链表实现反转的两种方式
要求:给出单链表的头节点 head ,请反转链表,并返回反转后的链表。
递归方式
由题目要求,我们可以画出链表转换前与转换后的图形; 转换前: 
转换后: 
疑问来了,我们如何通过递归来实现此功能呢? 在这里,我们单独对节点5与节点4进行分析:
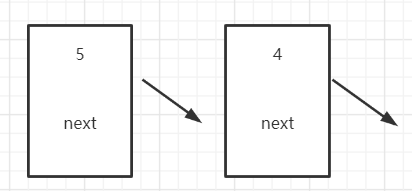
- 首先,如果我们需要将节点4的指针由原来的指向节点3改为指向节点5
- 根据题目,因为我们已知节点5,所以可以得到节点4的指针为:head.next.next
- 此时,我们只需将节点4的指针指向节点5即可:head.next.next=head.
- 最后,我们将节点5指向节点4的指针改为指向null:head.next=null.
具体实现如下图: 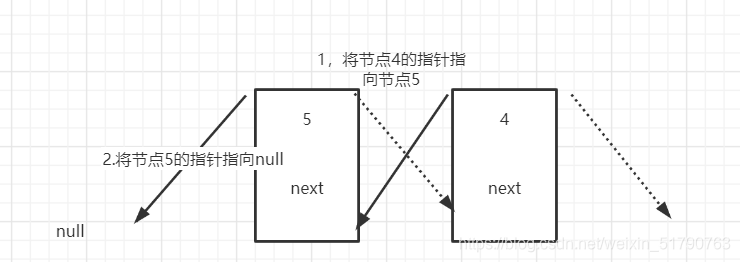 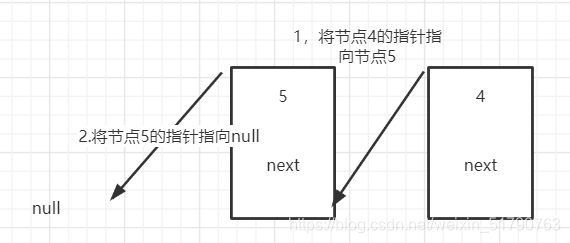 此时,我们就发现了规律:从节点5开始可以将节点4进行反向装换,那从节点4开始就可以将节点4与节点3进行转换。因此,我们只需通过递归的方法,从倒数第二个节点开始转换,就可以完成所有节点的反向转换。 以下为代码演示
public ListNode reverseList(ListNode head){
if(head==null||head.next==null)return head;
ListNode newHead=reverseList(head.next);
head.next.next=head;
head.next=newHead;
return newHead;
}
迭代方式
图解: 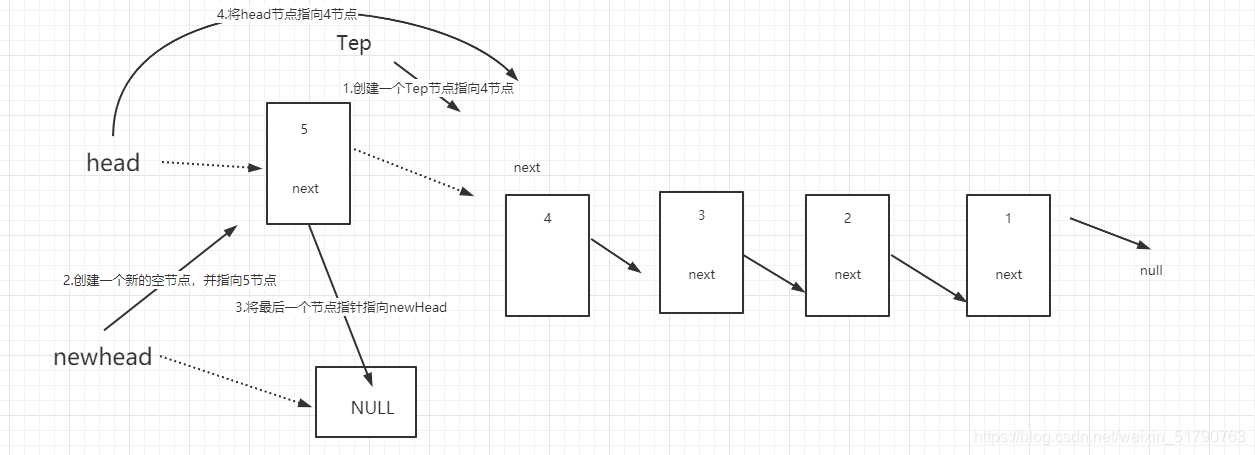
