链栈实验(链表实现)
用链式存储实现栈
头文件
#pragma once
struct Node {
Node* next;
int data;
};
class LinkStack
{
public:
LinkStack() { top = nullptr; };
~LinkStack();
void push(int x);
void pop();
int getTop();
bool empty();
private:
Node* top;
};
源文件
#include "LinkStack.h"
void LinkStack::push(int x) {
Node* p = new Node;
p->data = x;
p->next = top;
top = p;
}
void LinkStack::pop() {
if (top == nullptr)throw"下溢";
Node* p = nullptr;
p = top;
top = top->next;
delete p;
}
int LinkStack::getTop() {
return top->data;
}
bool LinkStack::empty() {
if (top == nullptr)return true;
return false;
}
LinkStack::~LinkStack() {
Node* p = nullptr;
while (p != nullptr) {
p = top;
top = top->next;
delete p;
}
};
测试程序
#include<iostream>
using namespace std;
#include"LinkStack.h"
int main() {
LinkStack st;
cout <<"判断栈是否为空:"<< st.empty()<<endl;
st.push(5);
st.push(2);
st.push(0);
cout << "取栈顶元素:" << st.getTop() << endl;
st.pop();
cout << "再取一次栈顶元素:" << st.getTop() << endl;
cout << "再次判断栈是否为空:" << st.empty() << endl;
}
测试结果
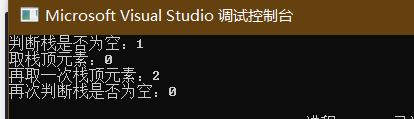
|