JZ5 用两个栈实现队列 链接: 题目链接.
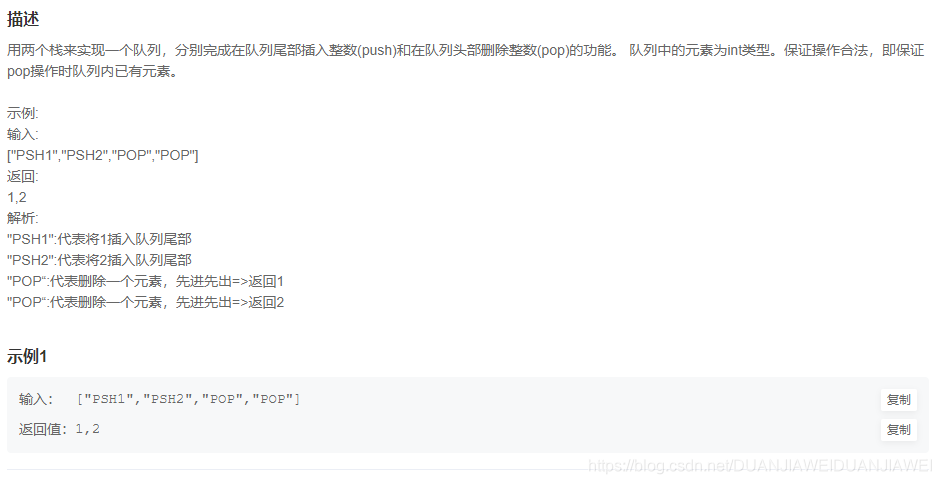
import java.util.Stack;
public class Solution {
Stack<Integer> stack1 = new Stack<Integer>();
Stack<Integer> stack2 = new Stack<Integer>();
public void push(int node) {
if(stack2.empty()){
stack2.push(node);
}else{
while(!stack2.empty()){
stack1.push(stack2.pop());
}
stack2.push(node);
while(!stack1.empty()){
stack2.push(stack1.pop());
}
}
}
public int pop() {
if(!stack2.empty()){
return stack2.pop();
}
return 0;
}
}
JZ6 旋转数组的最小数字 链接: 题目链接.
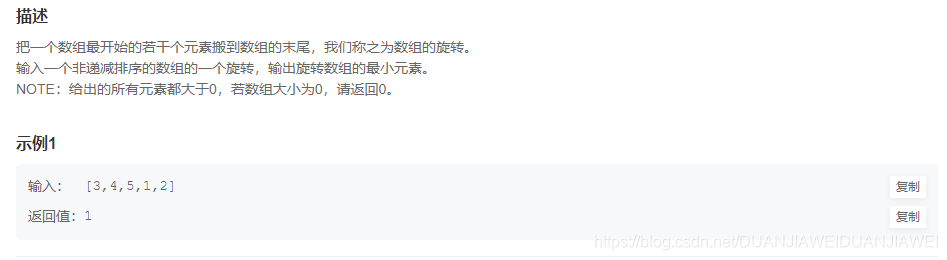 什么是非递减: 一共两种情况:
- 【1,2,3,4,5】
- 【1,2,3,3,4,5】
import java.util.ArrayList;
public class Solution {
public int minNumberInRotateArray(int [] array) {
if(array.length == 0){
return 0;
}
int left = 0;
int right = array.length-1;
//判断条件也可以改成:left != right
while(left < right){
if(array[left] < array[right]){
return array[left];
}
int mid = (left + right) >> 1;
if(array[mid] < array[right]){
right = mid;
}else if(array[mid] > array[right]){
left = mid + 1;
}else{
left++;
}
}
return array[right];
}
}
|