今天记录栈的相关学习
栈
栈的英文为(stack) 栈是一个先入后出(FILO-First In Last Out)的有序列表。 栈(stack)是限制线性表中元素的插入和删除只能在线性表的同一端进行的一种特殊线性表。 允许插入和删除的一端, 为变化的一端, 称为栈顶(Top), 另一端为固定的一端, 称为栈底(Bottom)。
栈的用处
- 子程序的调用: 在跳往子程序前, 会先将下个指令的地址存到堆栈中, 直到子程序执行完后再将地址取出, 以回到原来的程序中。
- 处理递归调用: 和子程序的调用类似, 只是除了储存下一个指令的地址外, 也将参数、 区域变量等数据存入堆栈中。
- 表达式的转换[中缀表达式转后缀表达式]与求值(实际解决)。
- 二叉树的遍历。
- 图形的深度优先(depth 一 first)搜索法。
数组进行模拟栈
package com.tunan.stack;
import java.util.Scanner;
public class ArrayStackDemo {
public static void main(String[] args) {
ArrayStack arrayStack = new ArrayStack(4);
String key = "";
boolean loop = true;
Scanner scanner = new Scanner(System.in);
while(loop) {
System.out.println("show: 显示栈");
System.out.println("exit: 退出栈");
System.out.println("push: 入栈");
System.out.println("pop: 出栈");
System.out.println("请输入你的选择");
key = scanner.next();
switch (key) {
case "show":
arrayStack.showStack();
break;
case "push":
System.out.println("请输入入栈参数");
int value = scanner.nextInt();
arrayStack.push(value);
break;
case "pop":
try {
int res = arrayStack.pop();
System.out.printf("出栈数据是%d \n", res);
} catch (Exception e) {
System.out.println(e.getMessage());
}
break;
case "exit":
scanner.close();
loop = false;
System.out.println("退出成功");
break;
default:
break;
}
}
}
}
class ArrayStack {
private int maxSize;
private int[] stack;
private int top = -1;
public ArrayStack(int maxSize) {
this.maxSize = maxSize;
stack = new int[maxSize];
}
public boolean isFull() {
return top == maxSize - 1;
}
public boolean isEmpty() {
return top == -1;
}
public void push(int val) {
if(isFull()) {
System.out.println("栈满,无法进行入栈操作");
return;
}
top++;
stack[top]=val;
}
public int pop() {
if(isEmpty()) {
throw new RuntimeException("栈空,无法进行出栈操作");
}
int res = stack[top];
top--;
return res;
}
public void showStack(){
if(isEmpty()) {
System.out.println("栈空");
return;
}
for (int j=top; j>-1;j--){
System.out.printf("stack[%d] = %d \n", j, stack[j]);
}
}
}
数组模拟栈的操作相对简单,因此这里给出一个数组模拟栈的更进一步的应用:
实现计算器操作
这里首先实现一个简单的中缀表达式计算操作,所谓的中缀,就是我们平时所见最多的表达式形式,比如 722-5+1-5+3-4/2
实现这样操作的思路是: 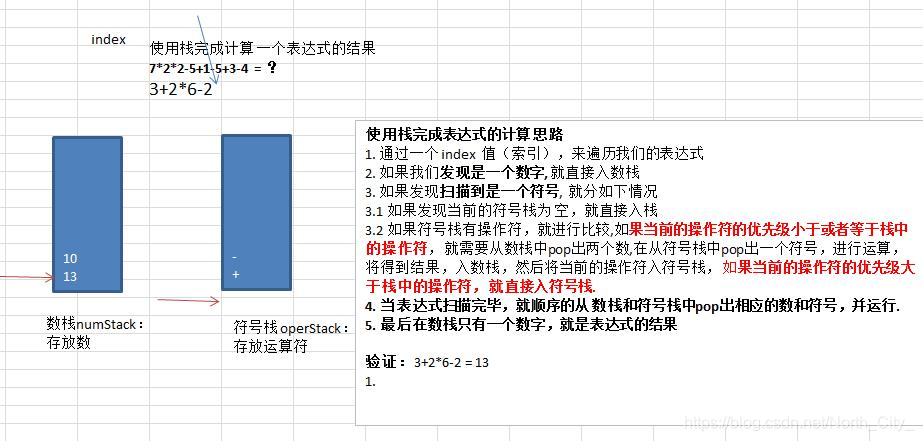
代码实现如下:
package com.tunan.stack;
public class Calculator {
public static void main(String[] args) {
String expression = "7*2*2-5+1-5+3-4/2";
calStack numStack = new calStack(10);
calStack operStack = new calStack(10);
int index = 0;
int num1 = 0;
int num2 = 0;
int oper = 0;
int res = 0;
