前言
利用动态数组实现了循环队列,这是静态的队列,缺点是需要预设大小,当队列满时,无法再插入新的数据,只有等队头的数据被取走以后才能往队列放入新的数据。 除了动态数组分配实现的队列之外,还可以使用链表实现队列,这种方式动态创建节点需要的内存,当有新的数据节点要加入时,才去申请内存空间,不需要预设大小,整个队列需要的内存空间不需要连续,并且插入删除更容易实现。但是同时也带来存取速度慢的缺点,操作也比数组的方式更加复杂。 其实用链表实现队列的方式十分简单,只需要在单链表的基础上,增加一个尾指针即可。因为队列的特点是“先进先出”,因此我们只需要在一头一尾两个指针,就可以快速地在队头取出数据,在队尾插入数据。
提示:以下是本篇文章正文内容,下面案例可供参考
一、实现过程
1、定义结构体结构
typedef struct Queue_Node {
QUEUE_TYPE data;
struct Queue_Node *next;
}QueueNode;
2、创建一个结构体指针
- ①、创建头指针:QueueNode *head;
- ②、创建尾指针:QueueNode *tail;
QueueNode *head;
QueueNode *tail;
3、数据入队列
- ①、创建一个结构体指针 new_node
- ②、通过malloc动态分配地址块,将该地址块的首地址传给 new_node
- ③、通过判断 new_node == NULL 查看 malloc 是否分配内存空间成功,为空则表示分配失败,打印错误信息
- ④、将数据赋值给 new_node 指针指向的data域
- ⑤、通过判断 head == NULL 确定该队列是否为
- 1.1)队列为空则将头指针 head 和尾指针 tail 都指向新节点【new_node 指向的内存空间】
- 2.1)队列不为空则将 “ 尾指针 tail 指向的内存空间中的next域 ” 指向 “ new_node 指向的内存空间 ”
- 2.2)将尾指针 tail 指向 “ new_node 指向的内存空间 ”【尾指针指向新节点】
void enqueue(QUEUE_TYPE value) {
QueueNode *new_node;
new_node = (QueueNode *)malloc(sizeof(QueueNode));
if (new_node == NULL)
perror("malloc fail\n");
new_node->data = value;
if (head == NULL) {
head = new_node;
tail = new_node;
} else {
tail->next = new_node;
tail = new_node;
}
}
4、数据出队列
- ①、判断该队列是否为空
- ②、创建一个结构体指针 front_node
- ③、将 “ 头指针指向的内存空间 ” 传给 “ front_node ”,使得 front_node 指向第一个节点
- ④、将 “ front_node 指向内存空间中的next的值 ”【第二个节点的首地址】传给 头指针 head,使得头指针 head 指向第二个节点
- ⑤、将 front_node 指向的内存空间通过 free() 释放掉【释放第一个节点的内存空间】
- ⑥、如果这时 头指针 head 指向为空,说明该队列已为空,将尾指针 tail 也设置为指向为空
void dequeue(void) {
assert(!is_empty());
QueueNode *front_node;
front_node = head;
head = front_node->next;
free(front_node);
if (head == NULL)
tail = NULL;
}
5、判断队列是否为空
- ①、在队列中,头指针 head 指向为空,则说明该队列为空,所以只需要返回 head == NULL 的结果
- 返回值为1:表明队列为空
- 返回值为0:说明队列不为空
int is_empty(void) {
return head == NULL;
}
6、获取队列第一个数据
- ①、判断队列是否为空
- ②、返回头指针 head 所指向 内存空间 中的 data域数据
QUEUE_TYPE get_head(void) {
assert(!is_empty());
return head->data;
}
7、获取队列最后一个数据
- ①、判断队列是否为空
- ②、返回尾指针 tail 所指向 内存空间 中的 data域数据
QUEUE_TYPE get_tail(void) {
assert(!is_empty());
return tail->data;
}
8、清空队列数据
- ①、只要当前队列不为空,就逐个对数据进行出队列操作,直到队列为空
void empty_queue(void) {
while (!is_empty())
dequeue();
}
二、具体代码
#include <stdio.h>
#include <string.h>
#include <malloc.h>
#include <assert.h>
#define QUEUE_TYPE int
typedef struct Queue_Node {
QUEUE_TYPE data;
struct Queue_Node *next;
}QueueNode;
QueueNode *head;
QueueNode *tail;
int is_empty(void) {
return head == NULL;
}
void enqueue(QUEUE_TYPE value) {
QueueNode *new_node;
new_node = (QueueNode *)malloc(sizeof(QueueNode));
if (new_node == NULL)
perror("malloc fail\n");
new_node->data = value;
if (head == NULL) {
head = new_node;
tail = new_node;
} else {
tail->next = new_node;
tail = new_node;
}
}
void dequeue(void) {
assert(!is_empty());
QueueNode *front_node;
front_node = head;
head = front_node->next;
free(front_node);
if (head == NULL)
tail = NULL;
}
QUEUE_TYPE get_head(void) {
assert(!is_empty());
return head->data;
}
QUEUE_TYPE get_tail(void) {
assert(!is_empty());
return tail->data;
}
void empty_queue(void) {
while (!is_empty())
dequeue();
}
void print(void) {
printf("List data: ");
if (is_empty()) {
printf("NULL\n");
} else {
QueueNode *node;
node = head;
while (node) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
printf("front data: %d\t", get_head());
printf("tail data: %d\n", get_tail());
}
printf("\n");
}
int main(int argc, char **argv) {
print();
printf("Data queuing\n");
enqueue(10); enqueue(8); enqueue(6);
enqueue(4); enqueue(2); enqueue(0);
print();
printf("Data out of queue\n");
dequeue(); dequeue();
print();
printf("Data queuing\n");
enqueue(1); enqueue(3); enqueue(5);
print();
printf("Empty queue\n");
empty_queue();
print();
}
三、测试结果
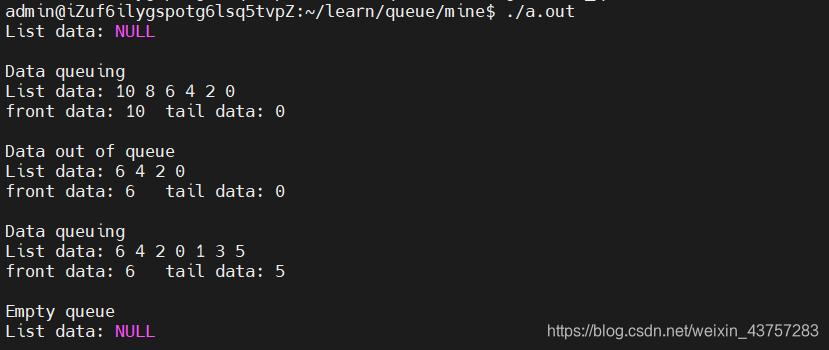
总结
以上是对链表实现队列的一些理解,如有写的不好的地方,还请各位大佬不吝赐教。
|