二叉树遍历——先序遍历
遍历顺序如下图:依次遍历左孩子,到头了就转向右孩子 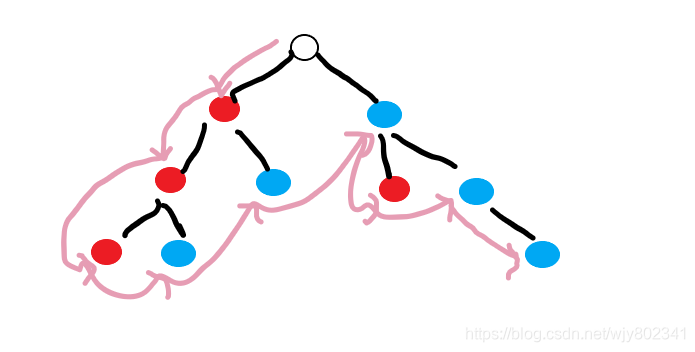
#include<iostream>
#include<stack>
using namespace std;
struct BT
{
int val;
BT* left;
BT* right;
};
struct Tree
{
BT *root;
};
void insert(Tree* tree, int val)
{
BT* temp, *newnode;
newnode = new BT;
newnode->val = val;
newnode->right = NULL;
newnode->left = NULL;
if (tree->root==NULL)
{
tree->root = newnode;
}
else
{
temp = tree->root;
while (NULL != temp)
{
if (temp->val<newnode->val)
{
if (temp->right==NULL)
{
temp->right = newnode;
return;
}
else
{
temp = temp->right;
}
}
else
{
if (temp->left == NULL)
{
temp->left = newnode;
return;
}
else
{
temp = temp->left;
}
}
}
}
}
void preorder(BT* ptr)
{
if (ptr != NULL)
{
std::cout << ptr->val << " ";
preorder(ptr->left);
preorder(ptr->right);
}
}
void preorder_1(BT* ptr)
{
stack<BT*>s;
s.push(ptr);
while (!s.empty())
{
BT* current;
current = s.top();
std::cout << "当前结点的值为: " << current->val << endl;
s.pop();
if(current->right!=NULL)s.push(current->right);
if (current->left != NULL)s.push(current->left);
}
}
void leftbranch(BT* ptr, stack<BT*>&s)
{
BT* current = ptr;
while (current!=NULL)
{
std::cout <<"当前节点为: " <<current->val;
if (current->right != NULL)s.push(current->right);
current = current->left;
}
}
void preorder_2(BT* ptr)
{
BT* current = ptr;
stack<BT*>st;
while (true)
{
leftbranch(current, st);
if (st.empty())break;
current = st.top();
st.pop();
}
}
int main()
{
Tree tree;
BT* b1 = new BT;
b1->val =10;
b1->left = new BT;
b1->left->val = 9;
b1->left->left = NULL;
b1->left->right = NULL;
b1->right = new BT;
b1->right->val = 11;
b1->right->left = NULL;
b1->right->right = NULL;
tree.root = b1;
preorder(tree.root);
std::cout << endl;
Tree* to_tree = &tree;
insert(to_tree, 20);
insert(to_tree, 7);
insert(to_tree, 10);
insert(to_tree, 34);
insert(to_tree, 13);
preorder_1(tree.root);
std::cout << endl;
preorder_2(tree.root);
std::cout << endl;
system("pause");
return 0;
}
|