Given the root of a binary tree, return the same tree where every subtree (of the given tree) not containing a 1 has been removed.
A subtree of a node node is node plus every node that is a descendant of node.
Example 1: 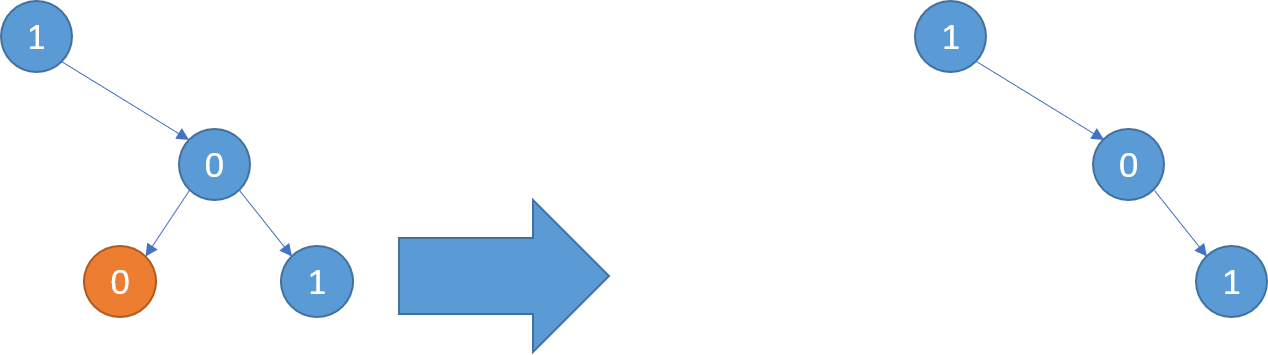
Input: root = [1,null,0,0,1] Output: [1,null,0,null,1] Explanation: Only the red nodes satisfy the property “every subtree not containing a 1”. The diagram on the right represents the answer. Example 2: 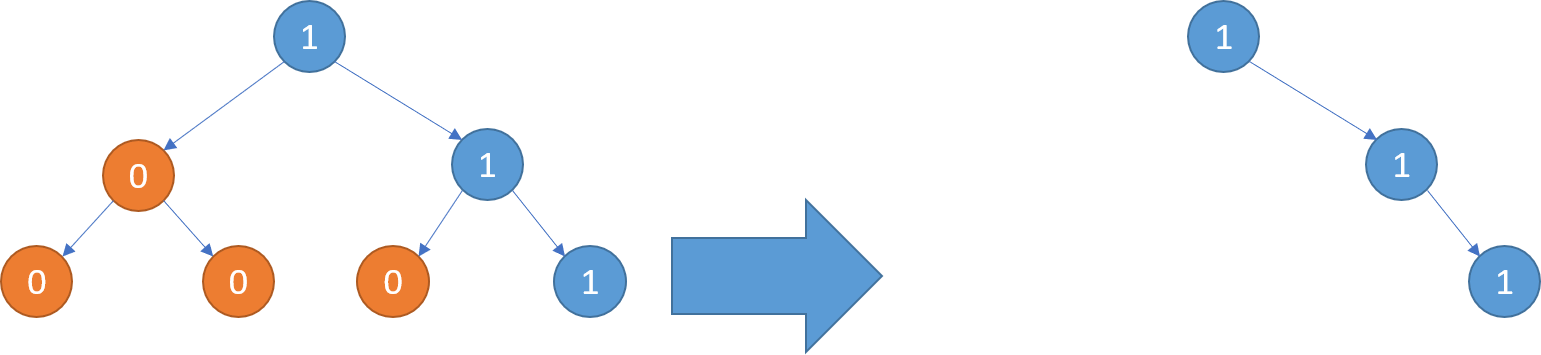
Input: root = [1,0,1,0,0,0,1] Output: [1,null,1,null,1] Example 3: 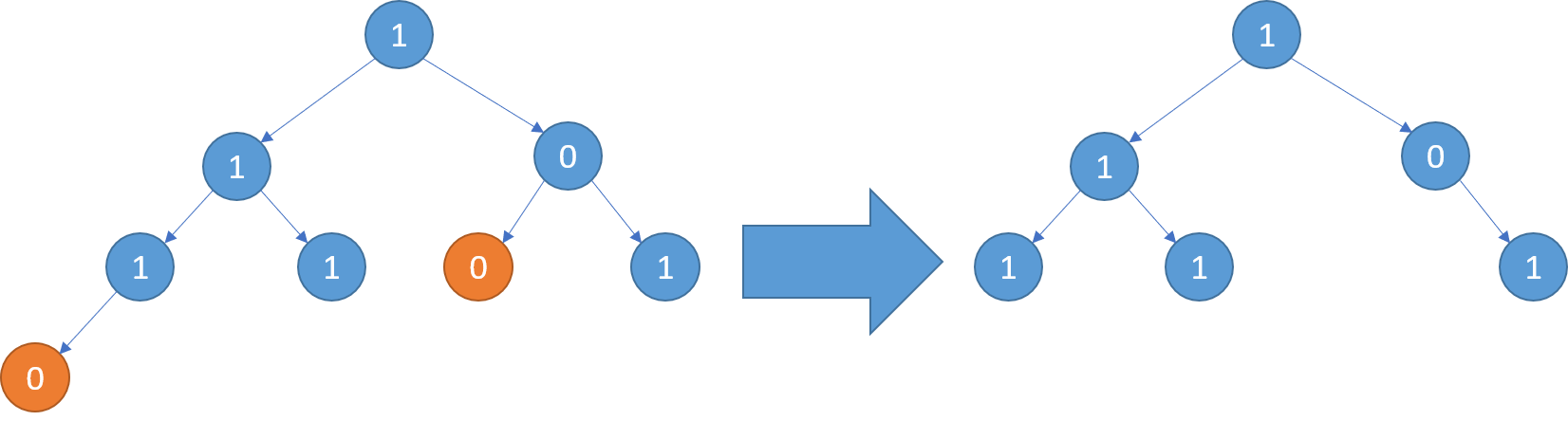
Input: root = [1,1,0,1,1,0,1,0] Output: [1,1,0,1,1,null,1]
Constraints:
The number of nodes in the tree is in the range [1, 200]. Node.val is either 0 or 1.
就两种情况
- 节点值是1的话,将左右节点进行筛选,然后返回当前节点
- 节点值是0的话,如果左右节点都是0,则返回空值,否则与节点值是1的情况相同
代码(Rust):
impl Solution {
fn rc(root: Option<Rc<RefCell<TreeNode>>>) -> Option<Rc<RefCell<TreeNode>>> {
if let Some(node) = root {
if node.borrow().val == 1 {
let mut left = node.borrow_mut().left.take();
let mut right = node.borrow_mut().right.take();
left = Solution::rc(left);
right = Solution::rc(right);
node.borrow_mut().left = left;
node.borrow_mut().right = right;
return Some(node);
} else {
let left = Solution::rc(node.borrow_mut().left.take());
let right = Solution::rc(node.borrow_mut().right.take());
if left.is_none() && right.is_none() {
return None;
}
node.borrow_mut().left = left;
node.borrow_mut().right = right;
return Some(node);
}
} else {
return None;
}
}
pub fn prune_tree(root: Option<Rc<RefCell<TreeNode>>>) -> Option<Rc<RefCell<TreeNode>>> {
Solution::rc(root)
}
}
|