char ch = ' ';
String keepNum = "";
while(true) {
ch = expression.substring(index, index+1).charAt(0);
if(operStack.isOper(ch)) {
if(!operStack.isEmpty()){
if(operStack.priority(ch) <= operStack.priority(operStack.peek())) {
num1 = numStack.pop();
num2 = numStack.pop();
oper = operStack.pop();
res = numStack.cal(num1, num2, oper);
numStack.push(res);
operStack.push(ch);
} else {
operStack.push(ch);
}
}else {
operStack.push(ch);
}
} else {
keepNum += ch;
if(index == expression.length() - 1){
numStack.push(Integer.parseInt(keepNum));
} else{
if(operStack.isOper(expression.substring(index+1, index+2).charAt(0))) {
numStack.push(Integer.parseInt(keepNum));
keepNum = "";
}
}
}
index++;
if(index >= expression.length()) {
break;
}
}
while(true) {
if(operStack.isEmpty()){
break;
}
num1 = numStack.pop();
num2 = numStack.pop();
oper = operStack.pop();
res = numStack.cal(num1, num2, oper);
numStack.push(res);
}
System.out.printf("表达式 %s = %d\n", expression, numStack.pop());
}
}
class calStack {
private int maxSize;
private int[] stack;
private int top = -1;
public calStack(int maxSize) {
this.maxSize = maxSize;
stack = new int[maxSize];
}
public int peek() {
if(isEmpty()) {
throw new RuntimeException("栈空,无法进行出栈操作");
}
int res = stack[top];
return res;
}
public boolean isFull() {
return top == maxSize - 1;
}
public boolean isEmpty() {
return top == -1;
}
public void push(int val) {
if(isFull()) {
System.out.println("栈满,无法进行入栈操作");
return;
}
top++;
stack[top]=val;
}
public int pop() {
if(isEmpty()) {
throw new RuntimeException("栈空,无法进行出栈操作");
}
int res = stack[top];
top--;
return res;
}
public void showStack(){
if(isEmpty()) {
System.out.println("栈空");
return;
}
for (int j=top; j>-1;j--){
System.out.printf("stack[%d] = %d \n", j, stack[j]);
}
}
public int priority(int oper) {
if(oper == '*' || oper == '/'){
return 1;
} else if(oper == '+' || oper == '-') {
return 0;
} else {
return -1;
}
}
public boolean isOper(char val) {
return val == '+' || val == '-' || val == '*' || val == '/';
}
public int cal(int num1, int num2, int oper){
int res = 0;
switch (oper) {
case '+':
res = num1 + num2;
break;
case '-':
res = num2 - num1;
break;
case '*':
res = num1 * num2;
break;
case '/':
res = num2 / num1;
break;
default:
break;
}
return res;
}
}
这里可以看出计算过程有一些繁琐,这也是中缀表达式的短板,虽然有利于人们去读,但对计算机却并不友好,并且上面的程序没有考虑到括号的情况。
下一节我将继续介绍前缀、中缀和后缀的表达,以及实现逆波兰的表达。
|