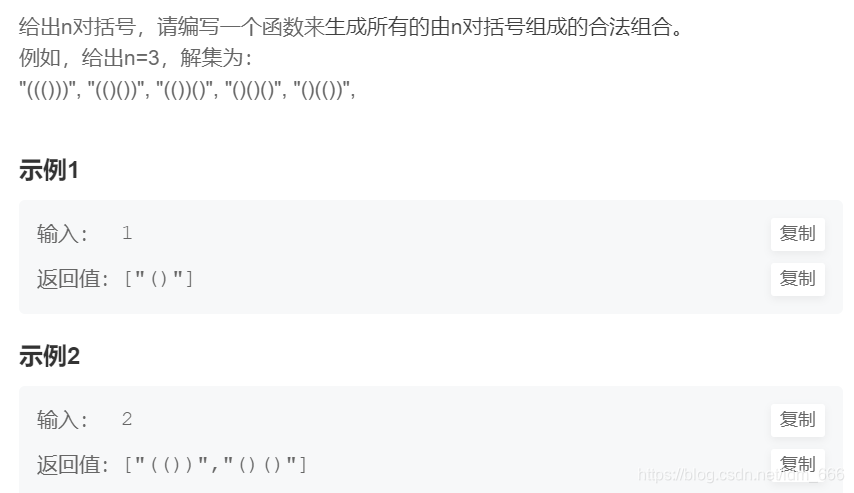
?
本题有两种解题思路,一种是回溯的思想,借助树来解释;另一种是动态规划思想。
详细解答稍后再写。
动态规划代码:
class Solution {
public:
/**
*
* @param n int整型
* @return string字符串vector
*/
vector<string> generateParenthesis(int n) {
// write code here
if (n == 0) return {};
if (n == 1) return { "()" };
vector<vector<string>> dp(n+1);
dp[0] = { "" };
dp[1] = { "()" };
for (int i = 2; i <= n; i++) {
for (int j = 0; j <i; j++) {
for (string p : dp[j])
for (string q : dp[i - j - 1]) {
string str = "(" + p + ")" + q;
dp[i].push_back(str);
}
}
}
return dp[n];
}
};
回溯代码:
class Solution {
public:
/**
*
* @param n int整型
* @return string字符串vector
*/
vector<string>res;
void dfs(string s,int lf,int rg,int n)
{
if(lf>n||rg>lf) return;
if(s.size()==2*n)
{
res.push_back(s);
return;
}
dfs(s+"(",lf+1,rg,n);
dfs(s+")",lf,rg+1,n);
}
vector<string> generateParenthesis(int n) {
// write code here
if(n==0) return res;
dfs("",0,0,n);
return res;
}
};
|