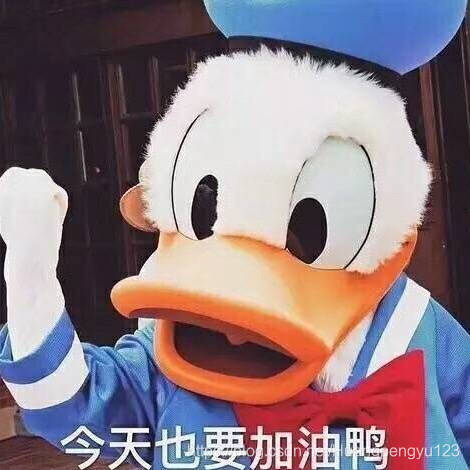
题目原文
An AVL tree is a self-balancing binary search tree. In an AVL tree, the heights of the two child subtrees of any node differ by at most one; if at any time they differ by more than one, rebalancing is done to restore this property. Figures 1-4 illustrate the rotation rules.
Now given a sequence of insertions, you are supposed to output the level-order traversal sequence of the resulting AVL tree, and to tell if it is a complete binary tree.
Input Specification:
Each input file contains one test case. For each case, the first line contains a positive integer N (≤ 20). Then N distinct integer keys are given in the next line. All the numbers in a line are separated by a space.
Output Specification:
For each test case, insert the keys one by one into an initially empty AVL tree. Then first print in a line the level-order traversal sequence of the resulting AVL tree. All the numbers in a line must be separated by a space, and there must be no extra space at the end of the line. Then in the next line, print YES if the tree is complete, or NO if not.
Sample Input 1:
5
88 70 61 63 65
Sample Output 1:
70 63 88 61 65
YES
Sample Input 2:
8
88 70 61 96 120 90 65 68
Sample Output 2:
88 65 96 61 70 90 120 68
NO
生词如下:
AVL 平衡二叉树 Adelson-Velsky and Landis Tree
insertions 插入
思路如下:
这题算是比较标准的套模板的题目了
主要就考察,如何构建一颗AVL树(平衡二叉树),只要会构建这题基本就解决了.
就是套用公式吧.
借鉴了算法笔记的代码如下:
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
struct node {
int v, height;
node* lchild, * rchild;
};
node* newNode(int v) {
node* Node = new node;
Node->v = v;
Node->height = 1;
Node->lchild = Node->rchild = nullptr;
return Node;
}
int getHeight(node* root) {
if (root == nullptr) return 0;
return root->height;
}
int getBalanceFactor(node* root) {
return getHeight(root->lchild) - getHeight(root->rchild);
}
void updateHeight(node* root) {
root->height = max(getHeight(root->lchild), getHeight(root->rchild)) + 1;
}
void L(node*& root) {
node* temp = root->rchild;
root->rchild = temp->lchild;
temp->lchild = root;
updateHeight(root);
updateHeight(temp);
root = temp;
}
void R(node*& root) {
node* temp = root->lchild;
root->lchild = temp->rchild;
temp->rchild = root;
updateHeight(root);
updateHeight(temp);
root = temp;
}
void insert(node*& root, int v) {
if (root == nullptr) {
root = newNode(v);
return;
}
if (v < root->v) {
insert(root->lchild, v);
updateHeight(root);
if (getBalanceFactor(root) == 2) {
if (getBalanceFactor(root->lchild) == 1) {
R(root);
}
else if (getBalanceFactor(root->lchild) == -1) {
L(root->lchild);
R(root);
}
}
}
else {
insert(root->rchild, v);
updateHeight(root);
if (getBalanceFactor(root) == -2) {
if (getBalanceFactor(root->rchild) == -1) {
L(root);
}
else if (getBalanceFactor(root->rchild) == 1) {
R(root->rchild);
L(root);
}
}
}
}
node* Create(int data[], int n) {
node* root = nullptr;
for (int i = 0; i < n; ++i) {
insert(root, data[i]);
}
return root;
}
void LayerOrder(node *root) {
queue<node > Q;
node T = *root;
Q.push(*root);
while (!Q.empty()) {
T = Q.front();
Q.pop();
if (T.v != root->v) printf(" ");
printf("%d", T.v);
if (T.lchild) {
Q.push(*T.lchild);
}
if (T.rchild) {
Q.push(*T.rchild);
}
}
}
bool isCompleteTree(node* root) {
queue<node*>q;
q.push(root);
bool flag = false;
while (!q.empty()) {
auto T = q.front();
q.pop();
if (T == nullptr) {
flag = true;
continue;
}
if (flag) return false;
q.push(T->lchild);
q.push(T->rchild);
}
return true;
}
int main(void) {
node* root = nullptr;
int N,t;
scanf("%d", &N);
for (int i = 0; i < N; ++i) {
scanf("%d", &t);
insert(root, t);
}
LayerOrder(root);
isCompleteTree(root);
if (isCompleteTree(root)) {
printf("\nYES");
}
else {
printf("\nNO");
}
return 0;
}
这题可以算是模板了吧.我个人感觉还是比较经典的.
感觉AVL的代码都差不多,一样的代码,一样的考试.要求会套用.
|