定义
栈(stack)是一个先入后出的有序列表。 栈是限制线性表中元素的插入和删除只能在线性表的同一段进行的一种特殊线性表。允许插入和删除的一端,称为栈顶(Top),另一端为固定的一端,称为栈底(Bottom)。 根据栈的定义可知,最先放入栈中元素在栈底,最后放入的元素在栈顶,而删除元素相反,最后放入的元素最先删除,最先放入的元素最后删除。 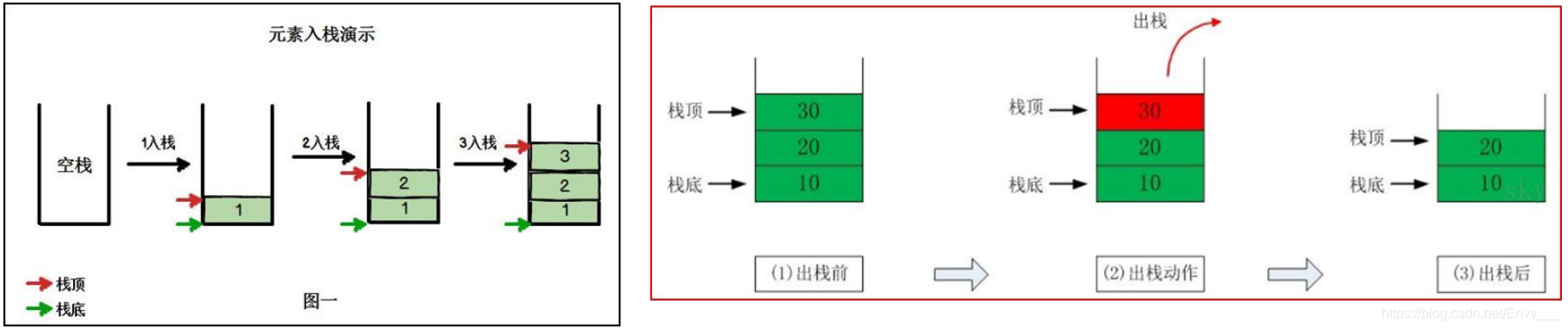 应用场景:
- 子程序的调用:在跳往子程序前,会先将下个指令的地址存到堆栈中,直到子程序执行完后再将地址取出,以回到原来的程序中。
- 处理递归调用:和子程序的调用类似,只是除了储存下一个指令的地址外,也将参数、区域变量等数据存入堆栈中。
- 表达式的转换[中缀表达式转后缀表达式]与求值(实际解决)。
- 二叉树的遍历。
- 图形的深度优先(depth 一 first)搜索法。
数组模拟栈
class ArrayStack {
private int maxSize;
private int[] stack;
private int top = -1;
public ArrayStack(int maxSize) {
this.maxSize = maxSize;
stack = new int[this.maxSize];
}
public boolean isFull() {
return top == maxSize - 1;
}
public boolean isEmpty() {
return top == -1;
}
public void push(int value) {
if (isFull()) {
System.out.println("栈满");
return;
}
stack[++top] = value;
}
public int pop() {
if (isEmpty())
throw new RuntimeException("栈空,没有数据");
return stack[top--];
}
public void list() {
if (isEmpty()) {
System.out.println("栈空");
return;
}
for (int i = top; i >= 0; i--) {
System.out.printf("stack[%d]=%d\n", i, stack[i]);
}
}
}
链表模拟栈
class LinkedListStack{
private int maxSize;
private ListNode head;
private int top = -1;
public LinkedListStack(int maxSize) {
this.maxSize = maxSize;
}
public boolean isFull() {
return top == maxSize - 1;
}
public boolean isEmpty() {
return top == -1;
}
public void push(ListNode listNode){
if (isFull()) {
System.out.println("栈满");
return;
}
top++;
listNode.next = head;
head = listNode;
}
public ListNode pop(){
if (isEmpty())
throw new RuntimeException("栈空,没有数据");
top--;
ListNode temp = head;
head = head.next;
return temp;
}
public void list(){
if(isEmpty())
throw new RuntimeException("栈空,没有数据");
ListNode temp = head;
while(temp != null){
System.out.println(temp);
temp = temp.next;
}
}
}
class ListNode{
public int num;
public ListNode next;
public ListNode(int num) {
this.num = num;
}
@Override
public String toString() {
return "ListNode{" +
"num=" + num +
'}';
}
}
|