(在途中第二步与第四步的描述卸载了一起) 第一步:创建一个新的头节点tep指向4节点(为下一步做准备) “ListNode tep=head.next” 第二步:再次创建一个新的空节点 ListNode newhead=null 第三步:将节点5指向newhead(若没有上一步,后面的4,3,2,1节点均会被处理回收掉) head=newhead 第四步:再将newhead指向5节点 newhead=head 第五步:将head指向4节点(因为已进有了tep节点指向4节点且,因此代码如下) head=tep 即得到结果如下图: 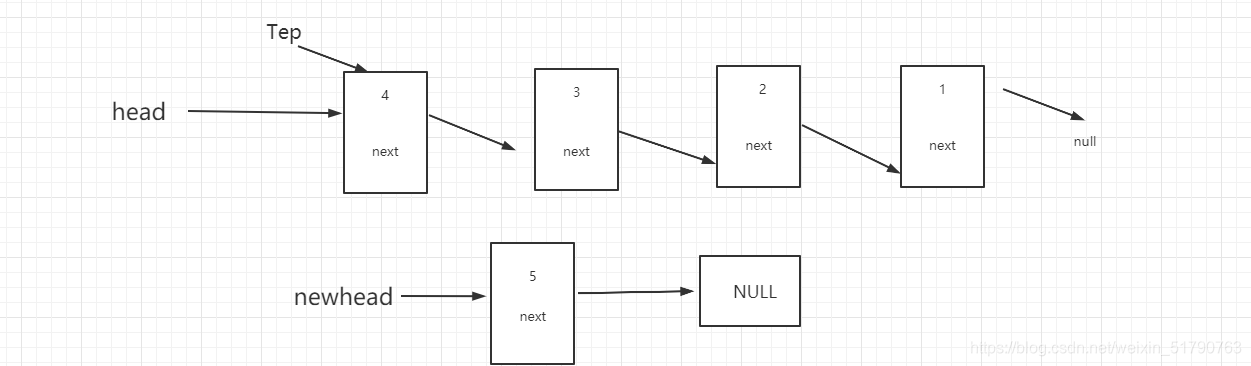 再来一次: 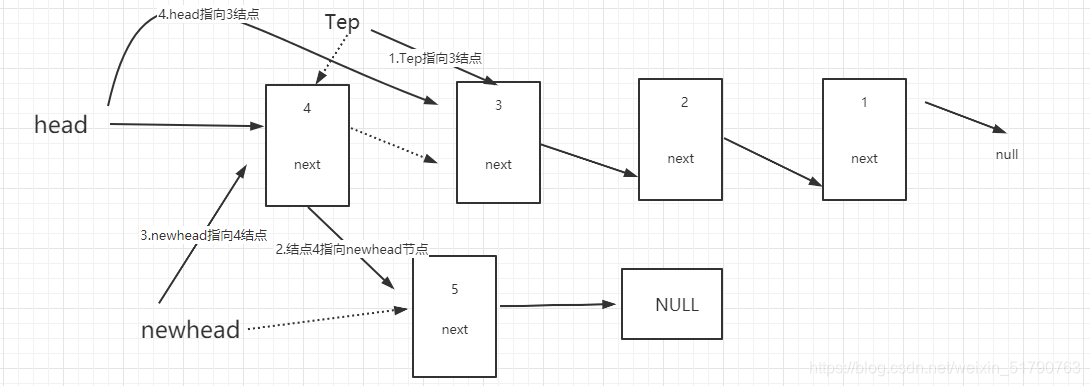
第一步:创建一个新的头节点tep指向3节点(为下一步做准备) “ListNode tep=head.next” 第二步:将节点4指向节点5(若没有上一步,后面的3,2,1节点均会被处理回收掉) head=newhead 第三步:将newhead指向4节点 newhead=head 第四步:将head指向3节点 head.next=tep 结果如下图: 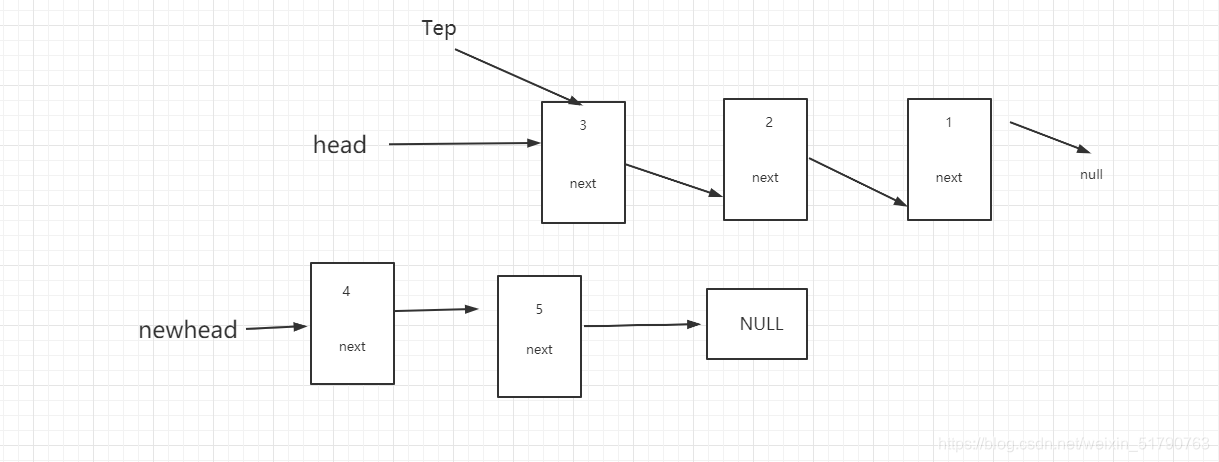 依次进行循环,即可将该链表反转,最后返回newhead; 全部代码如下:
public ListNode reverseList(ListNode head){
if(head==null||head.next==null)return head;
ListNode newhead=null
while(head!=null){
ListNode tep=head.next
head.next=newhead;
newhead=head;
head=tep;
}
return newhead;
}
|