二叉树的排序
把第一个数作为根结点,比根结点大的数放右边,比根结点小的数放左边 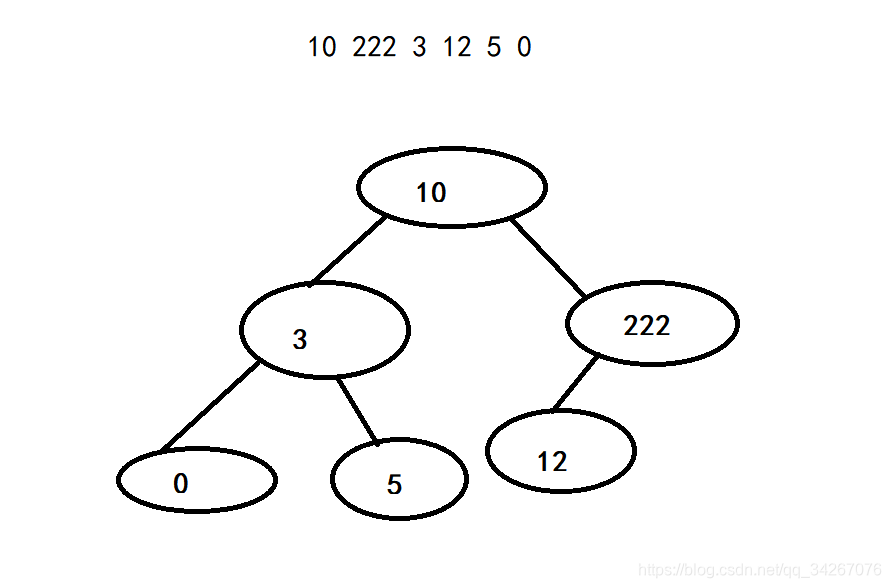 中序排列顺序(左根右):0 3 5 10 10 222 前序排列顺序(根左右):10 3 0 5 222 12 后续排列顺序(左右根):0 5 3 12 222 10 递归实现 首先创建结点类用于排序等功能的实现 public class Node {
private int data;
private Node leftNode;
private Node rightNode;
public Node(int data) {
this.data = data;
}
@Override
public String toString() {
return "Node{" +
"data=" + data +
'}';
}
public void add(Node node){
if(node == null){
return;
}
if(node.data < this.data){
if(this.leftNode == null){
this.leftNode = node;
}else{
this.leftNode.add(node);
}
}else{
if(this.rightNode == null){
this.rightNode = node;
}else{
this.rightNode.add(node);
}
}
}
public void midSort(){
if(this.leftNode != null){
this.leftNode.midSort();
}
System.out.println(this);
if(this.rightNode != null){
this.rightNode.midSort();
}
}
public void preSort(){
System.out.println(this);
if(this.leftNode != null){
this.leftNode.preSort();
}
if(this.rightNode != null){
this.rightNode.preSort();
}
}
public void postSort(){
if(this.leftNode != null){
this.leftNode.postSort();
}
if(this.rightNode != null){
this.rightNode.postSort();
}
System.out.println(this);
}
}
创建二叉树类用于控制结点 public class MyTree {
private Node root;
public void add(Node node){
if(root == null){
root = node;
}else{
root.add(node);
}
}
public void midSort(){
if(root == null){
System.out.println("根结点为空");
}else{
root.midSort();
}
}
public void preSort(){
if(root == null){
System.out.println("根结点为空");
}else{
root.preSort();
}
}
public void postSort(){
if(root == null){
System.out.println("根结点为空");
}else{
root.postSort();
}
}
}
测试类用于检验: public class Text {
public static void main(String[] args) {
int[] arr =new int[]{10,222,3,12,5,0};
MyTree myTree = new MyTree();
for(int i=0 ;i < arr.length ;i++){
myTree.add(new Node(arr[i]));
}
myTree.midSort();
System.out.println("-------------------------");
myTree.preSort();
System.out.println("-------------------------");
myTree.postSort();
}
}
非递归实现
public void preSort2(Node root){
if(root == null)
return;
Stack<Node> stack = new Stack<>();
stack.push(root);
while(!stack.isEmpty()){
Node node = stack.pop();
System.out.println(node.data);
if(node.rightNode != null){
stack.push(node.rightNode);
}
if(node.leftNode != null){
stack.push(node.leftNode);
}
}
}
public void midSort2(Node root){
if(root == null)
return;
Stack<Node> stack = new Stack<>();
Node cur = root;
while(!stack.isEmpty() || cur != null){
while (cur != null){
stack.push(cur);
cur = cur.leftNode;
}
Node node = stack.pop();
System.out.println(node.data);
if(node.rightNode != null){
cur = node.rightNode;
}
}
}
public void postSort2(Node root){
if(root == null)
return;
Stack<Node> stack = new Stack<>();
Stack<Node> stack2 = new Stack<>();
stack.push(root);
while(!stack.isEmpty()){
Node node = stack.pop();
stack2.push(node);
if(node.leftNode != null){
stack.push(node.leftNode);
}
if(node.rightNode != null){
stack.push(node.rightNode);
}
}
while (!stack2.isEmpty()){
System.out.println(stack2.pop().data);
}
}
|