BFS 的把问题抽象成图,从一个点开始,向四周开始扩散。一般来说,我们写 BFS算法都是用「队列」这种数据结构,每次将一个节点周围的所有节点加入队列。
BFS 相对 DFS 的最主要的区别是:BFS 找到的路径一定是最短的,但代价就是空间复杂度比 DFS大很多。
模板(改编自LABULADONG的算法小抄)
int BFS(Node start, Node target) {
Queue<Node> q;
Set<Node> visited;
q.push(start);
visited.add(start);
int step = 0;
while (!q . empty()) {
int sz = q.size();
for (int i = 0; i < sz; i++) {
Node cur = q.front();
q.pop();
if (cur is target)
return step;
for (Node x : cur.adj())
if (x not in visited) {
q.push(x);
visited.add(x);
}
}
step++;
}
}
leetcode111. 二叉树的最小深度
给定一个二叉树,找出其最小深度。 最小深度是从根节点到最近叶子节点的最短路径上的节点数量。 说明:叶子节点是指没有子节点的节点。 示例 1: 输入:root = [3,9,20,null,null,15,7] 输出:2 示例 2: 输入:root = [2,null,3,null,4,null,5,null,6] 输出:5 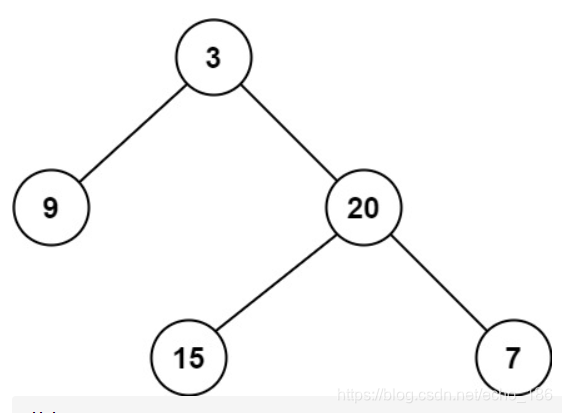
class Solution {
public:
int minDepth(TreeNode* root) {
if(root==nullptr)
{
return 0;
}
queue<TreeNode*> q;
q.push(root);
int depth=1;
while(!q.empty())
{
int size=q.size();
for(int i=0;i<size;i++)
{
TreeNode *cur=q.front();
q.pop();
if(cur->left==nullptr&&cur->right==nullptr)
{
return depth;
}
if(cur->left)
{q.push(cur->left);
}
if(cur->right)
{q.push(cur->right);
}
}
depth++;
}
return depth;
}
};
|