个人比较菜,只会瞎写一写bug,莫怪莫怪。
1.1数据结构杂论
数据结构是计算机必学课程,其可以提高效率
目前有三类:线性结构(数组,栈,链表),树结构(二叉树,AVL,线段树,哈夫曼树),图结构(邻接表,邻接矩阵)
数据库一般分装好直接使用SQL语言使用,哈希表,等完成数据库
哈夫曼树,.rar,.png,MP3,MP4,均使用到数据结构,tried的工作原理
图论算法DFS深度算法使用栈;BFS广度算法,使用队列
数据结构+算法=程序
使用Java语言(完全面向对象)
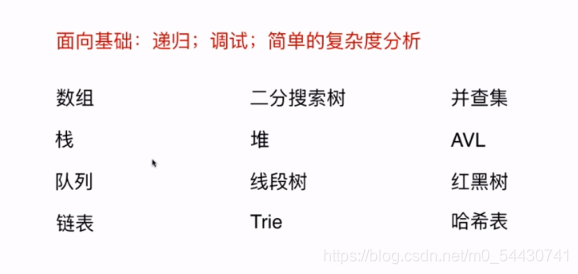
?
2.1数组
概念:把数据码排成一排进行存放
public class Main{
? ?public static void main(String[] args)
? {int[] arr=new int[10]
? }
}
2.1.1二次封装,数组类
public class Array
{
private int[] data;
private int size;
? ?//构造函数,传入数组的容量capacity构造Array
public Array(int capacity)
{
data = new int [capacity];
size = 0;
}
/*
无参数的构造函数,默认容量capacity为10
*/
public Array()
{
? ?this(capacity:10)}
}
//获取数组中的元素个数
public int getsize()
{return size;}
//获取数组容量
public int getCapacity()
{
? ?return data.length;
}
//返回数组是否为空
public boollean isempty()
{return ziae = 0;}
}
2.1.2数组的元素添加
1、数组末尾添加元素
//前方与上文相同
//在最后添加元素
public void addLast(int e)
{
if ( size == data.length)
? ? ? ?throw new illegalArgumentException("AddLast filed.Array is full")
? ?data[size] = e;
? ?size++;
? ?//data[size++]=e;
} ? ?
}
2、向指定位置添加
从后往前挪,防止前方覆盖后方,直到要添加位置
//在最前添加元素
public void addFirst(int e){
? ?add(index:0,e);
}
?
//在最后添加元素
public void addLast(int e)
{
?/* if ( size == data.length)
? ? ? ?throw new illegalArgumentException("AddLast filed.Array is full.");
? ?data[size] = e;
? ?size++;
? ?//data[size++]=e;
*/
add(size,e);
} ? ?
?
//在指定为index位置添加元素e
public void add(int index,int e)
{if (size == data.length)
throw new illegalArgumentException("AddLast filed.Array is full.");
if (index<0||index >size)
? ?throw new illegalArgumentException("Add faild.");
for (int i=size-1;i >= index ;i--)
{data[i+1]=data[i];
size++;
}
}
}
2.2查询,修改,搜索和删除元素
public static void main(String[] args)
{
? ?Array arr=new Array(capacity:20);
? ?for (int i=0;i<10;i++)
? ? ? ?arr.addLast(i);
? ? System.out.println(arr);
? ?arr.addFirst(e:-1);
? ?System.out.println(arr);
}
//获取索引位置元素
public int get(int index)
{
return data[index]
if (index<0||index>=size)
throw new illegalArgumentException("Add faild.");
}
//修改index索引位置的元素为e
public void set (int index,int e)
{
if (index<0||index>=size)
throw new illegalArgumentException("Add faild.");}
//对应索引,若不存在为-1 ? ? ? ? ? ? ? ? ? ? ? ? ? ?
public boolean contains(int e){
? ? ?for (int i=0;i<size;i++)
? ? ? ? ?if (data[i]==e)
? ? ? ? { ? ?return i;}
? ? ? ? return -1;
} ? ?
//删除指定位置
public int remove(int index){
if (index<0||index>=size)
throw new illegalArgumentException("Add faild.";)
int ret =data[index];
?for (int i=0;i<size;i++)
data[i-1]=data[i];
size--;
return ret;
}
public int removefirst(){return remove(0);}
public int removelast(){return remove(size-1);}
public void removeElement(int e){
? ?int index=find(e);
? ?if (index!=-1)
? ? ? ?remove(index);//只删除一个
}
?
?
?
|