LoopQueue.h
#include<iostream>
#include <cassert>
using namespace std;
//template<class T>
//class SeqQueue;
//template<class T>
//
//ostream& operator<< (ostream& out, SeqQueue<T>& Q)//将友元函数声明在前可以避免其警告友元函数未声明
//{
// cout << "front = " << Q.front << ", rear = " << Q.rear << endl;
// if (!Q.IsEmpty())
// {
// int i = Q.front;
// while (i != Q.rear)
// {
// cout << Q.elements[i] << " ";
// i = (++i) % maxSize;
// }
// }
// return out;
//
//}
template <typename T>
class LoopQueue
{
private:
int front, rear;
T* data;
int MAXSIZE;
public:
LoopQueue(int size) {
front = rear = 0;
MAXSIZE = size;
this->data = new T[MAXSIZE];
assert(this->data != NULL);
}
~LoopQueue() {
delete[] data;
}
bool push(const T& x);//入队列
bool pop(T& x);//出队列
bool getFront(T& x);//找队头
void show();
//判空
bool IsEmpty()const {
return (this->rear == this->front) ? true : false;
}
//判满
bool IsFull()const {
return ((this->rear + 1) % this->MAXSIZE == this->front) ? true : false;
}
//求队长
int getSize()const {
return(this->rear - this->front + this->MAXSIZE) % this->MAXSIZE;
}
//friend ostream& operator<<<>(ostream& out, SeqQueue<T>& Q);
};
//入队列
template<typename T>
bool LoopQueue<T>::push(const T& x) {
if (!this->IsFull()) {
this->data[this->rear] = x;
this->rear = (this->rear + 1) % this->MAXSIZE;
return true;
}
return false;
}
//出队列
template<typename T>
bool LoopQueue<T>::pop(T& x) {
if (!this->IsEmpty()) {
x = this->data[this->front];
this->front = (this->front + 1) % this->MAXSIZE;
return true;
}
return false;
}
//返回队头
template<typename T>
bool LoopQueue<T>::getFront(T& x) {
if (!this->IsEmpty()) {
x = this->data[this->front];
return true;
}
return false;
}
template <typename T>
void LoopQueue<T>::show() {
if (!this->IsEmpty()) {
cout << "front = " << this->front << ", rear = " << this->rear << endl;
int i = this->front;
while (i != this->rear)
{
cout << this->data[i] << " ";
i = (++i) % this->MAXSIZE;
}
cout << endl;
}
}
testLoopQueue.cpp
#include "LoopQueue.h"
#include <iostream>
using namespace std;
int main() {
LoopQueue<int> Q(7);
for (int i = 7; i > 0; i--) {
Q.push(i);
}
Q.show();
int q = 0;
for (int i = 3; i >= 0; --i)
Q.pop(q);
Q.show();
cout << "Size = " << Q.getSize() << endl;
}
运行结果:
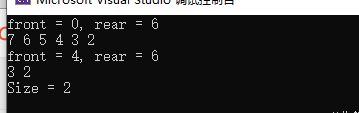
?
|