#include<iostream>
#define MaxSize 10
using namespace std;
typedef struct
{
int data[MaxSize];
}stack;
class Stack
{
private:
int top;
stack st;
public:
Stack()
{
top = -1;
}
void Push(int a[], int n)
{
for (int i = 0; i < n; ++i)
{
++top;
st.data[top] = a[i];
}
}
void Sort()//from the bottom of the stack to the top of the stack ,in descending order(从栈底到栈顶降序)
{
stack temp;
int highest=0;
int move= 0;
temp.data[move] = st.data[top];
cout<<"刚开始,直接将栈中的 "<<st.data[top]<<" 进入临时数组"<<endl;
--top;
int trip=0;//number of trips(趟数 )
while (top >= 0)
{
cout<<"第"<<++trip<<"趟"<<endl;
move= highest;
cout<<"重置移动位move= "<<move<<endl;
++highest;
cout<<"顶位加1为:"<<highest<<endl;
while(st.data[top] > temp.data[move]&&move>=0)
{
cout << "因为 " << st.data[top] << " > " << temp.data[move] << "临时数组" << temp.data[move] << "向上移" << endl;
temp.data[move+1] = temp.data[move];
--move;
}
if (move== -1)
{
cout<<"低位退至-1"<<"低位加1"<<"在0的位置插入"<<st.data[top]<<endl;
++move;
temp.data[move] = st.data[top];
--top;
}
else
{
cout<<"低位未退置负一跳出循环,表示低位所指的位置"<<temp.data[move]<<">"<<st.data[top]<<endl;
cout<<"低位加1插入"<<endl;
temp.data[move+1] = st.data[top];
--top;
}
}
for (int i =highest; i>=0; --i)
{
++top;
st.data[top] = temp.data[i];
}
}
void Pop()
{
for (; top >= 0; --top)
cout << st.data[top]<<'\t';
}
};
int main()
{
int a[6] = { 1,0,99,45,3,6 };
Stack s;
s.Push(a, 6);
s.Sort();
s.Pop();
}
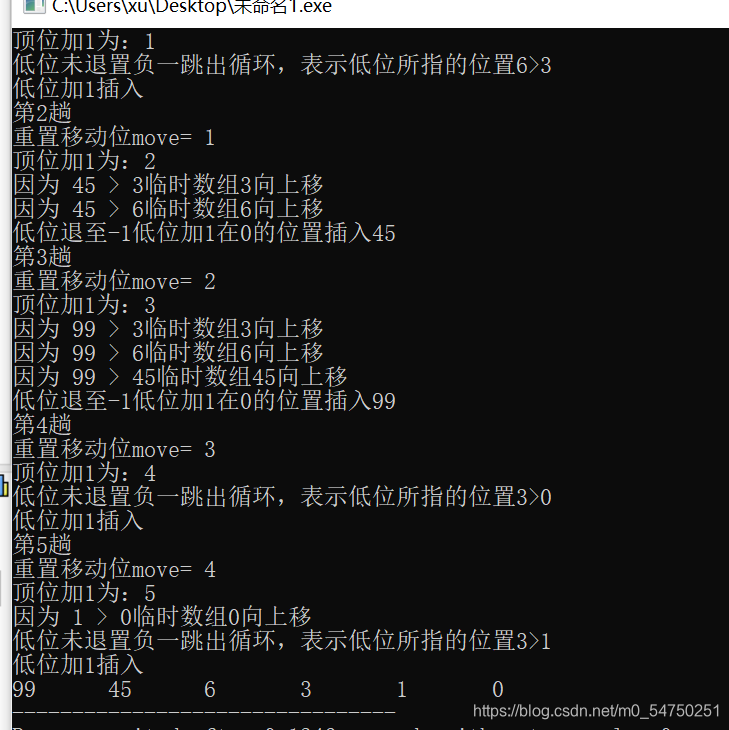
|